Top Strategies to Fix Common Application Security Vulnerabilities
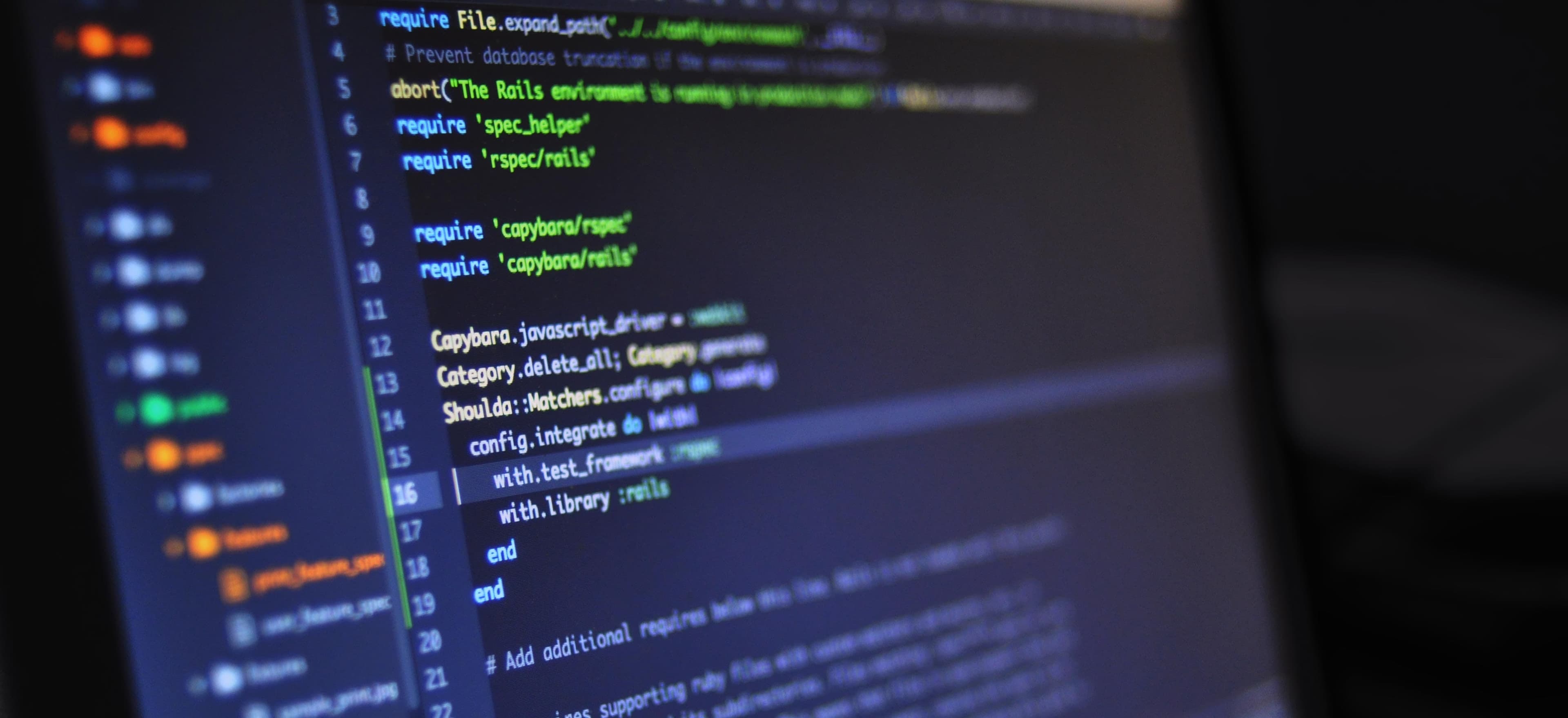
- Published on
Top Strategies to Fix Common Application Security Vulnerabilities
In today's rapidly evolving digital landscape, securing applications has become more critical than ever. Vulnerabilities can lead to massive data breaches, loss of user trust, and significant financial repercussions. This post highlights top strategies to fix common application security vulnerabilities and implements best practices designed to help developers build secure applications.
Understanding Application Security Vulnerabilities
Application security vulnerabilities are weaknesses in software or hardware that could be exploited by attackers. They can stem from various sources, including flawed code, misconfigurations, and inadequate monitoring processes. Common types of vulnerabilities include:
- Injection Flaws: Such as SQL injection or command injection.
- Cross-Site Scripting (XSS): Where attackers inject malicious scripts into trusted websites.
- Authentication Failures: E.g., poor password policies.
- Sensitive Data Exposure: Insufficient protection of confidential data.
