Mastering the Builder Pattern: Common Pitfalls to Avoid
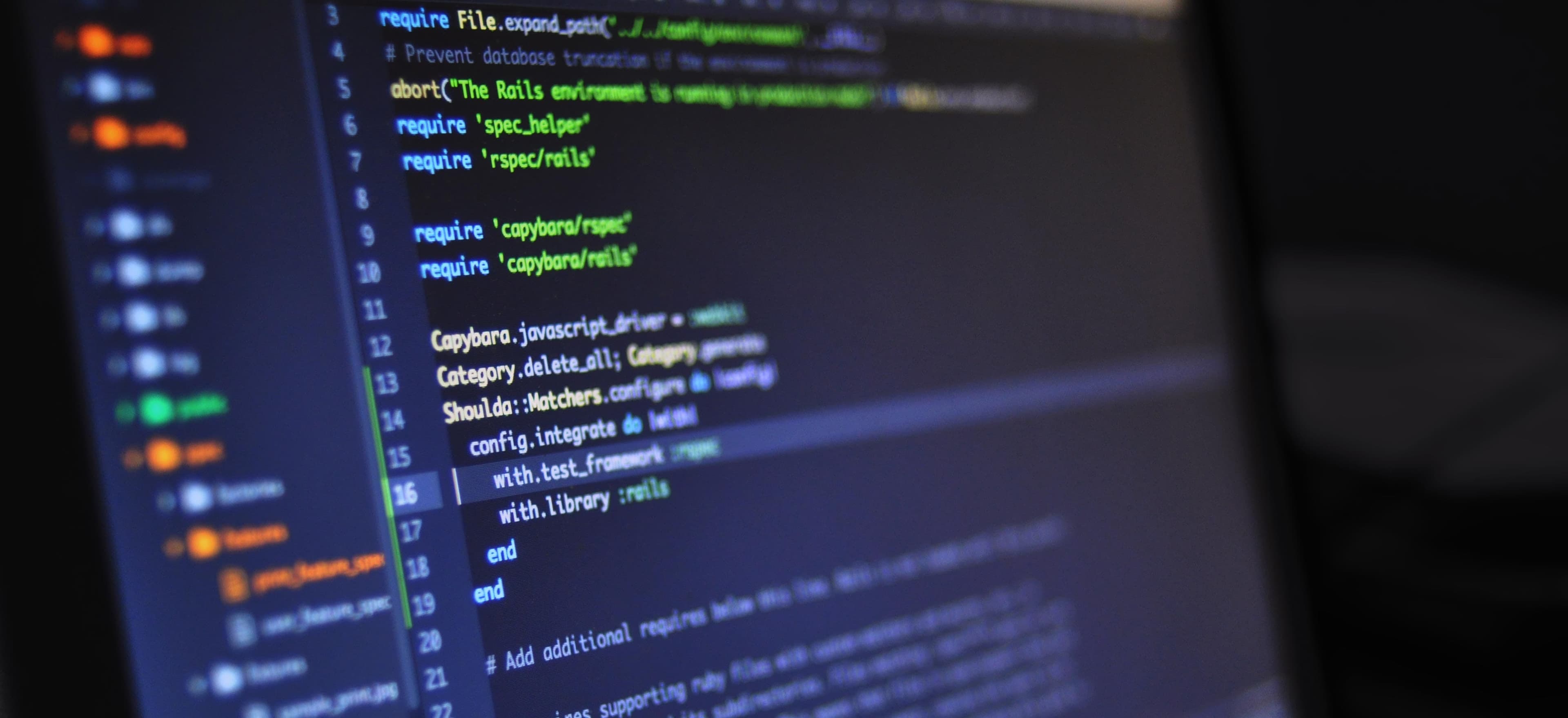
- Published on
Mastering the Builder Pattern: Common Pitfalls to Avoid
The Builder Pattern is a powerful design pattern that allows for the flexible construction of complex objects. Named for its ability to construct these objects step by step, it minimizes the complexity of object creation and enhances the readability of the code. However, like any design pattern, it comes with its own set of pitfalls. In this blog post, we will explore the Builder Pattern in Java, highlight common mistakes developers make, and provide strategies to avoid them.
What is the Builder Pattern?
The Builder Pattern is a creational pattern focused on constructing complex objects step by step. Unlike using a constructor that requires many parameters, particularly when there are default values or optional configurations, the Builder Pattern promotes a more readable and manageable way to create an object.
Advantages of the Builder Pattern
- Readability: Each step in the object creation process is clear and understandable.
- Immutability: Builders can return immutable objects, making your code thread-safe.
- Fluency: The method chaining used in builders enables a fluid and intuitive API.
Basic Structure of the Builder Pattern
Here is a simplified structure of how the Builder Pattern works in Java:
public class House {
private final int floors;
private final int rooms;
private final String color;
private House(HouseBuilder builder) {
this.floors = builder.floors;
this.rooms = builder.rooms;
this.color = builder.color;
}
public static class HouseBuilder {
private final int floors; // Required
private int rooms = 1; // Default value
private String color = "White"; // Default value
public HouseBuilder(int floors) {
this.floors = floors;
}
public HouseBuilder rooms(int rooms) {
this.rooms = rooms;
return this;
}
public HouseBuilder color(String color) {
this.color = color;
return this;
}
public House build() {
return new House(this);
}
}
}
In this example, the House
class has private constructors and a chained builder class, HouseBuilder
. The build()
method constructs the House
object.
