How Escape Analysis Impacts Long-Take Performance in JVM
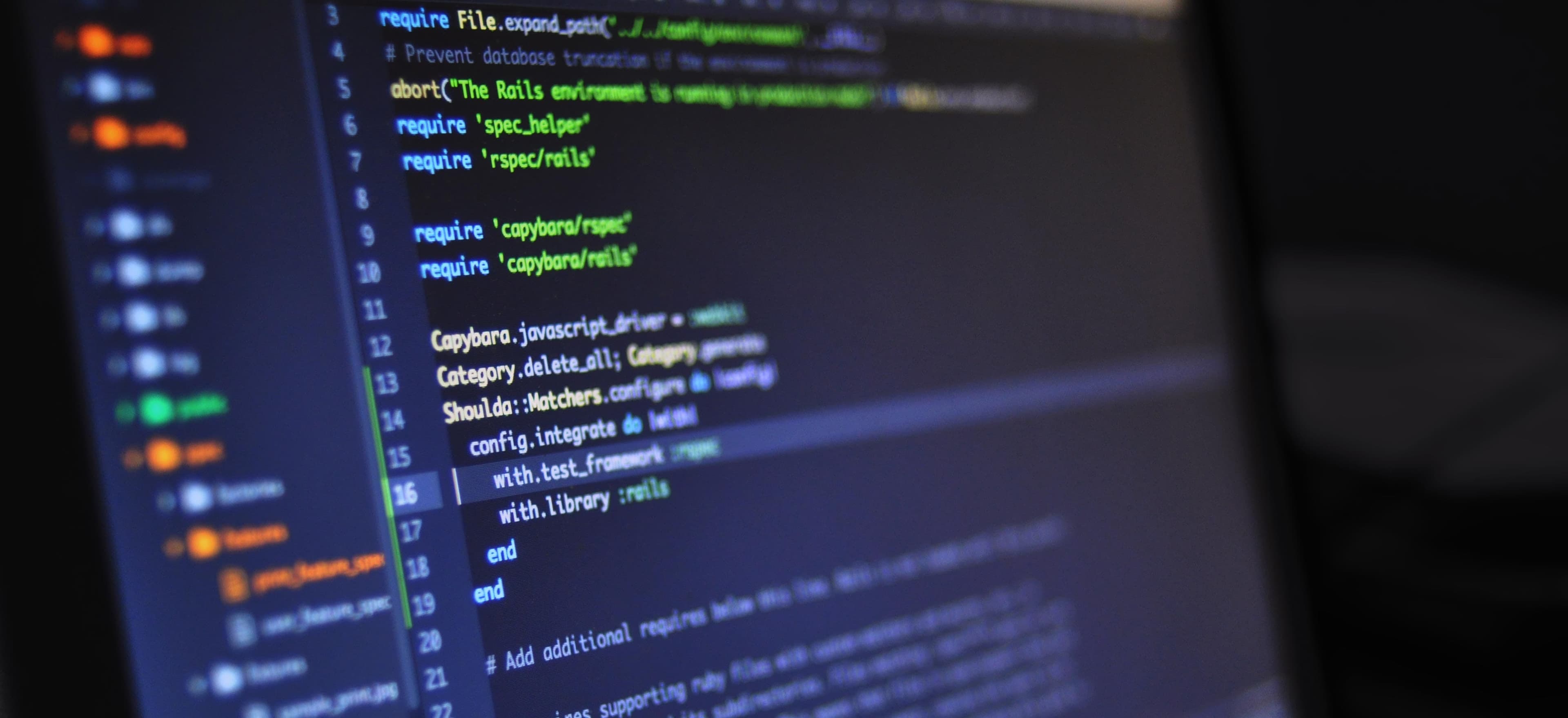
- Published on
How Escape Analysis Impacts Long-Take Performance in JVM
Java Virtual Machine (JVM) is a powerful engine that drives Java applications, enabling them to run on various platforms seamlessly. While the JVM is renowned for its efficiency, underlying mechanisms such as Escape Analysis play a crucial role in enhancing performance, particularly for long-lived tasks. In this blog post, we'll explore what Escape Analysis is, its implications on memory management, and how it significantly impacts the performance of long-running applications.
What is Escape Analysis?
Escape Analysis is a form of static program analysis performed by the JVM. Its primary goal is to determine the scope of object references within a method or thread:
- Inside the Method: The object does not escape the context of its creation.
- Escaping: The object is visible outside the method or thread context.
The findings of this analysis have profound implications for memory allocation and garbage collection.
