Overcoming Challenges in Consumer-Driven Contract Testing
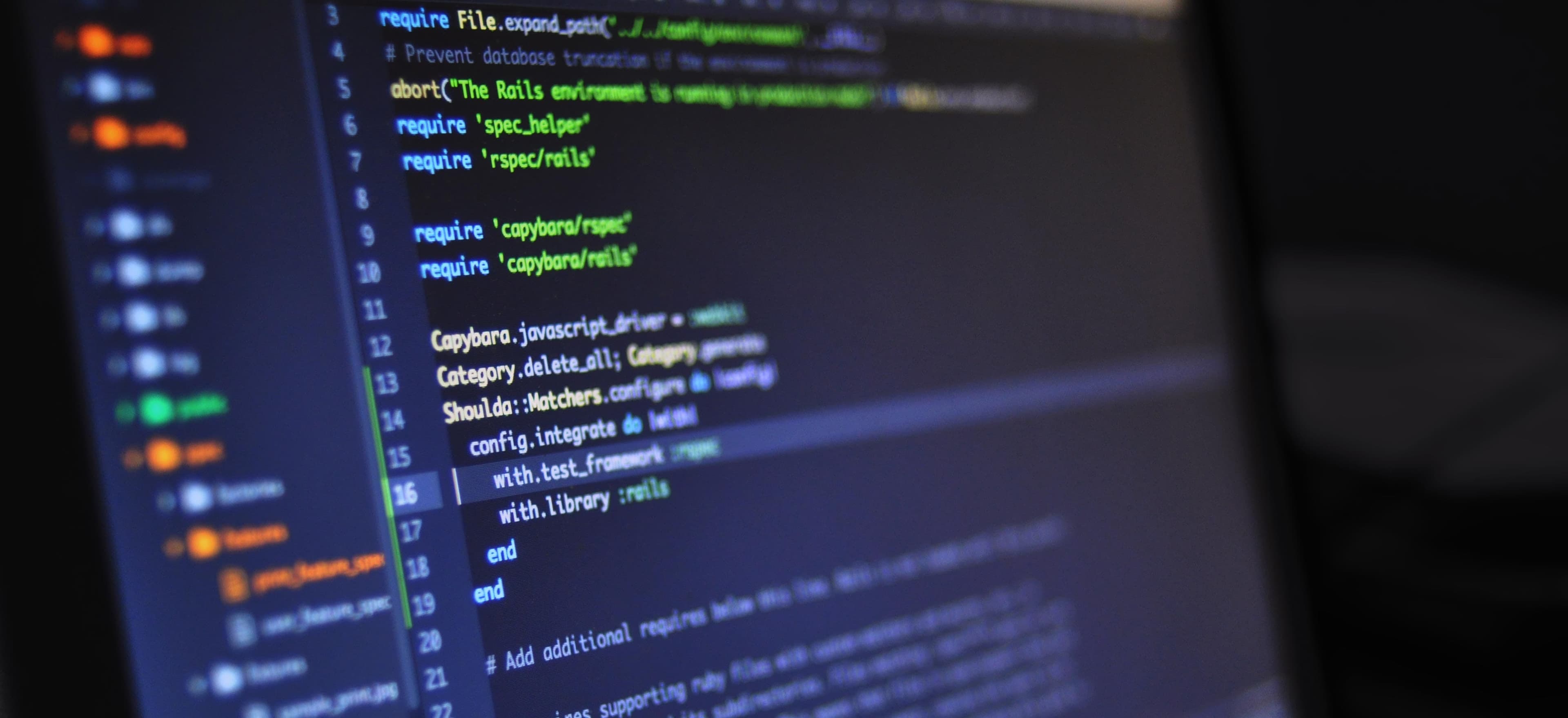
- Published on
Overcoming Challenges in Consumer-Driven Contract Testing
In today's software development landscape, ensuring reliability and compatibility between microservices is paramount. One approach that has gained traction in recent years is Consumer-Driven Contract Testing (CDCT). It enables teams to define and validate the expected interactions between services, reducing integration issues. However, while CDCT brings many benefits, it also presents several challenges. In this blog post, we will explore these challenges and discuss strategies to overcome them.
What is Consumer-Driven Contract Testing?
Consumer-Driven Contract Testing is a methodology where the consumer of a service defines its expectations (the contract), which the provider implementation must adhere to. The idea is simple: if the consumer can validate the contract against the provider, integration becomes a seamless experience.
For example, imagine a payment service that needs to communicate with an inventory service. The payment service can define what to expect in the response from the inventory service, ensuring that it can process the transaction accurately.
Benefits of CDCT
Before we dive into the challenges, let's quickly highlight the benefits of CDCT:
- Reduced Integration Issues - By having a clear contract that outlines expectations, teams can identify errors early.
- Better Collaboration - Consumers and providers can work together effectively to ensure mutual understanding.
- Faster Iteration - Teams can iterate quickly thanks to automated tests based on contracts.
The Challenges of Consumer-Driven Contract Testing
Despite the advantages of CDCT, teams often encounter roadblocks. Let’s take a closer look at some of the most common challenges and how to overcome them.
1. Evolving Consumer Requirements
One of the most significant challenges in CDCT is that consumer requirements can change frequently. When a consumer updates its needs, it may invalidate existing contracts, leading to frequent breakages.
