Why Ignoring Singleton Locks Can Break Your Java EE App
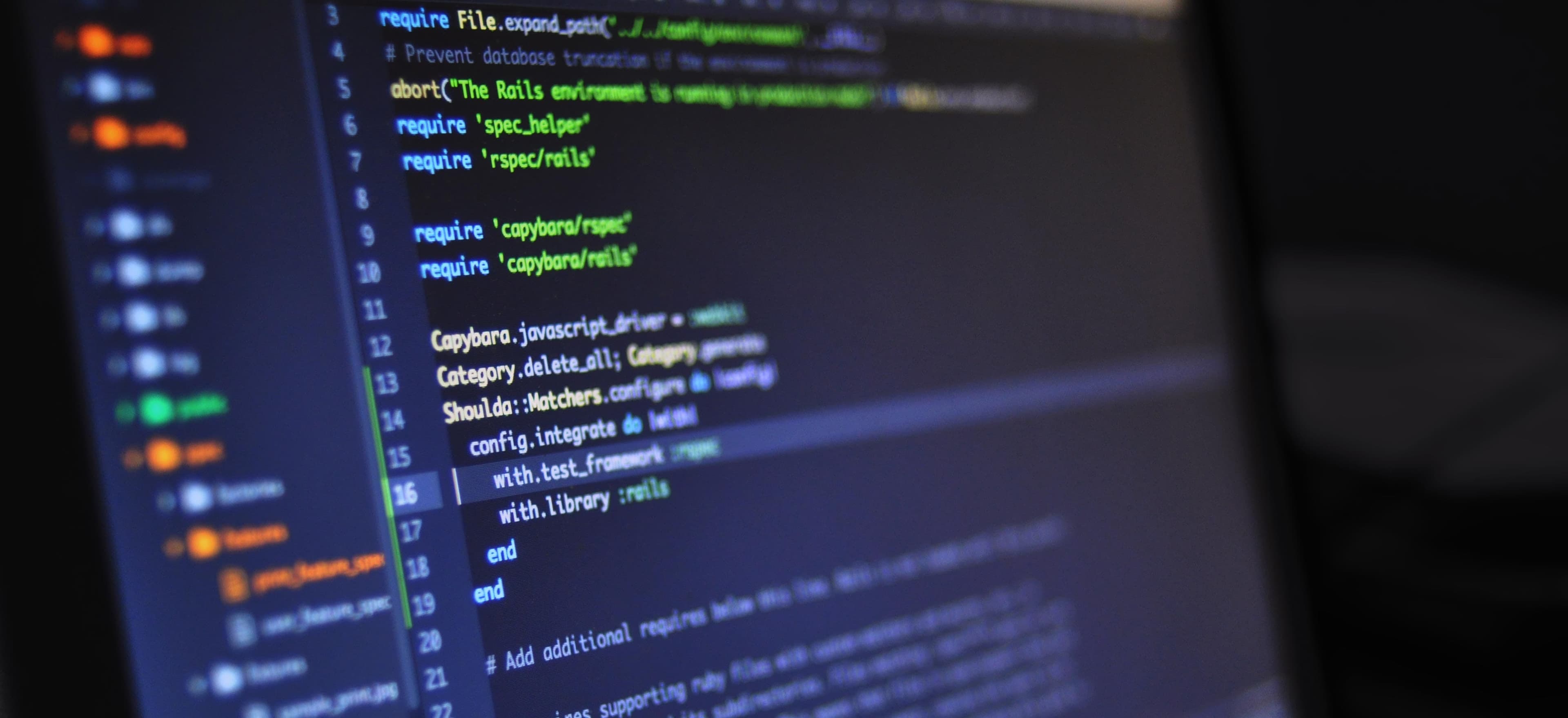
- Published on
Why Ignoring Singleton Locks Can Break Your Java EE App
Java EE (Enterprise Edition) is a robust framework that aids in the development of large-scale, multi-tiered, and distributed applications. While Java EE provides many advanced features, developers should pay close attention to concurrency control, especially when it includes singleton patterns. Ignoring singleton locks can lead to unintended consequences that may cripple your application. In this post, we will explore the significance of singleton locks, the potential pitfalls of ignoring them, and best practices to ensure your Java EE application remains robust and responsive.
Understanding the Singleton Pattern
The Singleton pattern is a design pattern that restricts instantiation of a class to one "single" instance. It is particularly helpful when managing shared resources in multithreaded applications. In Java, the typical implementation involves the following elements:
- A private constructor, to prevent external instantiation.
