Common Pitfalls in Microservices: Best Practices to Avoid
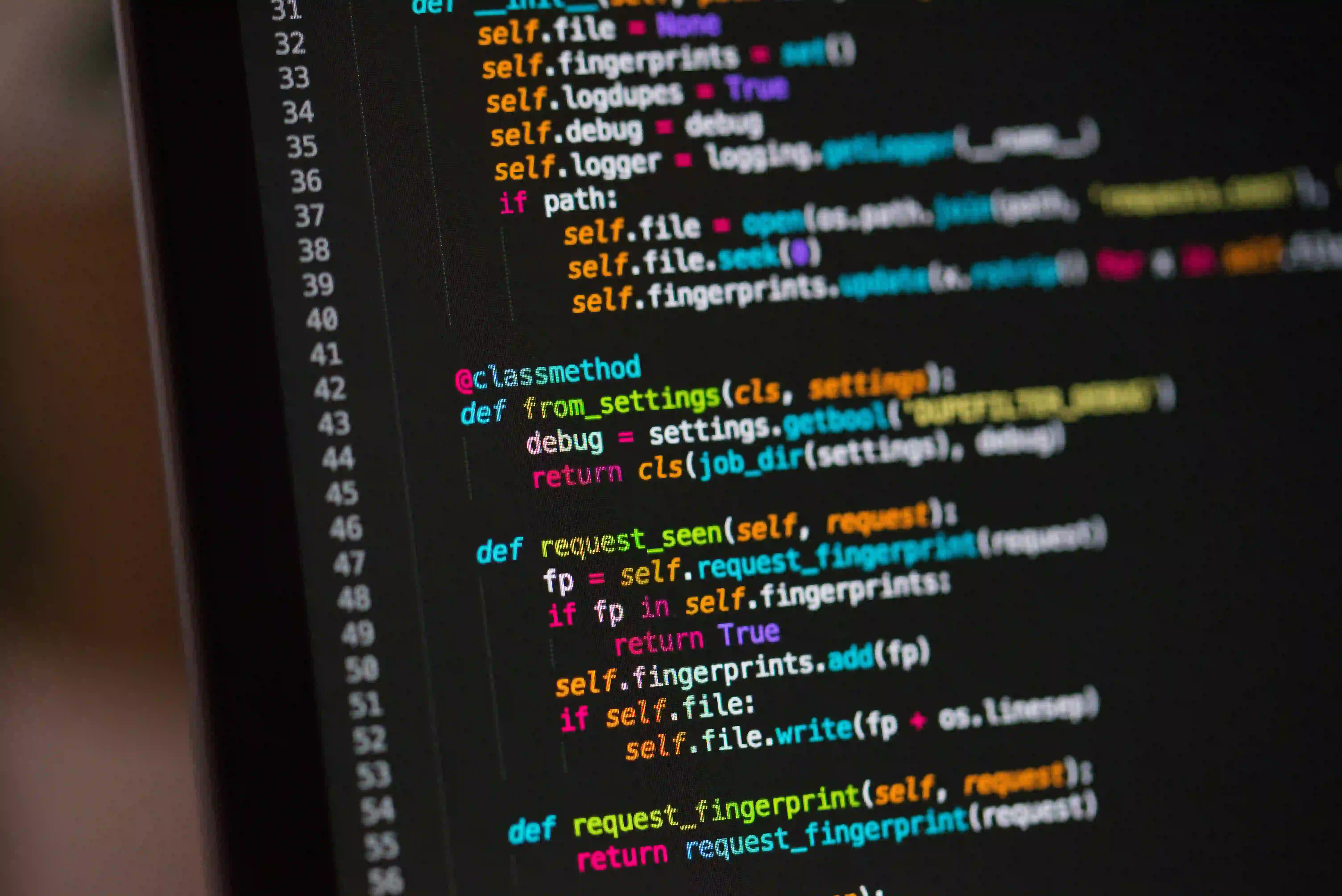
Common Pitfalls in Microservices: Best Practices to Avoid
Microservices architecture has gained immense popularity in recent years due to its promise of scalability, flexibility, and ease of deployment. However, transitioning from monolithic applications to microservices can be fraught with challenges. In this post, we'll explore common pitfalls associated with microservices and discuss best practices to avoid them.
Table of Contents
- What are Microservices?
- Common Pitfalls
- 2.1 Over-Engineering
- 2.2 Inefficient Communication
- 2.3 Data Management Challenges
- 2.4 Handling Failures
- Best Practices to Avoid Pitfalls
- 3.1 Emphasize Domain-Driven Design
- 3.2 Optimize API Communication
- 3.3 Adopt a Distributed Data Management Strategy
- 3.4 Implement Robust Monitoring and Logging
- Conclusion
What are Microservices?
Microservices architecture breaks down a large application into smaller, independently deployable services. Each service is built around a specific business function and communicates with others through well-defined APIs, typically over HTTP. This architectural pattern can enhance development speed, improve scalability, and allow teams to work autonomously.
