Troubleshooting Common Deployment Issues in Spring Boot Apps
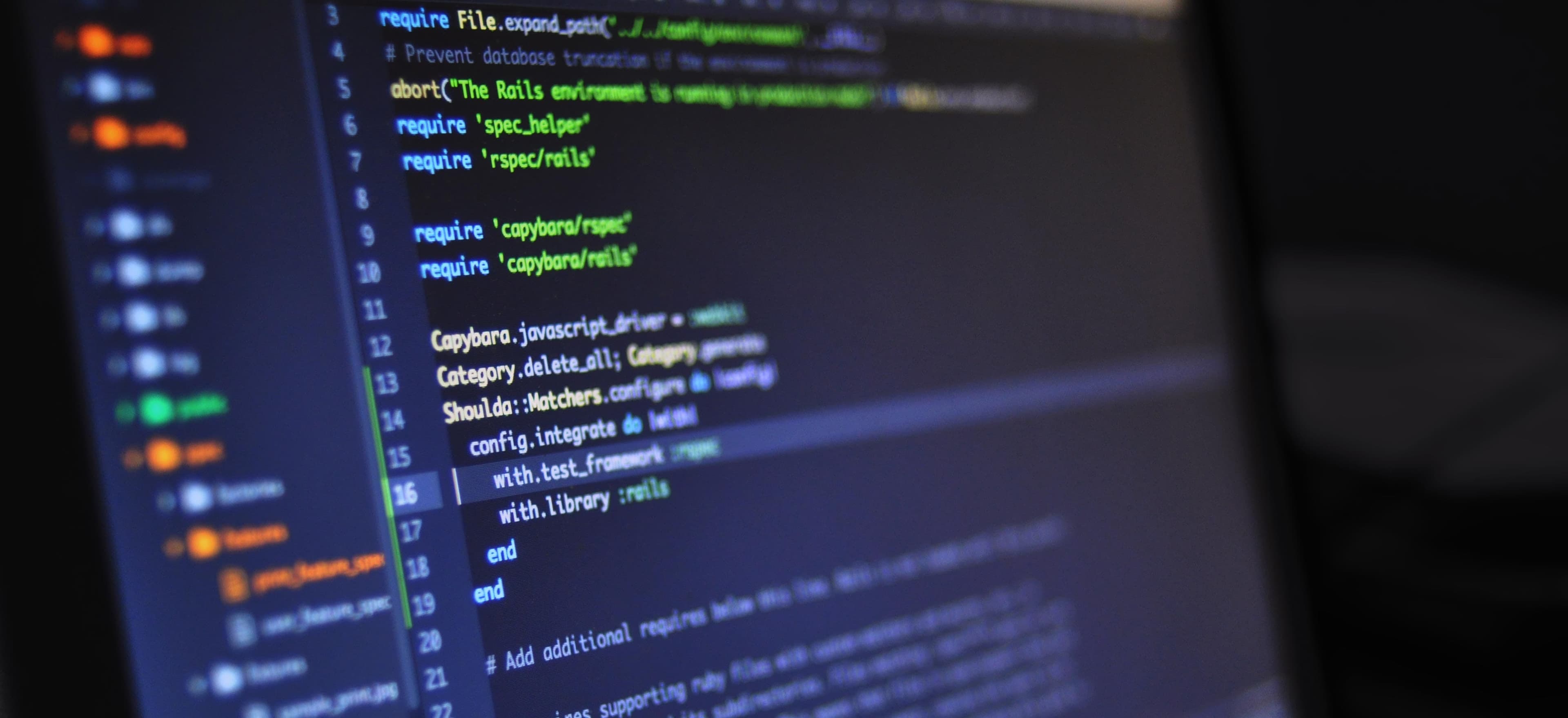
- Published on
Troubleshooting Common Deployment Issues in Spring Boot Apps
Spring Boot has revolutionized the way developers build Java applications by offering a simplified model for creating production-ready applications. However, even with its exceptional ease of use, deployment issues can arise. In this blog post, we’ll explore common deployment problems in Spring Boot applications and how you can resolve them effectively.
