Mastering Java Decorators: Overcoming Performance Issues
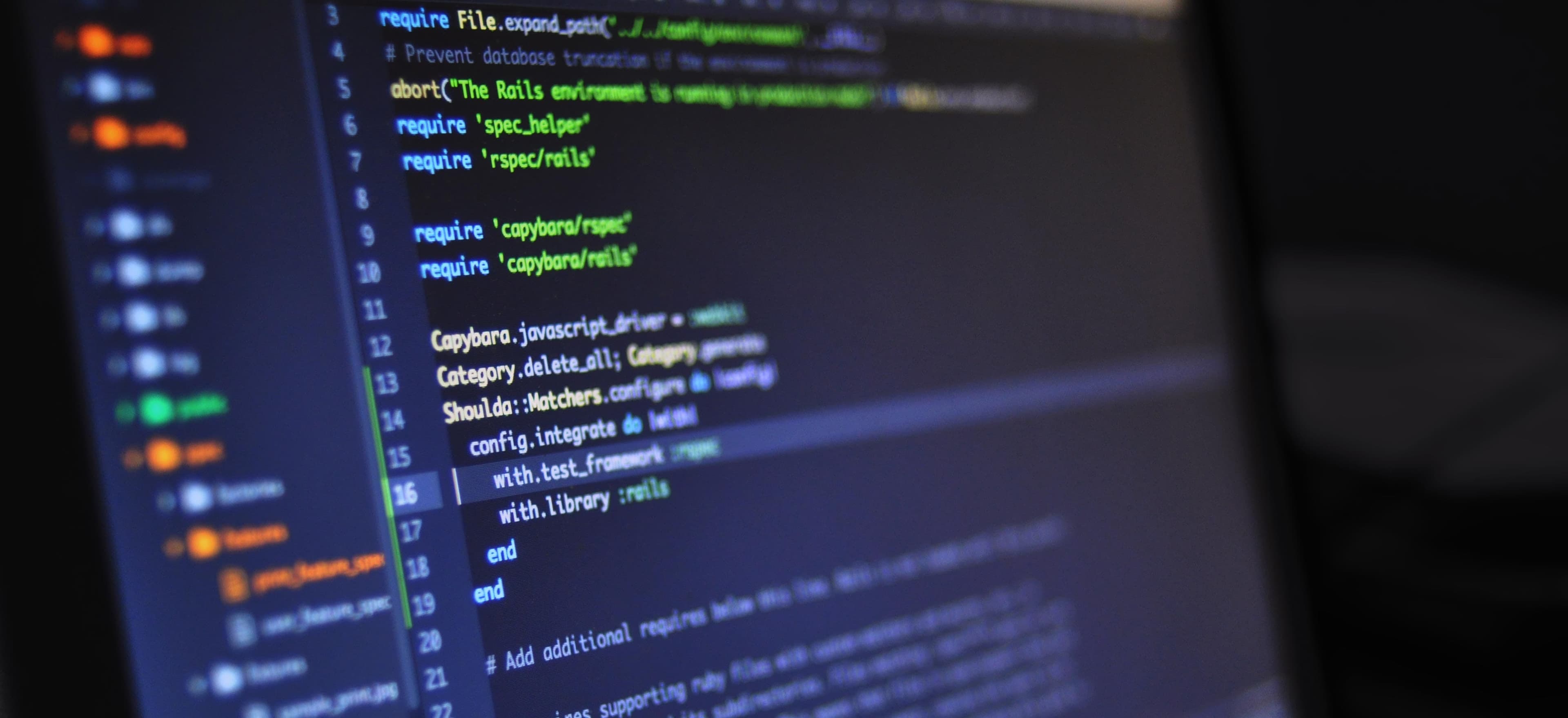
- Published on
Mastering Java Decorators: Overcoming Performance Issues
Java has long been a go-to language for developers due to its versatility and powerful features. One of the design patterns often discussed among developers is the Decorator pattern. It's a sophisticated way to enhance the functionality of classes dynamically. However, while it offers numerous benefits, improper use can lead to performance issues. This blog post will delve into the Java Decorator pattern, its advantages, potential pitfalls, and performance optimization techniques.
Understanding the Decorator Pattern
The Decorator pattern is part of the structural design patterns in software design. It allows behavior and responsibilities to be added to individual objects, either statically or dynamically, without affecting other objects from the same class.
