Common Pitfalls in Spring Field Dependency Injection
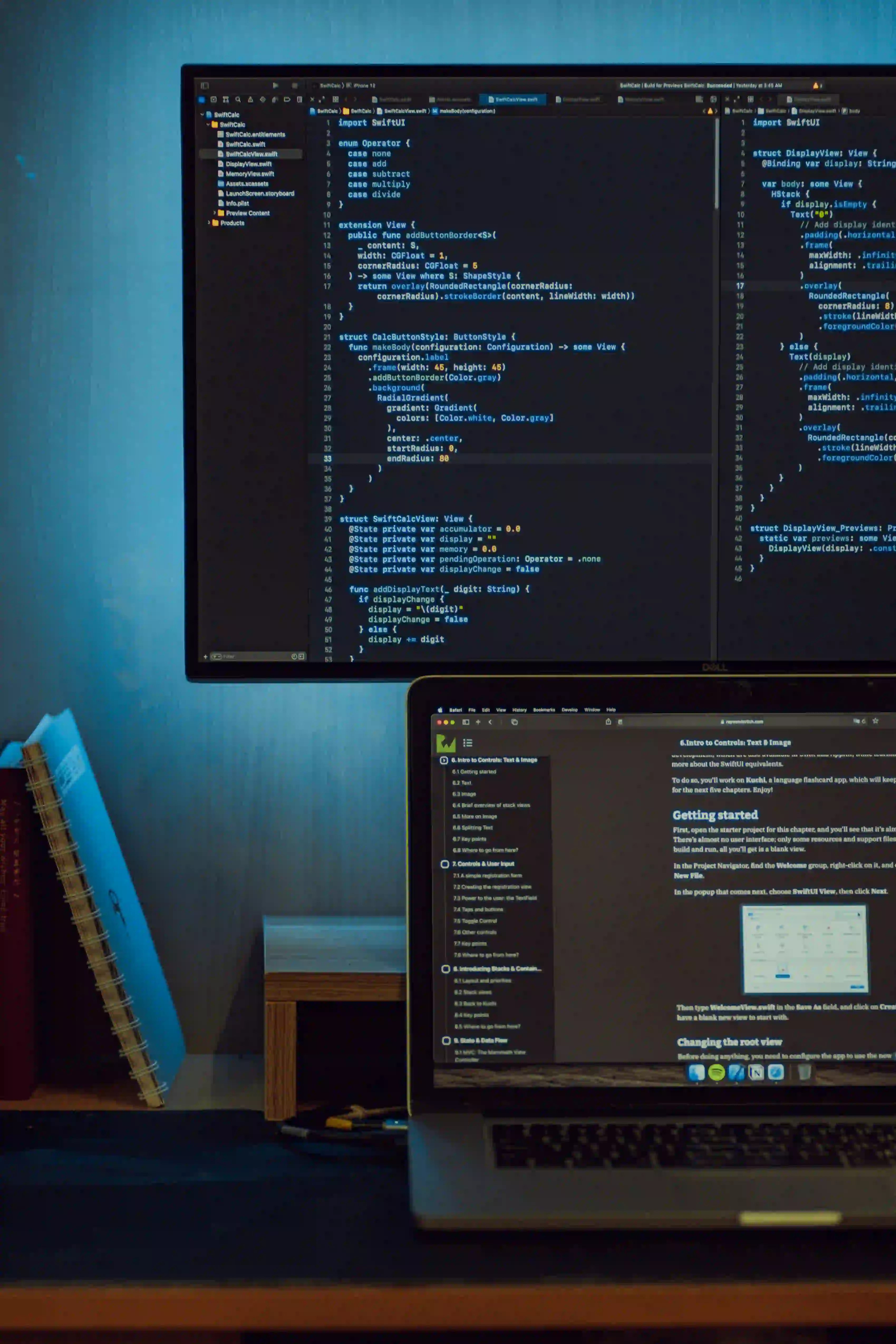
Common Pitfalls in Spring Field Dependency Injection
Spring Framework has revolutionized how Java developers create applications by introducing the concept of Dependency Injection (DI). Among various DI methods in Spring, field injection is often lauded for its ease of use. However, it isn't without its challenges. In this blog post, we will delve into the common pitfalls of field dependency injection in Spring, discuss best practices, and provide solutions to potential issues.
Let's start by understanding how field dependency injection works in Spring.
What is Field Dependency Injection?
