Securing Remote JMS Clients on OpenShift: Key Challenges
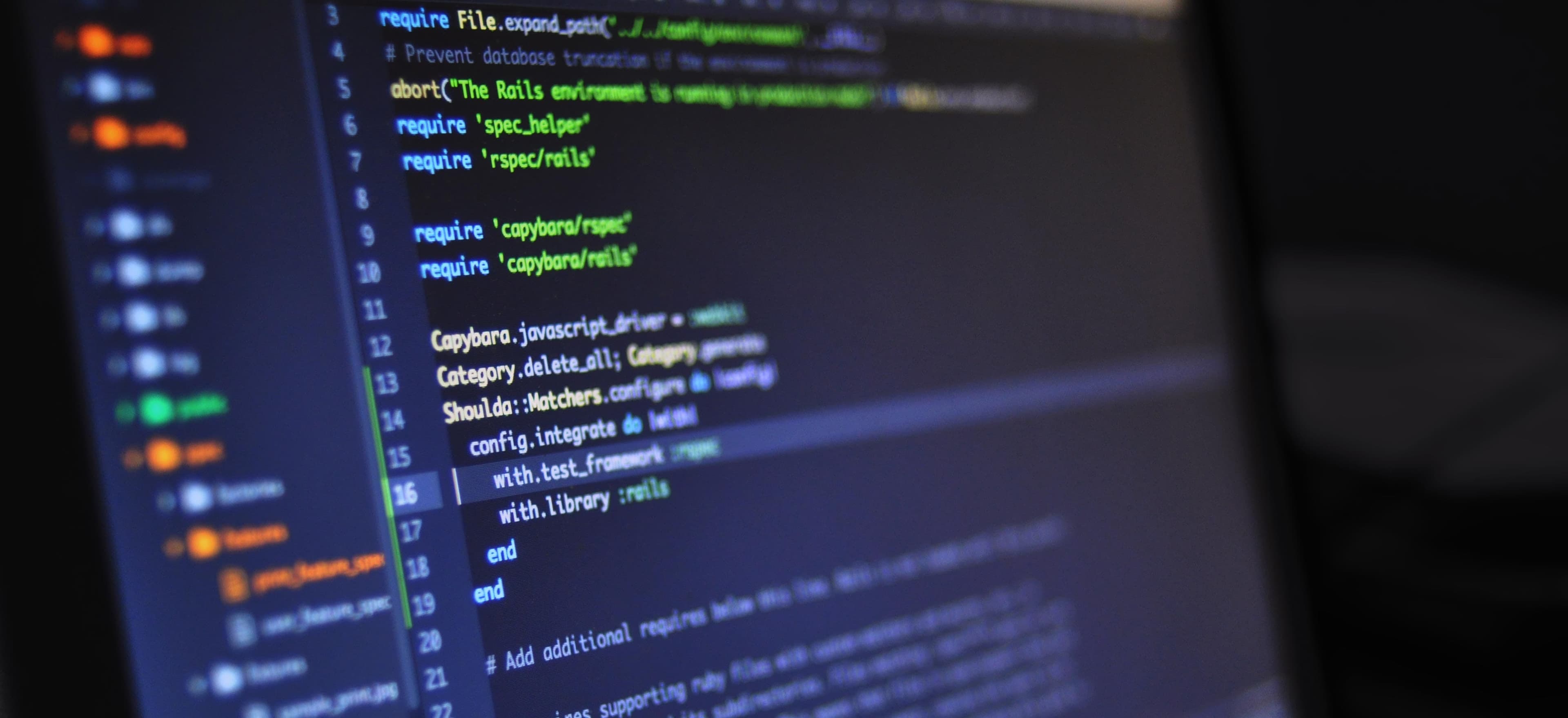
- Published on
Securing Remote JMS Clients on OpenShift: Key Challenges
As enterprises increasingly transition to cloud-native architectures, the need for secure communication between distributed components becomes paramount. One such component is Java Message Service (JMS) clients, which play a crucial role in enterprise messaging systems. This article delves into the challenges of securing remote JMS clients on OpenShift, offering insights and solutions for effective configurations.
Understanding JMS and Its Role in Cloud Applications
JMS is a Java API that provides a way for Java applications to create, send, receive, and read messages. In cloud environments, especially on platforms like OpenShift, the decoupled nature of messaging can significantly enhance scalability, reliability, and flexibility.
