Common Java Design Pattern Pitfalls to Avoid
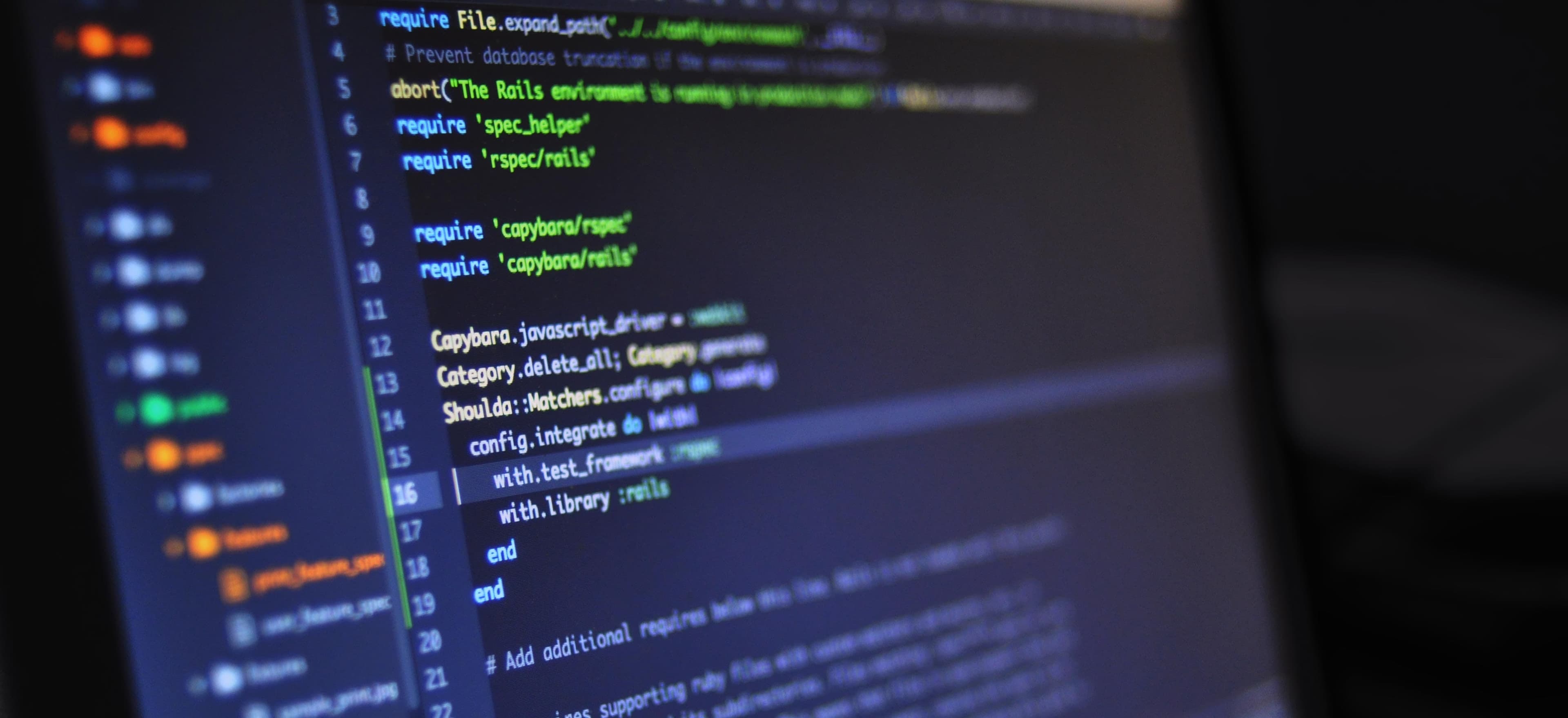
- Published on
Common Java Design Pattern Pitfalls to Avoid
Design patterns are essential tools in a developer’s toolkit, providing tested and proven solutions to common problems. However, misuse or misunderstanding of these patterns can lead to bigger issues down the road. In this blog post, we'll delve into common pitfalls associated with Java design patterns and how to avoid them.
Understanding Design Patterns
Before we jump into the pitfalls, it's critical to understand what design patterns are. In Java, design patterns are standard terminology used in software design to solve common design problems and optimize code. These include creational, structural, and behavioral patterns.
- Creational Patterns: Focus on object creation mechanisms.
- Structural Patterns: Deal with object composition to form larger structures.