The Components of the Decorator Pattern
- Component: Defines the interface for objects that can have responsibilities added to them.
- Concrete Component: A class that implements the Component interface. It’s a basic instance that can have additional responsibilities added to it.
- Decorator: The abstract class that holds a reference to a Component object and defines the interface that conforms to the Component’s interface.
- Concrete Decorators: Classes that extend the Decorator class and add additional behaviors or responsibilities.
Example of the Decorator Pattern
Let's consider a simple example where we decorate a Coffee
class to add different functionalities.
// Step 1: Component Interface
public interface Coffee {
String getDescription();
double cost();
}
// Step 2: Concrete Component
public class SimpleCoffee implements Coffee {
public String getDescription() {
return "Simple Coffee";
}
public double cost() {
return 2.00;
}
}
// Step 3: Decorator
public abstract class CoffeeDecorator implements Coffee {
protected Coffee decoratedCoffee;
public CoffeeDecorator(Coffee coffee) {
this.decoratedCoffee = coffee;
}
public String getDescription() {
return decoratedCoffee.getDescription();
}
public double cost() {
return decoratedCoffee.cost();
}
}
// Step 4: Concrete Decorators
public class MilkDecorator extends CoffeeDecorator {
public MilkDecorator(Coffee coffee) {
super(coffee);
}
public String getDescription() {
return decoratedCoffee.getDescription() + ", Milk";
}
public double cost() {
return decoratedCoffee.cost() + 0.50;
}
}
public class SugarDecorator extends CoffeeDecorator {
public SugarDecorator(Coffee coffee) {
super(coffee);
}
public String getDescription() {
return decoratedCoffee.getDescription() + ", Sugar";
}
public double cost() {
return decoratedCoffee.cost() + 0.20;
}
}
Why Use the Decorator Pattern?
- Single Responsibility: By using decorators, you can keep the functionality of each component clearly defined.
- Flexibility: You can add behaviors at runtime. For example, you can add both milk and sugar to your coffee without creating multiple subclasses.
- Open/Closed Principle: New functionality can be added without modifying existing code.
Potential Performance Issues
While the Decorator pattern is beneficial, it may introduce performance issues under certain circumstances. Here are some common pitfalls:
- Increased Complexity: Too many layers of decorators can make code difficult to understand and maintain.
- Overuse of Decorators: Applying decorators indiscriminately can lead to performance bottlenecks due to increased method calls and layer overhead.
- Memory Overhead: Each decorator creates additional objects. If used excessively, this increases memory consumption.
Optimizing Performance with Decorators
-
Limit the Depth of Decorators: Try to keep the number of decorators to a minimum. Ensure that each decorator serves a clear purpose.
Instead of stacking multiple decorators, consider combining behaviors into a single decorator where feasible. -
Use Composition Over Inheritance: Favor composition when creating decorators. For instance, if a functionality does not require extending the base class behavior significantly, prefer creating a standalone utility or helper class.
-
Caching Results: If your decorators perform expensive computations, consider caching the results. This avoids duplicated calculations for repeatedly called methods.
Example of Caching
Here's a simple implementation of caching decorators:
import java.util.HashMap;
import java.util.Map;
public class CachedCoffeeDecorator extends CoffeeDecorator {
private static final Map<String, Double> cache = new HashMap<>();
public CachedCoffeeDecorator(Coffee coffee) {
super(coffee);
}
@Override
public double cost() {
String description = decoratedCoffee.getDescription();
if (cache.containsKey(description)) {
return cache.get(description);
}
double cost = decoratedCoffee.cost();
cache.put(description, cost);
return cost;
}
}
Explanation of Caching Example
- Map Cache: We use a HashMap to store computed costs based on the coffee description. This minimizes redundant calculations by leveraging already-computed results.
- Performance: By checking the cache before performing any calculations, we can drastically reduce computational load when the same operations are repeated.
Final Considerations
The Decorator pattern in Java is a powerful tool for dynamically enhancing the behavior of classes. While it provides significant advantages in terms of flexibility and adherence to design principles, it’s essential to remain vigilant regarding potential performance issues. By understanding and applying the optimizations discussed, such as limiting the depth of decorators and leveraging caching, developers can master the use of decorators without sacrificing performance.
For additional reading on design patterns, check out the Introduction to Design Patterns or delve deeper into Java Performance Tuning to understand how to optimize your Java applications further.
Happy coding!
Checkout our other articles