Mastering Tomcat Shutdown: Avoiding Daemon Dilemmas
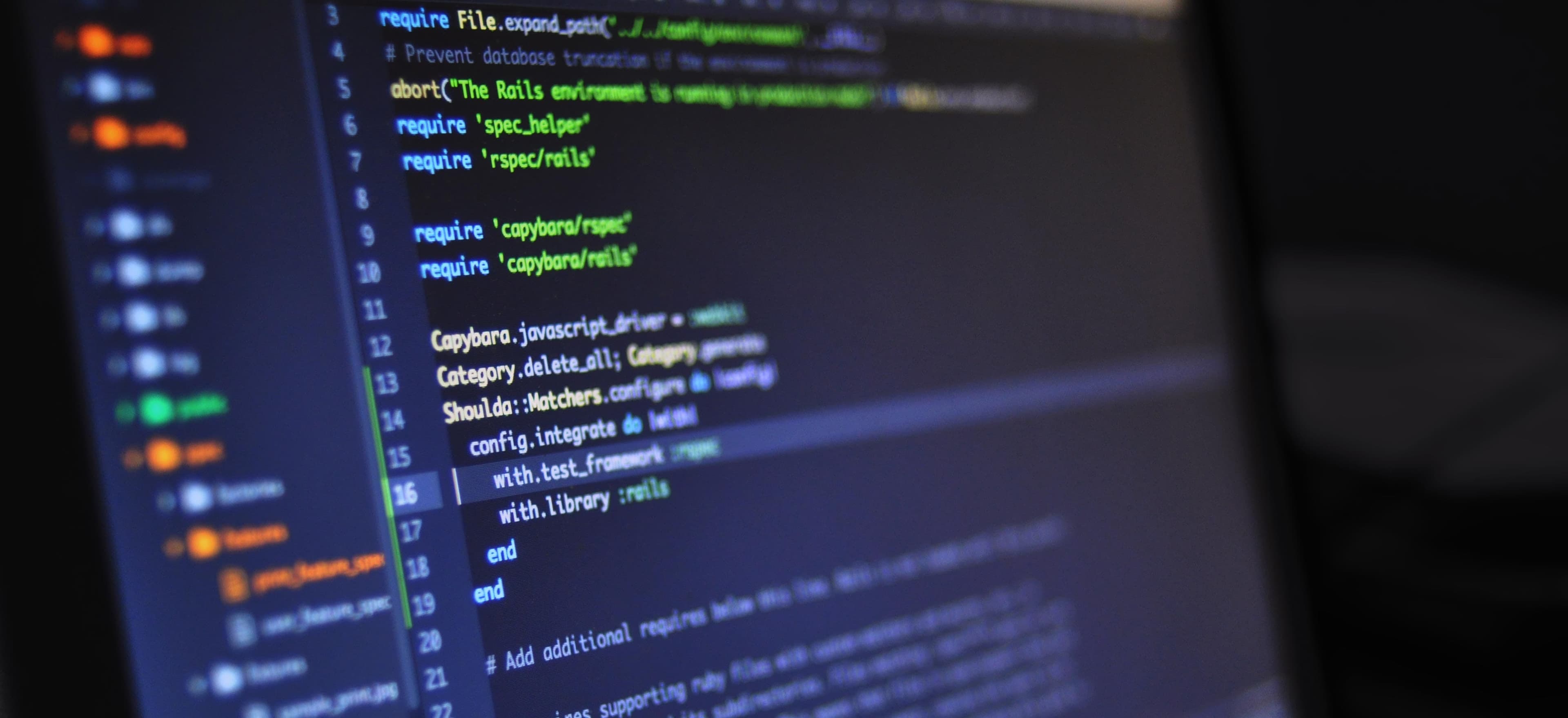
- Published on
Mastering Tomcat Shutdown: Avoiding Daemon Dilemmas
Apache Tomcat is one of the most widely used web servers and servlet containers. When deploying Java web applications, managing Tomcat's lifecycle activites—especially shutting it down gracefully—is crucial for ensuring reliable service and resource management. In this blog post, we will dive deep into strategies to master the shutdown process of Tomcat servers, helping you avoid common pitfalls and daemon dilemmas.
Understanding Tomcat's Lifecycle
Before we delve into the shutdown specifics, let's briefly discuss the lifecycle of a Tomcat server.
When you start a Tomcat server, several components are initialized:
- Connectors: These handle communication between the web server and clients.
- Contexts: Each web application runs in its own context.
- Lifecycle Listener: Monitors events in the server lifecycle.
