Unlocking the Mystery: Deep Visibility in Docker Microservices
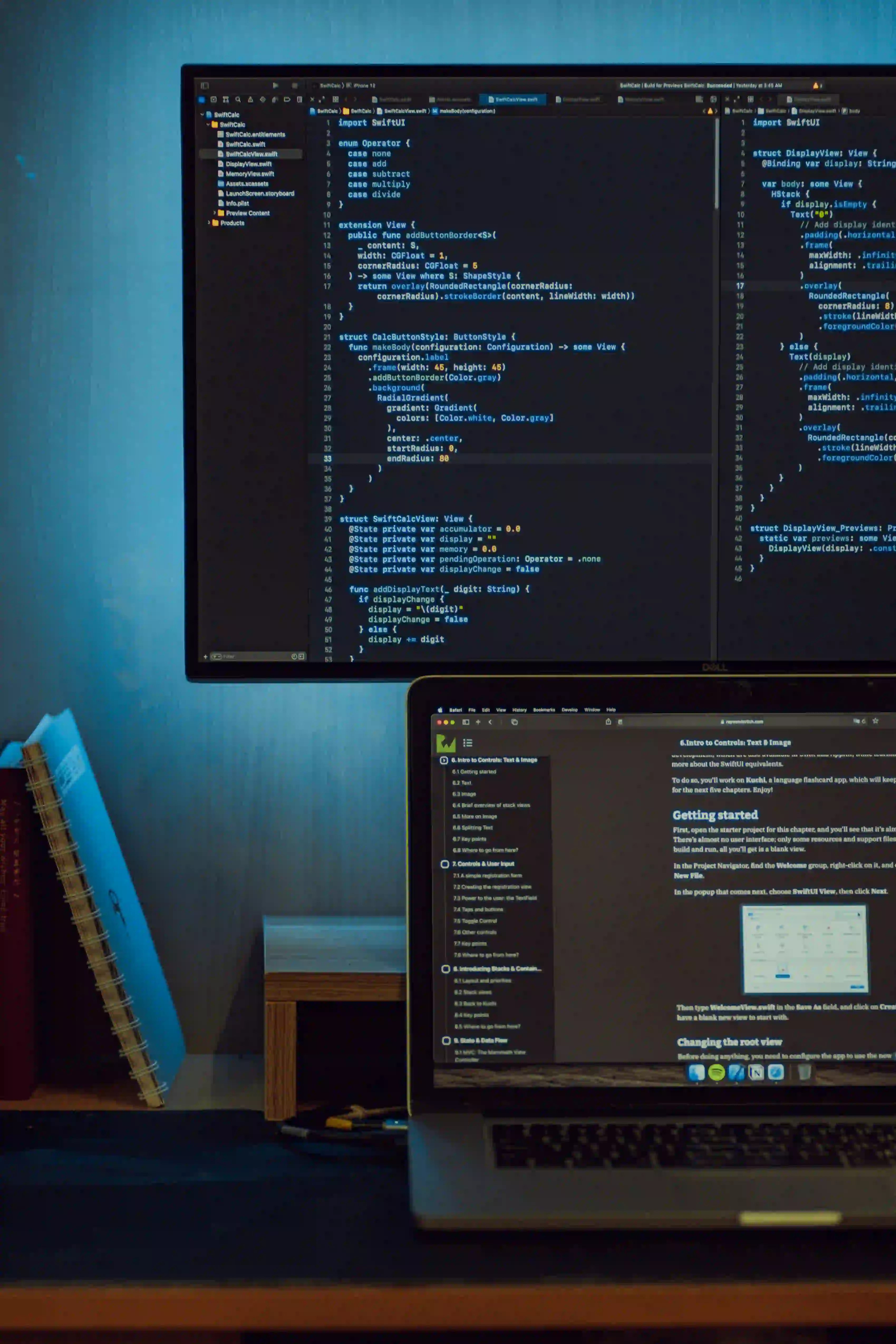
Unlocking the Mystery: Deep Visibility in Docker Microservices
As the world shifts increasingly towards microservices architecture, the need for deep visibility into these systems has never been more critical. Understanding the performance and behavior of microservices deployed in Docker containers is pivotal for effective monitoring and troubleshooting. This blog post dives into the techniques and tools that enable you to achieve deep visibility in Docker-based microservices applications.
Understanding Microservices Architecture
Microservices architecture breaks a large application into smaller, manageable, and loosely-coupled services. Each service can be developed, deployed, and scaled independently. This structure increases flexibility and scalability but also introduces complexity. A successful microservices architecture relies on multiple interacting services, so monitoring them is essential.