Why Escape Analysis Matters
Understanding whether an object "escapes" helps the JVM make decisions on how to allocate memory:
- Stack Allocation: If the object does not escape, the JVM can allocate it on the stack, which is faster than heap allocation. Stack memory is automatically reclaimed once the method completes, minimizing overhead.
- Heap Allocation: If the object escapes, it must be allocated on the heap. This requires the overhead of garbage collection (GC) when the object is no longer in use.
Ultimately, effective escape analysis reduces the frequency and duration of garbage collection, enhancing the application's runtime performance.
How Escape Analysis Works in JVM
Escape Analysis consists of several steps:
- Static Analysis: The JVM inspects method calls and object allocations during the compilation phase.
- Path Analysis: It traverses object access paths to determine if any reference escapes its context.
- Decision Making: Based on the analysis, it decides whether to allocate an object on the stack or the heap.
Example of Escape Analysis in Action
Consider the following Java code snippet:
public class EscapeAnalysis {
public void createObject() {
Helper helper = new Helper(); // Does not escape
helper.doSomething();
}
}
class Helper {
void doSomething() {
// Implementation
}
}
In this example, the Helper
object is created within the createObject()
method, and it does not escape this method; hence, the JVM can stack-allocate the helper
instance.
Conversely, if we modify the code to make the object escape:
public class EscapeAnalysis {
private Helper sharedHelper; // Field that can escape
public void setupHelper() {
sharedHelper = new Helper(); // The object escapes
}
}
Here, sharedHelper
is a class field, meaning the Helper
object can outlive the scope of the setupHelper
method. Thus, the JVM must allocate it on the heap.
Detailed Benefits of Escape Analysis for Long-Running Applications
Long-running applications often function continuously for days or even weeks, making efficient memory management paramount. Let's discuss some benefits of Escape Analysis in this context.
1. Reduction in Garbage Collection Pressure
Garbage Collection can be detrimental to the performance of long-running applications.
-
Less Frequent GC Events: Since Escape Analysis allows for stack allocation when an object is confined to a method, less pressure is placed on the heap. Therefore, the frequency of GC events is minimized.
-
Lower Latency: By reducing GC pauses, Escape Analysis contributes to a smoother performance profile for long-running applications.
2. Improved Object Lifetime Control
Objects with a well-defined scope benefit significantly from proper memory allocation.
-
Transient Objects: Many long-running applications utilize temporary objects. With Escape Analysis, such objects can be allocated on the stack, thus automatically reclaimed when they go out of scope.
-
Memory Efficiency: This efficient allocation strategy enables the JVM to manage memory safely and efficiently, yielding higher throughput.
3. Enhanced Throughput
Throughput measures the amount of work done in a given time period, vital for server applications.
-
Faster Method Execution: Allocating objects on the stack means faster memory allocation and deallocation, resulting in faster method execution overall.
-
More Concurrent Threads: Efficient memory use reduces overall system load, allowing the JVM to handle more concurrent threads smoothly.
4. Encouragement for Functional Programming Practices
Escape Analysis aligns well with functional programming practices, where immutability is favored:
- Immutability: Immutable types are less likely to escape, establishing them as ideal candidates for escape analysis. Since these types may exist only in method scope, stack allocation can often be applied.
Limitations of Escape Analysis
While Escape Analysis provides several advantages, it's essential to recognize certain limitations:
- Static Nature: Escape Analysis is a static analysis technique, meaning it cannot handle dynamic scenarios such as polymorphism or complex object interactions.
- Context Sensitivity: Certain patterns can cause the analysis to be overly simplistic, leading to potential performance regressions if not managed correctly.
- Misallocation: The JVM might incorrectly decide on allocation due to limitations in the analysis, leading to performance bottlenecks.
Practical Considerations
To effectively leverage Escape Analysis in your Java applications, consider the following:
- Write Method-Scope Confined Code: Aim to confine object allocations within methods as much as possible.
- Profile Your Applications: Use profiling tools such as VisualVM or YourKit to observe memory allocation and GC activity within your applications.
- Monitor Performance Metrics: Keep an eye on metrics such as response time, throughput, and memory usage to understand how Escape Analysis affects your applications in real-time.
The Last Word
Escape Analysis is a powerful optimization technique used by the JVM with significant implications for long-running applications. By enabling better memory allocation strategies, it enhances performance, reduces garbage collection pressure, and improves throughput. For Java developers aiming to achieve optimal long-term performance, understanding and utilizing Escape Analysis is essential.
If you're interested in how JVM optimizations impact performance further, check out the Java Performance Tuning guide from Oracle.
By taking the time to understand this aspect of the JVM, developers can build more efficient, responsive, and long-lasting applications. Happy coding!
Checkout our other articles