Common Pitfalls When Registering Entity Types with OpenJPA
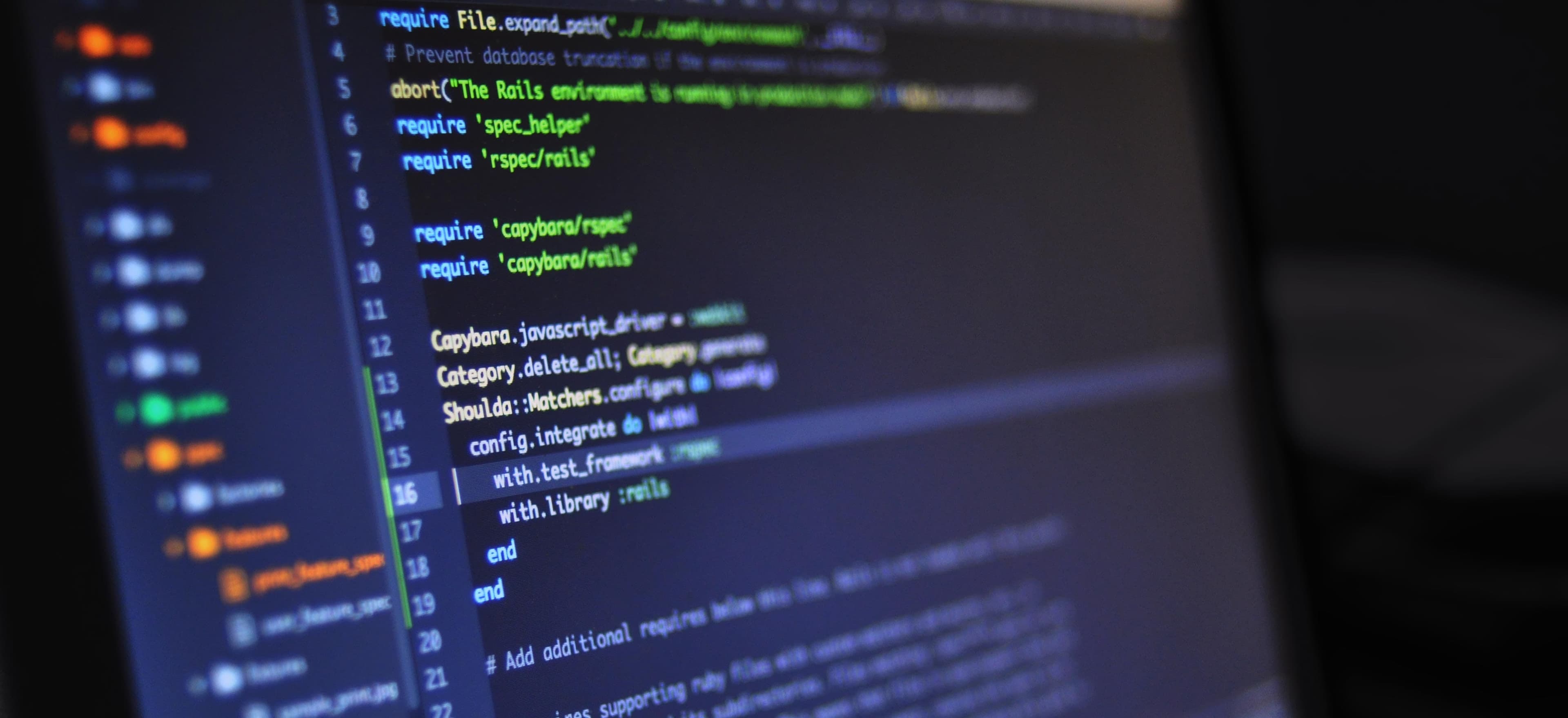
- Published on
Common Pitfalls When Registering Entity Types with OpenJPA
When working with Java Persistence, OpenJPA stands out as a robust framework for interacting with relational databases. While it simplifies data handling, pitfalls can arise if one is not careful, especially during the registration of entity types. In this blog post, we'll cover some common pitfalls encountered while registering entity types with OpenJPA, along with strategies to avoid these mistakes.
Understanding OpenJPA Entity Registration
Before diving into the pitfalls, let's clarify what entity registration means in OpenJPA. An entity in OpenJPA represents a mapping between a Java class and a database table. Thus, registering an entity means informing OpenJPA about the existence of this mapping.
Here's a basic example:
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
// Getters and Setters
}
In the code above, the @Entity
annotation denotes that the User
class is an entity that will be managed by OpenJPA.
Common Pitfalls
1. Not Using the @Entity Annotation
One of the most basic pitfalls is forgetting to use the @Entity
annotation on a Java class. Without this annotation, OpenJPA will not recognize the class as an entity.
