Understanding Lambda Functions and Final Variables in Java
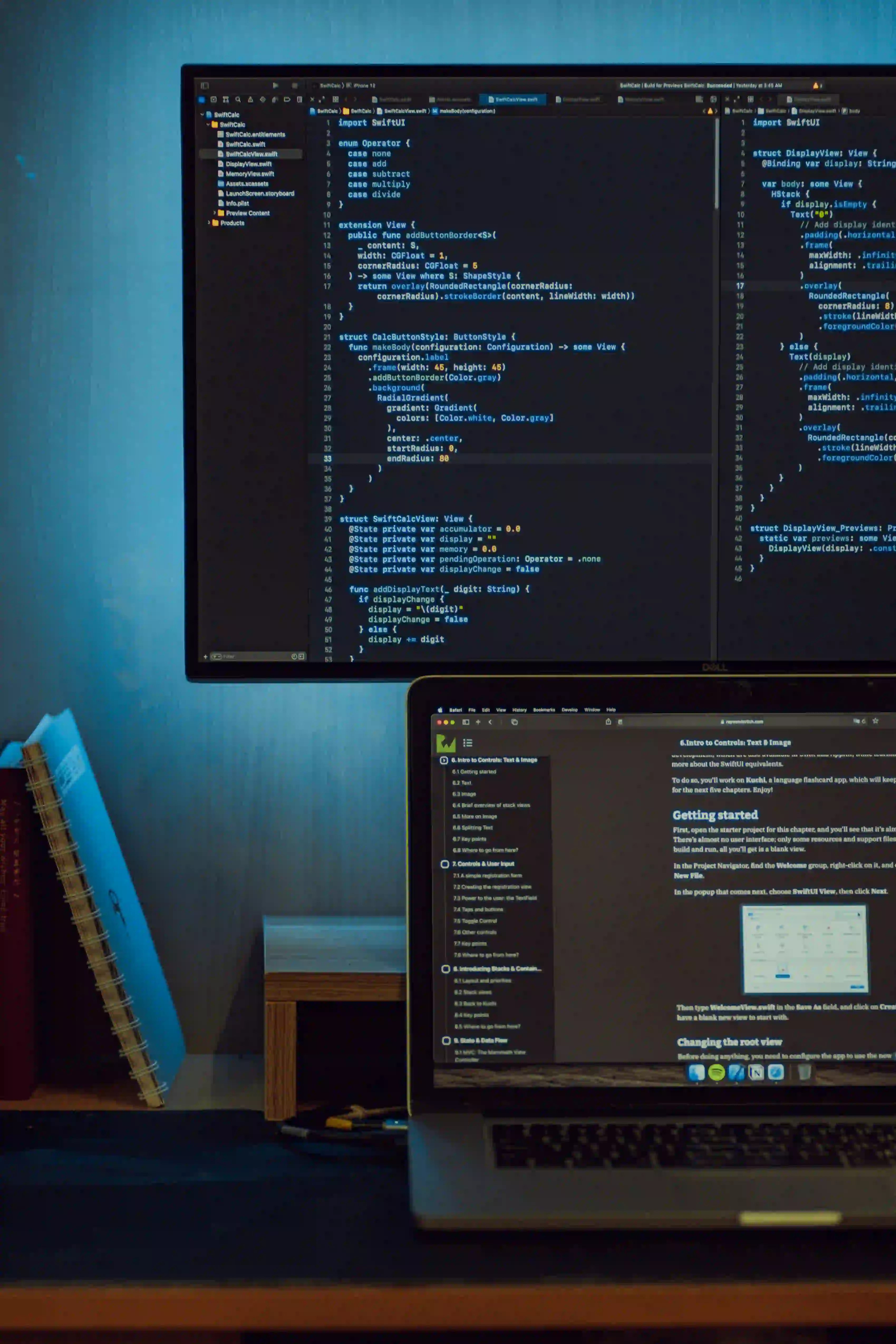
Understanding Lambda Functions and Final Variables in Java
Lambda functions, introduced in Java 8, have transformed the programming landscape by enabling functional programming capabilities. This paradigm shift allows developers to write cleaner and more efficient code. In this blog post, we will delve into lambda functions, exploring their syntax, benefits, and how they interact with final variables in Java. We will also provide code snippets for better understanding.
What is a Lambda Function?
A lambda function is an anonymous function — a function without a name. It can be used primarily to implement functional interfaces (interfaces with a single abstract method). Also, lambda functions can represent a block of code that can be passed around; they increase the expressiveness of the code and reduce the boilerplate.
