Common Challenges in Accessing Social Profiles with CloudRail
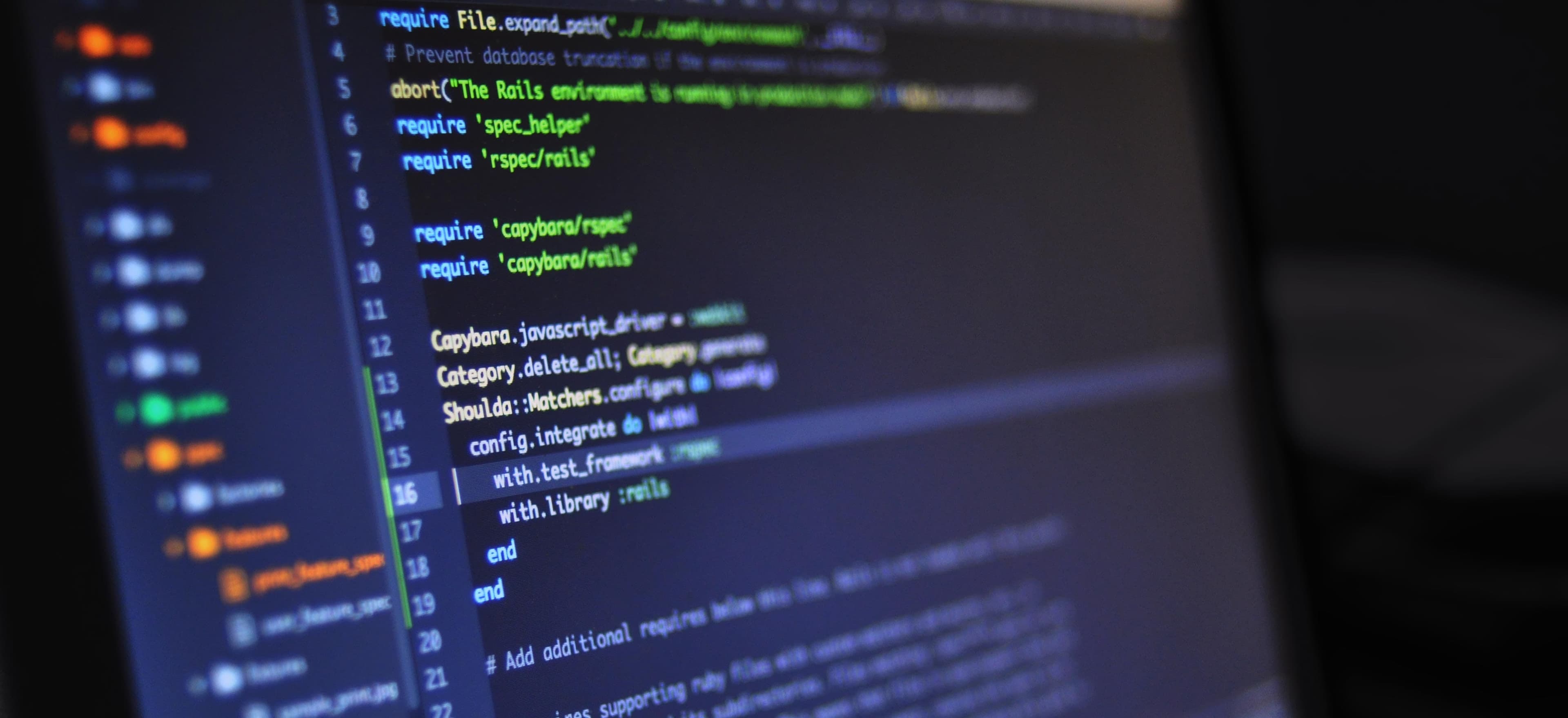
- Published on
Common Challenges in Accessing Social Profiles with CloudRail
In today's interconnected world, social media significantly enhances business and personal communication. Developers often integrate social profile access into their applications to enhance user experiences. CloudRail provides streamlined API access for multiple social networks, encapsulating the complexities into a single interface. However, like any technology, it comes with its own set of challenges. This blog post delves into some common challenges developers face when accessing social profiles with CloudRail while providing tips and code snippets to help you overcome these hurdles.
Understanding CloudRail
Before we dive into the challenges, let’s briefly review what CloudRail is. CloudRail is an abstraction layer that simplifies interactions with various APIs such as social networks, cloud storage services, and more. Here's what makes CloudRail appealing:
- Unified API: One API for multiple services, reducing the learning curve.
- Ease of Use: Simplifies OAuth authentication.
