Finding the Right Balance: Classes vs. Packages in Code
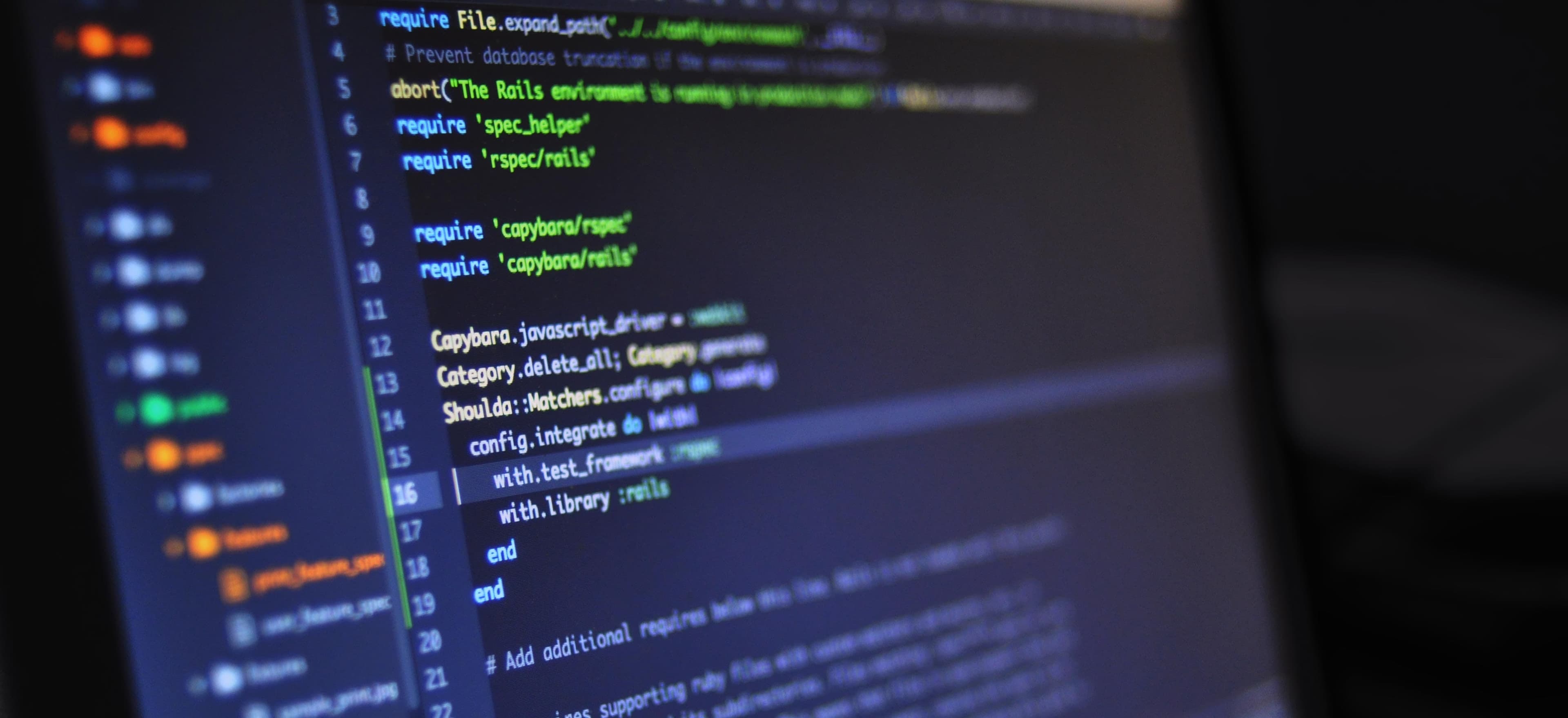
- Published on
Finding the Right Balance: Classes vs. Packages in Code
In the realm of Java development, two fundamental concepts stand tall: classes and packages. While both serve as integral components of object-oriented programming, they come with unique responsibilities and play critical roles in structuring code. The challenge lies in leveraging both effectively to create maintainable and scalable applications. In this blog post, we will explore the nuances between classes and packages, examine best practices in their usage, and illustrate the principles with code examples, allowing developers to find the right balance between the two.
Understanding Classes and Packages
What is a Class?
A class in Java serves as a blueprint for creating objects. It encapsulates data for the object and methods to manipulate that data. Think of it as a template that defines the structure and behavior of objects.
Example: Defining a Class
public class Car {
// Properties of the class
private String make;
private String model;
private int year;
// Constructor to initialize the Car object
public Car(String make, String model, int year) {
this.make = make;
this.model = model;
this.year = year;
}
// Method to display car details
public void displayCarInfo() {
System.out.println(year + " " + make + " " + model);
}
}
In this example, the Car
class contains three properties: make
, model
, and year
. It uses a constructor to create an instance and a method displayCarInfo
to provide information about the car. This encapsulation is a key principle of object-oriented programming, promoting data hiding and modularity.