However, with these benefits come security risks, particularly when it comes to remote JMS clients. Since these applications connect over the internet or a corporate network, various threats may compromise message integrity, confidentiality, and availability.
Key Challenges in Securing Remote JMS Clients on OpenShift
1. Network Security
One of the most significant challenges is ensuring secure communication over the network. Unencrypted data transmission can expose sensitive information. Here are a few measures to address this challenge:
- Transport Layer Security (TLS): Implementing TLS ensures that messages are encrypted during transit. This requires configuring both the JMS server and the client to establish a secure connection.
Here is a basic example of implementing TLS:
import javax.jms.*;
import javax.naming.InitialContext;
// Secure JMS Connection Factory
ConnectionFactory connectionFactory = (ConnectionFactory) new InitialContext().lookup("jms/secureConnectionFactory");
Connection connection = connectionFactory.createConnection("username", "password");
connection.start();
This code snippet shows how to set up a secure connection using JMS. The use of a secure connection factory with authentication ensures message security.
2. Authentication and Authorization
Authenticating users and authorizing access to resources is vital in any messaging application. OpenShift provides several mechanisms to enforce security, but you must implement them correctly.
- Client Certificates: Using client certificates for authentication allows JMS brokers to verify clients before allowing access. This can prevent unauthorized users from sending or receiving messages.
For initiating a secure connection with client certificates, you might configure the SSL context similar to the following:
System.setProperty("javax.net.ssl.keyStore", "path/to/keystore");
System.setProperty("javax.net.ssl.keyStorePassword", "password");
System.setProperty("javax.net.ssl.trustStore", "path/to/truststore");
System.setProperty("javax.net.ssl.trustStorePassword", "password");
new InitialContext();
Setting up the keystores for SSL-based communication is crucial for securing JMS clients. It involves configuring both the key and trust stores.
3. Message Integrity and Confidentiality
Ensuring that messages are not tampered with during transit is critical. OpenShift environments might expose applications to various vulnerabilities, potentially leading to unauthorized message modifications.
- Message Signing: Implementing message signing ensures that only the intended sender can produce the message. This can be achieved through digital signatures.
Here’s how to create a signed message:
import javax.jms.*;
import java.security.*;
Message message = session.createTextMessage("Hello Secure World!");
Signature signature = Signature.getInstance("SHA256withRSA");
signature.initSign(privateKey);
signature.update(message.getBody(String.class).getBytes());
byte[] signedMessage = signature.sign();
By signing your messages, you provide a means to verify their integrity and authenticity when received.
4. Securing Message Brokers
The JMS broker acts as the central point for message transmission. Its security is paramount. Vulnerabilities in the broker can expose all communication to interception and manipulation.
- Role-Based Access Control (RBAC): Utilize RBAC to restrict which clients can publish or consume messages, thus minimizing exposure.
For example, if using Apache ActiveMQ as your broker, configure your broker properties to manage user roles effectively through XML or YAML configurations.
<plugins>
<jaasAuthenticationPlugin>
<jaasConfigFile>${activemq.home}/etc/users.properties</jaasConfigFile>
</jaasAuthenticationPlugin>
</plugins>
This configuration secures the broker by defining user authentication and their roles, ensuring only legit clients can send or receive messages. The users' properties file specifies usernames and passwords.
5. Managing Secrets and Credentials
In a cloud environment, managing and securing credentials is essential. Hard-coding credentials in your application is a common pitfall.
- Using Kubernetes Secrets: OpenShift leverages Kubernetes secrets to store sensitive information securely. Use those instead of embedding credentials in your source code.
Example of creating a secret for your JMS configuration:
oc create secret generic jms-auth --from-literal=username=myuser --from-literal=password=mypassword
This command generates a secret that can be referenced by your applications to retrieve necessary credentials securely without hard-coding them.
Wrapping Up
Securing remote JMS clients on OpenShift involves a multi-faceted approach encompassing network security, authentication, message integrity, broker security, and credential management. Each challenge presents its own complexities, but with best practices and proper tools, organizations can build a robust messaging infrastructure that safeguards their data.
To dive deeper into securing JMS clients, consider exploring JMS Security Best Practices and the security features offered by OpenShift.
By navigating these challenges effectively, businesses can leverage the advantages of JMS while maintaining the highest levels of security. Ensure you stay updated with the latest security practices, as the landscape continues to evolve alongside technologies.
Checkout our other articles