Troubleshooting Okta Single Sign-On with Vert.x Servers
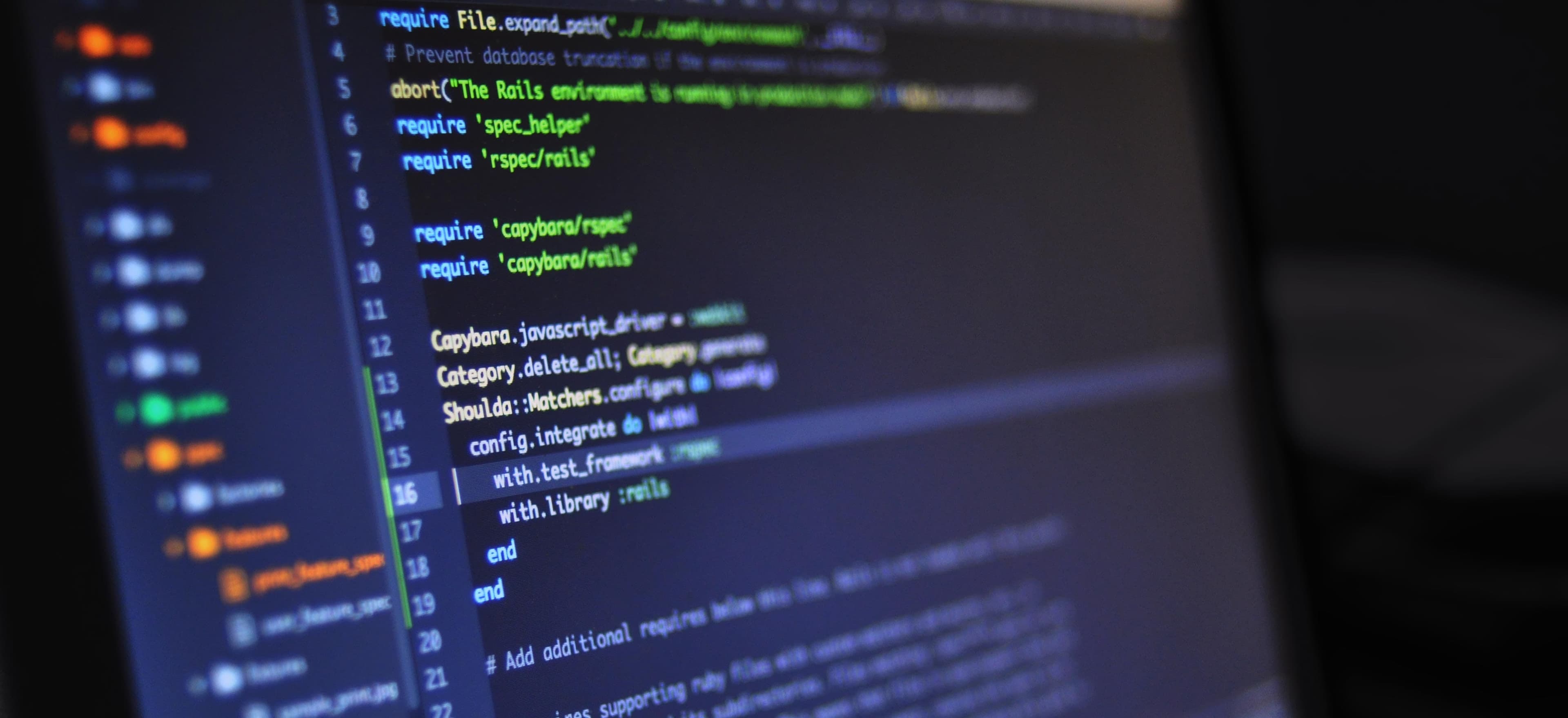
- Published on
Troubleshooting Okta Single Sign-On with Vert.x Servers
Implementing Single Sign-On (SSO) with Okta for your Vert.x application can streamline the user experience, making it simple and secure. However, when issues arise, troubleshooting becomes imperative. In this blog post, we’ll explore common problems, solutions, and best practices for integrating Okta SSO with Vert.x servers.
Understanding Okta SSO and Vert.x
What is Okta?
Okta is a cloud identity management service. It provides SSO, allowing users to access multiple applications with one set of credentials, enhancing both security and user experience.
What is Vert.x?
Vert.x is a toolkit for building reactive applications on the Java Virtual Machine (JVM). It simplifies the development of scalable web applications. Its asynchronous nature makes it an excellent choice for applications requiring high performance under heavy loads.
Setting Up Okta SSO with Vert.x
Before we get into troubleshooting, it's essential to understand how Okta SSO integrates with a Vert.x server.
- Create an Okta Application: Visit the Okta Developer Console and set up a new OIDC application.
- Configure Application Settings: Specify callback URLs, sign-in redirect URIs, and any necessary scopes.
