Overcoming Builder Pattern Complexity in Software Design
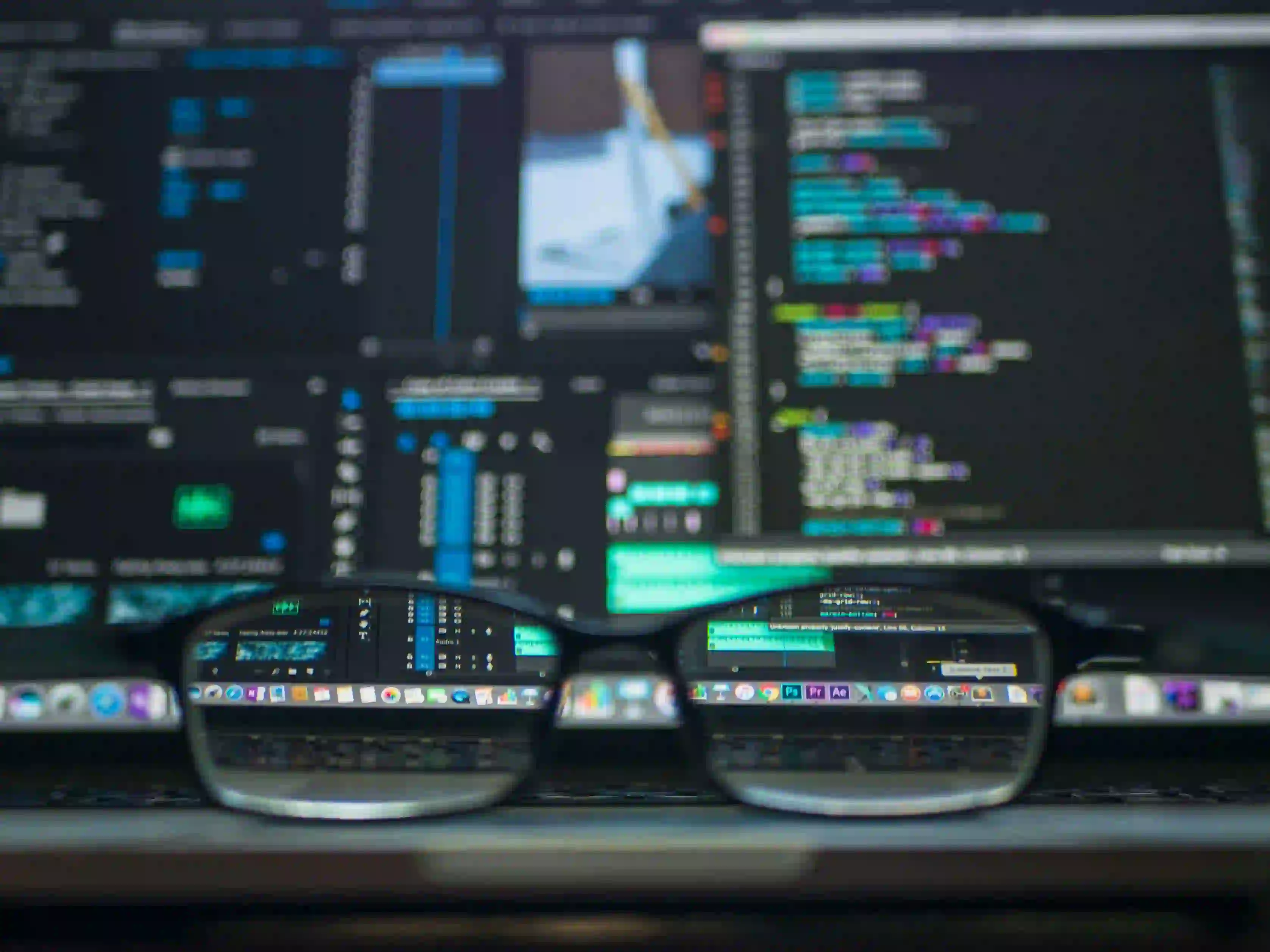
Overcoming Builder Pattern Complexity in Software Design
When it comes to software design, the Builder pattern stands out as an elegant solution for constructing complex objects. Yet, it is often viewed as overly complex or cumbersome. In this blog post, we will delve into the intricacies of the Builder pattern, discuss its advantages and disadvantages, and explore strategies to simplify its implementation. By the end of this post, you will not only understand how to implement the Builder pattern more effectively but also grasp the nuances that often lead to its misuse.
Understanding the Builder Pattern
The Builder pattern is one of the creational design patterns, allowing developers to construct objects step by step. Unlike static factory methods or constructors, the Builder separates the construction of a complex object from its representation. This separation provides better control over the construction process.
