Avoiding the Seven Deadly Sins of Software Testing
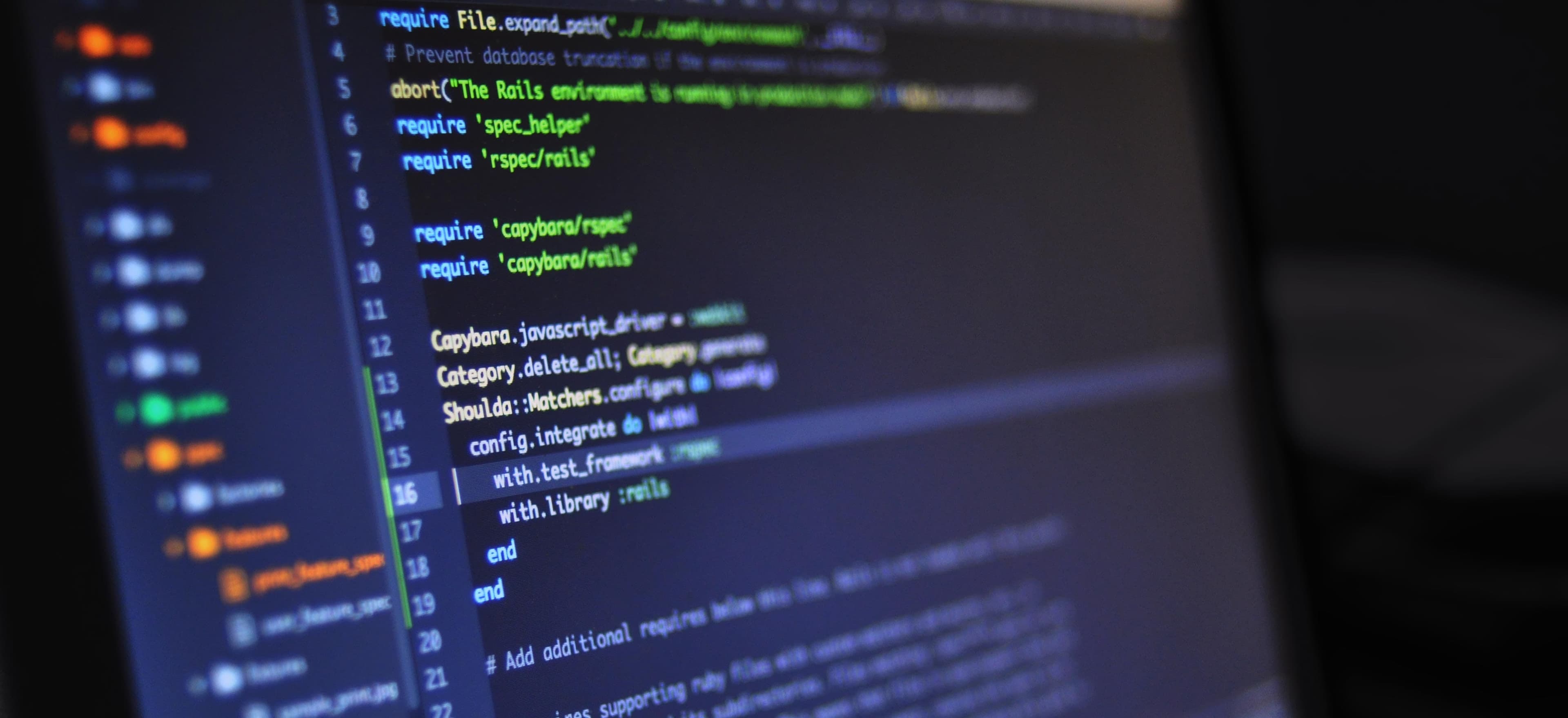
- Published on
Avoiding the Seven Deadly Sins of Software Testing
Software testing is a crucial process in this age of rapid software development. However, jumping into testing without a thoughtful strategy can lead to significant pitfalls. Understanding and avoiding the seven deadly sins of software testing can help ensure efficient and effective testing practices. This blog post will explore these pitfalls in detail and offer insights on how to avoid them.
1. The Sin of Inadequate Test Coverage
What is it?
Inadequate test coverage occurs when tests do not sufficiently exercise the application code. This leads to undetected bugs and issues which may impact user experience.
Why Avoid It?
