Struggling with Markutils CLI in Java? Here's the Fix!
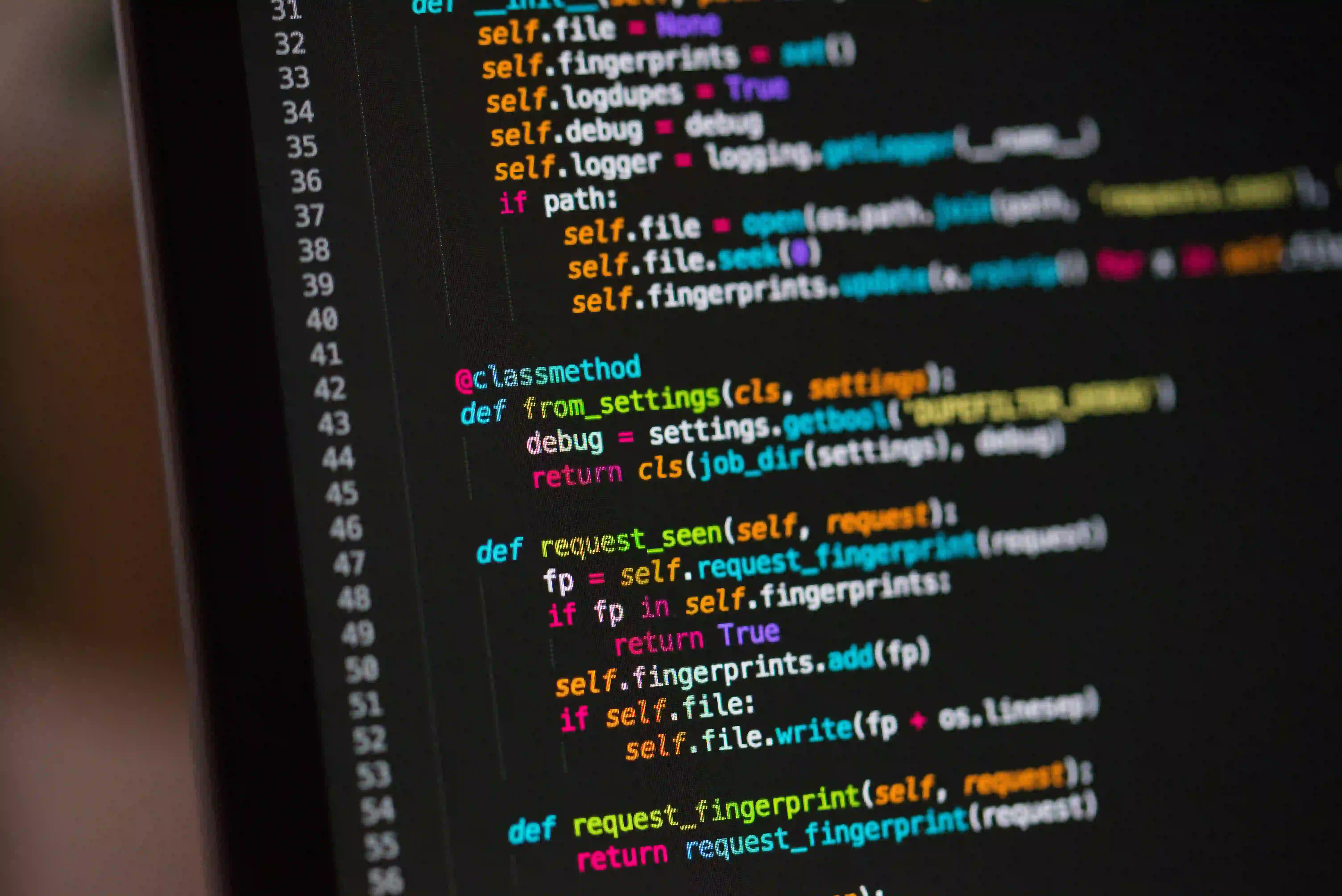
Struggling with Markutils CLI in Java? Here's the Fix!
Are you working with Markutils in your Java projects and facing challenges with the Command Line Interface (CLI)? Youβre not alone. Many developers encounter issues when trying to integrate libraries like Markutils into their Java applications. In this blog post, we will break down common problems, provide solutions, and give you a clear understanding of how to work with Markutils. Plus, we'll showcase some useful Java code snippets to help you make the most of this interface.
What is Markutils?
