Boosting Performance: Common Pitfalls of Singleton Streams
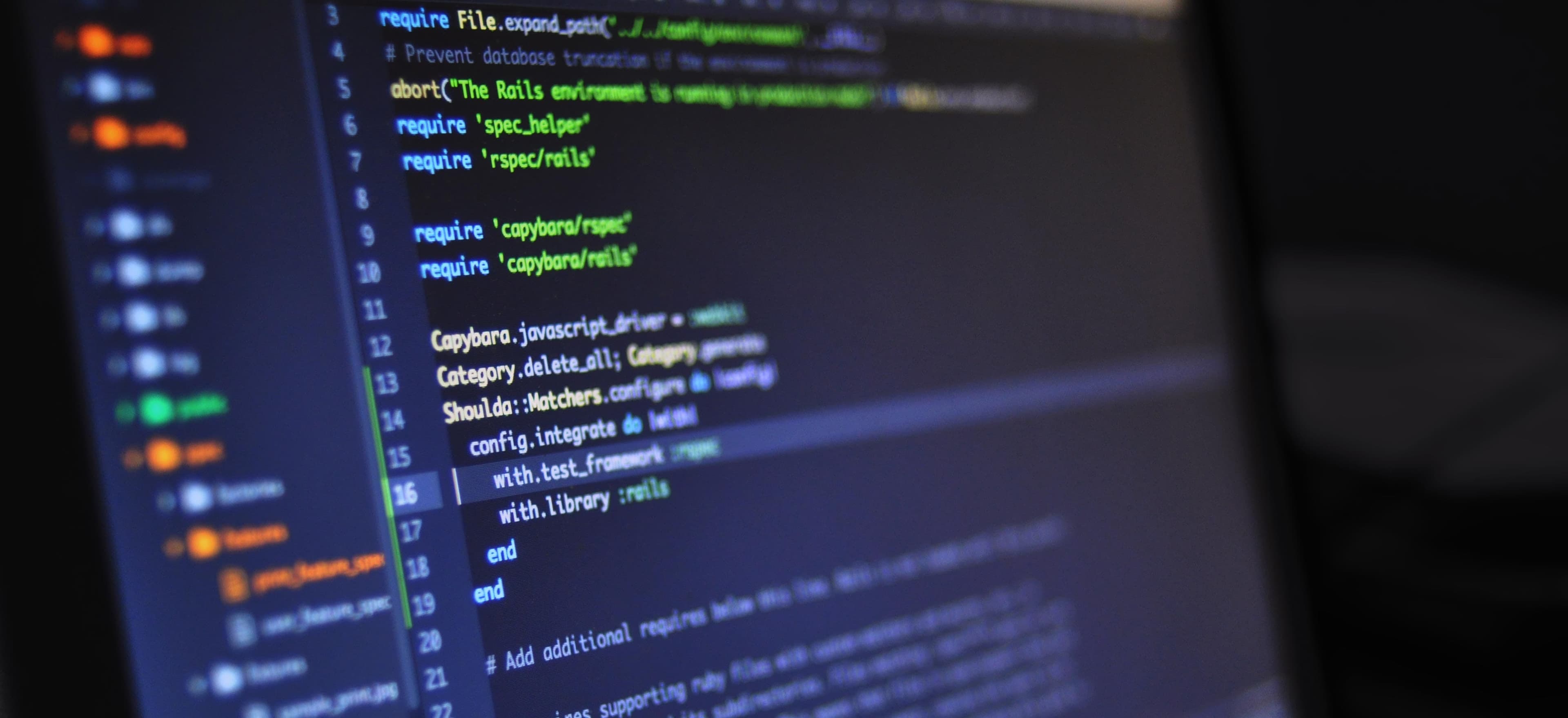
- Published on
Boosting Performance: Common Pitfalls of Singleton Streams
Java has given developers immense power when it comes to data manipulation, especially with the introduction of the Stream API in Java 8. Despite its advantages, many developers encounter pitfalls when using singleton streams. This blog post will discuss these common pitfalls, provide illustrative examples, and offer practical solutions to boost performance and efficiency.
Understanding Streams in Java
Before diving into the pitfalls, it's essential to grasp what streams are. A stream in Java is a sequence of elements supporting sequential and parallel aggregate operations. It enables developers to process collections of objects in a functional style.
