Mastering Servlet API: Common Interview Pitfalls to Avoid
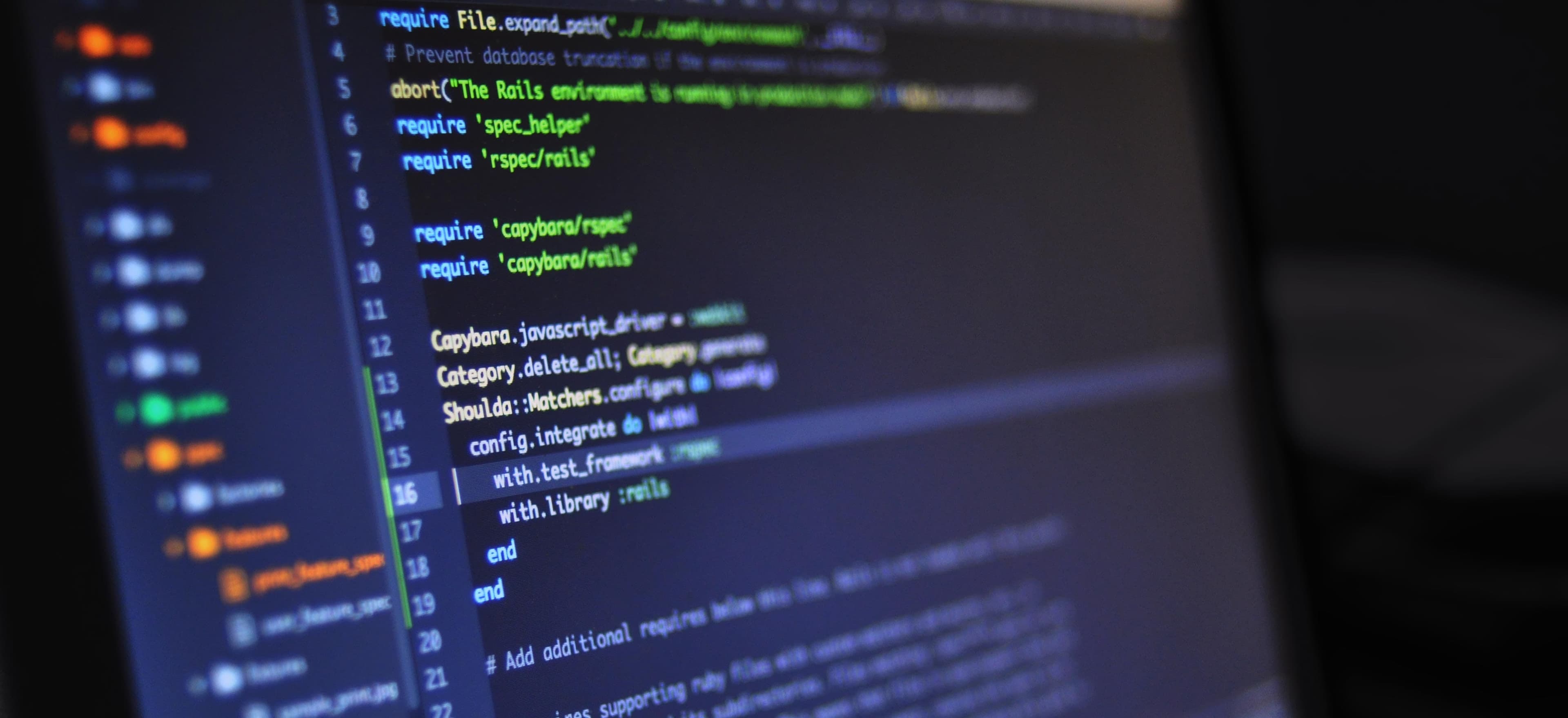
- Published on
Mastering Servlet API: Common Interview Pitfalls to Avoid
When it comes to Java web development, understanding the Servlet API is crucial. It is the foundation for building dynamic web applications and forms the backbone of many frameworks like Spring and JavaServer Faces (JSF). For aspiring Java developers, mastering the Servlet API not only enhances your skills but also prepares you for technical interviews. This blog post will cover common pitfalls to avoid during interviews that revolve around the Servlet API.
What is the Servlet API?
The Servlet API is a part of Java EE (Enterprise Edition) and provides the necessary classes and interfaces for creating servlets. A servlet is a Java class that handles HTTP requests and responses, running on a web server. It is essential for building Java-based web applications, allowing developers to create dynamic content and manage user sessions.
Key Components of the Servlet API
-
HttpServlet:
- Base class for handling HTTP requests.
- Provides methods like
doGet
,doPost
,doPut
, anddoDelete
to respond to different HTTP methods.
-
ServletConfig:
- Holds servlet configuration information.
- Accessible through the
getServletConfig()
method.
-
ServletContext:
- Provides information about the web application.
- Accessible through the
getServletContext()
method, useful for sharing data among servlets.
-
HttpServletRequest and HttpServletResponse:
- Interfaces that provide methods for reading the request data and writing the response.