Your application can only ensure quality if its features are thoroughly tested. Inadequate test coverage can mean more critical bugs slip through unnoticed, leading to costly fixes in production.
How to Prevent This
Adopt approaches like Equivalence Partitioning and Boundary Value Analysis to improve the quality of your test cases.
public class Calculator {
public int add(int x, int y) {
return x + y;
}
}
// Example of Equivalence Partitioning
public class CalculatorTest {
@Test
public void testAdd() {
Calculator calc = new Calculator();
assertEquals("Regular addition test", 5, calc.add(2, 3)); // Valid case
System.out.println("Tested valid addition case.");
}
}
By systematically ensuring you validate all types of input scenarios, you can enhance your test coverage significantly.
2. The Sin of Ignoring Automated Testing
What is it?
Automated testing is often underutilized or neglected altogether, leading to repetitive manual testing efforts.
Why Avoid It?
Manual testing is labor-intensive and error-prone. Automation can significantly reduce the time and effort required for extensive test suites.
How to Prevent This
Utilize testing frameworks such as JUnit or TestNG for Java to automate your testing process.
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class CalculatorTest {
private Calculator calc = new Calculator();
@Test
public void testAdd() {
assertEquals(5, calc.add(2, 3));
}
@Test
public void testAddNegativeNumbers() {
assertEquals(1, calc.add(-2, 3));
}
}
Each test case here can be re-run multiple times without additional manual effort, freeing up precious resources.
3. The Sin of Poorly Defined Requirements
What is it?
Poorly defined requirements lead to ambiguity in what needs to be tested.
Why Avoid It?
Testing against unclear requirements can lead to focus on the wrong features, wasting time and resources.
How to Prevent This
Engage with stakeholders early in the process to gather clear and concise requirements. Tools like JIRA can help track these requirements effectively.
4. The Sin of Over-Testing
What is it?
Over-testing refers to an excessive focus on testing both functionality that is non-essential and edge cases that are improbable.
Why Avoid It?
While covering edge cases can be important, excessive testing can stall your development process and waste resources.
How to Prevent This
Use risk-based testing to prioritize which areas of your application need the most thorough testing. Focus on the "what ifs" that matter most to your users.
5. The Sin of Neglecting Documentation
What is it?
Neglecting testing documentation can lead to misunderstandings and a lack of clarity around test cases, environments, and outcomes.
Why Avoid It?
Good documentation is crucial for facilitating communication among team members, especially those who may join the project later.
How to Prevent This
Use a well-defined documentation process, including tools like Confluence, where each test case, environment detail, and outcome is documented.
6. The Sin of Not Retesting
What is it?
Once a bug is fixed, failing to execute a retest to confirm the fix is a critical mistake.
Why Avoid It?
Not retesting can mean the issue remains unresolved, or even worse, that it introduces new bugs into an already functioning component.
How to Prevent This
Implement a regression testing suite that runs every time there are code changes.
public class CalculatorTest {
private Calculator calc = new Calculator();
@Test
public void testAddAfterBugFix() {
// Assume a bug was fixed related to this functionality
assertEquals(4, calc.add(1, 3)); // Retesting clarified functionality
}
}
By having a structured regression testing plan, you can ensure that previously tested functionalities still work as expected.
7. The Sin of Failing to Automate Repetitive Tests
What is it?
Repetitive tasks in testing can drain resources and lead to burnout over time.
Why Avoid It?
By manually executing repetitive tests, your testers will spend valuable hours on tasks that could be automated.
How to Prevent This
Identify repeated tests and automate them using established tools.
public class SmokeTestSuite {
@Test
public void testSmoke() {
Calculator calc = new Calculator();
assertEquals("Smoke test for add", 7, calc.add(3, 4));
// Further smoke tests to ensure primary functionality works.
}
}
Automating repetitive tests not only saves time but also reduces the risk of human error.
Bringing It All Together
Avoiding the seven deadly sins of software testing—Inadequate Test Coverage, Ignoring Automation, Poorly Defined Requirements, Over-Testing, Neglecting Documentation, Not Retesting, and Failing to Automate Repetitive Tests—can dramatically improve the software testing process in your organization.
By implementing these strategies, your testing efforts will be more focused, efficient, and ultimately more successful. To dive deeper into software testing concepts, check out Software Testing Fundamentals or explore tools on JUnit Documentation suitable for your Java projects.
Happy Testing!
Checkout our other articles