When it comes time to shut down, it’s essential to target the entire servlet container, ensuring all resources are released properly.
The Importance of Graceful Shutdown
A graceful shutdown ensures that:
- Existing requests are completed.
- No new requests are accepted.
- Resources are released properly, preventing memory leaks.
In contrast, an abrupt termination can lead to incomplete transactions, corrupted data, and resource locks, you're better off avoiding.
How to Shut Down Tomcat Gracefully
Using the Shutdown Script
Tomcat provides shutdown.sh
(Linux/Unix) or shutdown.bat
(Windows) scripts located in the <TOMCAT_HOME>/bin
directory. Running these scripts initiates a graceful shutdown.
Command Line Example
From the terminal, execute:
cd /path/to/tomcat/bin
./shutdown.sh
Remember: You should check the Tomcat configuration for the shutdown port in server.xml
. The default is 8005
.
Why Use This Approach?
This is the simplest method to signal the Tomcat server to shut down properly, allowing it to complete ongoing requests.
Sending a Shutdown Command through jmxproxy
Another approach is to connect via JMX (Java Management Extensions). This method offers a programmatic way to manage the server's lifecycle.
Example Code Snippet
import javax.management.MBeanServerConnection;
import javax.management.ObjectName;
import javax.management.remote.JMXConnector;
import javax.management.remote.JMXConnectorFactory;
import javax.management.remote.JMXServiceURL;
public class TomcatJMXShutdown {
public static void main(String[] args) {
try {
JMXServiceURL url = new JMXServiceURL("service:jmx:rmi:///jndi/rmi://localhost:9000/jmxrmi");
JMXConnector jmxConnector = JMXConnectorFactory.connect(url, null);
MBeanServerConnection mbsc = jmxConnector.getMBeanServerConnection();
ObjectName objectName = new ObjectName("Catalina:type=Server");
mbsc.invoke(objectName, "stop", null, null);
jmxConnector.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
Commentary on this Approach
By leveraging JMX, you can easily manage server behavior programmatically, integrating it into your applications or scripts. This is useful for automated deployments or monitoring systems.
Deploying a Custom Lifecycle Listener
If you need to implement custom shutdown behavior, creating a lifecycle listener could be beneficial. Here's a simple example:
Example Code Snippet
import org.apache.catalina.LifecycleEvent;
import org.apache.catalina.LifecycleListener;
import org.apache.catalina.LifecycleState;
public class CustomShutdownListener implements LifecycleListener {
@Override
public void lifecycleEvent(LifecycleEvent event) {
if (event.getType().equals("stop")) {
System.out.println("Custom shutdown logic here...");
// Release resources or perform cleanup actions
}
}
}
Explanation
The lifecycle listener allows you to hook into Tomcat's shutdown process. By implementing this interface, you can execute custom logic during the stopping phase, like closing connections or saving state.
Avoiding Common Pitfalls
When shutting down Tomcat, be wary of some common issues:
-
Timeouts: The shutdown process can hit a timeout if long-running requests are active. It's advisable to assess your servlet response times and configure the
connectionTimeout
attribute accordingly. -
Thread Leaks: If your application creates threads directly (e.g., using
new Thread()
), ensure that all threads are appropriately terminated during shutdown. Failing to do so can prevent the JVM from exiting gracefully. -
Internal Socket Connections: Ensure that any connections established with database servers or other resources are closed. This prevents leaking connections in resource pools.
Integrating Shutdown into DevOps
Automating your Tomcat shutdown processes as part of your DevOps pipeline is a recommended practice. Using tools like Jenkins, you can script the shutdown process to ensure it's included in your deployment and scaling routine.
Example Pipeline Step
The following pipeline snippet demonstrates a simple shutdown command:
pipeline {
agent any
stages {
stage('Shutdown') {
steps {
sh '''
cd /path/to/tomcat/bin
./shutdown.sh
'''
}
}
// Other pipeline stages...
}
}
By integrating these practices, you can ensure that your deployment is both seamless and reliable.
My Closing Thoughts on the Matter
Mastering Tomcat shutdown procedures is integral for Java web application management. By understanding the lifecycle, implementing graceful shutdowns, and taking necessary precautions, developers can prevent issues that arise from improper terminations. Embracing these best practices not only enhances server performance but also ensures a better user experience.
If you want to learn more about server management and Tomcat configurations, consider checking out Apache Tomcat Documentation and supplementary content on Java Management Extensions.
Implementing these strategies into your server management routine keeps your applications running smoothly and efficiently, avoiding the dreaded daemon dilemmas. Happy coding!
Checkout our other articles