How to Tackle Latency Issues in JVM Memory with Java Streams
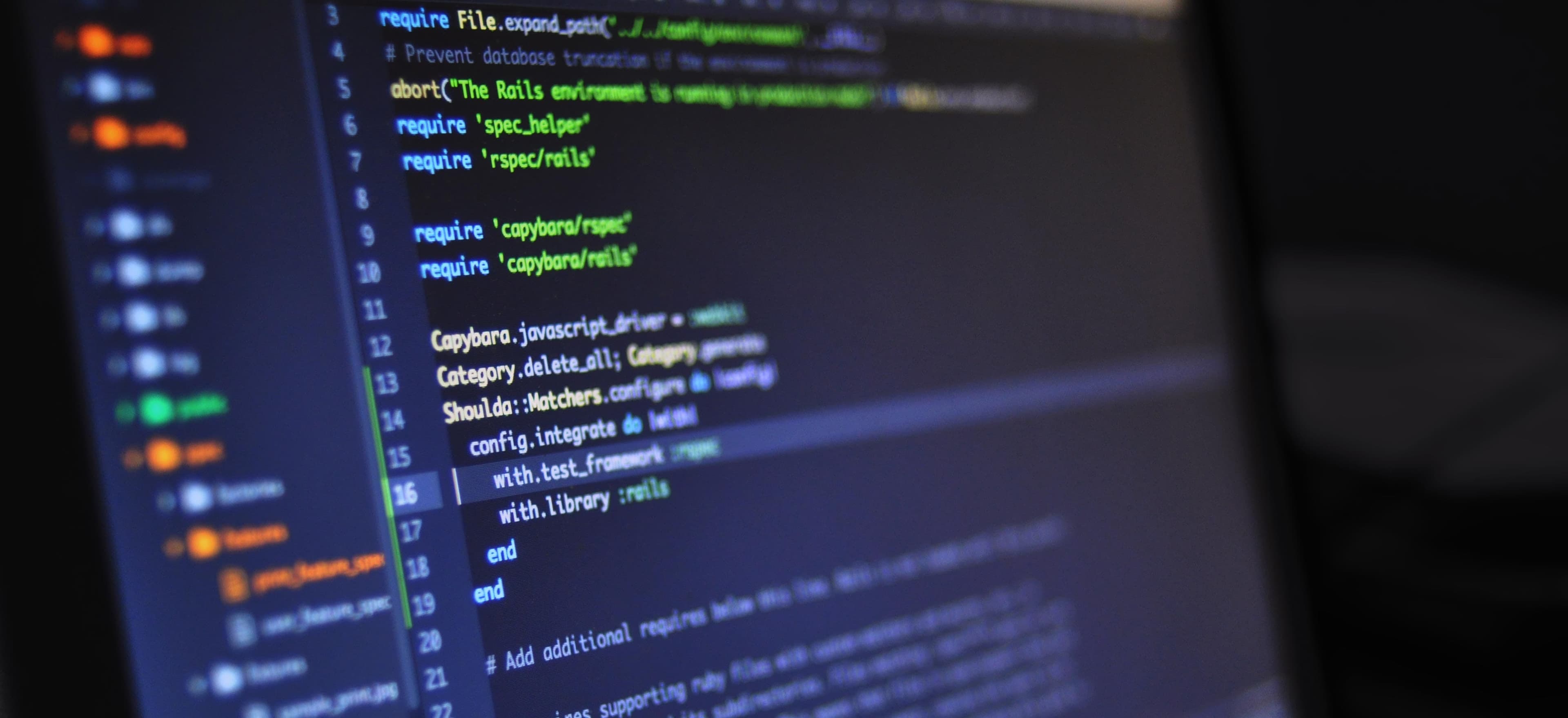
- Published on
How to Tackle Latency Issues in JVM Memory with Java Streams
Java Streams provide a powerful and expressive way to handle collections of data, but they can sometimes introduce latency issues, especially when working with large datasets or complex operations. In this post, we will explore strategies to tackle these latency issues, particularly focusing on memory consumption in the Java Virtual Machine (JVM).
Understanding Java Streams
Java Streams, introduced in Java 8, allow for functional-style operations on collections, making your code more readable and concise. Here’s a simple example of a stream that filters and collects integers from a list:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class StreamExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
List<Integer> evenNumbers = numbers.stream()
.filter(n -> n % 2 == 0)
.collect(Collectors.toList());
System.out.println("Even Numbers: " + evenNumbers);
}
}
In this example, the filter
method processes the data stream, selecting only even numbers. While streams offer a neat syntax, developers should be cautious about the potential latency implications associated with them.
