Why Your foreach Loops Might Be Failing: Common Pitfalls
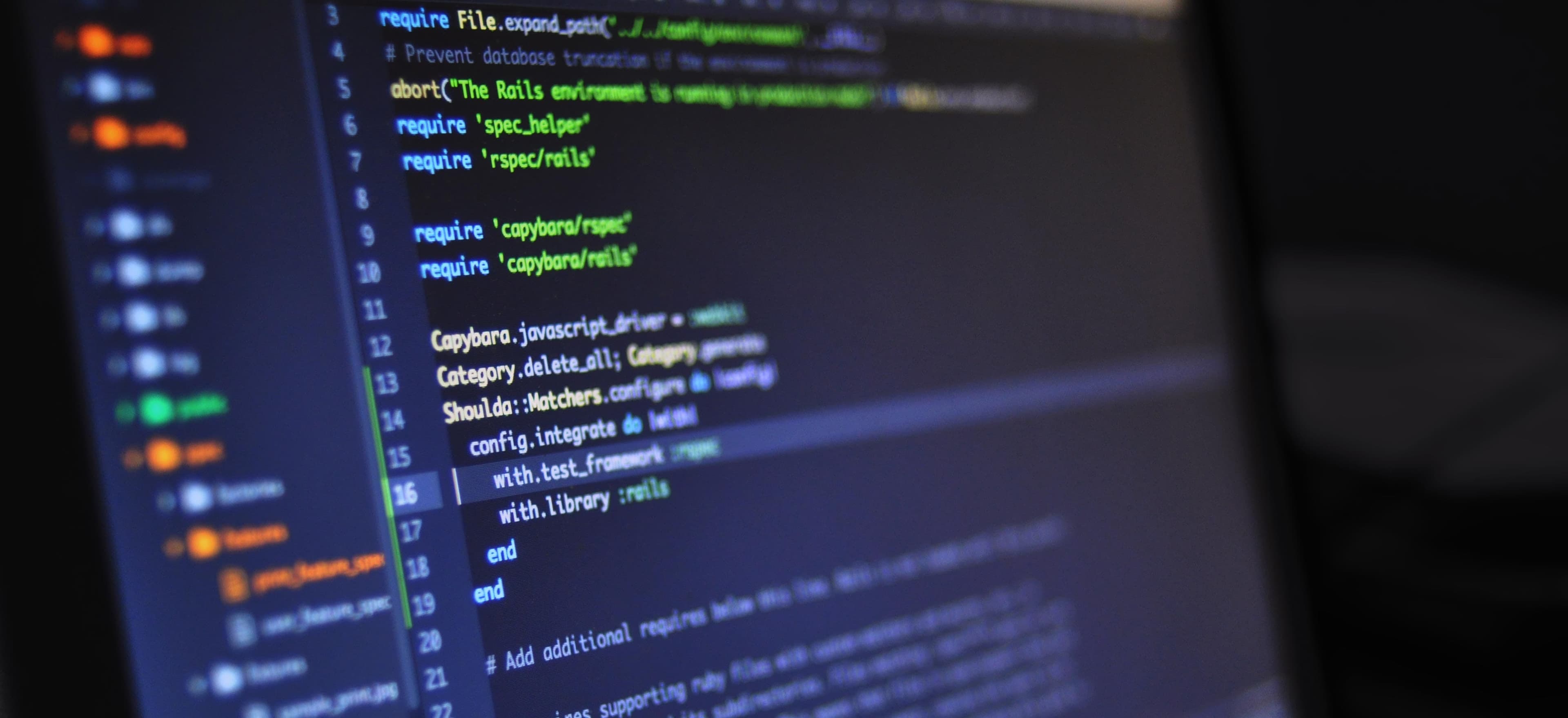
- Published on
Why Your foreach Loops Might Be Failing: Common Pitfalls
In Java programming, foreach
loops are a powerful feature, allowing developers to iterate over collections with ease. However, even seasoned developers can encounter issues when using them. In this blog post, we’ll delve into some common pitfalls associated with foreach
loops, providing clarity and solutions that will enhance your coding practice.
