Mastering Strategy Pattern: Avoiding Common Pitfalls
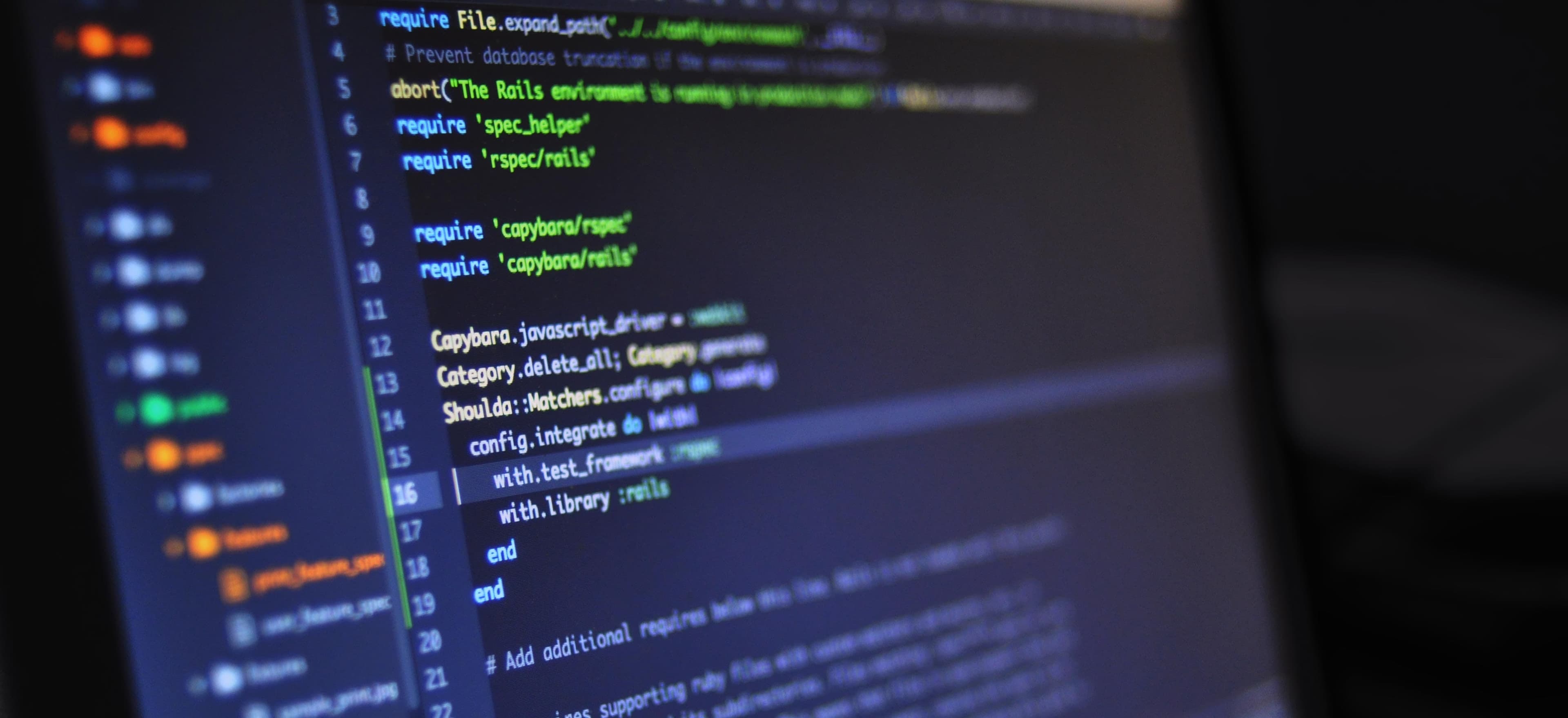
- Published on
Mastering Strategy Pattern: Avoiding Common Pitfalls
The Strategy Pattern is one of the most powerful design patterns in object-oriented programming. It's widely used in Java to define a family of algorithms, encapsulate each one of them, and make them interchangeable. However, like any design pattern, it comes with its own set of challenges and pitfalls. This blog post will dive deep into the Strategy Pattern, illustrate its use in Java, and highlight some common mistakes and pitfalls to avoid.
Understanding the Strategy Pattern
At its core, the Strategy Pattern enables a class to change its behavior based on the strategy that is selected at runtime. This is very beneficial when you want to avoid being bound to a specific implementation of an algorithm. Instead, you can vary the implementation as necessary without altering the clients that use it.
Key Components of Strategy Pattern
- Context: This is the class that will use the strategy.
- Strategy Interface: This interface will declare the methods that all concrete strategies should implement.
- Concrete Strategy Classes: These classes implement the Strategy interface with specific algorithms.