Field dependency injection is a technique where Spring automatically injects dependencies directly into the fields of a class. This is typically accomplished using the @Autowired
annotation:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
public class ExampleService {
@Autowired
private ExampleRepository exampleRepository;
public void performOperation() {
exampleRepository.doSomething();
}
}
While this approach is convenient, it can lead to issues, such as reduced testability and hidden dependencies. Let's examine these pitfalls in detail.
Pitfall 1: Hidden Dependencies
One of the most significant drawbacks of field injection is that it can lead to hidden dependencies. When dependencies are injected directly into fields, it becomes challenging to understand what a class requires for its operation.
Solution: Constructor Injection
To make dependencies explicit, prefer constructor injection over field injection. Constructor injection makes it clear what dependencies are necessary by requiring them as parameters. Here's how to implement it:
import org.springframework.stereotype.Component;
@Component
public class ExampleService {
private final ExampleRepository exampleRepository;
public ExampleService(ExampleRepository exampleRepository) {
this.exampleRepository = exampleRepository;
}
public void performOperation() {
exampleRepository.doSomething();
}
}
This method enhances code readability and maintainability, providing better insights into class design.
Pitfall 2: Difficulty in Unit Testing
Field injection can complicate unit testing. Since dependencies are hidden, you can't easily mock or set them in a testing environment. Consider the example:
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
@SpringBootTest
public class ExampleServiceTest {
@Autowired
private ExampleService exampleService;
@Test
void testPerformOperation() {
// Test logic here
}
}
In this scenario, the test cannot isolate ExampleService
effectively.
Solution: Use Constructor Injection with Mocking Frameworks
Using constructor injection allows you to pass mocked dependencies when creating the instance for testing:
import static org.mockito.Mockito.*;
public class ExampleServiceTest {
private ExampleRepository exampleRepositoryMock;
private ExampleService exampleService;
@BeforeEach
void setUp() {
exampleRepositoryMock = mock(ExampleRepository.class);
exampleService = new ExampleService(exampleRepositoryMock);
}
@Test
void testPerformOperation() {
exampleService.performOperation();
verify(exampleRepositoryMock).doSomething();
}
}
This way, your tests become more focused and easier to maintain.
Pitfall 3: Immutability Issues
Field injection can undermine the immutability of your classes. With field injection, fields can be changed after object construction. This could lead to inconsistent states, making your code less predictable.
Solution: Use Constructor Injection
Again, constructor injection can help maintain immutability. Once the dependencies are passed into the constructor, they can be marked as final
. Here’s an example:
public class ExampleService {
private final ExampleRepository exampleRepository;
public ExampleService(ExampleRepository exampleRepository) {
this.exampleRepository = exampleRepository;
}
}
By doing this, you ensure that your service maintains a consistent state throughout its lifecycle.
Pitfall 4: Circular Dependencies
Circular dependencies occur when two or more beans depend on each other. Field injection can make this problem less obvious than when you’re using constructor injection, which doesn’t permit circular dependencies by default.
Solution: Refactor Your Code
To resolve circular dependencies, you can refactor your code to eliminate unnecessary dependencies. If two classes depend on each other, you might consider extracting the common functionality into a third class or using interfaces.
Here's a more straightforward approach using setter injection to break the cycle:
@Component
public class ClassA {
private ClassB classB;
@Autowired
public void setClassB(ClassB classB) {
this.classB = classB;
}
}
@Component
public class ClassB {
private ClassA classA;
@Autowired
public void setClassA(ClassA classA) {
this.classA = classA;
}
}
Pitfall 5: Late Initialization
Field injection may lead to issues with late initialization of dependencies. If a field is initialized after the object has been constructed, there could be scenarios where the dependency is accessed before it’s set.
Solution: Use @PostConstruct
To avoid late initialization, you can use the @PostConstruct
annotation to ensure that a method runs right after the bean's properties have been set. However, it is still advisable to use constructor injection for better clarity.
import javax.annotation.PostConstruct;
@Component
public class ExampleService {
@Autowired
private ExampleRepository exampleRepository;
@PostConstruct
public void init() {
// Use exampleRepository safely after it's guaranteed to be initialized
}
}
Final Thoughts
While field dependency injection in Spring may seem easy to implement, it carries several pitfalls that can affect your application's maintainability, testability, and clarity. By opting for constructor injection, you can increase the transparency of dependencies, ensure immutability, and facilitate unit testing.
For more information on dependency injection in Spring, consider referring to the official Spring documentation and resources such as Spring in Action.
It's crucial as a developer to choose the right approach for dependency management, as your choice can significantly impact the overall quality of your code. By avoiding field injection pitfalls, you will foster a cleaner, clearer, and more maintainable codebase. Happy coding!