Unlocking the Challenges of Reactive Programming in Java Loom
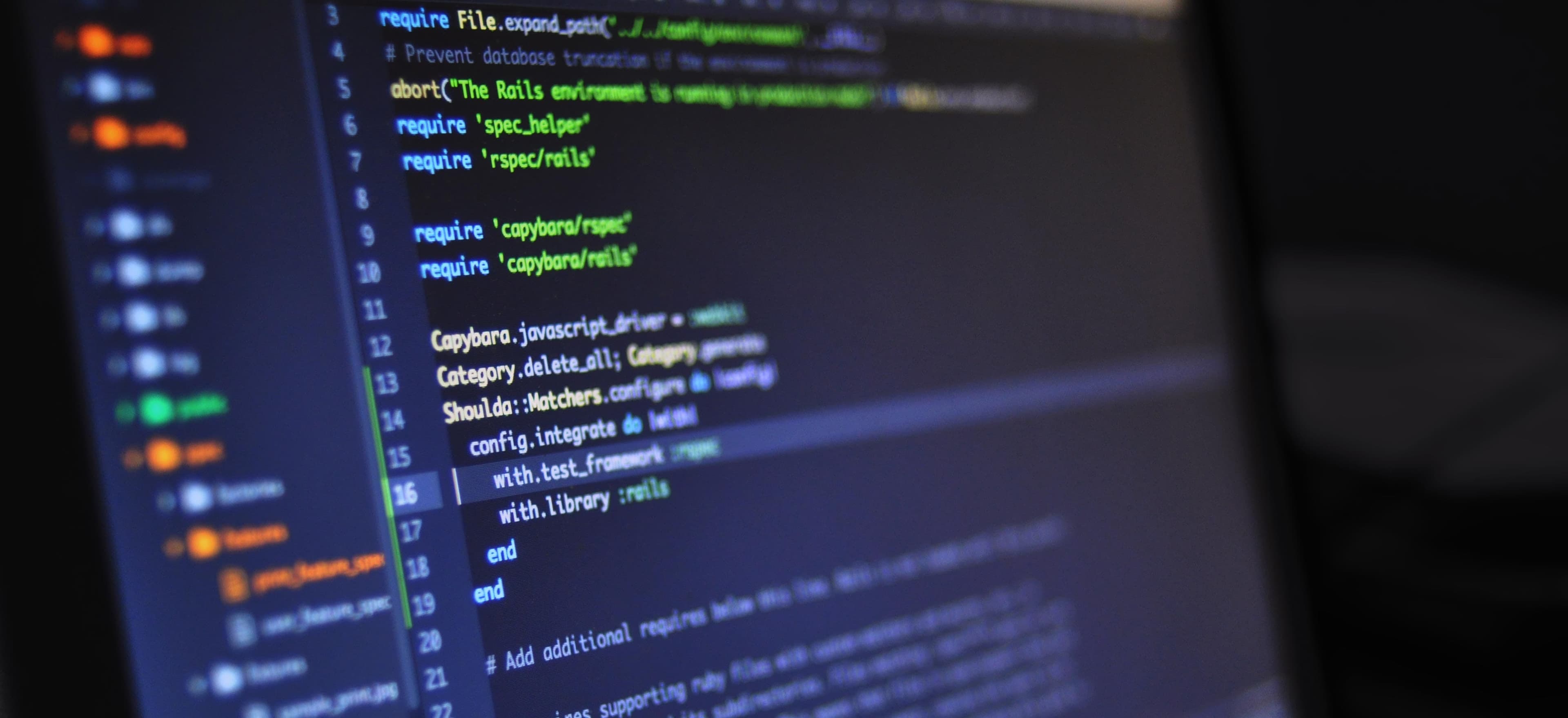
- Published on
Unlocking the Challenges of Reactive Programming in Java Loom
Reactive programming is becoming increasingly popular due to its ability to create highly responsive and dynamic applications. Java, with its rich ecosystem, is no stranger to this trend. Java Loom, a project aimed at enhancing Java's concurrency model, introduces lightweight, user-mode threads known as fibers. This evolution in Java can greatly simplify the complexities associated with reactive programming. In this blog post, we'll explore the challenges of reactive programming and delve into how Java Loom can address these issues effectively.
Understanding Reactive Programming
Before diving into the specifics of Java Loom, let's first understand what reactive programming is all about. Reactive programming is a programming paradigm that revolves around the concept of data streams and the propagation of change. This allows developers to work asynchronously and react to data as it arrives.