Common Pitfalls
While microservices offer several advantages, they can also introduce complexities. Below, we explore some of the common pitfalls developers encounter while implementing a microservices architecture.
2.1 Over-Engineering
One of the most prevalent pitfalls is the temptation to over-engineer microservices. Teams may be eager to utilize the latest technologies and design patterns, resulting in overly complex services. Instead of focusing on simplicity and delivering business value, they invest time in unnecessary features.
Avoiding Over-Engineering
- Focus on User Needs: Determine what features users require. Start with simple services and gradually enhance them as needed.
- Maintain Code Simplicity: Strive for clean and understandable code. Simple code is easier to maintain and debug.
2.2 Inefficient Communication
Microservices often rely on communication over the network. If not managed efficiently, inter-service communication can lead to latency and potential points of failure.
Communication Strategies
- Synchronous vs. Asynchronous: Understand when to use synchronous calls and when to use asynchronous communication. For example, user notifications might be handled better asynchronously.
- Consider Network Overhead: Minimize the number of calls to other services. Batch requests where possible to reduce communication overhead.
2.3 Data Management Challenges
In a microservices architecture, each service typically has its own database. This can lead to data management challenges, including consistency and orchestration.
Data Management Solutions
- Consistency Models: Depending on your application needs, choose between eventual consistency and strong consistency models.
- Distributed Transactions: Evaluate whether to implement a distributed transaction manager or use compensating transactions when needed.
2.4 Handling Failures
Failures in one microservice can impact others. Failure management is vital to prevent cascading failures that could compromise the entire system.
Resilience and Recovery
- Circuit Breaker Pattern: Implement the circuit breaker pattern to manage failures effectively. If a service fails consistently, allow the system to enter a 'tripped' state instead of continuously trying to call that service.
Here is a simplified example of how you might implement a circuit breaker pattern in Java:
public class CircuitBreaker {
private boolean open = false;
private int failureCount = 0;
private final int failureThreshold = 3;
public void callService() {
if (open) {
System.out.println("Circuit is open. Rejecting call.");
return;
}
try {
// Attempt to call an external service
externalServiceCall();
failureCount = 0; // Reset failure count upon success
} catch (Exception e) {
failureCount++;
if (failureCount >= failureThreshold) {
open = true; // Open circuit if threshold exceeded
System.out.println("Circuit opened due to failures.");
}
System.out.println("Service call failed.");
}
}
private void externalServiceCall() {
// Simulate a service call
throw new RuntimeException("Service failure.");
}
}
In this code, the CircuitBreaker
class tracks service failures. It opens the circuit after a specified threshold of failures, preventing further calls to the service until it has had a chance to recover.
Best Practices to Avoid Pitfalls
While pitfalls are common, there are established best practices to help navigate them effectively.
3.1 Emphasize Domain-Driven Design
Domain-Driven Design (DDD) can provide clarity when defining the boundaries of microservices. Each service should represent a specific business domain, promoting cohesion and independence.
- Bounded Contexts: Identify bounded contexts for your services to minimize overlap and ensure clear responsibilities.
- Collaborate Across Teams: Encourage collaboration between business stakeholders and development teams to ensure alignment on business needs.
3.2 Optimize API Communication
API design is crucial in microservices. Well-designed APIs can facilitate efficient communication between services.
- RESTful APIs: Use RESTful principles to create stateless, cacheable API endpoints that enhance performance.
- Documentation: Maintain thorough documentation for your APIs to simplify integration for different teams.
3.3 Adopt a Distributed Data Management Strategy
A sound data management strategy is critical for maintaining data integrity across microservices.
- Database Per Service: Follow the principle of each service managing its own database. This ensures that services can evolve independently.
- Event Sourcing: Consider adopting event sourcing to maintain a reliable history of changes and events for data synchronization.
3.4 Implement Robust Monitoring and Logging
Effective monitoring and logging are essential for identifying and troubleshooting issues in a microservices ecosystem.
- Centralized Logging: Use a centralized logging framework like ELK stack (Elasticsearch, Logstash, and Kibana) to collect and analyze logs from all microservices.
- Performance Metrics: Track performance metrics to identify bottlenecks within the architecture. Tools like Prometheus and Grafana can help visualize data trends.
The Last Word
Navigating the transition to a microservices architecture is filled with complexities. However, being aware of common pitfalls and applying best practices can lead to successful microservices implementation. Remember to focus on simplicity, optimize service communication, manage data strategically, and implement robust monitoring. With these strategies, you'll be well on your way to a resilient and scalable microservices architecture.
For more information on microservices and architectural patterns, check out the Microservices.io website by Chris Richardson. Happy coding!
By adhering to these best practices and avoiding common pitfalls, you will set your teams up for success in the microservices landscape. As microservices continue to evolve, stay adaptable and prepared for ongoing learning in this exciting domain.