What is a Package?
A package, on the other hand, is a namespace that organizes a set of related classes and interfaces. It helps to avoid name conflicts and can control access through visibility modifiers. Packages structure your application hierarchically and aid in the management of a large codebase.
Example: Creating a Package
// File: com/example/vehicles/Car.java
package com.example.vehicles;
public class Car {
// Class details... (same code as above)
}
In this snippet, the Car
class is placed within a package named com.example.vehicles
. This categorization allows for clear organization and helps identify where the class fits within the larger project.
The Importance of Balancing Classes and Packages
Creating a robust application requires more than just writing code. It involves careful planning and architecture. Balancing classes and packages is key to achieving manageable and understandable code. Here are a few considerations to keep in mind.
Readability and Maintainability
When classes are organized into relevant packages, your code becomes more readable. Developers should easily navigate the codebase and understand the relationships between classes. This is crucial for long-term maintenance.
Keep It Organized: Group classes that share functionality into the same package.
Example Advantage: If you have classes for user management (User
, UserService
, UserController
), placing them in a package like com.example.usermanagement
promotes clarity.
Encapsulation and Modularity
Packages encapsulate related classes, restricting access to certain parts of your codebase. This modularity allows developers to work on different sections without interfering with others. It also promotes reuse of common functionality.
Example: If your application has a set of utility classes (StringUtils
, DateUtils
), placing them in a package called com.example.utils
allows you to utilize these across various parts of the application without cluttering your main codebase.
Avoiding Class Overcrowding
One of the most common mistakes is to overload a single package with too many classes. This can lead to confusion and make finding the right component difficult.
Best Practice: Aim for a few classes per package that are cohesive and have a common purpose.
Linking the Concepts: Packages as Containers for Classes
Classes can be thought of as the building blocks of your application while packages are the containers that house these blocks. Just as a home requires a skilled architect to design its layout, your application needs a structure built on logical packages housing related classes.
When to Use Classes over Packages
While both classes and packages are critical to an effective Java application, certain situations call for prioritizing one over the other.
Focus on Reusability with Classes
If your application requires multiple instances of similar behavior or attributes, classes are essential. By leveraging inheritance and polymorphism, you can create robust hierarchies that maximize code reuse.
Example: Extending Classes
public class SUV extends Car {
private int groundClearance;
public SUV(String make, String model, int year, int groundClearance) {
super(make, model, year);
this.groundClearance = groundClearance;
}
public void displayOffRoadAbilities() {
System.out.println("Ground clearance: " + groundClearance + " inches.");
}
}
In this example, the SUV
class extends the Car
class, showcasing how subclasses enhance core functionalities while retaining shared properties and methods.
Prioritize Logical Grouping with Packages
When your codebase is getting unwieldy, focus on refining your package structures. Creating additional packages for related functionalities can simplify navigation and increase compilation efficiency.
Package Structure Example:
- com.example.vehicles (contains classes related to vehicles)
- Car.java
- Truck.java
- com.example.usermanagement (contains classes related to user operations)
- User.java
- UserService.java
This logical grouping allows developers to easily find the classes they need while keeping related functionality contained.
Best Practices for Working with Classes and Packages in Java
-
Follow Naming Conventions: Adopt a consistent naming strategy for packages and classes. Typically, package names are in lowercase (e.g.,
com.example.vehicles
), while class names are in CamelCase (e.g.,Car
). -
Limit the Size of Packages: Aim to keep classes bundled in a way that makes sense. If a package contains too many classes or becomes too large, it may be time to refactor.
-
Organize by Functionality: Group classes that serve a common purpose into packages. This not only improves clarity but also boosts collaboration among development teams.
-
Document Your Code: Use JavaDoc comments extensively. Clear documentation not only benefits you but also helps other developers who may work on the codebase later.
-
Utilize Access Modifiers: Carefully consider the protection level of your classes and packages. Default (package-private) classes and methods can limit access to within the package, which can be very beneficial when designing modular systems.
My Closing Thoughts on the Matter
Finding the right balance between classes and packages in a Java application is paramount for creating a well-structured and maintainable codebase. Classes represent the functional units of your application, while packages provide the organizational framework necessary to manage complexity. By adhering to best practices and focusing on readability, modularity, and logical organization, developers can create scalable applications that stand the test of time.
For those looking to dive deeper into Java's structure, you may find valuable resources on Oracle’s Java Documentation and Baeldung's Building Java Packages helpful as you navigate the world of object-oriented programming.
Embrace the power of classes and packages in your Java development journey, and enjoy the clarity and efficiency a coherent structure can bring.
Checkout our other articles