Overcoming WebSocket Connection Issues in AngularJS and Java EE
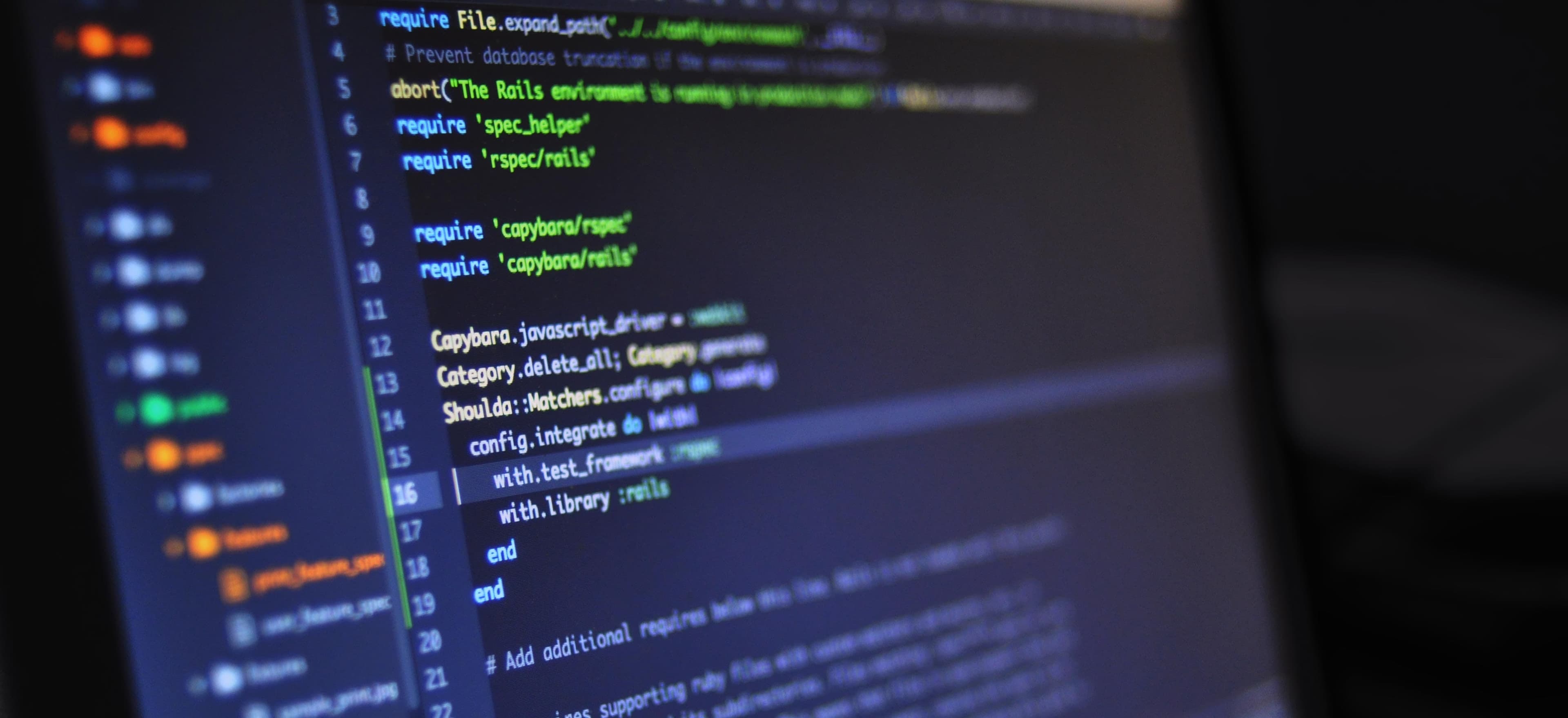
- Published on
Overcoming WebSocket Connection Issues in AngularJS and Java EE
WebSocket is a powerful technology that enables real-time communication between a client and a server. When combined with AngularJS on the front end and Java EE on the back end, it offers the potential for dynamic, interactive web applications. However, developers often encounter connection issues that can undermine the user experience. This post aims to discuss common WebSocket connection issues in AngularJS and Java EE and how to troubleshoot and resolve them effectively.
What are WebSockets?
WebSockets provide a full-duplex communication channel over a single TCP connection. Unlike HTTP, which is request-response-based, WebSockets allow for continuous, real-time data exchange. This makes them ideal for applications that require live updates, like chat applications or stock price monitors.