In reactive programming, the application can handle multiple streams of information concurrently, which is crucial for modern web applications that require real-time data processing. To illustrate this concept, consider an application that fetches updates from various data sources, like weather updates, stock prices, or social media feeds. A reactive approach allows these updates to be processed in a non-blocking manner.
Common Challenges in Reactive Programming
-
Complexity of Asynchronous Code: Managing multiple asynchronous operations can lead to "callback hell," where nested callbacks make the code unreadable and prone to bugs.
-
Error Handling: In a reactive system, where numerous stream events happen, debugging and error handling can become complicated. A failure in one part of the system can lead to cascading failures.
-
Resource Management: Reactive systems must handle a considerable number of concurrent tasks, and it can be challenging to manage memory and other resources efficiently.
-
Understanding Data Flows: As applications grow, so do the complexities of managing data flows and lifecycle states. Ensuring that all streams are handled correctly is vital.
Introducing Java Loom
Java Loom is a significant advancement in the Java ecosystem, targeting the challenges of concurrent programming. It introduces fibers, which are lightweight threads that can be suspended and resumed, allowing for more manageable concurrency. This alleviates the complexity often associated with traditional threading models.
How Java Loom Addresses Reactive Programming Challenges
Simplicity in Asynchronous Code
One of the hallmarks of Loom is its ability to simplify asynchronous programming. By using fibers, developers can write code that appears synchronous, even while executing asynchronously. This reduces the cognitive load when working with multiple data sources.
Here's a simple example that demonstrates how Loom makes handling asynchronous tasks easier:
import java.util.concurrent.CompletableFuture;
public class LoomExample {
public static void main(String[] args) {
CompletableFuture<Void> future = CompletableFuture.runAsync(() -> {
System.out.println("Fetching data...");
// Simulate a delay
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("Data fetched!");
});
// Do something else while waiting for the fetching task
System.out.println("Doing something else...");
// Wait for the future to complete
future.join();
}
}
Error Handling
Java Loom facilitates better error handling in reactive programming. By implementing structured concurrency, fibers allow you to better manage exceptions that occur in various parts of the application. For instance, you can use the try
-catch
mechanism in a cleaner manner compared to traditional approaches.
import java.util.concurrent.*;
public class ErrorHandlingExample {
public static void main(String[] args) {
CompletableFuture<Void> future = CompletableFuture.runAsync(() -> {
try {
// Simulated risky operation
riskyOperation();
} catch (Exception e) {
System.err.println("Error occurred: " + e.getMessage());
}
});
future.join();
}
private static void riskyOperation() {
throw new RuntimeException("Simulated failure");
}
}
Improved Resource Management
Managed fibers optimize resource usage. Loom allows hundreds of thousands of fibers to run concurrently, which is a significant improvement over traditional thread pools. Since fibers share a smaller footprint and are managed by the Java Virtual Machine, applications can scale more effectively without running into resource exhaustion issues.
import java.util.stream.IntStream;
public class ResourceManagementExample {
public static void main(String[] args) {
IntStream.range(0, 1_000_000).forEach(i -> {
// Each fiber runs a simple task
Thread.ofVirtual().start(() -> {
System.out.println("Running task " + i);
// Simulate some operation
});
});
// Delay the main thread to allow fibers to finish
try {
Thread.sleep(2000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
Reactivity with Structured Concurrency
Structured concurrency provides a way to group and manage concurrent tasks. With Loom, you can define scopes for fibers, making sure that they are completed or cleaned up properly upon exiting their context. This helps in maintaining clean data flows and systems.
import jdk.incubator.concurrent.ScopedValue;
public class StructuredConcurrencyExample {
public static void main(String[] args) {
ScopedValue<String> scopedValue = ScopedValue.newInstance();
Thread.ofVirtual().start(() -> {
scopedValue.set("Hello from fiber!");
System.out.println(scopedValue.get());
});
// The value will be cleaned up automatically after the fiber exits its scope
}
}
Lessons Learned
Java Loom represents a remarkable shift in how we can approach reactive programming. By addressing key challenges such as complexity, error handling, and resource management, Loom empowers developers to create responsive applications with less frustration and more natural code flow.
As we continue to explore the possibilities that reactive programming offers in tandem with Java Loom, we can expect to see a significant evolution in application design and development practices. For those who wish to explore these features further, check out the official Project Loom page for updates and insights.
Reactive programming becomes more manageable and efficient with Java Loom. This new paradigm gives developers the ability to think differently about concurrency, leading to more robust and scalable applications. Are you ready to embrace the future of reactive programming with Java Loom?
Checkout our other articles