The Importance of Deep Visibility
Deep visibility refers to the comprehensive insights into the functionality, performance, and health of your microservices. With microservices often dependent on one another, a failure or performance hit in one service can cascade through the entire system. Here’s why deep visibility is crucial:
- Proactive Monitoring: Identifying issues before they affect users helps maintain an optimal user experience.
- Root Cause Analysis: When failures occur, having deep visibility aids in pinpointing the exact cause instead of relying solely on guesswork.
- Performance Optimization: Continuous monitoring highlights performance bottlenecks that may affect system efficiency.
Tools for Achieving Deep Visibility in Docker Microservices
Several tools and practices can help you achieve deep visibility into your Docker microservices. Here we highlight a few popular choices:
1. Prometheus and Grafana
Prometheus is an open-source monitoring and alerting toolkit, especially suited for microservices environments. It collects metrics from your applications and centralizes the data, making it easier to visualize trends over time. Grafana complements Prometheus by providing beautiful visualizations.
Example: Setting Up Prometheus for a Java Service
First, ensure your Java application exposes metrics in the Prometheus format. Here's a simple example using Spring Boot:
import io.prometheus.scrape.RequestMappingHandlerAdapter;
import io.prometheus.simpleclient.spring.SpringBootPrometheus;
@SpringBootApplication
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
@Bean
public ServletRegistrationBean<HttpServlet> prometheusServlet() {
return new ServletRegistrationBean<>(
new HttpServlet() {
protected void doGet(HttpServletRequest req, HttpServletResponse resp) {
// Implementation to expose metrics
}
}, "/metrics");
}
}
Why This Matters:
Exposing a /metrics
endpoint allows Prometheus to scrape your application's metrics at specified intervals. This continuous feedback will ultimately help in identifying any anomalies and making data-driven decisions.
2. ELK Stack (Elasticsearch, Logstash, and Kibana)
The ELK stack is a powerful toolset for logging purposes. It allows you to gather logs from your microservices and analyze them. This is particularly useful when debugging or monitoring service interactions.
Setting Up ELK Stack
When deploying your Docker containers, ensure that logs are directed to a location that Logstash can access. Here’s a basic Docker command to run Logstash:
docker run --rm -it \
--name logstash \
-v "$(pwd)/logstash.conf":/usr/share/logstash/pipeline/logstash.conf \
-e "LS_JAVA_OPTS=-Xmx1g -Xms1g" \
docker.elastic.co/logstash/logstash:7.10.0
Why This Matters:
By collecting logs, you gain insights into the exceptional events within your services, which is invaluable for understanding the flow of your application.
3. Distributed Tracing with OpenTracing and Jaeger
Distributed tracing is essential for microservices to understand the flow of requests across multiple services. OpenTracing is a specification that provides a standard way for instrumenting your code. Jaeger is an open-source tool that provides distributed context propagation.
Example: Integrating Jaeger with a Spring Boot Application
To instrument your service, you will typically start by including Jaeger dependencies:
<dependency>
<groupId>io.jaegertracing</groupId>
<artifactId>jaeger-client</artifactId>
<version>0.30.0</version>
</dependency>
Then, initialize Jaeger in your application:
import io.jaegertracing.Configuration;
@Configuration
public class TracingConfiguration {
@Bean
public io.opentracing.Tracer jaegerTracer() {
return new Configuration("YourServiceName").getTracer();
}
}
Why This Matters:
With distributed tracing, you can visualize how requests interact with your services. This clarity aids significantly in debugging complex interaction designs.
Best Practices for Achieving Deep Visibility
- Automate Monitoring: Automate the collection of metrics, logs, and traces to reduce human error and improve response times.
- Centralize Data: Use aggregated dashboards to view logs, metrics, and tracing information in one place. This heightens your ability to respond to issues quickly.
- Instrument Your Code: Ensure that all microservices are instrumented appropriately to provide consistent metrics and logging formats.
- Set Alerts: Set up alerts for abnormal spikes in latency or error rates. Early warnings can prevent larger system failures.
The Bottom Line
Achieving deep visibility in Docker-based microservices is fundamental to maintaining the health and performance of modern applications. Tools like Prometheus, ELK Stack, and Jaeger play a vital role in ensuring that you have comprehensive insights into your systems. By taking a proactive approach and incorporating these tools into your workflow, you can illuminate the dark corners of your microservices architecture and improve your operational efficiency.
For further reading, check out Microservices Monitoring with Prometheus and Distributed Context Propagation with OpenTracing. Begin your journey toward deep visibility today to unlock the full potential of your Docker microservices!