Common Maven Issues in Selenium Test Automation
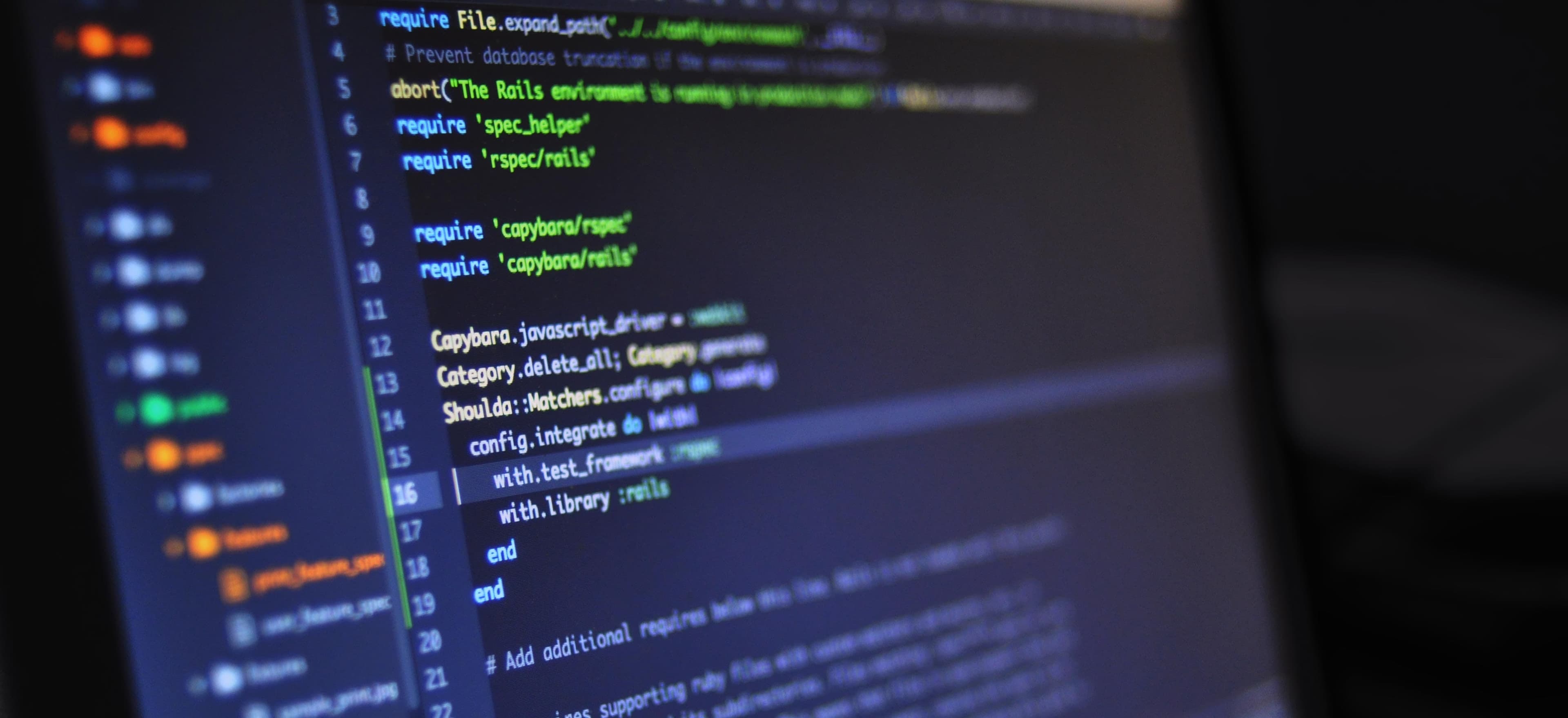
- Published on
Common Maven Issues in Selenium Test Automation
Maven has become a staple in the Java ecosystem, especially in the realm of test automation with Selenium. It simplifies the project management process by handling dependencies and builds efficiently. However, like any tool, it can be a source of frustrating issues. In this blog post, we will delve into some common Maven problems encountered when using Selenium for test automation. We will also provide solutions, code examples, and best practices to help you navigate these challenges effectively.