- A static variable to hold the single instance.
- A public static method that returns the instance.
Simple Singleton Implementation
public class Singleton {
private static Singleton instance;
// Private constructor
private Singleton() {}
// Get the only instance available
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
Commentary on Singleton Implementation
In the above code, we define a simple singleton. However, this implementation is not thread-safe. If two threads call getInstance()
simultaneously, we could end up with two instances. Thus, it leads us to the topic of locking.
Importance of Singleton Locks
In a concurrent environment, a “singleton” can easily become a “doubleton” or even worse—leading to multiple instances of a class that should only have one. This situation can occur in environments where multiple threads access the singleton instance concurrently.
Thread Safety
To ensure thread safety in a singleton, we can enhance the getInstance()
method as follows:
public class Singleton {
private static Singleton instance;
// Private constructor
private Singleton() {}
// Synchronized method to control access
public synchronized static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
Here, we used the synchronized
keyword, which allows only one thread to execute getInstance()
at a time, preventing multiple instances.
Commentary on Synchronized Singleton
While this ensures thread safety, it comes at a performance cost whenever getInstance()
is called. This is especially problematic in high-performance applications. Therefore, developers should explore other synchronization mechanisms, like using the "Bill Pugh Singleton" approach or "Initialization-on-demand holder idiom."
Problems with Ignoring Singleton Locks
Ignoring proper locking in singleton patterns can lead directly to a host of issues:
-
Inconsistency: If multiple parts of your application are accessing a non-locked singleton, they may operate on different states, leading to unpredictable behavior.
-
Resource Leaks: Without mutex locking, resources might be allocated multiple times without release, consuming system memory.
-
Database Constraints: In Java EE applications, singleton patterns often manage connections to databases. Multiple instances may open connections leading to exceeding the maximum number of allowable connections.
Example: Resource Management Failure
Consider an example where a singleton manages a database connection pool:
public class ConnectionPool {
private static ConnectionPool instance;
// Private constructor
private ConnectionPool() {
// Initialize connection pool
}
// Synchronized to prevent multiple instances
public synchronized static ConnectionPool getInstance() {
if (instance == null) {
instance = new ConnectionPool();
}
return instance;
}
public Connection getConnection() {
// Return a connection from the pool
}
}
Negative Consequence
If getInstance()
was unsynchronized, multiple threads might create multiple ConnectionPool
instances, each managing its own pool of connections. Such chaos can lead to resource exhaustion and a breakdown of application performance.
Best Practices for Using Singleton Locks in Java EE
-
Check Thread Local Variables: If scoped properly, Thread Local variables can reduce the need for synchronization while maintaining thread safety.
-
Use the Enum Singleton: It's a safe and efficient way to implement the singleton pattern in Java. Java guarantees that an enum type is instantiated only once.
public enum Singleton { INSTANCE; public void someMethod() { // Implementation here } }
-
Implement Lazy Loading with Double-Checked Locking: In high-performance applications, you can implement a double-checked locking mechanism that minimizes synchronization overhead.
public class Singleton { private static volatile Singleton instance; private Singleton() { } public static Singleton getInstance() { if (instance == null) { synchronized (Singleton.class) { if (instance == null) { instance = new Singleton(); } } } return instance; } }
Why Choose Double-Checked Locking?
This method improves performance by only synchronizing when the singleton instance is null. It minimizes the use of synchronized blocks, which can create bottlenecks in multi-threaded environments.
To Wrap Things Up
Understanding the importance of singleton locks in a Java EE application is not just a matter of following best practices; it is essential for the stability and reliability of your application. Ignoring these can lead to hard-to-track bugs, inconsistent application states, and performance degradation.
Incorporate appropriate singleton patterns with locking mechanisms and be vigilant about resource management. Employ strategies like double-checked locking, Thread Local variables, or Enum singletons. By doing so, you will help ensure a robust, high-performing Java EE application that can handle the demands of concurrent access.
For more details about Java concurrency models, check out the Java Concurrency in Practice book for a deeper dive into these topics.
Moreover, if you're looking for further reading on design patterns in Java, consider visiting Refactoring Guru for a comprehensive guide on how to implement various design patterns effectively.
Remember, in the world of Java EE, careful attention to singleton design can be the difference between a scalable application and a fragile one. Happy coding!
Checkout our other articles