Common Pitfalls in Java 9 Money API You Should Avoid
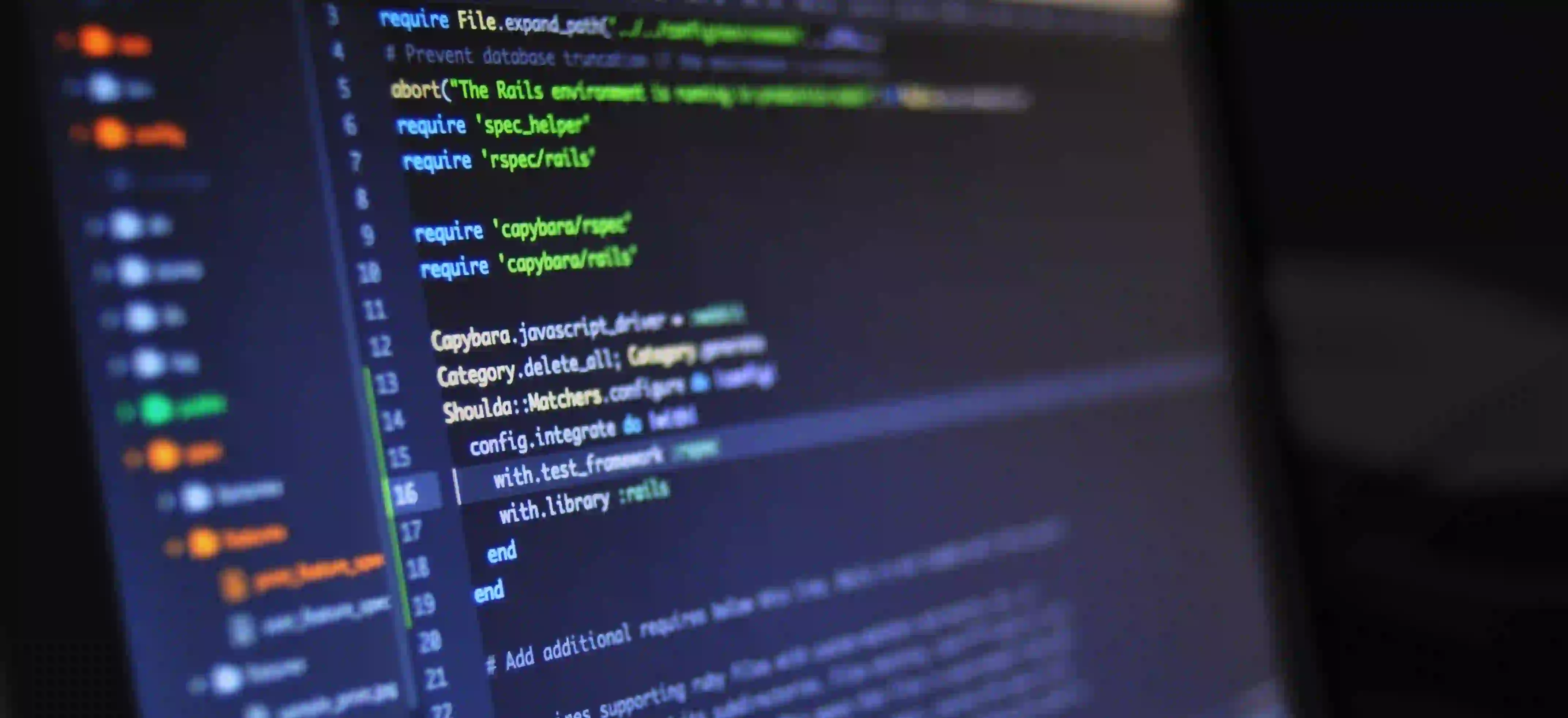
Common Pitfalls in Java 9 Money API You Should Avoid
The introduction of the Money API in Java 9 was a significant leap toward handling monetary values accurately within applications. This API aims to provide a reliable and straightforward way to work with currencies, capturing the complexities often associated with financial calculations. However, despite its improvements, developers can still encounter pitfalls when using this API. In this blog post, we will explore common mistakes and discuss best practices to ensure your financial applications are robust and error-free.
Understanding the Java 9 Money API
Before diving into potential pitfalls, let's briefly recap the key components of the Money API. Introduced in Java 9 as part of the java.money
package, the API includes classes such as Monetary
, MonetaryAmount
, and CurrencyUnit
. This design allows developers to create and manage monetary values while considering various currency units, providing an essential tool for financial applications.
Basic Example
Here's a basic example of creating and using an instance of the MonetaryAmount
class:
import javax.money.Monetary;
import javax.money.MonetaryAmount;
import javax.money.CurrencyUnit;
public class MoneyExample {
public static void main(String[] args) {
CurrencyUnit usd = Monetary.getCurrency("USD");
MonetaryAmount amount = Monetary.getDefaultAmountFactory().setCurrency(usd).setNumber(100.50).create();
System.out.println("Amount: " + amount);
}
}
In this snippet, we establish a currency unit and create a monetary amount. These operations are foundational before exploring common pitfalls. Now, let’s delve into pitfalls you might encounter—and how to avoid them.