Understanding Common Deployment Issues
Before diving into solutions, it's essential to recognize the typical deployment issues developers face. The most common challenges include:
- Environment Configuration Problems
- Dependency Conflicts
- Database Connectivity Issues
- Insufficient Memory Allocation
- Application Port Conflicts
- Incorrect Build Artifact
Let’s explore each of these in detail.
1. Environment Configuration Problems
Issue
Environment misconfigurations can lead to various runtime exceptions. This is often due to differences between development and production environments. A frequently missed configuration aspect is application.properties
or application.yml
.
Solution
Ensure that environment-specific properties are defined correctly. For instance, in application-production.properties
, you might have:
spring.datasource.url=jdbc:mysql://production-db:3306/mydb
spring.datasource.username=prod_user
spring.datasource.password=securepassword
Why This Matters
Inconsistencies in environment variables between your local setup and production can lead to connectivity issues or application failures.
Additional Reading
For detailed configuration guides, refer to the Spring Boot Configuration Documentation.
2. Dependency Conflicts
Issue
Spring Boot simplifies dependency management through the spring-boot-starter
packages. However, using incompatible or conflicting versions can lead to the infamous NoSuchBeanDefinitionException
.
Solution
Always manage dependencies through Maven
or Gradle
. Use the dependency:tree
command in Maven to identify conflicts:
mvn dependency:tree
Code Example
The following Maven snippet will help you exclude a problematic dependency:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<exclusions>
<exclusion>
<groupId>org.glassfish</groupId>
<artifactId>javax.el</artifactId>
</exclusion>
</exclusions>
</dependency>
Why This Matters
Managing dependencies correctly is crucial; incompatible versions can lead to runtime exceptions that are difficult to trace.
3. Database Connectivity Issues
Issue
Database connectivity is fundamental. Spring Boot applications often throw SQLSyntaxErrorException
if the database schema is not compatible or the URL is misconfigured.
Solution
Ensure that your database driver is included in your dependencies, and double-check your connection string. Here's an exemplary property configuration:
spring.datasource.url=jdbc:mysql://localhost:3306/mydb?useSSL=false
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
spring.jpa.hibernate.ddl-auto=update
Why This Matters
Database connection parameters must be accurate; a single character error in your URL can cause a complete application failure.
Additional Reading
Explore more about Spring Data JPA for advanced database connection scenarios.
4. Insufficient Memory Allocation
Issue
Spring Boot applications can consume significant memory, especially with large datasets. An OutOfMemoryError can crash your application.
Solution
Configure your Java application with appropriate memory settings. You can set these parameters in your JAVA_OPTS
environment variable:
export JAVA_OPTS="-Xms512m -Xmx1024m"
Why This Matters
Memory allocation affects performance directly. Insufficient memory will lead to application crashes, impacting user experience.
5. Application Port Conflicts
Issue
Applications listen on network ports to provide services. If two applications use the same port, you'll face PortAlreadyInUseException
.
Solution
Change your application's port in application.properties
:
server.port=8081
Why This Matters
Port conflicts can easily arise, especially in development environments with multiple microservices.
Additional Reading
For an overview of Spring Boot's embedded server options, check out the Spring Boot Web Application Documentation.
6. Incorrect Build Artifact
Issue
Deploying an incorrect artifact can create unexpected behaviors. This can happen when multiple builds are done and older versions overwrite the newer ones.
Solution
Always verify the build artifacts in your CI/CD pipeline. Use specific tags or version numbers to ensure you're deploying the correct version:
mvn clean package -DskipTests
Why This Matters
Deploying incorrect artifacts can lead to functionality that either doesn’t exist or performs unexpectedly.
Additional Reading
For CI/CD best practices, review the Spring Boot DevOps Guide.
Lessons Learned
Deployment issues in Spring Boot applications can be daunting, but with a systematic approach, most can be resolved swiftly. From environment configurations to dependency management, understanding these common problems will empower you as a Spring Boot developer.
Next Steps
For a comprehensive understanding, be sure to explore the official Spring Boot documentation and engage with the community. Troubleshooting is part of the learning process—stay persistent!
If you found this post useful, consider sharing it with your peers or checking out other resources on Java and Spring Boot development. Remember, even the best frameworks have challenges; it's how we tackle them that counts!