Common Pitfalls in the Builder Pattern
While the Builder Pattern offers significant advantages, developers often encounter pitfalls when implementing this pattern. Below are some common mistakes along with explanations and solutions.
1. Overcomplicating the Builder
Pitfall: Developers often add too many parameters and methods, leading to a complex builder that nullifies the pattern's advantages.
Solution: Keep the builder simple. Only include parameters that significantly change the object’s state.
// Good: Simple builder focusing on essential properties
public class SimpleHouse {
private final int floors;
private final String color;
private SimpleHouse(SimpleHouseBuilder builder) {
this.floors = builder.floors;
this.color = builder.color;
}
public static class SimpleHouseBuilder {
private final int floors; // Required
private String color = "White"; // Default value
public SimpleHouseBuilder(int floors) {
this.floors = floors;
}
public SimpleHouseBuilder color(String color) {
this.color = color;
return this;
}
public SimpleHouse build() {
return new SimpleHouse(this);
}
}
}
By focusing only on essential properties, the code remains clean and easy to manage.
2. Forgetting to Validate Parameters
Pitfall: Neglecting to validate input values in the builder can lead to undesirable states in the constructed object.
Solution: Implement validation logic within the build()
method. This ensures that the object is in a correct state before it is created.
public SimpleHouse build() {
// Validate parameters
if (floors <= 0) {
throw new IllegalArgumentException("Floors must be greater than zero");
}
return new SimpleHouse(this);
}
Incorporating validation helps maintain data integrity and improves robustness.
3. Ignoring Immutability
Pitfall: Some developers forget that the objects built by the builder should be immutable, allowing for safe threading practices.
Solution: Ensure that all fields in the target class are marked as final
and only set through the builder.
public class ImmutableHouse {
private final int floors;
private final String color;
// Constructor and builder remain unchanged
}
Immutability fosters more predictable and easier-to-debug code.
4. Not Accounting for Default Values
Pitfall: Failing to manage default values can lead to objects in an inconsistent and undesirable state.
Solution: Clearly define defaults within the builder and give users the option to override them.
public HouseBuilder color(String color) {
this.color = color != null ? color : "White"; // Explicit default handling
return this;
}
This keeps your API clear while ensuring sensible defaults.
5. Making Builders Difficult to Use
Pitfall: When the builder interface is convoluted, it can deter usage.
Solution: Maintain a fluent interface by strategically naming methods and allowing chaining.
public HouseBuilder withRooms(int rooms) {
this.rooms = rooms;
return this;
}
// Usage
House house = new HouseBuilder(2).withRooms(4).build();
A fluent interface improves usability and supports clean, readable code.
6. Poor Documentation
Pitfall: Insufficient documentation can mislead other developers or even your future self.
Solution: Always document your builder class and its methods clearly.
/**
* Builder for a House object.
* @param floors required number of floors for the house.
*/
public HouseBuilder(int floors) {
this.floors = floors;
}
Good documentation is essential for maintaining code, especially in team environments.
Using the Builder Pattern Effectively
Now that we've discussed common pitfalls, how can you use the Builder Pattern effectively? Here are some strategies:
-
Limit Builder Scope: Ideally, keep the builder within the same file as the class it builds. This encapsulation helps maintain focus.
-
Utilize Inner Classes: If your builder is intended only to support the outer class, make the builder an inner class.
-
Consider Using Fluent Interfaces: This enhances usability and creates a better coding experience.
-
Handle Collection Properties Carefully: If your object has a collection as a property, consider how you wish to handle it. For instance, allowing dynamic changes or copying could impact immutability.
Here is a more advanced example that includes a collection of features:
public class AdvancedHouse {
private final Set<String> features;
private AdvancedHouse(AdvancedHouseBuilder builder) {
this.features = builder.features;
}
public static class AdvancedHouseBuilder {
private final Set<String> features = new HashSet<>();
public AdvancedHouseBuilder addFeature(String feature) {
features.add(feature);
return this;
}
public AdvancedHouse build() {
return new AdvancedHouse(this);
}
}
}
To Wrap Things Up
Understanding the Builder Pattern and its potential pitfalls is crucial for developing clean and manageable Java applications. By avoiding common mistakes such as overcomplication, lack of validation, and poor documentation, you can leverage the Builder Pattern effectively.
By mastering these concepts, you pave the way for delivering more robust and understandable code. The next time you need to create complex objects, remember the Builder Pattern and keep these pitfalls at bay.
For further reading, you may want to check out the Gang of Four Design Patterns book or the Oracle Java Tutorial on Design Patterns.
By avoiding the identified pitfalls, and applying the strategies discussed, you can ensure that the Builder Pattern becomes a valuable tool in your coding toolkit. Happy coding!
Checkout our other articles