- Add Vert.x Dependencies: Include relevant libraries in your
pom.xml
for a Maven project. This might include needed dependencies for OAuth 2.0 and OpenID Connect.
Example Dependencies in pom.xml
<dependency>
<groupId>io.vertx</groupId>
<artifactId>vertx-web</artifactId>
<version>4.4.1</version>
</dependency>
<dependency>
<groupId>com.okta.sdk</groupId>
<artifactId>okta-spring-boot-starter</artifactId>
<version>3.0.0</version>
</dependency>
This setup will enable your Vert.x application to perform OAuth 2.0 authentication with Okta.
Common Issues and Troubleshooting Steps
1. Callback URL Issues
Problem: The application does not redirect back to the correct URL after authentication.
Solution: Verify that the callback URL registered in the Okta application matches exactly with the URL your application is using. This includes checking for trailing slashes and HTTP vs. HTTPS discrepancies.
2. Unauthorized Client
Problem: You receive an error stating "Unauthorized Client" when attempting to authenticate.
Solution: This typically means your client ID and client secret may be incorrect. Double-check your application's configuration against the Okta settings. Ensure that you've configured your client application as allowed to use the implicit or authorization code flow based on your needs.
Example Code: Configuring Vert.x for SSO
Here’s a basic example of how to configure your Vert.x application for Okta SSO.
Router router = Router.router(vertx);
router.route("/login").handler(context -> {
String redirectUri = "http://yourapp/callback";
String clientId = "yourClientId";
String clientSecret = "yourClientSecret";
String authorizationUrl = "https://yourOktaDomain/oauth2/default/v1/authorize?" +
"client_id=" + clientId + "&" +
"redirect_uri=" + redirectUri + "&" +
"response_type=code&" +
"scope=openid";
context.response().redirect(authorizationUrl);
});
Why: This sets up the login route to redirect users to Okta for authentication. Properly setting the redirect URI and your client credentials are essential steps in this flow.
3. Token Expiry Issues
Problem: Users frequently get logged out due to token expiry.
Solution: Adjust the token lifetime settings in the Okta Admin dashboard. Additionally, implement token refresh logic in your Vert.x application to ensure users remain logged in as long as possible.
4. CORS Issues
Problem: When making API calls after login, you may encounter Cross-Origin Resource Sharing (CORS) issues.
Solution: Ensure your Vert.x server is configured to enable CORS. You can do this by setting appropriate headers in your response.
Example Code: Configuring CORS in Vert.x
router.route().handler(CorsHandler.create("*")
.allowedMethods(Arrays.asList(HttpMethod.GET, HttpMethod.POST, HttpMethod.PUT, HttpMethod.DELETE))
.allowedHeaders(Arrays.asList("Authorization", "Content-Type"))
);
Why: This CORS configuration allows your Vert.x application to accept requests from different origins, which is crucial when developing SPAs that interact with the backend services.
5. User Profile Retrieval Issues
Problem: You are unable to fetch user details after authentication.
Solution: Ensure that the correct scopes are requested during the authorization flow. The profile information can typically be retrieved using access tokens.
Example Code: Fetching User Profile
String userInfoUri = "https://yourOktaDomain/oauth2/default/v1/userinfo";
HttpClient client = HttpClient.create(vertx);
client.getAbs(userInfoUri)
.putHeader("Authorization", "Bearer " + accessToken)
.send(ar -> {
if (ar.succeeded()) {
// Handle the successful response
JsonObject userInfo = ar.result().bodyAsJsonObject();
} else {
// Handle error
}
});
Why: This code snippet demonstrates how to leverage the access token to request user information from Okta's userinfo endpoint. Proper token management helps successfully authorize API requests.
6. Logging and Error Handling
Problem: Lack of visibility into errors occurring during authentication.
Solution: Implement robust logging around the authentication process. Use Vert.x’s built-in logging capabilities, such as Logger
or integrating with popular logging libraries like SLF4J.
Best Practices
- Use HTTPS: Always use HTTPS for callback URIs and API requests to prevent man-in-the-middle attacks.
- Maintain Token Security: Store tokens securely and never expose them directly in the frontend code.
- Keep Your Libraries Updated: Regular updates to Vert.x and Okta libraries ensure you benefit from the latest security updates and features.
- Test Thoroughly: Conduct thorough testing for different flows, including logout and expired sessions.
By following these troubleshooting steps and best practices, integrating Okta Single Sign-On into your Vert.x application will become a seamless experience.
Key Takeaways
While integrating Okta Single Sign-On with Vert.x can lead to improved user experience and security, it is not without its challenges. By understanding common issues and applying effective troubleshooting techniques, you will not only resolve problems more quickly but also strengthen your application's architecture.
For more detailed documentation and further resources, consider visiting the following links:
Adopting a methodical approach to troubleshooting and configuration will ensure that your application harnesses the strengths of both Okta and Vert.x effectively. Get started today, and enjoy the benefits of streamlined authentication!
Checkout our other articles