Navigating Fetch Joins in JPA: Avoiding Common Pitfalls
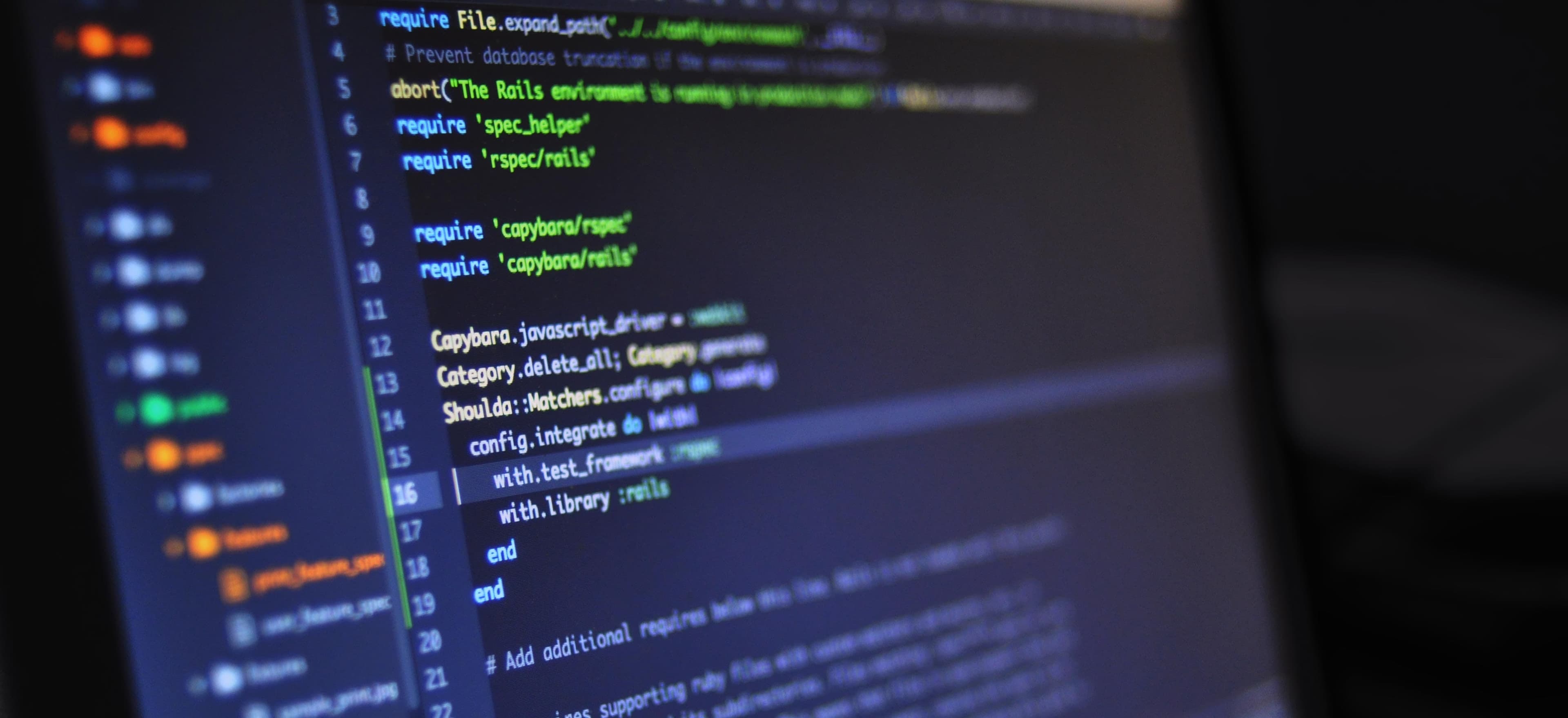
- Published on
Navigating Fetch Joins in JPA: Avoiding Common Pitfalls
Java Persistence API (JPA) is a powerful tool that provides a simplified way to handle relational data in Java applications. However, one of the most intricate areas of JPA is the concept of fetch joins. Fetch joins allow us to retrieve data from associated entities in a single query rather than executing multiple queries. While this feature can significantly enhance performance, it also comes with its own set of challenges.
