How to Tackle Errors with the Optional Type API
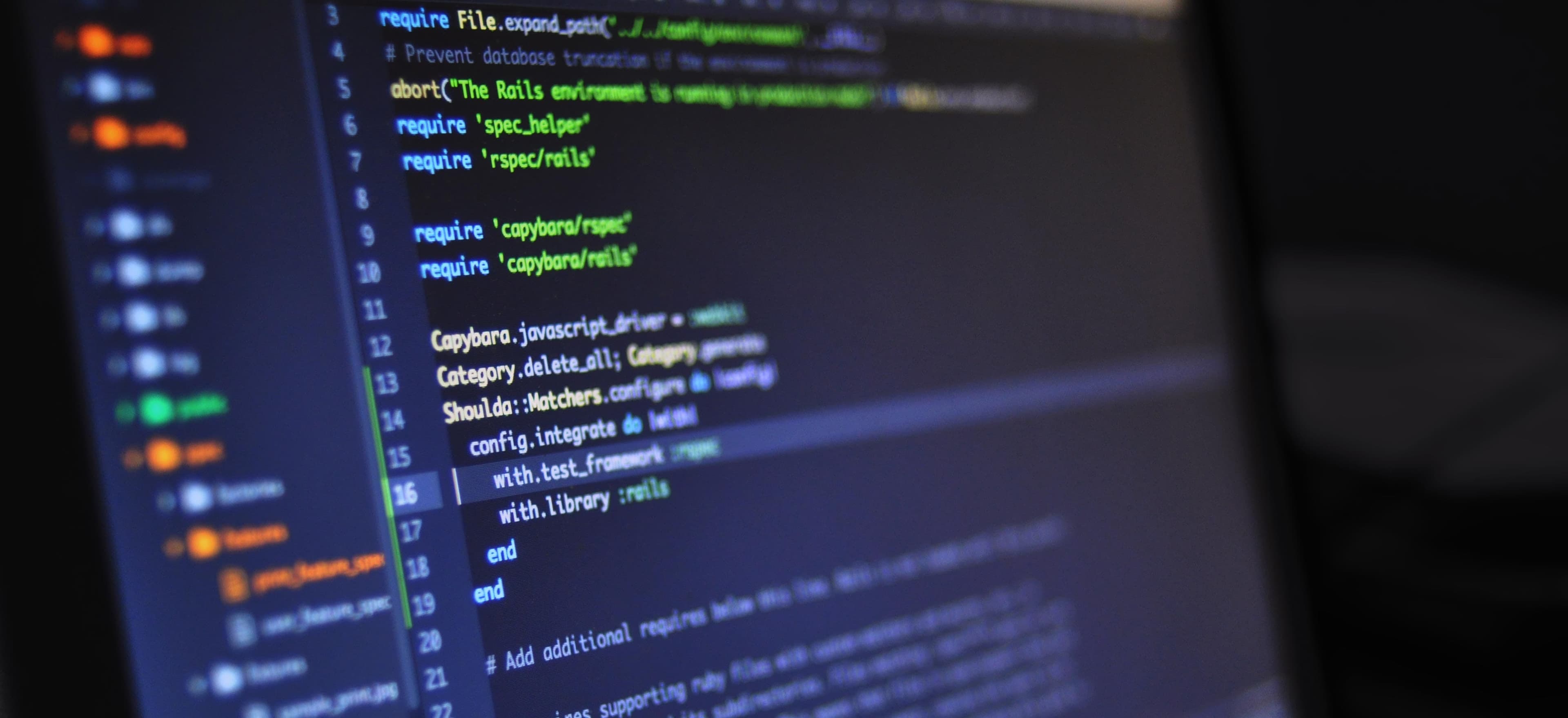
- Published on
How to Tackle Errors with the Optional Type API in Java
Java is known for its robust error handling, but traditional error handling strategies can make code tedious and sometimes confusing. The introduction of the Optional type in Java 8 offers a powerful alternative to null checks, reducing the occurrence of NullPointerExceptions and promoting a clearer intention in code. In this article, we will explore the Optional type API, its advantages, and how it can help programmers manage errors effectively.
Understanding Optional
The Optional<T>
class is a container object which may or may not contain a non-null value. This encapsulation provides a way to express that a value may be absent without needing to resort to null references.
Here is how you can declare an Optional variable:
Optional<String> optionalString = Optional.of("Hello, World!");
Why Use Optional?
-
Type Safety: Unlike using null, Optional explicitly states that a value may be absent, promoting clearer code.
-
Chaining: Optional comes with several methods, which allow for chaining operations that can be performed on values.
-
Better Code Readability: Using an Optional object makes your intention clear to others reading your code.
