Overcoming Java's Steep Learning Curve for Beginners
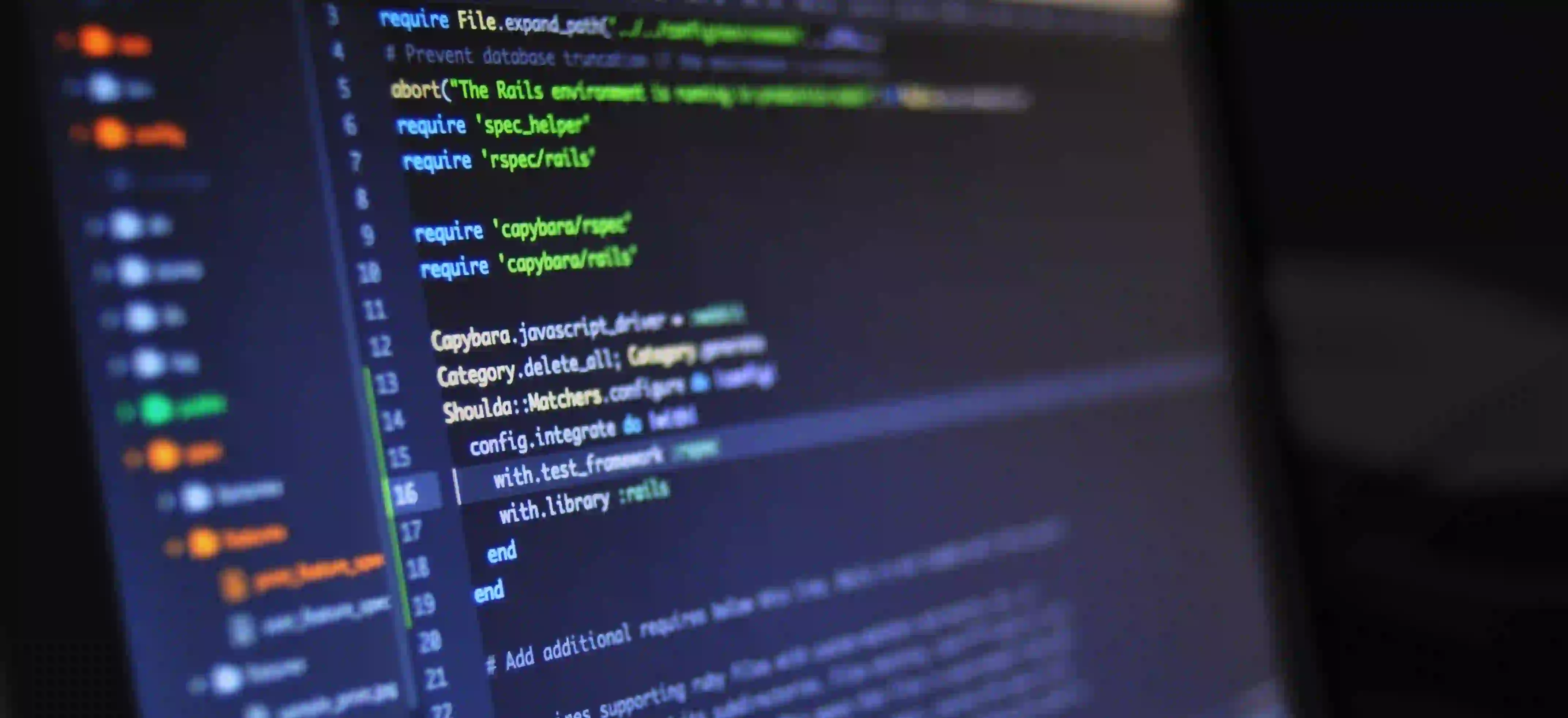
Overcoming Java's Steep Learning Curve for Beginners
Java is one of the most widely-used programming languages in the world. Its versatility enables developers to build everything from mobile applications to large-scale enterprise systems. However, beginners often feel overwhelmed by its complexities and rich ecosystems. In this blog post, we will explore effective strategies to overcome Java's steep learning curve and enhance your coding journey.
Why Learn Java?
Before we dive into the strategies, it is crucial to understand why Java is a valuable skill to acquire:
- Platform Independence: Java's "Write Once, Run Anywhere" capability allows applications to run on any device with a Java Runtime Environment (JRE).
- Strong Community Support: The vast community provides a wealth of resources including forums, libraries, and frameworks.
- Rich Ecosystem: With frameworks like Spring, Hibernate, and JUnit, Java developers can create robust applications efficiently.
