Common Pitfalls in Java Externalization You Should Avoid
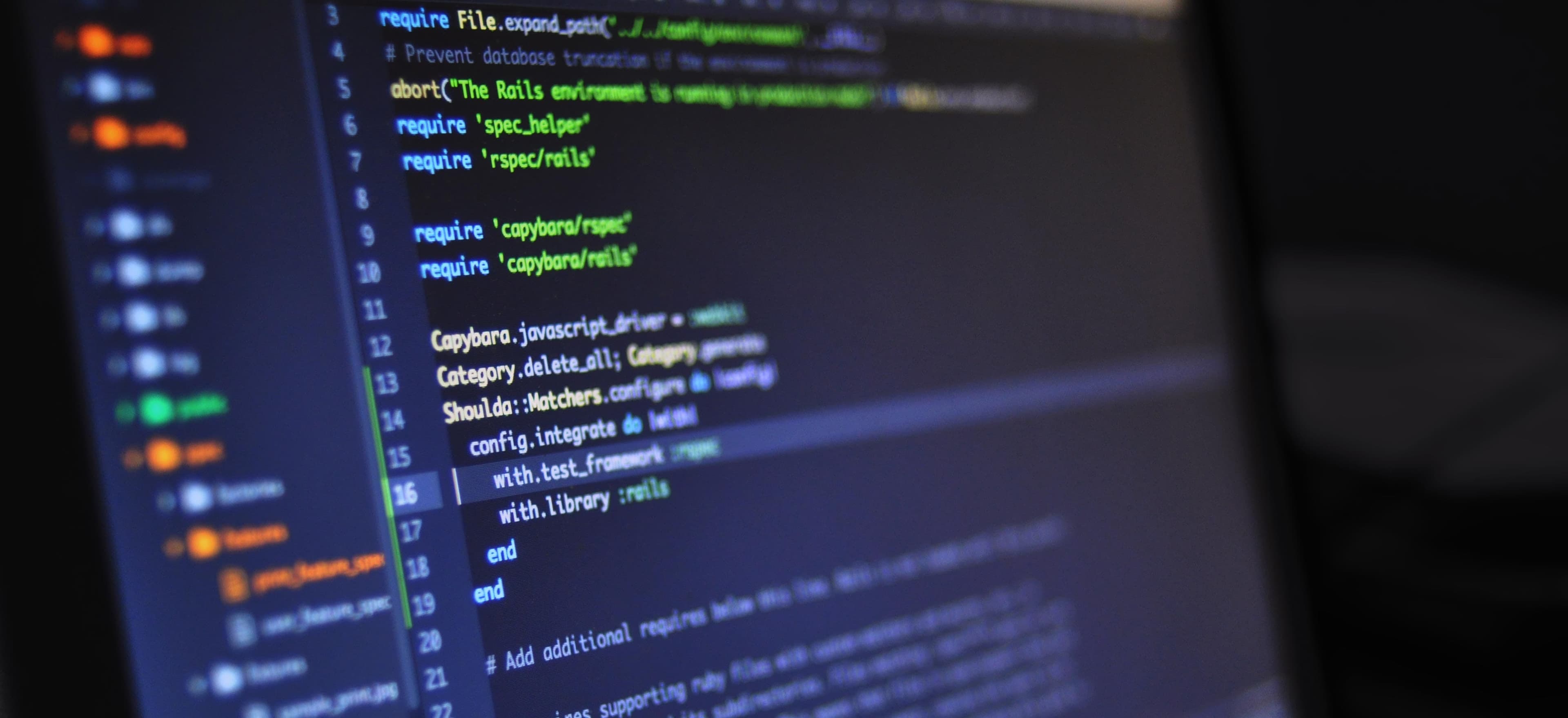
- Published on
Common Pitfalls in Java Externalization You Should Avoid
Java's serialization mechanism is a powerful tool for persisting objects for later recovery. However, when it comes to data serialization, developers often encounter some common pitfalls, particularly with externalization. Understanding these pitfalls can save you from the headache of debugging and ensure that your application runs as intended. Let’s delve into what externalization is, why it matters, and how to steer clear of its common traps.
What is Externalization?
Externalization in Java refers to a mechanism that allows developers to control the serialization process of objects. Unlike Java's built-in serialization, which saves the entire object state by default, externalization provides flexibility for customizing the serialization process. The Externalizable
interface, which extends Serializable
, mandates two methods: writeExternal(ObjectOutput out)
and readExternal(ObjectInput in)
.