Table of Contents
- What is Maven?
- Common Maven Issues
- Dependency Conflicts
- Incorrect POM File Configuration
- Build Failures
- Repository Accessibility
- Conclusion
- References
What is Maven?
Maven is a powerful build automation tool primarily used for Java projects. It manages a project’s build lifecycle, dependencies, and documentation, making it easier to maintain and develop Java applications. When combined with Selenium, a popular web automation framework, Maven helps streamline the process of writing and executing automated tests.
Common Maven Issues
Let’s explore some common Maven-related issues faced during Selenium test automation and how to resolve them.
1. Dependency Conflicts
Dependency conflicts occur when two or more libraries that your project relies on have incompatible versions.
Solution:
To identify and resolve dependency conflicts, you can use the mvn dependency:tree
command. This command displays a tree structure of your project’s dependencies and their versions. You can analyze the output to locate version mismatches.
Here is an example of how to force a specific version of a dependency in your pom.xml
:
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>4.1.0</version>
</dependency>
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-chrome-driver</artifactId>
<version>4.1.0</version>
</dependency>
In this case, we are specifying the version 4.1.0
for both Selenium dependencies to minimize conflicts.
2. Incorrect POM File Configuration
The Project Object Model (POM) file is fundamental to any Maven project. A poorly configured POM can lead to various issues, including misspecified dependencies.
Solution:
Ensure your pom.xml
file is correctly configured. Below is an example of a minimal pom.xml
file for a Selenium project:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>selenium-tests</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>4.1.0</version>
</dependency>
</dependencies>
</project>
Above, we’ve defined the group ID, artifact ID, and version for our project, as well as the necessary dependencies. Checking that your dependencies include the correct group and artifact IDs is essential.
3. Build Failures
Build failures can be one of the most exasperating issues in Maven. Various reasons, including missing dependencies or compilation errors, can cause them.
Solution:
To identify build issues during compilation, use the command:
mvn clean install
This command compiles your project, runs tests, and packages the build artifacts. Always check the console output for specific errors. Here’s a common problem: if you have a compilation error, the logs will show you the affected lines and the corresponding messages.
Example:
public class SampleTest {
@Test
public void exampleTest() {
WebDriver driver = new ChromeDriver();
driver.get("http://www.example.com");
assertEquals("Example Domain", driver.getTitle());
driver.quit();
}
}
If the above code results in a compilation failure, the logs will indicate issues such as "unable to resolve symbol" for WebDriver
. Make sure you’ve imported the required libraries correctly in your pom.xml
.
4. Repository Accessibility
Sometimes, a Maven build fails due to inaccessible repositories. This can be due to several reasons — network issues, repository downtime, or misconfigured repository settings.
Solution:
Verify your internet connection and check if the repository URLs specified in your settings.xml
file or pom.xml
are correct. You may also want to try to access these repositories through your browser, ensuring they are responsive.
Here’s a simple check example in settings.xml
that includes additional repository configurations if you have internal or corporate repositories:
<profiles>
<profile>
<id>internal-repo</id>
<repositories>
<repository>
<id>central</id>
<url>http://repo.maven.apache.org/maven2</url>
</repository>
<repository>
<id>my-internal-repo</id>
<url>http://mycompany.com/maven-repo</url>
</repository>
</repositories>
</profile>
</profiles>
This snippet ensures that Maven can access both public and internal repositories, reducing the likelihood of accessibility issues.
Final Thoughts
Working with Maven in Selenium projects involves navigating through several common issues. From dependency conflicts to repository accessibility, each challenge can typically be resolved with the right knowledge and approach. By understanding the essential configurations and making use of commands like mvn dependency:tree
or mvn clean install
, you can quickly troubleshoot and resolve issues.
Utilizing tools like Maven not only aids in managing Java projects but also assists in maintaining a clean and efficient setup for test automation tasks. If you encounter persistent Maven issues, consider consulting the Maven Documentation for in-depth guidance.
References
By understanding and preemptively addressing common issues, you will ensure a smoother experience in test automation with Selenium and Maven. Happy testing!