Mastering Java Concurrency: Overcoming CyclicBarrier Pitfalls
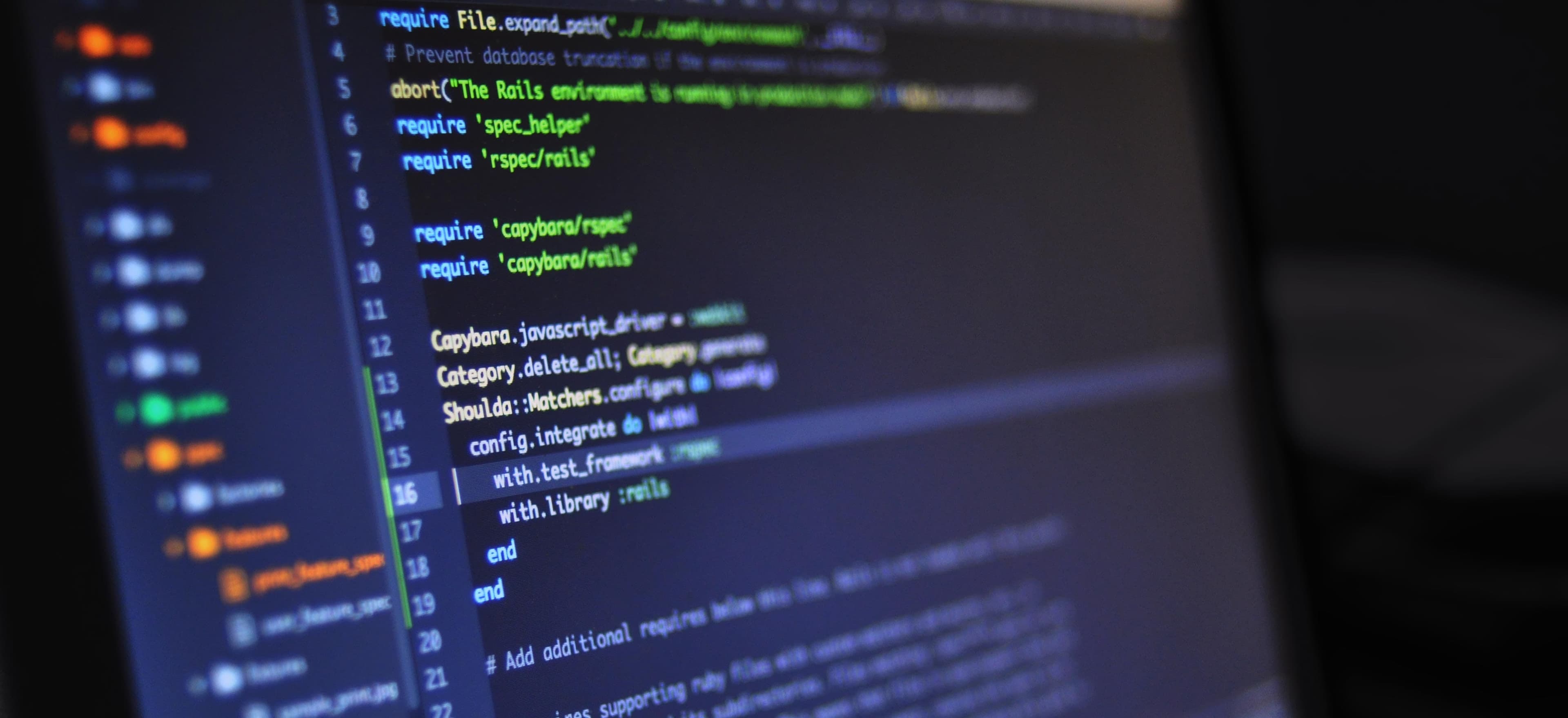
- Published on
Mastering Java Concurrency: Overcoming CyclicBarrier Pitfalls
Java concurrency is a critical topic for developers who strive to write efficient, high-performance applications. With the rise of multi-core processors and distributed systems, understanding how to manage threads effectively is more important than ever. Among the features Java offers to facilitate thread management are classes like CyclicBarrier
. While powerful, CyclicBarrier
can sometimes lead to pitfalls if not correctly implemented. In this guide, we'll explore these potential issues and how to effectively use CyclicBarrier
.
Understanding CyclicBarrier
Before diving into pitfalls, it's essential to understand what CyclicBarrier
is and how it works.
What is CyclicBarrier?
A CyclicBarrier
is a synchronization aid that allows a set of threads to all wait for each other to reach a common barrier point. Once all participating threads have reached the barrier, they are released to continue their execution. This feature is particularly useful in scenarios where a group of threads needs to coordinate their execution—such as in simulations or parallel algorithms.
