Understanding EJB Inheritance Pitfalls in Java Development
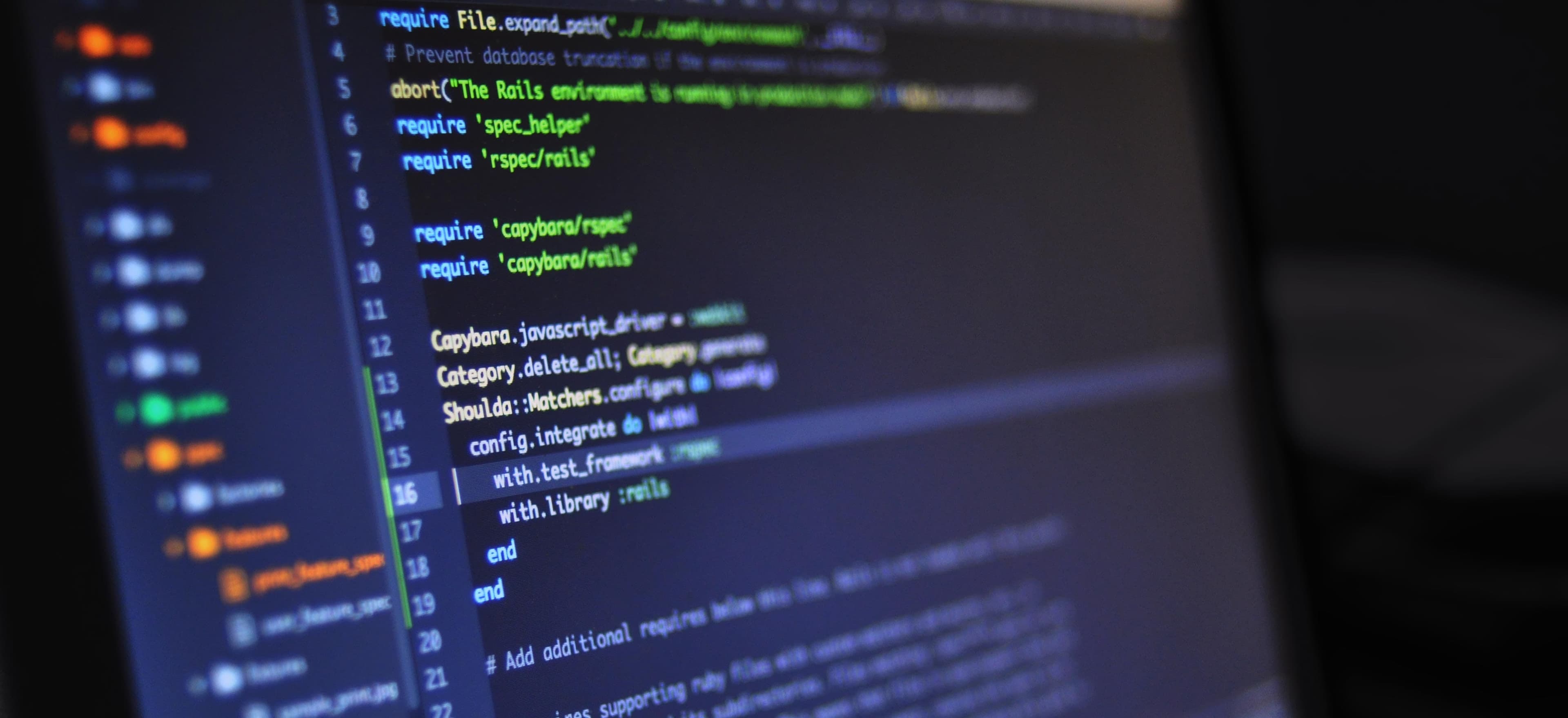
- Published on
Understanding EJB Inheritance Pitfalls in Java Development
Enterprise JavaBeans (EJB) is a powerful framework for building scalable, secure, and transactional enterprise-level applications in Java. While EJB provides many advantages, it is essential to understand the implications of using inheritance within this context. This blog post will explore the pitfalls of EJB inheritance and provide insights on how to avoid common mistakes.
What is EJB?
EJB is part of the Java EE (Jakarta EE) specification that simplifies large-scale enterprise applications. It enables developers to focus on business logic while EJB handles system-level concerns like transaction management, security, and concurrency.
EJB variants:
- Session Beans: Encapsulate business logic. They can be stateless or stateful.
- Message-Driven Beans (MDBs): Asynchronously process messages from a messaging service.