By recognizing these advantages, you can appreciate the value of investing time into learning Java.
Strategy 1: Build a Strong Foundation
Start with the Basics
Java has core principles that every programmer should master. Begin your journey by understanding:
- Syntax: Learn about variables, data types, operators, and control structures.
- Object-Oriented Programming (OOP): Java is fundamentally OOP-focused. Grasp concepts like classes, objects, inheritance, polymorphism, encapsulation, and abstraction.
To illustrate the principles of classes and objects, consider the following code snippet:
class Car {
// Attributes
String color;
String model;
// Constructor
Car(String color, String model) {
this.color = color;
this.model = model;
}
// Method
void drive() {
System.out.println("The " + color + " " + model + " is driving.");
}
}
// Main Method
public class Main {
public static void main(String[] args) {
// Creating an object
Car myCar = new Car("red", "Toyota");
myCar.drive();
}
}
Why is this important? Understanding classes and how to create objects will help you design more complex systems in the future.
Resource Recommendation
Courses like Java for Beginners by Coursera or Codecademy's Learn Java provide structured learning paths that prioritize fundamental concepts.
Strategy 2: Practice, Practice, Practice
Code Every Day
Engagement and consistency are key when it comes to learning Java. Dedicate time each day to writing Java code. Make it a habit! Start solving simple problems like:
- Fibonacci Series
- String Reversal
- Factorial Calculation
Below is a simple implementation of the Fibonacci series:
public class Fibonacci {
public static void main(String[] args) {
int n = 10, firstTerm = 0, secondTerm = 1;
System.out.println("Fibonacci Series till " + n + ":");
for (int i = 1; i <= n; ++i) {
System.out.print(firstTerm + ", ");
// Compute the next term
int nextTerm = firstTerm + secondTerm;
firstTerm = secondTerm;
secondTerm = nextTerm;
}
}
}
Why is this important? Practicing basic algorithms sharpens your problem-solving skills and helps cement your understanding of programming concepts.
Engage with Coding Challenges
Websites like LeetCode or HackerRank offer challenges that can propel your learning.
Strategy 3: Embrace Debugging
Understanding and Fixing Errors
Instead of fearing errors, embrace them as learning opportunities. Java provides comprehensive error messages and debugging tools.
Consider the following code that contains a deliberate error:
public class DebugExample {
public static void main(String[] args) {
int a = 10;
int b = 0;
// Deliberate error
int result = a / b; // Division by zero
System.out.println("Result: " + result);
}
}
When you run this code, an ArithmeticException
will be thrown.
Why is this important? Understanding errors teaches you not only how to program but also how to think critically. Debugging improves your coding vivacity by pushing you to diagnose problems.
Strategy 4: Work on Projects
Real-World Application
Working on personal projects is one of the best ways to solidify your learning. Construct applications that solve real-world problems. Here are some project ideas:
- Task Manager: A simple application to track daily tasks.
- Library Management System: Administrate book lending and returning.
- Weather App: Fetch data from weather APIs and display forecast information.
For a weather application, here's a skeletal structure:
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.Scanner;
public class WeatherApp {
public static void main(String[] args) {
// API endpoint for fetching weather data
String apiKey = "YOUR_API_KEY";
String city = "London";
String weatherEndpoint = "http://api.openweathermap.org/data/2.5/weather?q=" + city + "&appid=" + apiKey;
try {
URL url = new URL(weatherEndpoint);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
// Check response code
if (conn.getResponseCode() == 200) {
Scanner sc = new Scanner(url.openStream());
while (sc.hasNext()) {
System.out.println(sc.nextLine()); // Print the raw JSON data
}
sc.close();
} else {
System.out.println("Error: " + conn.getResponseCode());
}
} catch(Exception e) {
e.printStackTrace();
}
}
}
Why is this important? Projects not only improve your skills, but they also provide you with something tangible to showcase when entering the job market.
Strategy 5: Join a Community
The Importance of Networking
Connecting with other Java learners and seasoned developers can provide you with insights and support that self-study cannot.
- Join forums like Stack Overflow or Java subreddit.
- Participate in local coding meetups.
Why is this important? Being active within a community helps you stay motivated and makes learning a collective experience.
Final Thoughts
Overcoming the steep learning curve of Java requires dedication, practice, and the right set of resources. Start with the fundamentals, develop good coding habits, embrace debugging, work on practical projects, and connect with others.
With time, you will not only understand Java but also become adept at using it to create powerful applications. The journey may be long, but the destination promises rewarding opportunities.
Keep coding, keep learning!