- Cross-platform compatibility: Works across various programming languages.
However, inheriting these benefits isn't devoid of challenges. Let’s examine some of the most common ones:
1. Authentication Issues
The Challenge
Authentication is often the first hurdle developers meet when integrating any social media API. With OAuth 2.0 being the standard for social login, the process can become cumbersome if not handled correctly.
Solution
CloudRail abstracts the authentication process, but you must ensure that your application is correctly set up on the social platform’s developer console.
Code Example
import com.cloudrail.si.interfaces.ISocialProfile;
import com.cloudrail.si.services.Facebook;
public class SocialAuthExample {
public void authenticate() {
Facebook facebook = new Facebook("YOUR_APP_ID", "YOUR_APP_SECRET");
// This will prompt the user to authenticate
String authUrl = facebook.getAuthorizationUrl();
System.out.println("Authenticate using this URL: " + authUrl);
}
}
Why It Matters: The user must authenticate correctly for the app to gain any access. If either the app ID or secret is incorrect, the authentication process will fail.
2. Rate Limiting
The Challenge
Social media APIs have rate limits to prevent abuse. This means your application may receive errors if it makes too many requests in a short period.
Solution
Implement an exponential backoff strategy to handle rate limits. This involves incrementally increasing the wait time between retries when making requests.
Code Example
public void fetchUserData(ISocialProfile profile) {
int retries = 0;
while (retries < 5) {
try {
// Attempt to fetch user data
ProfileData data = profile.getUserData();
System.out.println("Data Retrieved: " + data);
break; // Exit loop on success
} catch (RateLimitException e) {
System.err.println("Rate limit reached. Retrying in " + (1 << retries) + " seconds...");
try {
Thread.sleep((1 << retries) * 1000);
} catch (InterruptedException ie) {
Thread.currentThread().interrupt();
}
retries++;
}
}
}
Why It Matters: Avoiding the rate limit means maintaining a seamless user experience without constant disruptions.
3. Data Consistency
The Challenge
Accessing social profiles often involves cross-referencing data with multiple sources. Data inconsistency can arise due to various factors like user settings or API changes.
Solution
Implement checks to ensure data integrity and provide fallbacks to handle inconsistent data.
Code Example
public void handleUserData(ISocialProfile profile) {
User data = profile.getUserData();
if (data == null || !data.isValid()) {
System.out.println("User data is inconsistent, falling back...");
fallbackToDefaultUserData();
} else {
System.out.println("User Data: " + data);
}
}
private void fallbackToDefaultUserData() {
// Retrieve and display default user data
System.out.println("Loading default data...");
}
Why It Matters: Ensuring consistent data improves application reliability and user trust.
4. Limited API Features
The Challenge
CloudRail simplifies API requests, but some advanced features may not be fully supported. Developers may need to flatten the API, losing granular control.
Solution
Use CloudRail for basic integrations and access the underlying APIs directly for complex functionalities.
Code Example
public void customFeature(ISocialProfile profile) {
// Utilizing CloudRail for basic data
ProfileData data = profile.getUserData();
// If more features are required, make a raw API call
HttpResponse response = makeAdvancedApiCall(data.getId());
if (response.isSuccessful()) {
// Process response
processAdvancedFeatures(response);
} else {
System.err.println("Failed to fetch advanced features.");
}
}
Why It Matters: Knowing how to work with the raw API gives you the flexibility needed for special cases.
5. Handling User Permissions
The Challenge
Different social platforms require different permissions for user data access. Users may revoke permissions at any time, impacting your app’s functionality.
Solution
Request only the permissions you need and regularly check for permission status.
Code Example
public void checkUserPermissions(ISocialProfile profile) {
PermissionStatus status = profile.getPermissionsStatus();
if (!status.isGranted()) {
System.out.println("Requesting permissions...");
profile.requestPermissions("email, profile");
} else {
System.out.println("Permissions are granted.");
}
}
Why It Matters: Adhering to the principle of least privilege enhances user trust and complies with privacy regulations.
The Bottom Line
Accessing social profiles through CloudRail can greatly enhance connectivity within your applications. However, developers must be vigilant about authentication, rate limits, data consistency, API feature limitations, and user permissions. Addressing these challenges proactively ensures a smooth integration process and an excellent user experience.
By implementing the provided strategies and code examples, you can navigate the complexities of social profile access more effectively. For a deeper dive into the intricacies of connecting with social media APIs, you can refer to the following resources:
- OAuth 2.0 Simplified
- CloudRail Documentation
If you have further questions or need help troubleshooting specific issues, please feel free to drop a comment below!
Checkout our other articles