Common Selenium Mistakes Newbies Make and How to Avoid Them
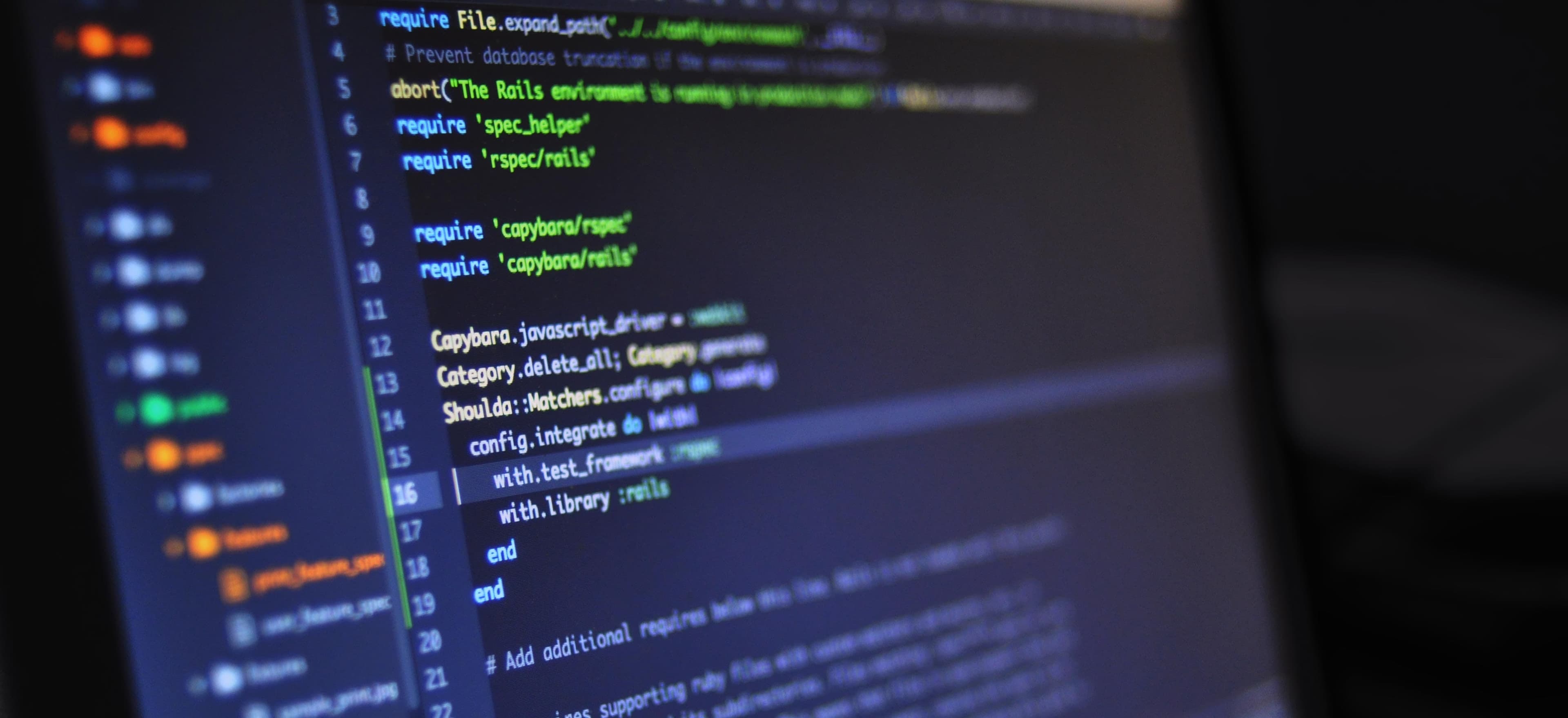
- Published on
Common Selenium Mistakes Newbies Make and How to Avoid Them
Selenium is a powerful tool for automating web applications for testing purposes. However, new users often struggle with some common pitfalls that can lead to ineffective scripts and wasted time. This blog post will highlight these mistakes and provide actionable solutions to help you become a more proficient Selenium user. Let's dive right in.
1. Ignoring Implicit and Explicit Waits
The Mistake
One of the most common mistakes newbies make is ignoring waits. They often proceed with scripts that attempt to interact with web elements before they are fully loaded, leading to ElementNotFoundException
errors.