According to the OWASP Top Ten, the top vulnerabilities to watch out for include:
- Injection
- Broken Authentication
- Sensitive Data Exposure
- XML External Entities (XXE)
- Broken Access Control
- Security Misconfiguration
- Cross-Site Scripting (XSS)
- Insecure Deserialization
- Using Components with Known Vulnerabilities
- Insufficient Logging & Monitoring
Strategy 1: Input Validation and Output Encoding
Understanding the Importance
Input validation ensures that the data your application accepts is both safe and as expected. By validating input, you can prevent injection flaws and XSS attacks. Likewise, output encoding ensures that data transmitted back to users is safely rendered, thus defending against XSS.
Code Example with Commentary
Here's an example of input validation in Java that checks for valid usernames:
public boolean isValidUsername(String username) {
// Regex limiting usernames to 4-15 alphanumeric characters
return username.matches("^[a-zA-Z0-9]{4,15}$");
}
Why This Matters: The regex pattern restricts the username format, preventing attempts to inject malicious scripts or characters. Always use input validation on the server-side, regardless of client-side checks.
Strategy 2: Implementing Parameterized Queries
Understanding the Importance
Parameterized queries are key in mitigating SQL injection vulnerabilities. This practice ensures that user input is treated as data, not code.
Code Example with Commentary
Below is a parameterized query using JDBC:
String sql = "SELECT * FROM users WHERE email = ?";
try (PreparedStatement stmt = connection.prepareStatement(sql)) {
stmt.setString(1, userEmail); // userEmail is user input
ResultSet rs = stmt.executeQuery();
}
Why This Matters: By using PreparedStatement
, we separate SQL code from data input. This prevents attackers from injecting SQL commands through user input.
Strategy 3: Strong Authentication Mechanisms
Understanding the Importance
Implementing strong authentication policies not only protects sensitive data but also drastically reduces the risk of unauthorized access.
Key Practices
- Use multifactor authentication (MFA) to add another layer of security.
- Implement strong password policies (minimum length, complexity, expiration).
- Ensure account lockout mechanisms on repeated failed login attempts.
Code Implementation for Password Policy
To enforce a strong password policy, validate passwords during registration:
public boolean isValidPassword(String password) {
// Password must contain at least one uppercase, one lowercase, one digit, and be 8-20 characters long
return password.matches("^(?=.*[a-z])(?=.*[A-Z])(?=.*\\d)[A-Za-z\\d]{8,20}$");
}
Why This Matters: By enforcing a strong password policy, you help protect users' accounts from being easily compromised.
Strategy 4: Encrypt Sensitive Data
Understanding the Importance
Sensitive data exposure is one of the most prevalent issues. Encrypting sensitive data, both in transit and at rest, is crucial.
Code Example to Implement AES Encryption
Here’s how to encrypt data using AES in Java:
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
public byte[] encryptData(String data) throws Exception {
SecretKey secretKey = KeyGenerator.getInstance("AES").generateKey();
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
return cipher.doFinal(data.getBytes());
}
Why This Matters: This code snippet demonstrates secure data encryption. Encrypting sensitive data protects it from unauthorized access, even if a breach occurs.
Strategy 5: Regular Security Testing
Understanding the Importance
Regular security testing helps identify vulnerabilities before they can be exploited. This can include dynamic and static application testing (DAST/SAST).
Tools for Regular Testing
- OWASP ZAP: A free and open-source web application security scanner.
- Burp Suite: A popular tool for testing web application security.
Integration into CI/CD Pipeline
Integrating security testing into your Continuous Integration/Continuous Deployment (CI/CD) pipeline will ensure that security tests are run automatically.
# Sample YAML configuration for integrating OWASP ZAP with Jenkins
pipelines {
agent any
stages {
stage('Build') {
steps {
script {
// Your build code goes here
}
}
}
stage('Test') {
steps {
sh 'zap.sh -cmd -quickurl http://your-app-url -quickout zap_report.html'
}
}
}
}
Why This Matters: Automating security tests in your CI/CD pipelines ensures that vulnerabilities are caught early, keeping your applications secure from the very beginning.
Strategy 6: Logging and Monitoring
Understanding the Importance
Effective logging and monitoring of applications help track incidents and ensure that response strategies can be executed promptly.
Code Example for Logging
Example of using logging in a Java application:
import java.util.logging.Logger;
public class ApplicationLogger {
private static final Logger logger = Logger.getLogger(ApplicationLogger.class.getName());
public void logUserAction(String action) {
logger.info("User action logged: " + action);
}
}
Why This Matters: By logging significant user actions, you can have an audit trail that helps identify malicious activity, ultimately aiding in quicker response to incidents.
Final Thoughts
Security vulnerabilities threaten applications across the board. Employing these strategies—effective input validation, parameterized queries, strong authentication mechanisms, data encryption, regular security testing, and comprehensive logging—will significantly reduce the risk of exploitation.
By being proactive in building secure applications, organizations can protect sensitive data and maintain user trust. For more details on implementing security measures, check out the OWASP Cheat Sheet Series.
Invest time in application security today and safeguard your digital landscape for tomorrow.
Checkout our other articles