-
Avoiding Null References: Reduces potential NullPointerExceptions, making your code cleaner and safer.
Creating Optionals
The Optional class provides several static methods to create instances:
Optional.of(T value)
: Creates an Optional with a non-null value.Optional.ofNullable(T value)
: Creates an Optional that may or may not contain a value (can be null).Optional.empty()
: Returns an empty Optional.
Example of Creating Optionals
Let's look at how to create Optionals:
String value1 = "Hello, Java";
Optional<String> opt1 = Optional.of(value1); // Non-null value
Optional<String> opt2 = Optional.ofNullable(null); // Can be empty
Optional<String> opt3 = Optional.empty(); // Empty Optional
Why Use Optional.ofNullable
?
Using Optional.ofNullable(value)
can be particularly useful when dealing with data that may not always be present, such as user input, database records, or API responses.
Accessing Values in Optional
Once you have an Optional object, you will often want to access its value. However, it is crucial to do so in a safe manner.
Retrieve Value Safely
You can retrieve a value from an Optional in multiple ways:
- Using
isPresent()
: Check if a value is present before accessing it. - Using
ifPresent()
: Perform an action if a value is present. - Using
get()
: Retrieve the value directly, but throws an exception if not present. (Use sparingly) - Using
orElse(T other)
: Provide a default value if the optional is empty. - Using
orElseGet(Supplier<? extends T> other)
: Provide a dynamic default value through a Supplier. - Using
orElseThrow(Supplier<? extends X> exceptionSupplier)
: Throw an exception if no value is present.
Code Example
Optional<String> optionalValue = Optional.ofNullable(null);
// Accessing the value safely
if (optionalValue.isPresent()) {
System.out.println("Value: " + optionalValue.get());
} else {
System.out.println("No value present");
}
// Using orElse
String result = optionalValue.orElse("Default Value");
System.out.println("Result: " + result); // Will print "Default Value"
// Using orElseGet
String dynamicResult = optionalValue.orElseGet(() -> "Dynamic Default Value");
System.out.println("Dynamic Result: " + dynamicResult); // Will print "Dynamic Default Value"
// Using orElseThrow
try {
String value = optionalValue.orElseThrow(() -> new IllegalArgumentException("Value is absent"));
} catch (IllegalArgumentException e) {
System.out.println(e.getMessage()); // Will print "Value is absent"
}
Why Use orElse
and orElseGet
?
Using orElse
provides a simple fallback default value, while orElseGet
is more efficient since it only computes the fallback value if needed.
Transforming Values
Optional also allows us to transform values in a type-safe and null-safe manner.
Using map()
The map
function can be used to apply a function if a value is present.
Optional<String> optionalName = Optional.of("John");
Optional<Integer> nameLength = optionalName.map(String::length);
nameLength.ifPresent(length -> System.out.println("Name Length: " + length));
Why Use map()
?
This method helps you avoid null checks, providing a clean way to handle transformations.
Using flatMap()
Sometimes, you may need the function to return an Optional itself. In this case, use flatMap()
.
Optional<String> optionalEmail = Optional.of("user@example.com");
Optional<String> domain = optionalEmail.flatMap(email -> {
if (email.contains("@")) {
return Optional.of(email.split("@")[1]);
} else {
return Optional.empty();
}
});
domain.ifPresent(d -> System.out.println("Domain: " + d)); // Will print "Domain: example.com"
When to Use flatMap()
?
Use flatMap()
when the function you are applying returns an Optional, allowing you to chain multiple operations without nesting.
Filtering Values
You can filter the Optional value based on a condition using the filter()
method.
Optional<String> optionalUsername = Optional.of("Admin");
Optional<String> filteredUsername = optionalUsername.filter(user -> user.length() > 5);
filteredUsername.ifPresent(user -> System.out.println("Valid Username: " + user)); // No output
Why Use filter()
?
This method allows you to impose additional conditions on your Optional values, simplifying the error handling in your application.
To Wrap Things Up
The Optional type API in Java provides a powerful mechanism to handle errors and reduce the likelihood of NullPointerExceptions. By encapsulating the presence or absence of a value, it encourages better programming practices.
Key Takeaways
- Utilize
Optional
to signify nullable values clearly. - Use
map()
,flatMap()
, andfilter()
for safe and effective transformations. - Rely on
orElse()
,orElseGet()
, andorElseThrow()
to manage defaults and error cases efficiently.
For further reading on error handling in Java, you can refer to Java Optional Documentation.
By mastering the Optional type API, you’ll enhance your code's reliability and maintainability, paving the way for a more sustainable coding approach in your Java projects.
Checkout our other articles