When to Use Strategy Pattern
Use the Strategy Pattern when:
- You have multiple algorithms for a specific task but want to encapsulate them.
- You want to use these algorithms interchangeably.
- You want to eliminate conditional statements that decide which algorithm to use.
Implementing the Strategy Pattern in Java
Here’s a basic example that illustrates how to implement the Strategy Pattern in Java.
Step 1: Define the Strategy Interface
public interface PaymentStrategy {
void pay(int amount);
}
The PaymentStrategy
interface declares a method pay(int amount)
that all concrete strategies will need to implement.
Step 2: Implement Concrete Strategies
public class CreditCardPayment implements PaymentStrategy {
@Override
public void pay(int amount) {
System.out.println("Paid " + amount + " using Credit Card.");
}
}
public class PayPalPayment implements PaymentStrategy {
@Override
public void pay(int amount) {
System.out.println("Paid " + amount + " using PayPal.");
}
}
public class BitcoinPayment implements PaymentStrategy {
@Override
public void pay(int amount) {
System.out.println("Paid " + amount + " using Bitcoin.");
}
}
In the above snippets, we have three different classes (CreditCardPayment
, PayPalPayment
, and BitcoinPayment
) implementing the PaymentStrategy
. Each provides its own version of the pay
method.
Step 3: Create the Context Class
public class ShoppingCart {
private PaymentStrategy paymentStrategy;
public void setPaymentStrategy(PaymentStrategy paymentStrategy) {
this.paymentStrategy = paymentStrategy;
}
public void checkout(int amount) {
if (paymentStrategy == null) {
throw new IllegalStateException("Payment strategy not set.");
}
paymentStrategy.pay(amount);
}
}
The ShoppingCart
class serves as the context. It holds a reference to a PaymentStrategy
and defines a method checkout
that processes the payment.
Step 4: Using the Strategy Pattern
public class Main {
public static void main(String[] args) {
ShoppingCart cart = new ShoppingCart();
cart.setPaymentStrategy(new CreditCardPayment());
cart.checkout(500);
cart.setPaymentStrategy(new PayPalPayment());
cart.checkout(600);
cart.setPaymentStrategy(new BitcoinPayment());
cart.checkout(700);
}
}
In the Main
class, we create a ShoppingCart
instance and dynamically set different payment strategies. The output will demonstrate the flexibility of the Strategy Pattern.
Common Pitfalls to Avoid
While the Strategy Pattern can simplify your code in many scenarios, there are several common pitfalls developers often encounter.
Pitfall 1: Overusing the Pattern
Not every situation requires a Strategy Pattern. If there is only a single algorithm to execute, using the Strategy Pattern may lead to unnecessary complexity. Always assess whether a simpler solution may suffice.
Pitfall 2: Inconsistent Interface Design
When implementing various strategies, ensure that all strategy classes adhere closely to the interface you've defined. If a method's signature or behavior deviates, it can lead to maintenance nightmares and confusion later on.
Pitfall 3: Poor Naming Conventions
Naming strategies intuitively can save developers a lot of time in understanding the purpose of each strategy. Avoid generic names like StrategyA
or StrategyB
. Instead, use descriptive names that reflect the functionality, like CreditCardPayment
.
Pitfall 4: Not Utilizing Encapsulation Properly
Sometimes developers expose strategy classes unnecessarily. Try to encapsulate your strategy logic in the context class as much as possible. This can improve the readability and maintainability of your code.
Pitfall 5: Tight Coupling to Context
Avoid tight coupling between your context and concrete strategy classes. Rely on interfaces instead of hardcoded class types. This makes it easier to swap out different strategies without impacting clients that depend on those strategies.
When Not to Use the Strategy Pattern
The Strategy Pattern may not be suitable when:
- The algorithms are not likely to change; hardcoding may be sufficient.
- You have a limited number of straightforward variants of an algorithm that don't warrant the overhead of creating multiple classes.
For further insights on design patterns in Java, you can check out Refactoring Guru's Design Patterns which provides extensive documentation on various patterns.
Lessons Learned
Mastering the Strategy Pattern involves understanding both its strengths and weaknesses. By avoiding common pitfalls and applying the pattern judiciously, you can create flexible and maintainable code that adapts well to changing requirements.
The Strategy Pattern allows for cleaner code and adheres to the Open/Closed principle, making your system easier to extend without modifying existing code. As you leverage this design pattern in your Java applications, keep in mind the best practices and pitfalls we've discussed.
Ultimately, the goal is to write clean, maintainable, and efficient code that stands the test of time. These insights will give you a robust foundation to successfully implement the Strategy Pattern in your projects. Start experimenting with different strategies and see how best to utilize this powerful design pattern to your advantage!