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
Stream<String> nameStream = names.stream();
In this snippet, we establish a stream from a list of names. This lets us leverage functional programming by applying operations like filtering, mapping, and reducing.
The Singleton Stream Concept
A singleton stream is simply a stream that contains a single element. For instances where a single item is frequently needed in data processing, some developers dynamically create a singleton stream.
Stream<String> singleNameStream = Stream.of("Alice");
While this approach seems clean, there are a few performance pitfalls.
Common Pitfalls of Singleton Streams
1. Unnecessary Object Creation
Creating a singleton stream involves object creation, which adds overhead. If you're calling singleton streams in a loop or repeatedly, you could be creating multiple objects unnecessarily.
Example:
for (int i = 0; i < 1000; i++) {
Stream<String> singleNameStream = Stream.of("Alice");
singleNameStream.forEach(System.out::println);
}
Why This is a Pitfall:
In the above code, a new Stream object is created for each iteration leading to excessive memory consumption and GC overhead.
Solution:
Instead, consider reusing the same stream.
Stream<String> singleNameStream = Stream.of("Alice");
for (int i = 0; i < 1000; i++) {
singleNameStream.forEach(System.out::println);
}
2. Lack of Parallelism
Singleton streams typically fetch a single element, which may lead developers to overlook concurrency options available through parallel streams.
Example:
Stream<String> singleNameStream = Stream.of("Alice");
singleNameStream.parallel().forEach(System.out::println);
Why This is a Pitfall:
The use of parallel()
doesn't yield performance benefits here as a singleton stream cannot be effectively parallelized. If you're working with larger data sets, this could significantly underutilize available processing power.
Solution:
Use partitioned data sets instead.
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.parallelStream().forEach(System.out::println);
3. Unintended Lazy Evaluation
Streams are lazily evaluated. If not handled correctly, this can lead to unintended behaviors, particularly with singleton streams.
Example:
Stream<String> singleNameStream = Stream.of("Alice").filter(n -> {
System.out.println("Filtering: " + n);
return n.startsWith("A");
});
Why This is a Pitfall:
The filtering does not occur until a terminal operation calls for it. If you mistakenly think that the filter is executed immediately, you might be caught off guard.
Solution:
Understanding terminal vs. intermediate operations is crucial. Ensuring your stream pipeline concludes with a terminal operation will prevent confusion.
long count = singleNameStream.count(); // Triggers filtering
4. Not Utilizing Collectors
Singleton streams can sometimes be misunderstood in terms of their utility. Developers might overlook using collectors, leading to inefficient data processing.
Example:
Stream<String> singleNameStream = Stream.of("Alice");
String result = singleNameStream.collect(Collectors.joining(", "));
Why This is a Pitfall:
If you think of single streams as standalone evidences, you could be missing out on the rich capabilities of the Stream API. Collectors provide an efficient way to collect results by stream processing.
Solution:
Incorporate collectors appropriately and understand their power.
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
String result = names.stream()
.collect(Collectors.joining(", "));
5. Excessive Use in Functional Interfaces
The intuitive nature of singleton streams may cause programmers to call them in functional interfaces, leading to boilerplate code.
Example:
Runnable runnable = () -> Stream.of("Alice").forEach(System.out::println);
runnable.run();
Why This is a Pitfall:
Calling a singleton stream in this manner could lead to unwanted object creation every time the Runnable is executed. This not only reduces performance but also results in code that’s hard to maintain.
Solution:
Use of method references or variables instead of inline stream construction.
Stream<String> singleNameStream = Stream.of("Alice");
Runnable runnable = () -> singleNameStream.forEach(System.out::println);
runnable.run();
Best Practices for Using Singleton Streams
-
Limit Usage: Only use streaming APIs when necessary.
-
Reuse Streams: Reusability can dramatically improve performance.
-
Understand Evaluation: Know when evaluation occurs to prevent runtime surprises.
-
Prefer Collectors: Utilize collectors for data manipulations instead of relying solely on streams.
-
Leverage Parallel Streams: For larger data sets, consider implementing parallel streams effectively instead of singleton streams.
Closing the Chapter
While singleton streams offer simplicity, they come with pitfalls that can hinder performance. By recognizing the potential traps and utilizing the best practices outlined above, you can enhance the efficiency of your Java applications. For further reading on Java Streams, check out resources such as the official Java Documentation or read about Stream Performance.
Feel free to ask about any specific use cases or examples you might want to see!
References
Checkout our other articles