Setting Up Your AngularJS Application
Basic AngularJS WebSocket Client
Before diving into troubleshooting connection issues, let’s establish a basic WebSocket client in AngularJS.
angular.module('app', [])
.controller('MainController', ['$scope', '$window', function($scope, $window) {
var socket = new WebSocket('ws://localhost:8080/my-websocket');
socket.onopen = function() {
console.log('WebSocket connection established');
// Example of sending a message to the server
socket.send(JSON.stringify({ message: 'Hello, Server!' }));
};
socket.onmessage = function(event) {
var message = JSON.parse(event.data);
console.log('Received message from server:', message);
};
socket.onclose = function() {
console.log('WebSocket connection closed');
};
socket.onerror = function(error) {
console.error('WebSocket error observed:', error);
alert('An error occurred with the WebSocket connection. Please refresh the page.');
};
}]);
Explanation of the Code
-
WebSocket Connection: The WebSocket is initialized with the server URL (
ws://localhost:8080/my-websocket
). -
Event Handlers:
onopen
: Logs a message to the console once the connection is established. It also sends a greeting message to the server.onmessage
: Receives messages from the server and logs them.onclose
: Notifies the client when the connection is closed.onerror
: Handles any errors that may occur with the WebSocket connection.
This is a basic setup to verify the client-side WebSocket implementation.
Setting Up Your Java EE WebSocket Server
Next, you need a simple Java EE WebSocket server to communicate with the AngularJS client.
Java EE WebSocket Server Example
import javax.websocket.OnClose;
import javax.websocket.OnMessage;
import javax.websocket.OnOpen;
import javax.websocket.Session;
import javax.websocket.server.ServerEndpoint;
@ServerEndpoint("/my-websocket")
public class MyWebSocketServer {
@OnOpen
public void onOpen(Session session) {
System.out.println("WebSocket connection established.");
}
@OnMessage
public void onMessage(String message, Session session) {
System.out.println("Received message from client: " + message);
// Echo the received message back to the client
sendMessage(session, "Server received: " + message);
}
@OnClose
public void onClose(Session session) {
System.out.println("WebSocket connection closed.");
}
private void sendMessage(Session session, String message) {
// Sending a message back to client
session.getAsyncRemote().sendText(message);
}
}
Breakdown of the Server Code
-
ServerEndpoint: Defines the server's WebSocket endpoint. The client connects to
ws://localhost:8080/my-websocket
. -
Event Annotations:
@OnOpen
: Logs a message when a client connects.@OnMessage
: Receives a message from a client, logs it, and sends a confirmation back.@OnClose
: Logs when the connection is closed.
-
Message Handling: The
sendMessage
method handles sending messages back to the client asynchronously.
Common WebSocket Connection Issues
1. Unable to Establish a Connection
Symptoms: The onopen
event is never triggered, and the WebSocket connection does not establish.
Possible Causes:
- Incorrect URL: Ensure the URL in the AngularJS WebSocket constructor is correct.
- CORS Issues: If your client and server are on different origins, you may face Cross-Origin Resource Sharing (CORS) issues.
Solution: Verify your URL and ensure your Java EE server is configured to handle CORS.
To allow CORS in your Java EE application, you can add a filter:
import javax.servlet.*;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
public class CORSFilter implements Filter {
public void doFilter(ServletRequest req, ServletResponse res, FilterChain chain) throws IOException, ServletException {
HttpServletResponse response = (HttpServletResponse) res;
response.setHeader("Access-Control-Allow-Origin", "*");
response.setHeader("Access-Control-Allow-Methods", "GET, POST, PUT, DELETE, OPTIONS");
response.setHeader("Access-Control-Allow-Headers", "Content-Type, Authorization");
chain.doFilter(req, res);
}
public void init(FilterConfig filterConfig) {}
public void destroy() {}
}
2. Connection Drops Unexpectedly
Symptoms: The onclose
event is triggered, and you get disconnected without warning.
Possible Causes:
- Network Stability: Unstable network connections can cause WebSockets to drop.
- Timeouts: If the server or client sets a timeout, the connection may get closed.
Solution: Implement a heartbeat mechanism to keep the connection alive. You can send a ping message from the client at regular intervals.
setInterval(function() {
if (socket.readyState === WebSocket.OPEN) {
socket.send(JSON.stringify({ type: 'ping' }));
}
}, 30000); // send a ping every 30 seconds
3. Error Handling
Symptoms: Frequent error events or inability to send/receive messages.
Possible Causes:
- Invalid Message Format: The messages being sent or received do not adhere to JSON or the expected format.
- Server-side Exceptions: An exception in the Java EE server can cause errors that prevent proper handling.
Solution: Always wrap your send logic in try-catch blocks and validate the message formats:
@OnMessage
public void onMessage(String message, Session session) {
try {
// Validate message format
if (isValidMessage(message)) {
sendMessage(session, "Server received: " + message);
} else {
session.getAsyncRemote().sendText("Invalid message format.");
}
} catch (Exception e) {
e.printStackTrace();
}
}
The Bottom Line
WebSocket connections can introduce complexities, but with the right setup and error handling mechanisms, you can ensure smooth and efficient communication between your AngularJS frontend and Java EE backend. Adopting best practices for both error handling and network communication will enhance the user experience and reliability of your applications.
For further reading on WebSockets, consider checking out the following resources:
- WebSocket API Documentation
- Java EE WebSocket Tutorial
With these best practices laid out and examples provided, you are now equipped to tackle any WebSocket connection issues in your AngularJS and Java EE applications. Happy coding!
Checkout our other articles