Accessing Data in Thymeleaf: Common Pitfalls to Avoid
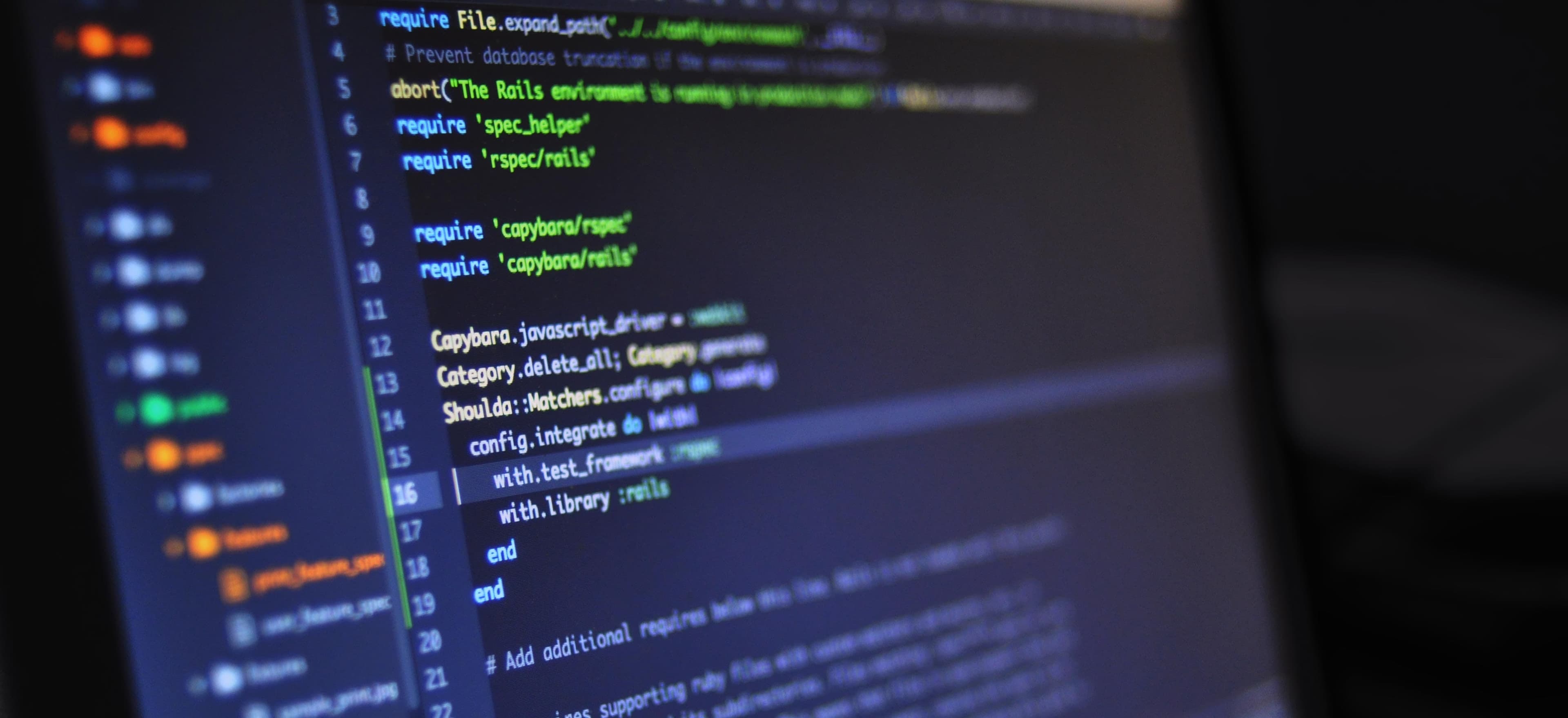
- Published on
Accessing Data in Thymeleaf: Common Pitfalls to Avoid
Thymeleaf has grown into one of the most widely used Java templating engines, particularly in Spring applications. It offers an intuitive way to render views while maintaining seamless integration with Spring MVC. However, like any powerful tool, being prone to certain pitfalls is all too common. In this post, we will explore some common mistakes developers encounter when accessing data in Thymeleaf and how to avoid them, ensuring your web applications run smoothly.
What is Thymeleaf?
Thymeleaf is a modern server-side Java template engine. It allows users to create dynamic HTML pages in a straightforward manner, enabling the separation of concerns, which is vital in programming. Thymeleaf is primarily used for web applications, but it can be useful in other scenarios too, such as generating emails or rendering XML.
