Is Technical Debt Just an Excuse for Poor Code Quality?
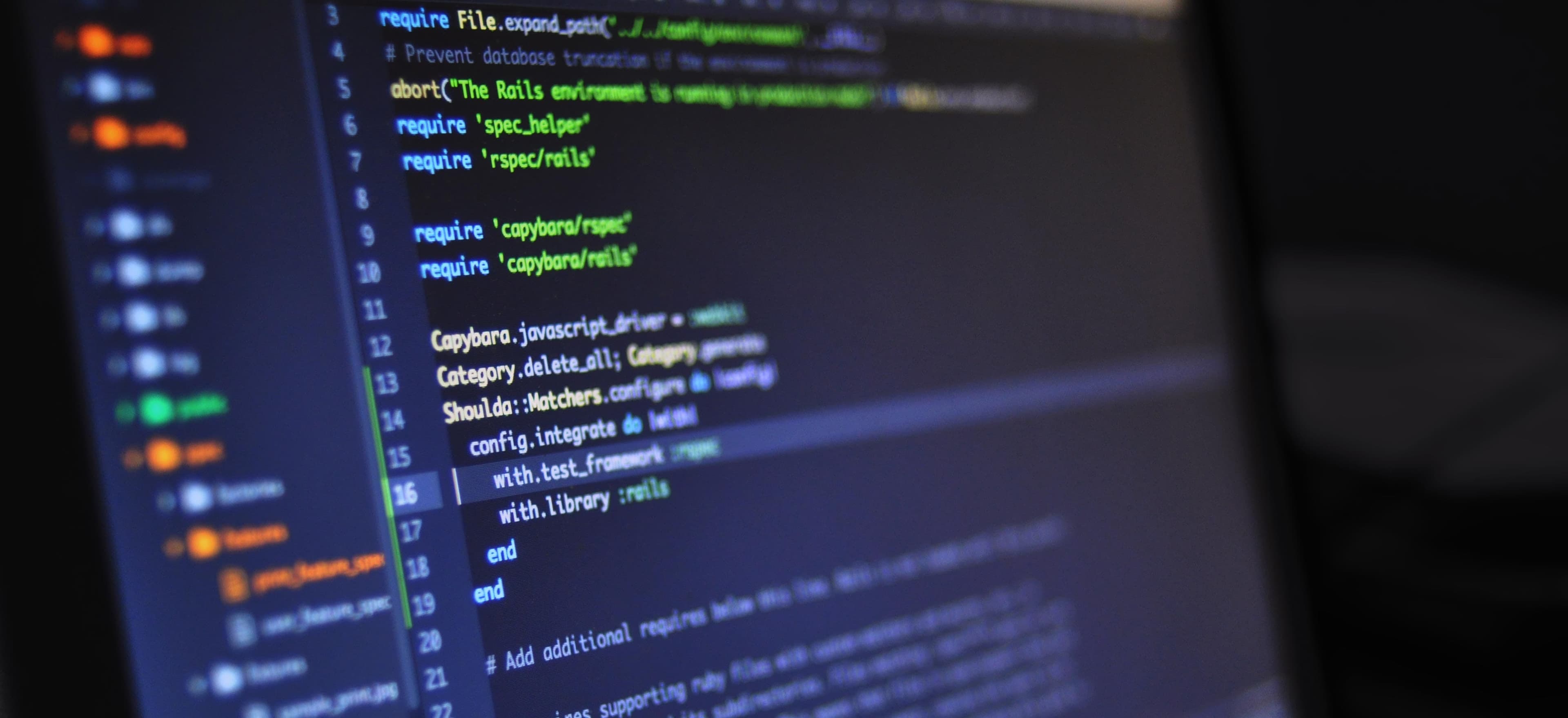
- Published on
Is Technical Debt Just an Excuse for Poor Code Quality?
In the realm of software development, the term "technical debt" is often tossed around, but its implications and meanings can be nebulous. Many developers and project managers use it to justify subpar code quality. But is technical debt merely an excuse for poor coding practices? In this blog post, we will delve into the concept of technical debt, assess its true significance, and provide guidance on managing it effectively.