In this blog post, we'll delve into fetch joins in JPA, exploring the benefits, possible pitfalls, and how to utilize them effectively to ensure optimal performance. This guide is tailored for both beginners and experienced developers seeking to refine their skills.
Understanding Fetch Joins
What are Fetch Joins?
Fetch joins are used in JPA queries to fetch associated entities eagerly. They allow JPA developers to retrieve a primary entity and its related entities in one go, reducing the overhead of multiple queries.
For instance, consider a simple example where we have two entities: Author
and Book
. An Author
can write multiple Books
, and we might want to retrieve an Author
along with their Book
list.
Basic Example
SELECT a FROM Author a JOIN FETCH a.books
In this query, we fetch the Author
and their corresponding Books
in one database call. The primary objective is to avoid the n+1 select problem, where fetching related entities requires multiple database queries.
Benefits of Fetch Joins
- Performance Improvement: A single query reduces the number of database calls.
- Simplifies Object Retrieval: Results are returned with relationships already established, making it easier to navigate the object graph.
- Data Integrity: Fetching related data ensures you don’t receive stale or incomplete data.
Common Pitfalls with Fetch Joins
Despite their advantages, fetch joins can lead to several common pitfalls. Understanding these issues will help you avoid them.
1. Cartesian Product Issues
One of the most common issues with fetch joins is the creation of a Cartesian product. This happens when the combination of multiple entities in a join results in a multiplication of rows in the result set.
Example
SELECT a FROM Author a JOIN FETCH a.books b LEFT JOIN FETCH b.reviews
In the above query, if an Author
has multiple Books
and each Book
has multiple Reviews
, the result set will have a row for every combination of Book
and Review
. This bloats the result set and can lead to performance issues.
Best Practice
- Limit the number of fetch joins in a single query. If more data is required, consider using multiple queries or batch fetching.
2. Unanticipated Memory Footprint
While fetch joins reduce the number of queries, they may increase the memory footprint. If an entity has several associations that are not needed for a particular operation, using fetch joins may unintentionally load a significant amount of data into memory.
Example Code
List<Author> authors = entityManager.createQuery("SELECT a FROM Author a JOIN FETCH a.books", Author.class).getResultList();
If an Author
has a huge number of Books
, this approach can lead to memory exhaustion.
Best Practice
- Use lazy loading where possible, and be judicious about when to use fetch joins. Always assess whether you need all associated data.
3. Transaction Management and Lazy Loading Issues
JPA's lazy loading behavior can cause issues when combined with fetch joins. If a fetch join is used in a transaction and subsequently accessed outside of that transaction, it may lead to LazyInitializationException
.
Example Code
@Transactional
public void fetchAuthors() {
List<Author> authors = entityManager.createQuery("SELECT a FROM Author a JOIN FETCH a.books", Author.class).getResultList();
// transaction may close here
authors.forEach(author -> author.getBooks().size()); // This may throw LazyInitializationException
}
Best Practice
- Always ensure that the transaction context is active when accessing fetched data or change your fetching strategy to avoid lazy loading.
4. Complexity in Queries
As more entities are joined using fetch joins, the query can become complex and harder to maintain. This makes it challenging to understand how changes in one part of the query affect performance.
Solution
- Break down complex queries into simpler ones and use method-level annotations to apply the necessary fetch strategy.
5. Debugging Difficulties
Debugging fetch join issues can be complicated. It’s not always clear where the problem lies, whether it's the query itself or how the data is being used after retrieval.
Best Practice
- Log SQL queries to the console during development to observe the generated SQL and help with debugging. You can do this by configuring your persistence.xml.
<property name="hibernate.show_sql" value="true"/>
<property name="hibernate.format_sql" value="true"/>
The Closing Argument
Fetch joins are a powerful feature of JPA that can greatly enhance performance by reducing the number of queries executed against your database. However, as we've explored, they come with their own set of challenges. By understanding these pitfalls and applying best practices, you can leverage fetch joins effectively while maintaining optimal performance and memory management.
For more detailed information on JPA and its various features, consider visiting the official Hibernate documentation.
As you continue to explore JPA, keep experimenting with fetching strategies and query designs. With practice, you will gain confidence in navigating fetch joins, enhancing your applications' efficiency and performance. Happy coding!
Checkout our other articles