Getting Started with Thymeleaf
To use Thymeleaf, you begin with a template structure often found in HTML files. These templates are enriched with Thymeleaf attributes that allow for dynamic content rendering.
Basic Setup
To start, ensure you have the necessary dependencies. If you are using Maven, add the following dependency to your pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
This line will enable Thymeleaf in your Spring Boot application, giving you access to its rich features.
Common Pitfalls When Accessing Data
While Thymeleaf is powerful, developers can often trip over common pitfalls. Here are key areas to navigate carefully.
1. Incorrect Object Context
One of the most typical pitfalls when using Thymeleaf is the incorrect context object being passed to the view. For instance, if you want to access an attribute called user
, you should ensure that the controller method provides it correctly.
Example Controller
@Controller
public class UserController {
@GetMapping("/user")
public String getUser(Model model) {
User user = new User("John", "Doe"); // Sample User
model.addAttribute("user", user);
return "user"; // points to `user.html` template
}
}
Thymeleaf Template Snippet
<p th:text="${user.firstName}">Default Name</p>
In the example above, by referencing user.firstName
in the template, Thymeleaf expects the user
object to exist in the model. An oversight in assigning the object can lead to a NullPointerException.
2. Misusing the th:if
and th:unless
Attributes
th:if
and th:unless
are powerful attributes for conditional rendering. However, misuse can lead to incomplete UI updates or unintended static content.
Correct Usage
Suppose you want to render user details only if a user is logged in:
<div th:if="${user != null}">
<p th:text="${user.fullName}">Anonymous User</p>
</div>
In this example, the user details will display only when the user
object is not null.
Common Mistake
Avoid using th:if
without understanding its impact on the rendered HTML. It creates an entirely different tree, which can lead to missing elements.
3. Thymeleaf and Collections: Indexing
Accessing list elements in Thymeleaf can be tricky due to how indexing works. If you are iterating through a list, you might be tempted to directly access an index.
Correct Iteration
Here's how to properly iterate through a list:
@GetMapping("/users")
public String getUsers(Model model) {
List<User> users = List.of(new User("John", "Doe"), new User("Jane", "Doe"));
model.addAttribute("users", users);
return "users"; // points to `users.html` template
}
<ul>
<li th:each="user : ${users}" th:text="${user.fullName}"></li>
</ul>
In this example, the th:each
attribute effectively handles the iteration without needing manual indexing, which can introduce bugs if not handled correctly.
4. Not Using the @ModelAttribute
Annotations
When you want to bind user input in forms and display model data, the @ModelAttribute
annotation is your friend. Forgetting it can lead to data not binding as expected.
Example Usage
@PostMapping("/user")
public String postUser(@ModelAttribute User user) {
// Process the user object
return "redirect:/users";
}
This ensures that data from a form submission maps directly to the User object, which can then be used in the Thymeleaf templates.
5. Failing to Escape User-Generated Content
Security is paramount in web applications. Failing to escape user-generated content can lead to XSS vulnerabilities. Thymeleaf automatically escapes content in text attributes, but when generating HTML, you need to be cautious.
Example of Safe Rendering
<p th:utext="${user.bio}"></p> <!-- Avoid this unless trusted -->
In the example above, using th:utext
to render user bio must be used carefully as it bypasses escaping.
6. Confusing URL and Path Variables
When working with Thymeleaf templates, managing the mapping of URL variables can surprise even seasoned developers. Using them incorrectly can lead to broken links.
Example of Correct Path Variable Usage
@GetMapping("/user/{id}")
public String findUserById(@PathVariable Long id, Model model) {
User user = userService.findUserById(id);
model.addAttribute("user", user);
return "user";
}
Thymeleaf Template Link Example
<a th:href="@{/user/{id}(id=${user.id})}">View User</a>
Remember to use the correct syntax to ensure the variable is substituted properly, maintaining consistency in routing.
The Bottom Line
Thymeleaf is a robust tool for rendering views in Spring applications, but it carries with it a set of common pitfalls. By keeping a close eye on the object context, using conditional attributes wisely, iterating collections correctly, binding user input appropriately, escaping user-generated content, and managing URL variables correctly, you can navigate these challenges effectively.
Further Reading
For more extensive guidelines and advanced usage, you can check out the Thymeleaf Documentation and Spring Framework Documentation for deeper insights into integrating Thymeleaf seamlessly within your applications.
With these insights, you can avoid common pitfalls and develop clean, efficient, and secure web applications using Thymeleaf. Happy coding!