Common Interview Pitfalls
- Ignoring the Lifecycle of a Servlet
When asked about servlets, many candidates skip over the servlet lifecycle. It is crucial to understand the stages through which a servlet goes, including:
- Loading: The servlet is loaded into memory.
- Initialization: The
init
method is called, where configuration parameters can be set up. - Request Handling: The servlet handles requests in the
service
method, typically delegating todoGet
,doPost
, etc. - Destruction: The
destroy
method is called when the servlet is taken out of service.
Code Example: Understanding Servlet Lifecycle
import javax.servlet.*;
import java.io.IOException;
public class MyServlet extends HttpServlet {
// This method is called once when the servlet is loaded
@Override
public void init() throws ServletException {
System.out.println("Servlet is being initialized.");
// Initialization code goes here
}
// This method is called for each request
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
System.out.println("Handling GET request.");
response.getWriter().println("Hello, World!");
}
// This method is called once when the servlet is destroyed
@Override
public void destroy() {
System.out.println("Servlet is being destroyed.");
// Clean-up code goes here
}
}
Why This Matters: Understanding the lifecycle helps you identify how to manage resources effectively, set initial parameters, and clean up.
- Confusing ServletContext with ServletConfig
Many candidates mix up ServletContext
and ServletConfig
. While both are crucial, they serve different purposes:
- ServletContext gives information about the entire web application, including application-wide parameters and shared resources.
- ServletConfig is specific to a particular servlet and holds configuration data unique to that servlet.
Key Differences
- Scope:
ServletContext
is application-scoped, whileServletConfig
is servlet-scoped. - Data Sharing:
ServletContext
can be used to share data across different servlets;ServletConfig
cannot.
Code Example: Using ServletContext and ServletConfig
import javax.servlet.*;
import java.io.IOException;
public class ContextConfigServlet extends HttpServlet {
@Override
public void init() throws ServletException {
ServletConfig config = getServletConfig();
String myParam = config.getInitParameter("myParam");
ServletContext context = getServletContext();
String appName = context.getInitParameter("appName");
System.out.println("Parameter from ServletConfig: " + myParam);
System.out.println("Parameter from ServletContext: " + appName);
}
}
Why This Matters: Understanding these differences allows you to utilize the correct context for parameters, promoting better application design.
- Not Handling Exceptions Properly
Exception handling can be a decisive factor during interviews. Candidates often forget to handle exceptions in the servlet correctly, leading to a bad user experience. Always ensure that your servlet can gracefully handle errors.
Code Example: Simple Error Handling
import javax.servlet.*;
import javax.servlet.http.*;
import java.io.IOException;
public class ErrorHandlingServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
try {
int result = 10 / 0; // This will cause an ArithmeticException
} catch (ArithmeticException e) {
response.sendError(HttpServletResponse.SC_BAD_REQUEST, "Invalid calculation");
}
}
}
Why This Matters: Proper error handling enhances user experience and makes your code robust, which interviewers appreciate.
- Overlooking Session Management
Interview candidates often fail to discuss session management, a crucial part of web application development. Servlets use HttpSession
for managing user sessions, enabling the server to associate requests with a specific user.
Code Example: Managing Sessions
import javax.servlet.*;
import javax.servlet.http.*;
import java.io.IOException;
public class SessionServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
HttpSession session = request.getSession();
Integer count = (Integer) session.getAttribute("count");
if (count == null) {
count = 0;
}
count++;
session.setAttribute("count", count);
response.getWriter().println("You have visited this page " + count + " times.");
}
}
Why This Matters: Discussing session management demonstrates your understanding of user state across multiple requests, a critical aspect of any web application.
- Failing to Understand the Deployment Descriptor
The web.xml
file is a deployment descriptor that defines servlet configurations, mappings, and parameters. Failing to discuss it when asked about servlets can be detrimental.
Example of web.xml Configuration
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee" version="3.1">
<servlet>
<servlet-name>MyServlet</servlet-name>
<servlet-class>com.example.MyServlet</servlet-class>
<init-param>
<param-name>myParam</param-name>
<param-value>myValue</param-value>
</init-param>
</servlet>
<servlet-mapping>
<servlet-name>MyServlet</servlet-name>
<url-pattern>/myServlet</url-pattern>
</servlet-mapping>
</web-app>
Why This Matters: Familiarity with web.xml
shows that you understand how servlets are configured and managed in a web application.
Bringing It All Together
Mastering the Servlet API is not just about knowing the syntax; it involves understanding the concepts, lifecycle, and usages in a real-world application. Avoiding the common interview pitfalls discussed above will prepare you to impress your interviewers and land that desired position.
To learn more about Java servlets, consider exploring the Java EE official documentation. Practice the code snippets included here in a test environment to see how they function. Good luck on your interview journey!
This is an excellent starting point for mastering the Servlet API and preparing for your next interview. Make sure to stay updated on the latest features and best practices in Java.