The Solution
Using Implicit Waits and Explicit Waits can help prevent these issues. Implicit waits set a time frame for the existence of elements, while explicit waits allow you to wait for a specific condition to occur.
Implicit Wait Example
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class ImplicitWaitExample {
public static void main(String[] args) {
WebDriver driver = new ChromeDriver();
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
driver.get("https://example.com");
// Trying to locate an element, the implicit wait will help if the element takes time to load.
driver.findElement(By.id("exampleId")).click();
driver.quit();
}
}
Why Use Implicit Waits? They provide a global wait time for all elements, which can simplify your script when dealing with numerous elements.
Explicit Wait Example
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class ExplicitWaitExample {
public static void main(String[] args) {
WebDriver driver = new ChromeDriver();
driver.get("https://example.com");
WebDriverWait wait = new WebDriverWait(driver, 10);
wait.until(ExpectedConditions.elementToBeClickable(By.id("exampleId"))).click();
driver.quit();
}
}
Why Use Explicit Waits? You can specify exactly what condition you are waiting for, instead of timing out after a set period.
2. Lack of Clear Locators
The Mistake
Another common mistake is using unreliable locators. Relying solely on id
or class
without ensuring their uniqueness can lead to confusing errors.
The Solution
Always prefer using unique locators—either by id
, CSS Selectors
, or XPath
. When appropriate, combine locators to ensure stability.
Example of Using XPath
driver.findElement(By.xpath("//div[@class='exampleClass']/button")).click();
Why Use XPath? XPath allows for more complex queries, enabling you to traverse the DOM to find elements reliably.
3. Hardcoding Values
The Mistake
Newbies often hardcode values in their Selenium scripts, leading to brittle tests that break easily with minor UI changes.
The Solution
Utilize configuration files or environment variables to store values that may change. This approach allows you to update your tests flexibly and appropriately.
Example of Using Properties File
import java.io.FileInputStream;
import java.io.IOException;
import java.util.Properties;
public class Configuration {
Properties properties;
public Configuration() {
properties = new Properties();
try {
FileInputStream input = new FileInputStream("config.properties");
properties.load(input);
} catch (IOException e) {
e.printStackTrace();
}
}
public String getUrl() {
return properties.getProperty("url");
}
}
Why Use a Configuration File? It centralizes your adjustable values, promoting better maintainability.
4. Not Understanding Page Object Model (POM)
The Mistake
New Selenium users often write scripts in a linear and unstructured way, leading to code that is hard to read and maintain.
The Solution
Implement the Page Object Model (POM) design pattern. This pattern enhances script clarity by separating page structure from test logic.
Example of Page Object Model
public class LoginPage {
private WebDriver driver;
// Elements
private By usernameField = By.id("username");
private By passwordField = By.id("password");
private By loginButton = By.id("login");
public LoginPage(WebDriver driver) {
this.driver = driver;
}
public void enterUsername(String username) {
driver.findElement(usernameField).sendKeys(username);
}
public void enterPassword(String password) {
driver.findElement(passwordField).sendKeys(password);
}
public void clickLogin() {
driver.findElement(loginButton).click();
}
}
Why Use POM? It promotes reusability and better organization of your tests.
5. Not Cleaning Up Resources
The Mistake
Failing to close the WebDriver instances properly can lead to resource leaks, which may eventually crash your test execution environment.
The Solution
Ensure to call driver.quit()
or driver.close()
at the end of your scripts.
Example of Clean-Up Code
public class CleanUpExample {
public static void main(String[] args) {
WebDriver driver = new ChromeDriver();
try {
driver.get("https://example.com");
// Perform actions
} finally {
driver.quit(); // Ensure driver is closed
}
}
}
Why Ensure Clean-Up? It prevents memory leaks and ensures that your automation testing environment remains resource-efficient.
Wrapping Up
Selenium is a robust framework for web application testing, but like any tool, it comes with its challenges. By avoiding these common mistakes, you will write cleaner, more effective, and maintainable tests.
As you advance in your Selenium journey, continuously educate yourself about best practices and design patterns. Experiment with different approaches, learn from your mistakes, and always keep improving.
For further reading on Selenium best practices, visit the SeleniumHQ Documentation to deepen your understanding and keep your knowledge up-to-date.
By being aware of these common pitfalls and implementing more effective strategies, you will ensure that your Selenium testing experience is not just productive, but also enjoyable. Happy Testing!
Checkout our other articles