Why Use Externalization Over Serialization?
- Control: You can control exactly what gets serialized.
- Efficiency: You can skip some fields or use compressed formats, potentially reducing the stored size.
- Backward Compatibility: You have more control over how data is serialized and deserialized, which can make it easier to handle versioning.
A Basic Example
Here’s a simple implementation of externalization:
import java.io.*;
public class Employee implements Externalizable {
private String name;
private transient int age; // transient means this field won't be serialized
// No-argument constructor is required for Externalizable
public Employee() {
}
public Employee(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public void writeExternal(ObjectOutput out) throws IOException {
out.writeUTF(name);
out.writeInt(age); // You have control over serialization
}
@Override
public void readExternal(ObjectInput in) throws IOException {
name = in.readUTF();
age = in.readInt(); // Corresponds with the write method
}
}
In this code:
- We implement
Externalizable
. - We provide a no-argument constructor, essential for deserialization.
- Methods
writeExternal
andreadExternal
control the serialization and deserialization process.
Common Pitfalls in Java Externalization
1. Forgetting the No-Argument Constructor
One of the most common mistakes is failing to provide a no-argument constructor. The Java ObjectInputStream requires this constructor during deserialization.
Why It Matters: Without it, Java cannot instantiate the object when reading.
Solution:
Always add a no-argument constructor to your Externalizable
classes, as shown in the Employee example.
2. Ignoring the transient
Modifier
In externalization, fields marked as transient
will not be serialized. However, if you do not handle these fields properly in your readExternal
method, you might lose critical data during deserialization.
Why It Matters: Losing a transient field could lead to incomplete or erroneous object states.
Solution:
Be mindful of which fields are transient
. You might need to initialize them to default values if you require the data later.
3. Improper Order of Serialization and Deserialization
When using writeExternal
and readExternal
, the order of writing and reading parameters must match precisely.
Why It Matters: If the order is mismatched, the object will not be reconstructed correctly.
Solution:
Stick to a protocol for field order in serialization, perhaps by documenting the order or creating a constant array to represent this.
Example:
@Override
public void writeExternal(ObjectOutput out) throws IOException {
out.writeUTF(name);
out.writeInt(age);
}
@Override
public void readExternal(ObjectInput in) throws IOException {
name = in.readUTF();
age = in.readInt(); // Order must match
}
4. Not Handling Exceptions Properly
Serialization and I/O operations can throw a variety of exceptions. Always ensure these are adequately caught and handled.
Why It Matters: Unhandled exceptions can lead to application crashes, making your system unstable.
Solution:
Implement proper exception handling within the writeExternal
and readExternal
methods:
@Override
public void writeExternal(ObjectOutput out) {
try {
out.writeUTF(name);
out.writeInt(age);
} catch (IOException e) {
System.err.println("IOException during serialization: " + e.getMessage());
}
}
@Override
public void readExternal(ObjectInput in) {
try {
name = in.readUTF();
age = in.readInt();
} catch (IOException e) {
System.err.println("IOException during deserialization: " + e.getMessage());
}
}
5. Failing to Consider Version Control
When modifying your Externalizable
classes over time, it’s crucial to implement version control.
Why It Matters: Different versions of the same class might lead to incompatible object states.
Solution:
Introduce a serialVersionUID
in your class and increment it when you make changes that affect serialization:
private static final long serialVersionUID = 1L; // Change this when structuring the class
6. Not Validating Data During Deserialization
Failure to validate the data when loading can introduce bugs or security vulnerabilities.
Why It Matters: This could result in corrupted objects or potential security flaws if bad data is stored.
Solution:
Consider validating all data after deserialization and throwing exceptions or correcting states accordingly.
@Override
public void readExternal(ObjectInput in) throws IOException {
name = in.readUTF();
age = in.readInt();
// Validate state
if (age < 0) {
throw new IOException("Invalid age value");
}
}
Lessons Learned
Java Externalization is a nuanced mechanism that provides improved control over serialization. However, pitfalls such as forgetting a no-argument constructor, ignoring transient fields, or neglecting exception handling can lead to significant issues in your applications.
By keeping these common pitfalls in mind and applying the suggested mitigation strategies, you can significantly enhance the reliability of your Java applications.
For more in-depth reading on Java serialization and externalization, consider checking the official Java documentation or the Java Tutorials.
With thoughtful implementation and diligent attention to detail, your journey with externalization can be smooth and rewarding.
Happy coding!
Checkout our other articles