Overcoming Challenges in Android Migrations: A Guide
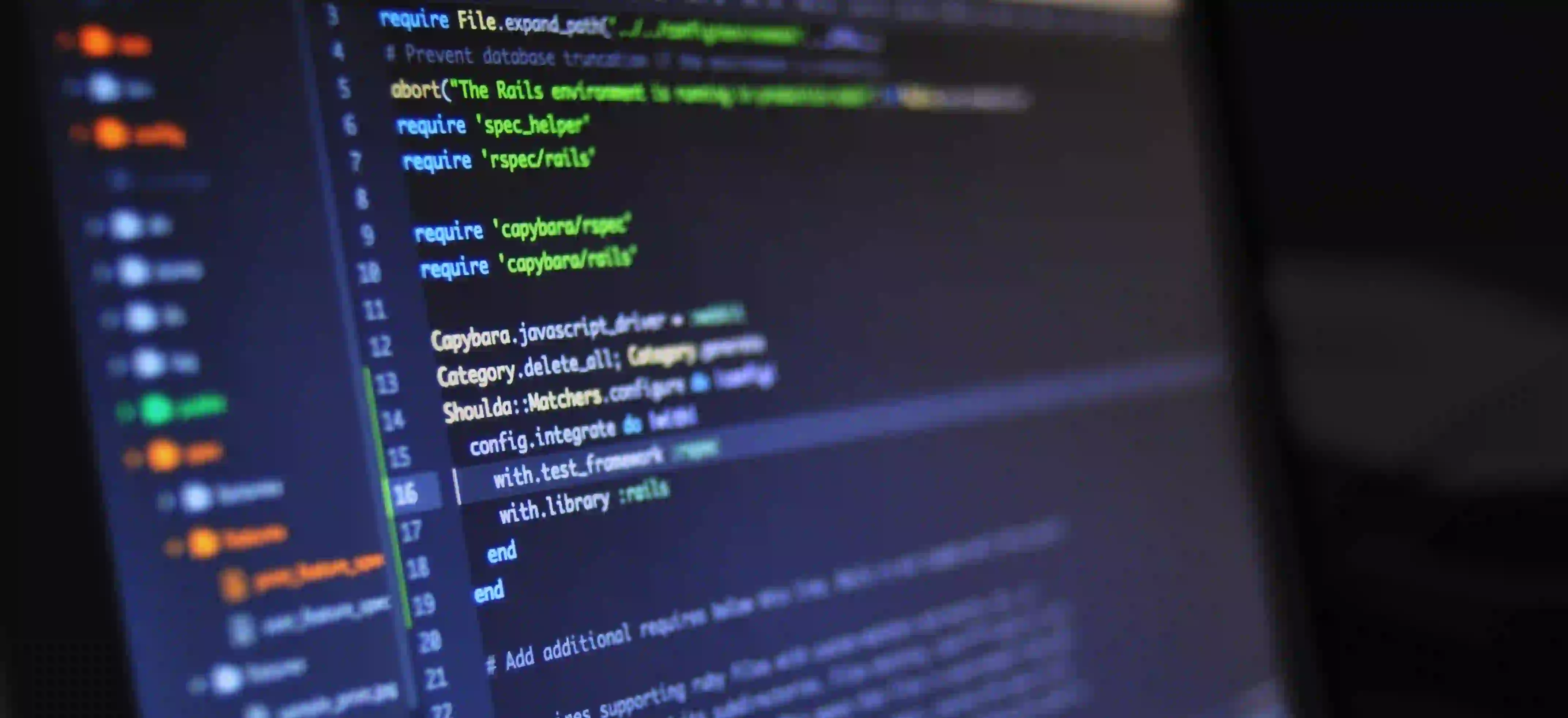
Overcoming Challenges in Android Migrations: A Comprehensive Guide
With the rapid evolution of technology, Android app developers frequently face the challenge of migrating their applications to newer versions or frameworks. Migrations can present a myriad of obstacles that, if not addressed properly, can lead to increased development time, bugs, and performance issues. In this guide, we will explore the common challenges encountered when migrating Android applications and provide practical solutions to overcome them.