You can create a CyclicBarrier
instance by specifying the number of parties (threads) that must wait at the barrier before any of them can continue. Here is how to create a simple CyclicBarrier
:
import java.util.concurrent.CyclicBarrier;
public class Example {
public static void main(String[] args) {
int numberOfThreads = 3;
CyclicBarrier barrier = new CyclicBarrier(numberOfThreads, () -> {
System.out.println("All parties have arrived at the barrier.");
});
}
}
In the code snippet above, we are creating a CyclicBarrier
with three parties. When all three threads reach the barrier, the specified action gets executed, printing a message to the console.
Example Scenario
Imagine a scenario where three threads perform different calculations but need to synchronize their results before moving on to the next stage of computation. The CyclicBarrier
allows them to wait for each other effectively.
Common Pitfalls with CyclicBarrier
While CyclicBarrier
can significantly simplify thread coordination, it can also introduce complexities if mismanaged. Here are some common pitfalls and how to avoid them.
1. Timing Issues
Problem: It can happen that one or more threads reach the barrier but are delayed because of processing time, which halts the execution of all threads at the barrier.
Solution: Ensure the barrier's reset mechanism is well understood. Once the barrier is reached, if any thread experiences an extended delay, it can cause others to wait indefinitely.
import java.util.concurrent.CyclicBarrier;
public class TimingIssue {
public static void main(String[] args) {
int numberOfThreads = 2;
CyclicBarrier barrier = new CyclicBarrier(numberOfThreads, () -> {
System.out.println("Barrier reached!");
});
Runnable task = () -> {
try {
// Simulating some work
Thread.sleep((long) (Math.random() * 1000));
System.out.println(Thread.currentThread().getName() + " has reached the barrier.");
barrier.await();
} catch (Exception e) {
e.printStackTrace();
}
};
Thread t1 = new Thread(task);
Thread t2 = new Thread(task);
t1.start();
t2.start();
}
}
In this code, two threads simulate variable processing time before reaching the barrier. This approach can lead to inefficiencies if timing doesn’t align.
2. Thread Interruption
Problem: If a thread is interrupted while waiting at the barrier, it can lead to sudden undesired behavior. When a thread calls await()
, it may throw an InterruptedException
.
Solution: Always handle interruptions gracefully. Consider providing fallback actions if a thread is interrupted.
import java.util.concurrent.CyclicBarrier;
public class InterruptionExample {
public static void main(String[] args) {
CyclicBarrier barrier = new CyclicBarrier(2);
Runnable task = () -> {
try {
System.out.println(Thread.currentThread().getName() + " is waiting at the barrier.");
barrier.await();
System.out.println(Thread.currentThread().getName() + " has crossed the barrier.");
} catch (InterruptedException e) {
System.out.println(Thread.currentThread().getName() + " was interrupted.");
} catch (Exception e) {
e.printStackTrace();
}
};
Thread thread = new Thread(task);
thread.start();
try {
Thread.sleep(100);
thread.interrupt(); // Interrupting the waiting thread
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
In this example, a thread is intentionally interrupted, showcasing how to manage interruptions properly. Using a try-catch block ensures that we acknowledge interruptions without crashing the application.
3. Avoiding Deadlocks
Problem: A deadlock can occur if threads do not properly move past the barrier due to incorrect or unexpected behavior.
Solution: Identify all potential paths to the barrier and ensure that every thread can reach it. Testing edge cases where one or more threads might fail or be delayed can help.
4. Additional Complexity with Reuse
Problem: CyclicBarrier
can be reused once its waiting parties have been released. However, if a CyclicBarrier
instance is reused improperly, it may lead to unexpected results.
Solution: Make sure you manage the reuse of barriers carefully and reset them when necessary.
import java.util.concurrent.CyclicBarrier;
public class ReuseExample {
public static void main(String[] args) {
CyclicBarrier barrier = new CyclicBarrier(3);
Runnable task = () -> {
try {
barrier.await();
System.out.println(Thread.currentThread().getName() + " has crossed the barrier.");
} catch (Exception e) {
e.printStackTrace();
}
};
for (int i = 0; i < 3; i++) {
new Thread(task).start();
}
// Resetting the barrier after use to avoid issues
try {
barrier.await(); // Wait for all to finish
barrier.reset(); // Resetting for future use
} catch (Exception e) {
e.printStackTrace();
}
}
}
5. Performance Concerns
Problem: Overuse of barriers can severely impact performance, especially if not all threads utilize the barrier adequately.
Solution: Limit the usage of CyclicBarrier
to necessary situations. Monitor performance metrics and adjust concurrency levels accordingly.
Wrapping Up
Cyclic barriers are a powerful component of multi-threading in Java, allowing threads to synchronize effectively. However, developers should be cautious about common pitfalls such as timing issues, thread interruptions, deadlocks, and complexity in reuse. By following best practices and being aware of these pitfalls, you can harness the full potential of CyclicBarrier
while maintaining performance and robustness in your Java applications.
For further reading on Java concurrency, consider checking these informative resources:
- Java Concurrency in Practice
- Java Documentation on CyclicBarrier
By mastering concurrency in Java, you’ll position yourself to develop more efficient and high-performing applications. Happy coding!
Checkout our other articles