Common Pitfalls in Creating Microservices with Spring Boot
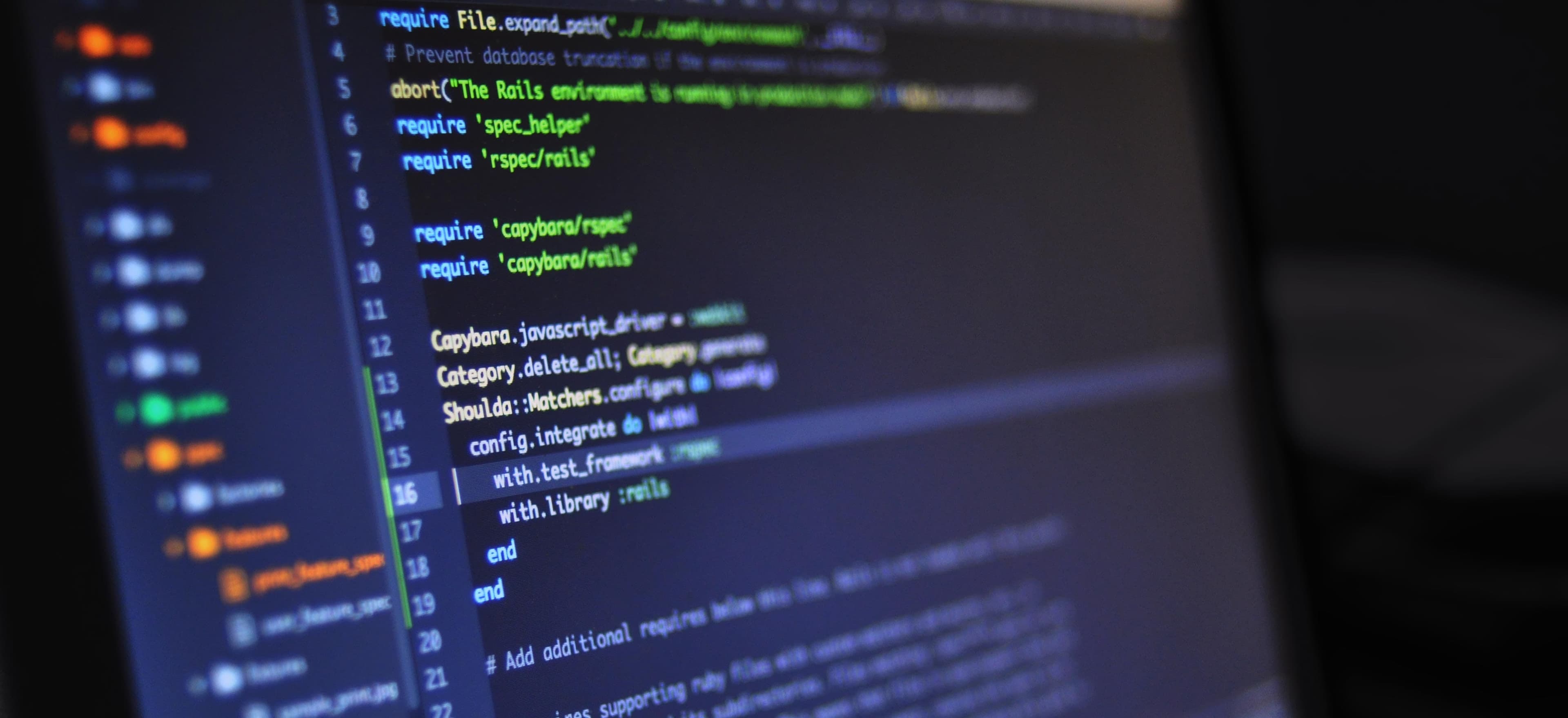
- Published on
Common Pitfalls in Creating Microservices with Spring Boot
Microservices architecture has gained popularity as a means to build scalable, agile, and resilient applications. Using Spring Boot, developers can create stand-alone Spring applications that operate independently in a microservices ecosystem. However, there are common pitfalls that teams face when developing microservices with Spring Boot. In this blog post, we will explore these pitfalls, provide code snippets to illustrate concepts, and offer best practices for avoiding common mistakes.
The Rise of Microservices Architecture
Microservices architecture divides applications into smaller, autonomous services that serve a specific business capability. This allows teams to develop, deploy, and scale services independently, leading to more efficient workflows. Still, while the benefits seem enticing, pitfalls lurk around every corner.
Pitfall 1: Over-Engineering Microservices
Problem: Developers often feel compelled to build services too granularly, leading to an excessive number of microservices.
Solution: Understand the business domain and the workflow before decomposing services. Aim for a balance that allows for modularity but avoids unnecessary complexity.