Markutils is a powerful Java library designed for parsing and converting Markdown into different formats. It is widely used for applications that require rendering documentation, README files, or any content written in Markdown syntax. However, like any library, it can present challenges, especially when using the CLI to process Markdown files.
Common Issues with Markutils CLI
Before diving into the solutions, let's outline some of the typical problems developers face when using the Markutils CLI:
- Incompatibility with Java Versions: Sometimes, using an outdated version of Java can lead to unexpected errors.
- Command Syntax Errors: Misunderstanding how to properly use the command line can cause frustration.
- File Not Found Errors: This often occurs when specifying paths incorrectly.
- Dependency Issues: Not having the required dependencies in your project can lead to runtime errors.
Pre-requisites
Ensure that you have the following set up on your machine:
- Java Development Kit (JDK): Version 8 or above is recommended.
- Maven: A popular build tool, often used in Java projects.
- Markutils: You can add it to your project dependencies.
Here is how you can add Markutils to your Maven project:
<dependency>
<groupId>org.commonmark</groupId>
<artifactId>commonmark</artifactId>
<version>0.17.0</version>
</dependency>
Fixing the Issues: Step-by-Step Guide
1. Check Java Compatibility
Make sure your Java version is compatible with Markutils. You can check your Java version by running the following command:
java -version
If you find that your version is outdated, consider updating your JDK. Hereβs a quick guide on how to install Java if needed.
2. Correct Command Syntax
Whenever you run a command using Markutils CLI, the syntax is crucial. Here is the correct way to call it:
java -jar markutils-cli.jar -i input.md -o output.pdf
Explanation:
java -jar
: This tells the command line to execute the jar file.markutils-cli.jar
: The name of the Markutils CLI jar.-i input.md
: Specifies the input Markdown file.-o output.pdf
: Indicates the output file in PDF format.
Tip
If you encounter a File Not Found
error, double-check the path for your input Markdown file to ensure it exists.
3. Handling File Not Found Errors
Incorrect file paths can derail your command. Always ensure you provide the correct relative or absolute path. Here is how a typical structure might look:
/project
βββ /src
βββ input.md
βββ markutils-cli.jar
To execute the command from the /project
directory:
java -jar markutils-cli.jar -i ./input.md -o ./output.pdf
4. Ensuring All Dependencies are Included
Dependency management is essential. If you see errors regarding missing classes or methods, check your pom.xml
(for Maven projects) to ensure all required libraries are included. You can always refer to the official Markutils repository for a complete list of dependencies.
Code Snippets for Enhancements
Often, the CLI isn't enough. You may need to programmatically use Markutils in your application. Here's how you can integrate Markutils in Java to parse a Markdown file:
import org.commonmark.parser.Parser;
import org.commonmark.renderer.html.HtmlRenderer;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Paths;
public class MarkdownConverter {
public static void main(String[] args) {
// Ensure that the path provided exists
String inputFilePath = "input.md";
String markdownContent = readMarkdownFile(inputFilePath);
String htmlContent = convertMarkdownToHtml(markdownContent);
// Output the HTML content, save it as needed
System.out.println(htmlContent);
}
public static String readMarkdownFile(String filePath) {
try {
return new String(Files.readAllBytes(Paths.get(filePath)));
} catch (IOException e) {
e.printStackTrace();
return "";
}
}
public static String convertMarkdownToHtml(String markdown) {
Parser parser = Parser.builder().build();
HtmlRenderer renderer = HtmlRenderer.builder().build();
return renderer.render(parser.parse(markdown));
}
}
Code Commentary
- Import necessary Markutils classes for parsing and rendering.
- Read the Markdown file into a string using Java's
Files
class for simplicity. - Use the
Parser
to convert Markdown text into a document node. - Render the node into HTML using the
HtmlRenderer
.
This snippet demonstrates how you can parse and convert Markdown content using Java code rather than CLI. This is especially useful for developers working on larger applications needing more control over Markdown processing.
Summary
Using Markutils CLI can be effective, but it requires attention to detail regarding command syntax and Java compatibility. Ensure you keep your environment up-to-date, verify file paths, and manage dependencies properly. Whether you choose to use Markutils directly via the CLI or integrate it into your Java application programmatically, the flexibility and robustness of this library can significantly enhance your Markdown processing capabilities.
By following the steps outlined in this article, you can effectively overcome common challenges and harness the full power of Markutils in your Java projects. If you have further questions or need additional resources, donβt hesitate to explore the extensive documentation available on the Commonmark website. Happy coding!