Preventing Java Code Style Errors with New Line Operator Formatting
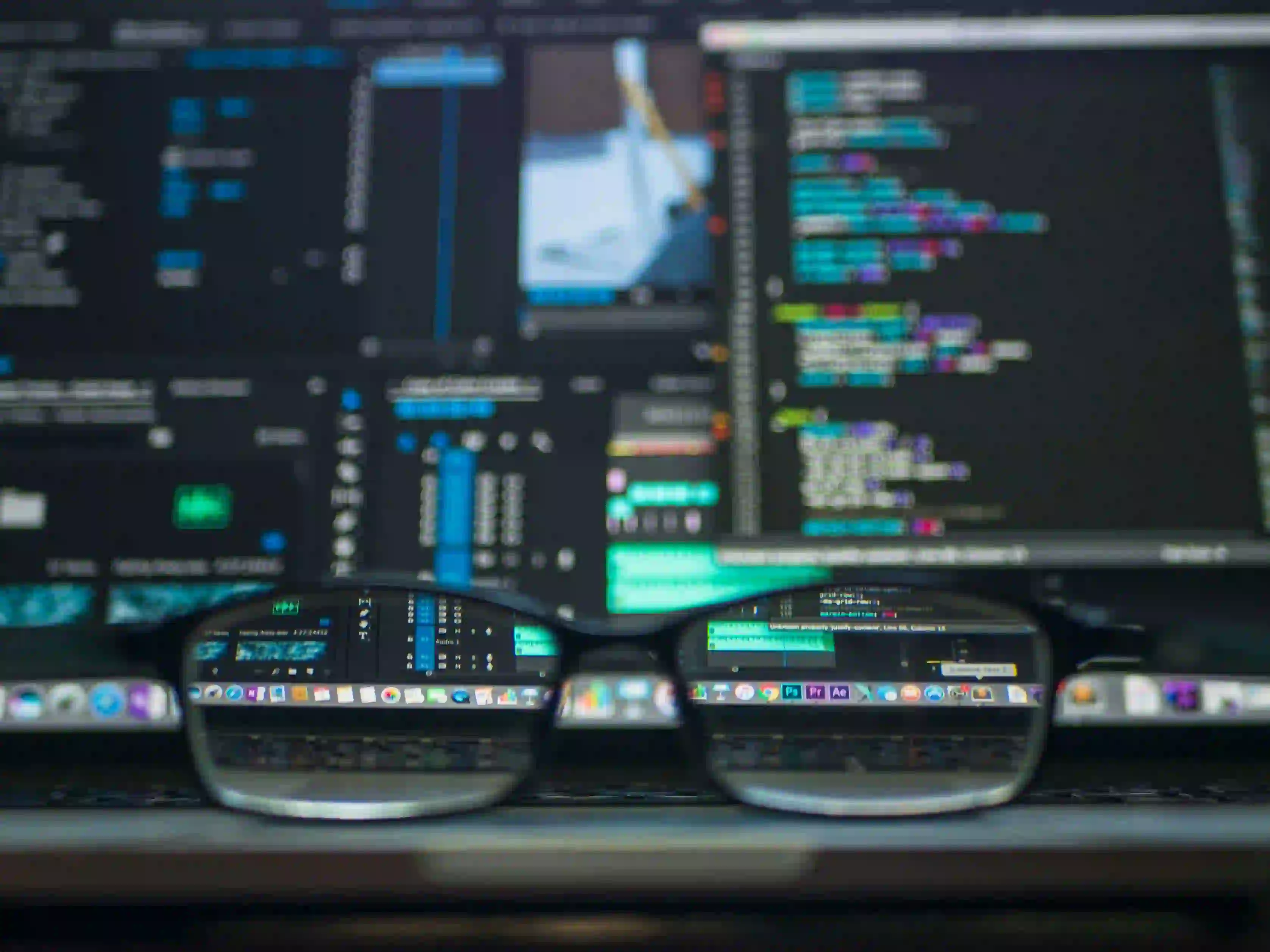
Preventing Java Code Style Errors with New Line Operator Formatting
Maintaining clean and readable code is a necessity in software development, especially in Java. Adopting consistent coding styles can make your code easier to read, maintain, and debug. One critical aspect often overlooked in Java development is the formatting of operators, particularly when it comes to new line handling.
In this blog post, we will explore how to prevent code style errors by utilizing new line operator formatting effectively. We will delve into the "why" behind it, provide some illustrative code snippets, and offer tips on maintaining a clean codebase.
Understanding Java Code Style
Java has a set of widely accepted conventions that help developers work collaboratively and understand each other's code. These conventions, such as the Java Coding Conventions, promote uniformity, allowing teams to spend less time on code review and more time on feature development.
When we talk about operator formatting—such as the placement of arithmetic or relational operators—it can greatly affect the readability of the code. It also aids in avoiding potential errors caused by misinterpreting the flow of control.
Importance of New Line Operator Formatting
The use of new line operators in Java code style is beneficial for several reasons:
- Improved Readability: Separating operators onto new lines makes the code easier to read and understand, allowing developers to recognize operations at a glance.
- Easier Debugging: When issues arise, it's quicker to identify the problem areas if the code follows a consistent structure.
- Enhanced Collaboration: If everyone on a team adheres to the same conventions, it becomes simpler for team members to work on each other's code without confusion.
Best Practices for New Line Operator Formatting
1. Placing Operators on New Lines
When using operators in Java, especially in complex expressions, it’s often beneficial to place operators on new lines. This makes logical operations clearer.
Example:
// Poorly formatted code
int total = a + b + c + d + e;
// Well-formatted code
int total = a
+ b
+ c
+ d
+ e;
In the second approach, placing each operator on a new line enhances visibility, making it easier for a reader to track the computation's flow.
2. Logical Conditions
When dealing with logical operators in conditional statements, it’s advisable to format them consistently.
Example:
// Poorly formatted code
if (a > b && b < c && c == d) {
// do something
}
// Well-formatted code
if (a > b
&& b < c
&& c == d) {
// do something
}
Writing logical conditions in this style can reduce mistakes and improve clarity, especially when extending or modifying the code in the future.
3. Method Chaining
When calling multiple methods in a chained manner, using new line operator formatting can help draw attention to the flow of calls.
Example:
String result = someObject.getString()
.trim()
.toUpperCase()
.substring(0, 5);
This formatting not only highlights each step but also helps in comprehending the chain of operations conducted on the object.
Using Tools for Consistent Formatting
To maintain and enforce consistent formatting across your codebase, consider using tools like:
-
Checkstyle: A development tool to help programmers write Java code that adheres to a coding standard.
-
SonarLint: An IDE extension that helps you detect and fix quality issues as you write code.
These tools can help identify and correct formatting issues automatically, ensuring adherence to your team’s chosen coding style.
Lessons Learned
In conclusion, maintaining a clean and consistent code style in Java—particularly in operator formatting—improves readability, reduces debugging time, and fosters collaboration among team members. Utilizing new line operator formatting is a simple yet effective practice that can significantly enhance the quality of your code.
As you work on your Java projects, consider implementing these practices and integrating tools that can maintain code quality. Simple changes today can lead to better coding habits and more efficient team collaboration tomorrow.
By preventing code style errors with thoughtful operator formatting, you set the foundation for a robust and maintainable codebase that stands the test of time.
Thank you for reading! If you found this post informative, please share it with fellow developers or comment with your thoughts below. Happy coding!