Understanding Android Migrations
Migrations refer to the process of moving your application codebase from one version of the Android platform to another or transitioning to a new architecture, such as from MVC to MVVM. This can involve updating dependencies, refactoring code, and ensuring compatibility with newer API levels.
Common Challenges of Android Migrations
-
API Changes New versions of Android often come with updated APIs. A method may be deprecated, and new alternatives introduced. This requires developers to understand the changes and adapt their code accordingly.
-
Third-party Library Compatibility Many applications rely on third-party libraries which may not be up-to-date with the latest Android versions. This can lead to version conflicts or problems when new features are introduced.
-
Gradle Build System Adjustments The Gradle build system is a pivotal element in Android development. Migrating often involves adjustments to the
build.gradle
file due to new dependencies or plugin versions. -
Testing and Debugging Migrations can introduce unexpected bugs. Adequate testing is necessary to identify issues stemming from the migration process.
-
User Experience Consistency With new UI components and libraries, maintaining a consistent user experience across different Android versions can be challenging.
Effective Strategies for Overcoming Migration Challenges
1. Preparing and Planning
Before embarking on a migration journey, thorough preparation and planning are paramount. Here are some practical steps:
-
Audit Your Code Review your existing codebase to identify deprecated APIs or third-party library versions that may not support the new Android version. Use tools like Lint to help identify potential issues.
-
Read the Release Notes Go through the release notes for the newer Android version to understand changes, deprecated methods, and new features.
Here’s a simple example of how to audit deprecated APIs:
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.N) {
// Use new method available from N onward
newMethod();
} else {
// Fallback for older versions
deprecatedMethod();
}
2. Upgrade Third-party Libraries
Ensure all third-party libraries used in your project are updated to their latest versions. You can utilize Maven or Gradle plugins to manage dependencies effectively.
A Gradle snippet for upgrading libraries looks like this:
dependencies {
implementation 'com.squareup.retrofit2:retrofit:2.9.0'
implementation 'com.squareup.okhttp3:logging-interceptor:4.9.0'
}
This code ensures you're leveraging the latest, most stable features from your libraries.
3. Modify the Gradle Build File
Updating the build.gradle
file is crucial when migrating. Pay particular attention to the compileSdkVersion
, targetSdkVersion
, and the dependencies section.
Example of a relevant section in the build.gradle
file:
android {
compileSdkVersion 34
defaultConfig {
applicationId "com.example.myapp"
minSdkVersion 21
targetSdkVersion 34
versionCode 1
versionName "1.0"
}
}
Updating these values ensures that your application compiles and runs correctly in line with new SDK features.
4. Conduct Thorough Testing
Use automated testing frameworks like JUnit and Mockito to carry out unit tests. For UI testing, consider tools like Espresso or UI Automator to ensure that the user interface remains intact throughout the migration.
5. Manage User Experience
Utilize features like Feature Flags to gradually roll out new functionalities, minimizing disruption. This approach allows you to test new features with a subset of users before a full deployment.
Example of a Feature Flag Implementation:
if (isFeatureEnabled("newFeature")) {
// Launch new UI or functionality
launchNewFeature();
} else {
// Fallback to old UI
launchOldFeature();
}
By managing features this way, you can maintain a consistent user experience while still evolving your app.
Keeping Communication Open
Migrations can be complicated, and communication within your development team is essential. Use collaborative tools like JIRA, Trello, or Slack to keep everyone informed and aligned.
Documentation Matters
Always maintain thorough documentation. Keep a record of what changes were made during the migration process, testing strategies, and updates to third-party libraries. Well-documented code takes the guesswork out of future migrations.
The Closing Argument
Migrating an Android application might feel daunting, but with proper planning and execution, you can navigate through the challenges successfully. From preparing your codebase to ensuring third-party library compatibility, every step counts towards a smoother migration process.
Remember, continuous testing and clear communication are your allies in overcoming migration obstacles. Embrace the learning curve, and soon enough, you'll be well-equipped to tackle the next migration.
For more information on Android app development, check out the official Android Developer Guide. Happy coding!