@RestController
@RequestMapping("/orders")
public class OrderController {
@Autowired
private OrderService orderService;
// Endpoint to create order
@PostMapping
public ResponseEntity<Order> createOrder(@RequestBody Order order) {
Order createdOrder = orderService.createOrder(order);
return new ResponseEntity<>(createdOrder, HttpStatus.CREATED);
}
}
- Why: This concentric approach allows developers to create a comprehensive order management system without needless fragmentation. Each service should encapsulate closely related functionalities.
Pitfall 2: Not Implementing Service Discovery
Problem: When services are not able to discover each other, it can lead to hardcoded configurations and difficulties in scaling.
Solution: Use a service discovery tool such as Eureka.
Eureka Configuration Example:
eureka:
client:
serviceUrl:
defaultZone: http://localhost:8761/eureka/
instance:
preferIpAddress: true
- Why: Service discovery allows microservices to keep a dynamic record of service instances, facilitating seamless communication as the system scales or changes.
Pitfall 3: Ignoring API Contracts
Problem: Without Api contracts, services might have interoperability issues and cause integration problems down the line.
Solution: Adopt OpenAPI/Swagger for documenting APIs.
Swagger Configuration Example:
@SpringBootApplication
@EnableSwagger2
public class ApiDocumentationConfig {
public static void main(String[] args) {
SpringApplication.run(ApiDocumentationConfig.class, args);
}
@Bean
public Docket api() {
return new Docket(DocumentationType.SWAGGER_2)
.select()
.apis(RequestHandlerSelectors.any())
.paths(PathSelectors.any())
.build();
}
}
- Why: This allows teams to generate and maintain API documentation effortlessly, leading to better client integration and reduced errors.
Pitfall 4: Centralized Database Architecture
Problem: Designing microservices with a shared database leads to tight coupling and defeats the purpose of the microservices model.
Solution: Each microservice should maintain its own database, aligned with the microservices principle of decentralization.
// Example of a separate Order repository
@Repository
public interface OrderRepository extends JpaRepository<Order, Long> {
}
- Why: This design decision ensures that each service remains independent. If one microservice fails or modifies its schema, others remain unaffected.
Pitfall 5: Synchronous Communication
Problem: Employing synchronous communication can lead to performance bottlenecks and cascading failures.
Solution: Implement asynchronous communication with events.
Spring Boot Event Example:
@Service
public class OrderService {
@Autowired
private ApplicationEventPublisher eventPublisher;
public Order createOrder(Order order) {
// Business logic ...
eventPublisher.publishEvent(new OrderCreatedEvent(order));
return order;
}
}
- Why: Asynchronous communication helps decouple services and improve performance. It also allows systems to remain functional even if one part experiences a failure.
Pitfall 6: Failing to Monitor and Log Microservices
Problem: Lack of monitoring results in reduced visibility and inefficiencies in troubleshooting.
Solution: Use tools such as Spring Boot Actuator and ELK stack for comprehensive logging.
// Actuator dependency
dependencies {
implementation 'org.springframework.boot:spring-boot-starter-actuator'
}
- Why: Actuators provide valuable insights into application health, metrics, and logging, enabling teams to respond quickly to issues.
Pitfall 7: Not Securing Microservices
Problem: Leaving services unguarded or relying on conventional authentication mechanisms can lead to vulnerabilities.
Solution: Implement a security framework, such as Spring Security, for robust security practices.
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().disable()
.authorizeRequests()
.anyRequest().authenticated()
.and()
.httpBasic();
}
}
- Why: Security should be a priority in all stages of development. Implementing Spring Security provides foundational security features and can be extended as needed.
Pitfall 8: Lack of CI/CD Practices
Problem: Without proper Continuous Integration/Continuous Deployment (CI/CD), deployments can become error-prone and slow.
Solution: Utilize tools such as Jenkins or GitHub Actions to enable automated testing and deployments.
Jenkinsfile Example:
pipeline {
agent any
stages {
stage('Build') {
steps {
sh './gradlew build' // Example command for Gradle
}
}
stage('Deploy') {
steps {
// Deployment steps here
}
}
}
}
- Why: CI/CD practices help maintain consistency and reliability across deployments, enabling more frequent releases and quicker fixes.
In Conclusion, Here is What Matters
In conclusion, while creating microservices with Spring Boot offers tremendous advantages, it’s essential to navigate the associated challenges carefully. By avoiding these common pitfalls and leveraging best practices, developers can build robust, scalable, and maintainable microservices architectures that deliver value to users.
For more insights into Spring Boot and microservices architecture, consider checking out these resources: Spring Boot Official Documentation, Microservices with Spring Boot, and Eureka for Load Balancing Microservices.
Remember, the road to successful microservices design is paved with thoughtful planning, discipline in implementation, and a commitment to continuous learning. Happy coding!
Checkout our other articles