Understanding the Foreach Loop
Before we explore the pitfalls, it's essential to have a clear understanding of what a foreach
loop is. Introduced in Java 5, the foreach
loop simplifies the syntax needed to iterate through arrays and collections.
Syntax Example
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
for (String name : names) {
System.out.println(name);
}
In this example, name
will take the value of each element in the names
list one by one, printing each name to the console. But, as straightforward as this may seem, there are scenarios where things can go awry.
Common Pitfalls
1. Modifying the Collection During Iteration
One of the most common issues with foreach
loops is modifying the underlying collection while iterating through it. This can lead to ConcurrentModificationException
.
Example
List<String> names = new ArrayList<>(Arrays.asList("Alice", "Bob", "Charlie"));
for (String name : names) {
if (name.equals("Bob")) {
names.remove(name); // This will throw ConcurrentModificationException
}
}
Solution
If you need to modify the collection, consider using an Iterator
.
Iterator<String> iterator = names.iterator();
while (iterator.hasNext()) {
String name = iterator.next();
if (name.equals("Bob")) {
iterator.remove(); // Safe removal
}
}
2. Interaction with Null Values
Another area where foreach
loops can fail is when they run into null values. Attempting to access or process elements that are null
can lead to NullPointerException
.
Example
List<String> names = Arrays.asList("Alice", null, "Charlie");
for (String name : names) {
System.out.println(name.toUpperCase()); // NullPointerException when name is null
}
Solution
Provide checks for null values before processing the elements.
for (String name : names) {
if (name != null) {
System.out.println(name.toUpperCase()); // Safe check
}
}
3. Using foreach
with Arrays of Primitives
Java’s foreach
loop doesn’t directly support arrays of primitive types. If you attempt to utilize a foreach on a primitive array, it might not behave as expected.
Example
int[] numbers = {1, 2, 3};
for (int number : numbers) {
System.out.println(number);
}
Solution
The above code is actually valid in Java, as it operates correctly. However, if you encounter errors while using a primitive type, it's best practice to use a wrapper class or convert to a collection type.
// Use Integer array instead
Integer[] numbers = {1, 2, 3};
for (Integer number : numbers) {
System.out.println(number);
}
4. Not Using Enhanced Features
Java foreach
loops lack some features available in traditional for
loops, such as the ability to track index values. This might lead to dissatisfaction when developers want to access the index of an element during iteration.
Example
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
for (String name : names) {
System.out.println(names.indexOf(name)); // Can produce unexpected results
}
Solution
If indexing is crucial to your logic, consider using a traditional for
loop.
for (int i = 0; i < names.size(); i++) {
System.out.println("Index: " + i + ", Name: " + names.get(i));
}
5. Misunderstanding Scope
In some situations, developers manage variables' scopes incorrectly. Variables declared within the foreach
loop are not accessible outside of its block.
Example
for (String name : names) {
String upperCaseName = name.toUpperCase();
}
// upperCaseName is inaccessible here
Solution
If you need to use the variable outside the loop, declare it beforehand.
String upperCaseName = "";
for (String name : names) {
upperCaseName = name.toUpperCase(); // Updated with last iteration value
}
System.out.println(upperCaseName);
6. Type Safety Issues
Using raw types in foreach
loops can lead to type safety issues. This is often encountered when working with collections or generics, which can lead to ClassCastException
.
Example
List rawList = new ArrayList();
rawList.add("Alice");
rawList.add(1); // Mixing types
for (String name : rawList) { // Will cause ClassCastException
System.out.println(name);
}
Solution
Make sure to use generic collections to enforce type safety.
List<String> stringList = new ArrayList<>();
stringList.add("Alice");
// stringList.add(1); // This will now cause a compile-time error
for (String name : stringList) {
System.out.println(name); // Safe iteration
}
Lessons Learned
Java's foreach
loops offer a user-friendly method for iterating over collections. By being aware of the common pitfalls and implementing the provided solutions, you can maximize their effectiveness and avoid frustration in your coding journey.
For further reading on loops and performance optimizations, consider checking out the Java documentation or exploring advanced tutorials on Java Collection Framework.
Happy coding! Remember, mastering foreach
loops and understanding their pitfalls is an essential step toward becoming an expert Java developer.
Checkout our other articles