Solution: Continuous Integration and Deployment (CI/CD)
By implementing a robust CI/CD pipeline, teams can automate contract verification whenever a change is made. This ensures that any change in consumer requirements is instantly tested against the provider, allowing for quick identification and resolution of issues.
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class InventoryServiceContractTest {
@Test
public void testInventoryResponse() {
// Simulating the response from the inventory service
String expectedResponse = "{\"available\":true,\"quantity\":5}";
String actualResponse = getInventoryResponse();
assertEquals(expectedResponse, actualResponse);
}
private String getInventoryResponse() {
// Simulate a call to the inventory service
return "{\"available\":true,\"quantity\":5}"; // Example response
}
}
In the above code snippet, we define a unit test that checks if the response from the inventory service meets the consumer's expectations. This test should run every time there is a new deployment, ensuring the contract is upheld.
2. Lack of Standardization
Teams may implement contract tests differently, leading to inconsistencies in how contracts are defined and validated. This lack of standardization can cause confusion and errors.
Solution: Use of Tools and Frameworks
Using contract testing frameworks such as Pact or Spring Cloud Contract can facilitate standardization. These tools offer a consistent way to define, verify, and publish contracts, making it easier for teams to collaborate.
For instance, here is how you can define a simple contract using Pact:
import au.com.dius.pact.consumer.Pact;
import au.com.dius.pact.consumer.ConsumerPactBuilder;
import au.com.dius.pact.consumer.junit.PactConsumerTest;
import au.com.dius.pact.consumer.junit.RestPactTestRule;
public class InventoryServiceConsumerTest extends PactConsumerTest {
@Pact(consumer = "PaymentService", provider = "InventoryService")
public RequestResponsePact createInventoryServicePact(PactDslWithProvider builder) {
return builder
.given("inventory exists for item 1")
.uponReceiving("A request for inventory")
.path("/inventory/1")
.method("GET")
.willRespondWith()
.status(200)
.body("{\"available\":true,\"quantity\":5}")
.toPact();
}
}
In the above example, a pact is created where the PaymentService
consumes a response from the InventoryService
. This clearly defines the expectations and ensures other team members can understand the contract at a glance.
3. Keeping Contracts Up to Date
When a provider changes its implementation, it may inadvertently break contracts set by consumers, especially if those contracts are not updated accordingly. Keeping contracts up to date becomes a cumbersome task as the number of services increases.
Solution: Contract Ownership and Maintenance
Assign contract owners who are responsible for keeping the contracts updated. This could be the developer team responsible for either the consumer or provider, ensuring someone is accountable for their correctness.
Additionally, consider implementing a contract versioning strategy. By having a version control system for contracts, both consumers and providers can evolve independently:
version: 1.1.0
contracts:
- id: "inventory_responses"
consumer: "PaymentService"
provider: "InventoryService"
interactions:
- description: "Get inventory for item 1"
request:
method: "GET"
path: "/inventory/1"
response:
status: 200
body: "{ \"available\": true, \"quantity\": 5 }"
The use of a YAML file to define contract versions enables easier tracking of changes and promotes better communication between teams.
4. Testing the Edge Cases
CDCT often leads teams to focus primarily on the happy path – the straightforward, expected scenarios. This can result in inadequate validation of edge cases, which can lead to unexpected runtime errors.
Solution: Comprehensive Test Coverage
Incorporate thorough test cases, including edge cases, into your contract tests. Here’s an example of a JUnit test that checks various scenarios.
@Test
public void testInventoryEdgeCases() {
// Assuming mock service to test various scenarios
assertInventoryResponse("/inventory/0", "{\"error\": \"Item not found\"}"); // Non-existent item
assertInventoryResponse("/inventory/1", "{\"available\":false,\"quantity\":0}"); // Sold out
}
private void assertInventoryResponse(String path, String expectedResponse) {
String actualResponse = getInventoryResponse(path);
assertEquals(expectedResponse, actualResponse);
}
By explicitly testing edge cases, the team can dramatically reduce the chances of runtime failures and ensure a robust interaction between services.
5. Communication Breakdowns
Even when contracts are defined, poor communication can lead to misunderstandings and incorrect implementations. Without clear dialogue between consumer and provider teams, discrepancies can arise.
Solution: Foster a Culture of Collaboration
Tools like Slack or Microsoft Teams can help facilitate real-time discussions, while regular stand-ups or review meetings can improve awareness of ongoing changes. Moreover, collaborative tools like Camunda for workflow automation and Jira for project management can enhance transparency.
Creating shared documentation that outlines service contracts and expectations can also aid communication, making it easier to keep everyone on the same page.
A Final Look
Consumer-Driven Contract Testing presents a powerful methodology for managing microservice interactions. While challenges abound, teams can overcome them by adopting CI/CD practices, utilizing standardized tools, maintaining contracts diligently, ensuring comprehensive test coverage, and promoting clear communication.
By harnessing the power of CDCT, teams can create a seamless integration experience and reduce the likelihood of conflicts in microservice architectures. As your development process matures, embracing these strategies will enhance the reliability of your service interactions, ultimately leading to a better user experience and more robust software applications.
Checkout our other articles