Pitfall 1: Ignoring Immutable Objects
One of the significant advantages of the Money API is its emphasis on immutability. Objects like MonetaryAmount
are immutable, which means once created, their state cannot change. This feature is beneficial because it prevents unintended side effects.
What to Avoid
Modifying monetary values directly or attempting to change their state can lead to unexpected results. For instance:
MonetaryAmount original = Monetary.getDefaultAmountFactory().setCurrency("USD").setNumber(100).create();
MonetaryAmount modified = original.add(50); // Correct usage
modified.setNumber(200); // Incorrect, throws UnsupportedOperationException
Solution
Always use methods like add
, subtract
, or multiply
to create new instances rather than trying to modify existing ones. The code above demonstrates the correct approach to handling immutability.
MonetaryAmount newAmount = original.add(Monetary.getDefaultAmountFactory().setCurrency("USD").setNumber(50).create());
System.out.println("New Amount: " + newAmount); // Outputs: New Amount: USD 150
Pitfall 2: Not Accounting for Currency Conversions
When dealing with multiple currencies, not considering the current exchange rate is a common oversight. Applications may inadvertently mix currencies without proper conversion, resulting in inaccurate calculations.
What to Avoid
Assuming that monetary amounts in different currency units can be manipulated interchangeably leads to unexpected behaviors:
MonetaryAmount usdAmount = Monetary.getDefaultAmountFactory().setCurrency("USD").setNumber(100).create();
MonetaryAmount eurAmount = Monetary.getDefaultAmountFactory().setCurrency("EUR").setNumber(90).create();
MonetaryAmount total = usdAmount.add(eurAmount); // Incorrect
Solution
Use CurrencyConversion
provided by the Money API for converting between different currencies. Here's how to do it correctly:
import javax.money.Monetary;
import javax.money.convert.CurrencyConversion;
import javax.money.convert.MonetaryConversions;
MonetaryAmount usdAmount = Monetary.getDefaultAmountFactory().setCurrency("USD").setNumber(100).create();
CurrencyConversion conversion = MonetaryConversions.getConversion("EUR");
MonetaryAmount eurAmount = usdAmount.with(conversion);
System.out.println("Converted Amount: " + eurAmount);
Pitfall 3: Misusing BigDecimal
for Monetary Calculations
In financial applications, using the BigDecimal
class is crucial for precision. However, some developers fall into the trap of using regular floating-point arithmetic, which can produce rounding errors.
What to Avoid
Reading monetary values into primitive types or BigDecimal
without proper handling can introduce inaccuracies. For example:
double price = 19.99; // Improper usage
double taxedPrice = price * 1.07; // Inaccurate due to floating-point arithmetic
Solution
Use MonetaryAmount
for all monetary calculations to maintain precision:
MonetaryAmount price = Monetary.getDefaultAmountFactory().setCurrency("USD").setNumber(19.99).create();
MonetaryAmount taxedPrice = price.multiply(1.07); // Maintains accuracy
System.out.println("Taxed Price: " + taxedPrice);
Pitfall 4: Neglecting Locale
The display format of monetary values can vary significantly depending on the locale. Ignoring this aspect can result in poorly formatted outputs that confuse users.
What to Avoid
Developers sometimes output amounts without considering user locale, leading to inconsistent representations. For instance:
System.out.println("Price: " + price); // Without locale awareness
Solution
Utilize the NumberFormat
class to format monetary amounts according to the locale:
import java.text.NumberFormat;
import java.util.Locale;
MonetaryAmount amount = Monetary.getDefaultAmountFactory().setCurrency("EUR").setNumber(1500.75).create();
NumberFormat formatter = NumberFormat.getCurrencyInstance(Locale.GERMANY);
System.out.println("Formatted Amount: " + formatter.format(amount.getNumber().numberValue(BigDecimal.class)));
Pitfall 5: Overcomplicating Code
Lastly, a common issue when using the Money API is overcomplicating the logic for simple tasks. This can lead to unreadable and difficult-to-maintain code.
What to Avoid
Avoid complex branching statements or convoluted methods that break down simple monetary operations:
MonetaryAmount totalAmount;
if (conditionOne) {
totalAmount = computeTotalOne();
} else if (conditionTwo) {
totalAmount = computeTotalTwo();
} else {
totalAmount = computeTotalThree();
}
// This can be simplified
Solution
Refactor your code to reuse methods and eliminate redundancy, concentrating on clarity:
MonetaryAmount totalAmount = computeTotal();
System.out.println("Total Amount: " + totalAmount);
Final Considerations
The Java Money API brought significant advancements to how we handle monetary values in Java. However, with new features come new challenges. Ensuring that you stay aware of immutability, currency conversions, precision with calculations, locale differences, and code complexity will help you create more robust applications.
By learning from these common pitfalls, you can write cleaner, more maintainable, and less error-prone code, providing a better experience both for yourself as a developer and for the users of your application.
For further reading, consider checking out JavaMoney's official documentation or additional resources on currency conversion.
Feel free to consult this post whenever you face challenges implementing the Java 9 Money API—because mastering money management in programming is an invaluable skill. Happy coding!