- Behavioral Patterns: Concerned with the interaction and responsibility among objects.
Common Pitfalls
1. Overusing Design Patterns
The Pitfall: Many developers are tempted to use design patterns for every problem, leading to over-engineering.
Example: Implementing the Singleton pattern where simple static fields would suffice can complicate the code unnecessarily.
Why to Avoid: Overcomplicating your code leads to maintainability issues and readability struggles. You may find yourself spending more effort managing your patterns than the issues they were intended to solve.
Recommendation: Apply design patterns judiciously. Always assess whether the benefits of a pattern outweigh its complexities before implementing it.
2. Misunderstanding the Purpose of Patterns
The Pitfall: Using a design pattern without fully understanding its intent can lead to incorrect implementations.
Example: The Observer pattern is often used incorrectly. Developers might create an Observer that notifies all subscribers every time it updates, disregarding the need for efficient and controlled notifications.
class Subject {
private List<Observer> observers = new ArrayList<>();
public void attach(Observer observer) {
observers.add(observer);
}
public void notifyObservers() {
for (Observer observer : observers) {
observer.update(this);
}
}
}
Why: Failing to grasp the true purpose of a pattern can lead to misuse, affecting code performance or functionality.
Recommendation: Take time to study the nuances of a design pattern before using it. The Gang of Four book is an excellent resource for deeper insights.
3. Not Following the Pattern's Structure
The Pitfall: Implementing patterns while skipping structural elements leads to functionality loss.
Example: In the Factory pattern, without the Factory method, you're likely to end up with a regular class instantiation rather than leveraging flexibility.
interface Product {
void use();
}
class ConcreteProductA implements Product {
public void use() { System.out.println("Using Product A"); }
}
class Factory {
public Product createProduct(String type) {
if ("A".equals(type)) {
return new ConcreteProductA();
}
return null; // or throw an exception
}
}
Why: Not adhering to established structures diminishes the advantages design patterns provide—like loose coupling and enhanced scalability.
Recommendation: Stick closely to the patterns' core structures during implementation. Understanding variations and alternatives is beneficial, but don’t stray too far from the established best practices.
4. Ignoring The Context
The Pitfall: A common mistake is applying patterns without considering the application's context, leading to ineffective solutions.
Example: Using the Command pattern for basic operations may complicate a simple CRUD application unnecessarily, where basic method calls would suffice.
Why: Patterns are designed for specific scenarios; ignoring the context can create unnecessary complications and reduce performance.
Recommendation: Evaluate the requirements of your application carefully to determine if a design pattern is truly necessary. Avoid the "pattern of the week" approach.
5. Inadequate Testing
The Pitfall: Relying on design patterns can create a false sense of security, leading to inadequate testing.
Example: If a developer assumes the Singleton pattern inherently prevents multiple instances and skips testing this assumption, critical bugs may arise when the application scales.
Why: Patterns don’t guarantee correctness or performance; they must still be validated through rigorous testing.
Recommendation: Implement thorough testing (unit tests, integration tests) even after adopting design patterns. Patterns can suggest a design but do not assure its correctness in your specific implementation.
6. Neglecting Documentation
The Pitfall: Failing to document the use of design patterns, especially in large codebases, creates confusion for future developers.
Why: Without proper documentation, the reasoning behind using specific design patterns is lost, making maintenance challenging, particularly for newcomers.
Recommendation: Document your design choices and the context in which you employed specific patterns. This habit pays off in collaborative environments or when maintaining your code later.
7. Premature Optimization
The Pitfall: Sometimes, developers implement complex design patterns for supposed performance benefits before identifying performance bottlenecks.
Why: This leads to unnecessary complexity without real gains, making the application harder to maintain and understand.
Recommendation: Apply design patterns based on the need and after profiling the application. Focus on clear implementations that simplify the code.
Wrapping Up
Java design patterns are powerful tools for crafting maintainable and scalable applications, but they come with their set of pitfalls.
Here are some takeaways:
- Use design patterns judiciously.
- Understand the purpose behind each pattern.
- Adhere to structure and context.
- Don't forget the importance of testing.
- Document your design choices for clarity.
- Avoid premature optimization.
By steering clear of these common pitfalls, you can make the most of design patterns while maintaining the integrity and readability of your code.
If you want to learn more about Java design patterns, please check out Refactoring Guru's Design Patterns for additional resources.
Happy coding!
Checkout our other articles