Syntax of Lambda Expressions
The syntax of a lambda expression consists of three parts:
- Parameters enclosed in parentheses.
- The arrow operator,
->
. - A body that represents the implementation.
The general syntax is:
(parameters) -> expression
// or
(parameters) -> { statements; }
Example of a Simple Lambda Expression
Let's create a simple example to illustrate a lambda expression. Here, we define a functional interface and then implement it using a lambda expression.
@FunctionalInterface
interface Greeting {
void sayHello(String name);
}
public class LambdaExample {
public static void main(String[] args) {
Greeting greeting = (name) -> System.out.println("Hello, " + name);
greeting.sayHello("World");
}
}
Why Use Lambda Functions?
- Readability: The code becomes cleaner and more readable.
- Reduced Boilerplate: Fewer lines of code are required, as we don't need separate classes for single-method interfaces.
- Ease of Use: They make it easier to pass behavior as parameters, enhancing code modularity.
Final Variables in Java
In Java, final variables are variables whose value cannot be changed once assigned. This characteristic makes them particularly useful for ensuring that particular values remain constant throughout an application, thus enhancing reliability. A final variable can be declared with the final
keyword.
Usage of Final Variables
Let’s illustrate final variables with an example:
public class FinalVariableExample {
private static final int MAX_ATTEMPTS = 5;
public void performTask() {
// The MAX_ATTEMPTS variable cannot be changed
for (int i = 0; i < MAX_ATTEMPTS; i++) {
System.out.println("Attempt " + (i + 1));
}
}
}
Why Use Final Variables?
- Immutability: Once set, the value cannot be changed, ensuring stability.
- Easy to Read: They are easier to read and maintain since their purpose is clear.
- Thread Safety: Helps in creating thread-safe classes by preventing accidental changes to the variable value.
Lambda Expressions With Final Variables
One interesting aspect of lambda expressions in Java is their interaction with final or effectively final variables.
Effectively Final Variables
In Java, a variable is considered “effectively final” if it is not modified after its initialization. Even if you don’t declare the variable as final, as long as you don’t change its value, it can be accessed in lambda expressions.
Let’s see an illustration of this.
public class LambdaWithFinal {
public static void main(String[] args) {
final String greetingPrefix = "Hello"; // Explicitly final
// Effectively final variable
String name = "World"; // Not declared final but not modified
Greeting greeting = (n) -> System.out.println(greetingPrefix + ", " + n);
greeting.sayHello(name);
}
}
In this example, greetingPrefix
is explicitly declared as final. The name
variable is not but acts as effectively final since its value does not change. Both variables can be utilized within the lambda expression.
Why Can Only Final or Effectively Final Variables be Used?
The design decision behind this behavior is mainly related to variable scope and thread safety. Since lambda expressions can exist beyond the scope of their enclosing method, allowing mutable variables could lead to unpredictable results and concurrency issues. By restricting access to final or effectively final variables, Java ensures that the code remains predictable and safer.
Benefits of Using Lambda Functions with Final Variables
- Clarity and Safety: Code becomes clear regarding which variables maintain their value throughout execution.
- Simplification: It reduces complexity by lowering the number of classes needed to handle function behavior.
- Enhanced Performance: Functional-style programming is generally faster due to optimizations in how lambdas are executed.
Real-World Use Cases
1. Event Handling in GUI Applications
In GUI applications, lambda expressions simplify event handling. For instance, if you have a button that requires a click event action, implementing that action as a lambda reduces boilerplate.
button.addActionListener(e -> System.out.println("Button Clicked"));
2. Filtering and Mapping Collections
Lambda functions streamline operations on collections. For example, Java Streams API uses lambda expressions extensively for filtering, transforming, and aggregating data.
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
List<String> filteredNames = names.stream()
.filter(name -> name.startsWith("A"))
.collect(Collectors.toList());
System.out.println(filteredNames);
3. Custom Sort Using Lambda
You can also use lambda functions to customize sorting behavior without creating separate classes.
List<String> fruits = Arrays.asList("Banana", "Apple", "Orange");
Collections.sort(fruits, (a, b) -> a.compareTo(b));
System.out.println(fruits);
Closing the Chapter
Understanding lambda functions and final variables in Java opens up new avenues for code simplification and readability. They promote cleaner code and are essential for anyone aiming to write modern Java applications. As you harness lambda functions, remember their relationship with final and effectively final variables to master functional programming in Java.
Additional Resources
- Java Documentation on Lambda Expressions
- Effective Java (3rd Edition) for best practices on Java programming.
If you have any questions or feedback, feel free to leave a comment below! Happy coding!