The Builder Pattern Structure
A typical implementation of the Builder pattern involves four main components:
- Product: The complex object being created.
- Builder: An interface or abstract class defining methods for creating the parts of the product.
- Concrete Builder: Implements the Builder interface and defines the specific representations.
- Director: Responsible for managing the construction process.
Here's a simple visualization:
+--------------+
| Director |
+--------------+
|
| uses
V
+--------------+
| Builder |<-----------------+
+--------------+ |
| +-----+
| builds | Product |
| +-----+
V
+---------------------+
| ConcreteBuilder |
+---------------------+
A Basic Example in Java
Let’s proceed with a basic implementation of the Builder pattern in Java. We will create a Car
with various attributes:
// Product
public class Car {
private final String engine;
private final String color;
private final int seats;
// private constructor to enforce the use of Builder
private Car(CarBuilder builder) {
this.engine = builder.engine;
this.color = builder.color;
this.seats = builder.seats;
}
@Override
public String toString() {
return "Car [engine=" + engine + ", color=" + color + ", seats=" + seats + "]";
}
// Static inner Builder class
public static class CarBuilder {
private String engine;
private String color;
private int seats;
public CarBuilder setEngine(String engine) {
this.engine = engine;
return this; // allow chaining
}
public CarBuilder setColor(String color) {
this.color = color;
return this; // allow chaining
}
public CarBuilder setSeats(int seats) {
this.seats = seats;
return this; // allow chaining
}
public Car build() {
return new Car(this); // create the Car instance
}
}
}
Why Use the Builder?
- Clarity: The Builder makes the instantiation of complex objects clearer and easier to understand.
- Immutability: The Car object is immutable (its state can't be changed once created), which is beneficial in multi-threaded applications.
- Flexibility: Each builder method can be optional, allowing the client code to specify only the parameters it cares about.
Usage would look like this:
public class Main {
public static void main(String[] args) {
Car car = new Car.CarBuilder()
.setEngine("V8")
.setColor("Red")
.setSeats(4)
.build();
System.out.println(car);
}
}
Challenges of Using the Builder Pattern
While the Builder pattern has its advantages, there are challenges:
-
Excessive Boilerplate: The Builder pattern can introduce a lot of boilerplate code, especially when there are many attributes to manage. This can lead to a complex codebase that becomes hard to maintain.
-
Cognitive Overhead: Developers unfamiliar with the pattern may find the separation of concerns confusing, as it can obscure the actual construction logic of the product.
-
Over-Engineering: Developers might apply the Builder pattern even when it is unnecessary. For instance, using it on simple objects with only a couple of fields can over-complicate the design.
Strategies to Overcome Complexity
To mitigate these challenges, consider the following strategies:
1. Use Fluent Interfaces Wisely
Fluent interfaces allow for method chaining, creating a more readable code structure. In the example above, notice how each setter method returns this
. This pattern promotes a clean and expressive construction process.
2. Group Related Parameters
When constructing objects with numerous attributes, group related parameters to simplify the interface. For example, in a Person
class, create a nested AddressBuilder
for address-related attributes.
public class Person {
private final String name;
private final Address address;
private Person(PersonBuilder builder) {
this.name = builder.name;
this.address = builder.address;
}
public static class Address {
private String street;
private String city;
// Getters...
}
public static class PersonBuilder {
private String name;
private Address address;
public PersonBuilder setName(String name) {
this.name = name;
return this;
}
public PersonBuilder setAddress(Address address) {
this.address = address;
return this;
}
// Other builder methods…
public Person build() {
return new Person(this);
}
}
}
3. Limit the Builder's Responsibility
Ensure that the Builder's role is limited to building the object. Avoid adding validation or business logic inside the Builder. Maintain single responsibility to keep the codebase manageable.
4. Consider Composition Over Inheritance
If a product can be built using existing objects, consider using composition rather than the Builder pattern. This often results in simpler and more maintainable code. Here's a link to a resource that dives deeper into composition: Composition vs Inheritance.
Lessons Learned
The Builder pattern is a powerful design pattern that can significantly improve the clarity and manageability of your code when constructing complex objects. However, its implementation must be approached carefully to avoid unnecessary complexity and over-engineering.
By leveraging fluent interfaces, limiting responsibilities, and considering simpler alternatives when appropriate, developers can mitigate the challenges associated with the Builder pattern and enhance their overall software design.
Feel free to dive deeper into the Builder pattern and its applications in software design through these resources: Design Patterns in Java and explore its benefits for your next project! Happy coding!