Latency Issues in JVM Memory
Latency issues can arise from several factors within JVM memory when using streams:
- Memory Consumption: Streaming can lead to higher memory usage due to the accumulation of large intermediate results.
- Garbage Collection Pressure: If streams are generating numerous temporary objects, this can increase pressure on the garbage collector.
- Inefficient Pipeline: Stream operations, if not well-optimized, can lead to unnecessary computations.
Understanding these issues is the first step toward mitigation.
Strategies to Mitigate Latency Issues
1. Use Primitive Streams
Java Streams come in various types, with primitive streams (IntStream, LongStream, DoubleStream) being a great way to avoid boxing overhead, which can affect memory performance.
Here’s a quick example of using an IntStream
:
import java.util.stream.IntStream;
public class PrimitiveStreamExample {
public static void main(String[] args) {
int sum = IntStream.rangeClosed(1, 10)
.filter(n -> n % 2 == 0)
.sum();
System.out.println("Sum of Even Numbers: " + sum);
}
}
In this code, IntStream
avoids the overhead of boxing from int to Integer, resulting in better performance and lower memory usage.
2. Efficient Stream Pipelining
Ensure your streams are operating in an efficient manner. For example, using filter
before map
can reduce the number of computations performed.
Consider this inefficient example:
List<Integer> processedNumbers = numbers.stream()
.map(n -> n * n) // First maps all numbers
.filter(n -> n % 2 == 0) // Then filters
.collect(Collectors.toList());
Instead, rethink the order:
List<Integer> processedNumbers = numbers.stream()
.filter(n -> n % 2 == 0) // First filter
.map(n -> n * n) // Then map
.collect(Collectors.toList());
This change minimizes the work done on values that will be filtered out.
3. Limit Intermediate Results
Be mindful of intermediate operations that can generate large datasets in memory. Use short-circuiting operations wherever applicable. The findFirst()
operation is a great example:
Optional<Integer> firstEven = numbers.stream()
.filter(n -> n % 2 == 0)
.findFirst(); // Immediately stops further processing after finding the first even number
This approach ensures that we do not unnecessarily process the entire dataset when only one result is needed.
4. Adjust the Garbage Collector
If you are dealing with a high-frequency processing system, consider tuning your JVM garbage collector settings.
You can specify garbage collection options when launching your Java application. For example:
java -XX:+UseG1GC -XX:MaxGCPauseMillis=200 -jar YourApp.jar
The G1 garbage collector is designed to minimize latency, making it suitable for applications that require consistent response times.
5. Parallel Streams
For computationally heavy tasks, using parallel streams can be beneficial. However, use them judiciously, as they can also increase contention and lead to additional overhead if not managed properly.
Here’s how you can implement it:
List<Integer> evenNumbers = numbers.parallelStream()
.filter(n -> n % 2 == 0)
.collect(Collectors.toList());
System.out.println("Even Numbers (Parallel): " + evenNumbers);
Parallelism can significantly reduce processing time for large datasets, but remember to benchmark performance as the overhead may not always be justified.
6. Caching Results
For repeated calculations, consider caching results. Using an in-memory cache might save computation time and reduce latency in scenarios where data doesn't change frequently.
One way is to use Java's Map
interface:
import java.util.HashMap;
import java.util.Map;
public class CachingExample {
private static final Map<Integer, Integer> cache = new HashMap<>();
public static int expensiveComputation(int n) {
return cache.computeIfAbsent(n, key -> {
// Simulate an expensive operation.
return key * key;
});
}
public static void main(String[] args) {
System.out.println(expensiveComputation(5)); // Computes
System.out.println(expensiveComputation(5)); // Uses cache
}
}
Caching avoids repeated computations on the same inputs, thus improving overall performance.
The Closing Argument
Latency issues in JVM memory while working with Java Streams can be tackled effectively with the right approach. By implementing primitive streams, optimizing the order of operations, limiting intermediate results, adjusting garbage collector settings, employing parallel streams when appropriate, and caching results, developers can significantly enhance the performance of their applications.
These techniques won't just improve the speed of your applications; they will also lead to lower memory usage and more predictable garbage collection, helping you build robust Java applications.
For a deeper dive into Java Streams, you can refer to Oracle's Official Documentation and for more advanced JVM tuning techniques, check out The Java™ Tutorials.
By understanding the intricacies of Java Streams and JVM memory management, you can ensure your applications run efficiently and responsively, even as the complexity of data grows. Happy coding!
Checkout our other articles