What is Technical Debt?
Technical debt is a metaphor introduced by Ward Cunningham that conveys the idea of inevitable compromise in software development. Just as financial debt accrues interest, so does technical debt accumulate costs over time. When developers take shortcuts, whether for tighter deadlines or to bypass complex problems, they accumulate technical debt.
Types of Technical Debt
-
Deliberate Debt: This occurs with a conscious decision to prioritize rapid delivery over perfect implementation. While this can be valid in certain scenarios, it requires acknowledging the future work needed to pay it back.
-
Inadvertent Debt: Often unnoticed, this type of debt accumulates due to evolving technology or poorly understood requirements. Teams may find themselves stuck with legacy code that no longer serves their needs.
-
Bit Rot: This happens when code becomes outdated due to lack of updates or changes in external libraries and tools, making maintenance increasingly difficult.
-
Design Debt: Poor design choices early in a project that require future refactoring or enhancement.
Is It Really a Justification for Poor Code Quality?
The Argument for Technical Debt
Technical debt can be a legitimate strategy. It allows teams to deliver value quickly or release an initial version of a product that can be iterated upon. It can also enable agility in a fast-paced environment.
public class FastTrackDevelopment {
public String getGreeting(String name) {
return "Hello, " + name; // Quick and simple implementation
}
}
In the code snippet above, while the method is straightforward, it's missing important features such as input validation. However, in a rush to market, this may be acceptable initially.
The Argument Against Technical Debt
On the flip side, treating technical debt as an excuse for poor code can lead to chaos. It may foster a culture of negligence, where developers bypass best practices, thus disregarding the long-term health of the codebase.
When teams continually ignore technical debt, the codebase can become more complex and challenging to maintain. This complexity may slow down development speed more than the original deadlines would have allowed.
Managing Technical Debt
Acknowledgment
The first step in managing technical debt is acknowledging its presence. Teams should regularly evaluate their code quality and recognize any areas of accumulated debt.
Prioritization
Not all technical debt is equal. Assess which debts have the most significant impacts on the system. For example, a piece of legacy code using outdated frameworks might hold back new developments.
public class LegacySystem {
public void oldMethod() {
// This code is outdated and not following current standards
}
}
It’s often wiser to prioritize making improvements to critical functionalities. Keeping a clean backlog of technical debt can also help track the debt over time and prioritize it accordingly.
Refactoring
Schedule regular refactoring sessions, even if preliminary versions are out there. This could involve:
- Cleaning Code: Refine the existing code without altering its core logic.
- Improving Documentation: Ensure that code is well documented for future developers.
- Automated Testing: Introduce unit tests to ensure that refactored components work as intended.
public class ImprovedGreeting {
public String getGreeting(String name) {
if (name == null || name.isEmpty()) {
throw new IllegalArgumentException("Name cannot be null or empty");
}
return "Hello, " + name;
}
}
The snippet above demonstrates a better practice, providing input validation to avoid runtime errors. Such improvements reduce the technological debt that was inadvertently built over time.
Balance
Achieving an optimal balance between speed and quality is crucial. Speed should not come at the expense of maintainable, scalable, and reliable code.
The Role of Agile Methodologies
Agile practices can assist in managing technical debt. Utilizing frameworks such as Scrum or Kanban facilitates continuous integration and delivery, allowing teams to pay down technical debt incrementally.
Agile retrospectives also provide teams an opportunity to reflect on their technical debt challenges and develop strategies to address them moving forward.
Closing the Chapter
While some use technical debt as a catch-all phrase to sidestep accountability for poor code quality, it should be understood as a multi-faceted concept within software development. Technical debt can be both a strategic mechanism and a cautionary tale, depending on how it’s managed.
The real question becomes one of management: developers must recognize and address technical debt systematically to foster a healthy codebase while balancing the demands of business agility.
For further reading, consider exploring Martin Fowler's article on technical debt and its implications in software development.
By approaching technical debt thoughtfully and proactively, teams can avoid the pitfalls of neglect and lay the groundwork for high-quality software that stands the test of time and needs. Remember, every line of code is an investment; choose wisely!
Checkout our other articles