- Entity Beans: Represent persistent data. However, this model is now primarily replaced by the Java Persistence API (JPA).
The Essence of Inheritance in EJB
Using inheritance in EJB aims to promote code reuse and create a well-structured application. However, it comes with its own set of challenges that developers must navigate carefully.
Common EJB Inheritance Pitfalls
-
Complexity of the Class Hierarchy
Inheritance can lead to complex class hierarchies, making the code difficult to understand and maintain. When multiple beans inherit from a parent, tracking the flow of business logic becomes cumbersome. This complexity can defeat the purpose of creating clean, maintainable code.
@Stateless public class BaseService { public void commonMethod() { // Common logic here } } @Stateless public class UserService extends BaseService { public void createUser() { commonMethod(); // Additional logic for creating user } }
Why? While this code snippet shows a straightforward base service, managing multiple extensions can complicate how methods are overridden and can lead to unexpected behavior.
-
EJB Proxy Usage
The EJB container often uses proxies to manage bean instances. When inheritance is involved, it may not be clear how proxying works. If a subclass overrides a method, you may end up invoking the superclass method instead, introducing inconsistencies.
@Stateless public class BaseService { public void performAction() { // some implementation } } @Stateless public class SpecificService extends BaseService { @Override public void performAction() { super.performAction(); // Calls base implementation // additional specifics } }
Why? This can confuse developers about what implementation is called at runtime. It’s crucial to understand how your EJB container handles method invocations for subclasses.
-
Configuration Challenges
Inheritance can also complicate your deployment and configuration settings. EJB relies on annotations and XML descriptors for configuration. Subclass configurations may not inherit configurations from superclasses as expected.
<session> <ejb-name>UserService</ejb-name> <local>com.example.service.UserServiceLocal</local> </session> <!-- No automatic inheritance; each subclass requires separate entries -->
Why? This leads to the risk of inconsistently configured beans, further increasing maintenance workload.
-
Impact on Persistence Management
When using JPA alongside EJB, inheritance can create issues in entity relationships. JPA uses different strategies (e.g., TABLE_PER_CLASS, SINGLE_TABLE) for entity inheritance, affecting how entities are persisted and retrieved.
@Entity @Inheritance(strategy = InheritanceType.SINGLE_TABLE) public abstract class BaseEntity { @Id private Long id; // common fields } @Entity public class UserEntity extends BaseEntity { private String username; }
Why? Using the wrong inheritance strategy can lead to performance issues and complicated transformations, particularly when dealing with large datasets.
Best Practices to Avoid Inheritance Pitfalls
Understanding the pitfalls is only the first step; knowing how to avoid them makes you a better developer. Here are some strategies:
-
Prefer Composition Over Inheritance
Instead of inheriting from a base EJB class, consider using composition. This often leads to cleaner, more maintainable code.
@Stateless public class UserService { private final CommonService commonService; @Inject public UserService(CommonService commonService) { this.commonService = commonService; } public void createUser() { commonService.commonMethod(); // Create user logic } }
Why? This design pattern promotes loose coupling, leading to greater flexibility and testability.
-
Use Interfaces for Contracts
Use interfaces to define contracts rather than implementing inheritance. This promotes better design by adhering to programming principles like Favor Composition over Inheritance.
public interface UserServiceLocal { void createUser(); } @Stateless public class UserService implements UserServiceLocal { public void createUser() { // logic here } }
Why? Interfaces help to decouple your code and facilitate easier testing and maintenance.
-
Explicit Configuration
When using inheritance, ensure that every class is explicitly configured. Avoid relying solely on inherited configurations to specify how the EJB should behave.
Why? This eliminates confusion about which entity is configured and guarantees that any changes made are well understood.
-
Test Thoroughly
Ensure that your EJB beans, especially those using inheritance, are thoroughly tested. This helps identify any unexpected behavior early on.
@RunWith(MockitoJUnitRunner.class) public class UserServiceTest { @InjectMocks private UserService userService; // Mock the dependencies and write unit tests }
Why? Comprehensive testing can highlight problems in the class hierarchy or configuration, preventing runtime issues.
In Conclusion
While EJB inheritance can enhance code organization through reuse, it can also create pitfalls that impede development. By understanding these challenges and implementing best practices such as favoring composition over inheritance and maintaining explicit configurations, you'll increase code maintainability and clarity.
If you'd like to dive deeper into Java EE development principles and best practices, refer to the official documentation. Happy coding!
Additional Reading
Understanding these principles will not only make you a better programmer but will also lead to the development of robust enterprise applications.
Checkout our other articles