Strategy to Avoid: Always ensure that each entity class is annoted with @Entity
if you want it to be managed by OpenJPA.
2. Improperly Configuring Primary Key Annotations
The primary key is crucial for every entity as it uniquely identifies each instance. OpenJPA requires that you specify a primary key using the @Id
annotation. A common mistake is not specifying a primary key, which leads to runtime exceptions.
Example of Proper Configuration:
import javax.persistence.*;
@Entity
public class Product {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
private String productName;
// Getters and Setters
}
Here, the id
field is annotated properly to ensure it acts as the primary key.
3. Inconsistent Naming Conventions
In databases, inconsistency in naming (e.g., using underscores vs. camelCase) can lead to confusion and mapping issues. OpenJPA is case-sensitive, which means that an entity’s field name must match the respective column name case in the database.
How to Avoid: Maintain consistent naming conventions in both your Java classes and database schemas. For example, if your database column is named product_name
, then your entity field should replicate this format.
4. Misusing Fetch Types
Choosing the wrong fetching strategy can lead to performance issues or unexpected behavior. OpenJPA supports two fetch types: FetchType.LAZY
and FetchType.EAGER
.
FetchType.LAZY
: Loads related entities on demand.FetchType.EAGER
: Loads related entities immediately.
Misusing these can either bloat your memory with unnecessary data or lead to N+1 select problems.
Example:
@Entity
public class Order {
@Id
@GeneratedValue
private Long orderId;
@OneToMany(fetch = FetchType.LAZY)
private List<Product> products;
// Getters and Setters
}
In this example, products
will be loaded only when accessed, reducing initial loading time.
5. Not Managing Transactions Properly
OpenJPA operates within a transactional context. Failing to manage transactions properly can lead to data inconsistencies. Always wrap your operations within a transaction.
Example:
EntityManager entityManager = ...; // your entity manager
EntityTransaction transaction = entityManager.getTransaction();
try {
transaction.begin();
// Perform operations
User user = new User();
user.setName("John Doe");
entityManager.persist(user);
transaction.commit();
} catch (RuntimeException e) {
transaction.rollback();
throw e; // Rethrow or handle the exception
} finally {
entityManager.close();
}
This way, if an error occurs, the transaction will roll back, preserving the state of the database.
6. Ignoring Cascade Types
Entity relationships can be complex, especially when dealing with parent-child scenarios. Not properly setting cascade types can lead to data integrity issues.
Example of Relationships with Cascade:
@Entity
public class Customer {
@Id
@GeneratedValue
private Long customerId;
@OneToMany(cascade = CascadeType.ALL)
private List<Order> orders;
// Getters and Setters
}
Setting CascadeType.ALL
on the orders
relationship ensures that any action on the Customer
entity will be reflected on Order
entities appropriately.
7. Forgetting to Update Database Schema
After modifying the entity classes, forgetting to synchronize the underlying database schema can cause discrepancies. This will lead you to runtime exceptions during data access.
Remedy: Use database migration tools like Liquibase or Flyway to manage changes to your database schema effectively. This keeps your database schema in sync with your entity definitions.
8. Incorrectly Mapping Relationships
Mapping relationships incorrectly can lead to issues during data retrieval. Be sure to use the appropriate annotations based on relationships: @OneToOne
, @OneToMany
, @ManyToOne
, and @ManyToMany
.
Example:
@Entity
public class Department {
@Id
@GeneratedValue
private Long departmentId;
@OneToMany(mappedBy = "department")
private List<Employee> employees;
// Getters and Setters
}
Here, @OneToMany
properly defines that one department can relate to multiple employees.
Best Practices for Entity Registration
-
Always Annotate: Remember to annotate all entity classes appropriately.
-
Use Consistent Naming: Choose a naming convention and stick with it across your code and database.
-
Leverage Transactions: Properly manage your transactions to maintain data integrity.
-
Test Thoroughly: Always test your changes in a staging environment before deploying them.
-
Keep Learning: OpenJPA has a learning curve. Stay updated with its documentation to leverage its full potential.
For more insights on JPA, check out the OpenJPA official documentation or dive into detailed explanations in the Java Persistence API (JPA) Guide.
Closing Remarks
Navigating the registration of entity types in OpenJPA demands careful consideration and awareness of potential pitfalls. By remaining vigilant about the common mistakes outlined above and adhering to best practices, you can leverage OpenJPA to create robust, efficient, and maintainable applications. Continual learning and adaptation are key to mastering JPA and enhancing your application's data management capabilities.
Through this exploration, we've examined and encapsulated vital information for successfully registering entity types within OpenJPA. By doing so, we aim to equip developers with the knowledge to avoid pitfalls and embrace best practices. Happy coding!
Checkout our other articles