Mastering User Input in IntelliJ Plugin Development
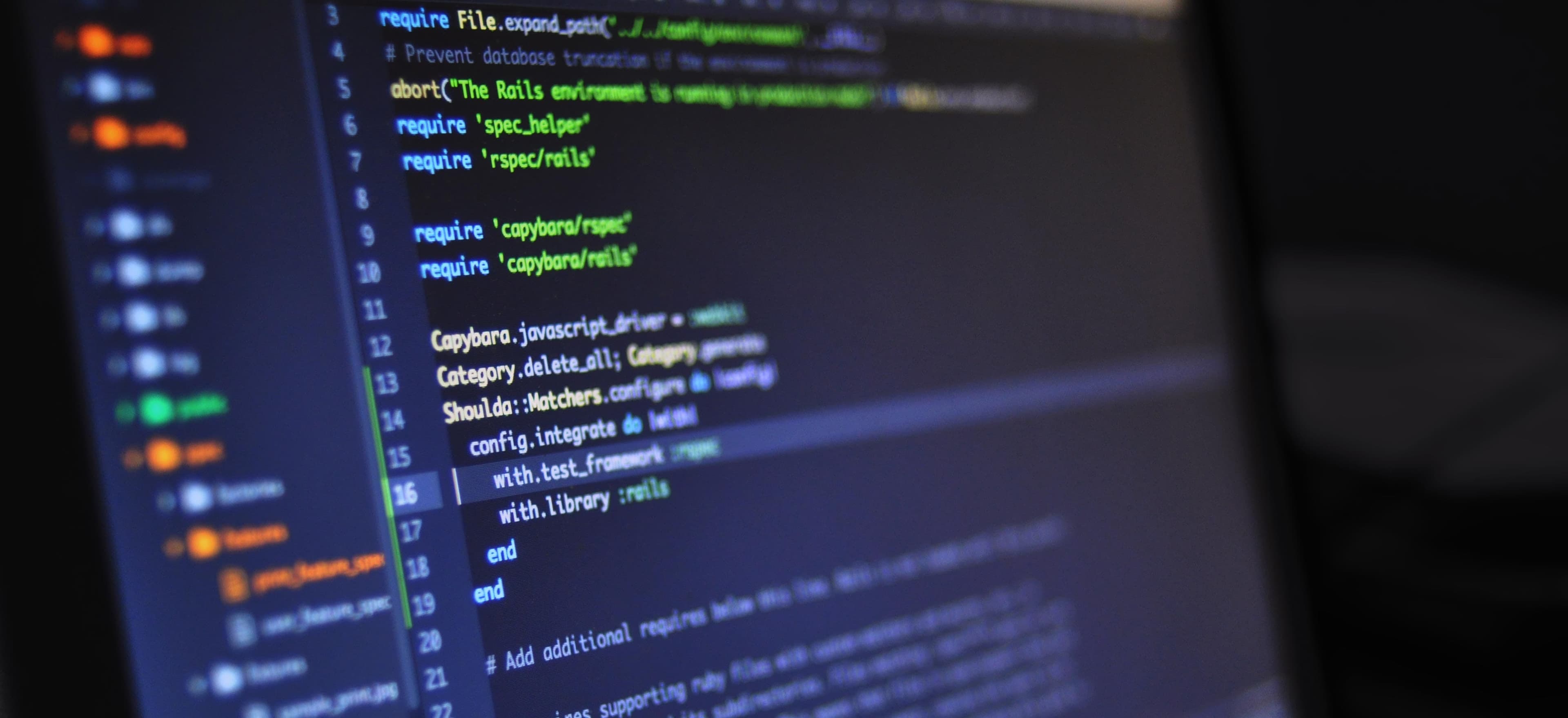
- Published on
Mastering User Input in IntelliJ Plugin Development
Developing plugins for IntelliJ IDEA is an exciting venture that allows developers to extend the capabilities of one of the most popular Integrated Development Environments (IDEs). A key feature of any plugin is its ability to interact with users effectively. User input is critical because it determines how the plugin behaves and responds. In this blog post, we will explore various methods to handle user input in IntelliJ plugin development, providing examples and explanations along the way.
Understanding the Plugin Environment
Before diving into user input mechanisms, it's essential to understand the IntelliJ plugin architecture. IntelliJ plugins are mostly developed in Java or Kotlin. They leverage the IntelliJ Platform SDK, which includes various APIs for creating user interfaces, managing project files, and more.
For more detailed documentation on the IntelliJ Platform SDK, visit the IntelliJ Platform SDK DevGuide.
Common User Input Scenarios
User input can take many forms in a plugin:
- Text input via dialog boxes
- File selection from the file system
- Choosing options from dropdown menus or lists
- Configuration settings through a settings panel
Handling these inputs correctly creates a better user experience. Let's start with some of the most common methods for collecting user input.
Creating Input Dialogs
The simplest way to gather user input is through modal dialog boxes. The Messages
class provides a straightforward way to display these to users.
Here's a basic example of creating a simple input dialog.
Example: Simple Input Dialog
import com.intellij.openapi.ui.Messages;
public void showInputDialog() {
String input = Messages.showInputDialog(
"Please enter your name:",
"Input Required",
Messages.getInformationIcon()
);
if (input != null) {
// Process the input
System.out.println("User input: " + input);
} else {
System.out.println("User cancelled the input.");
}
}
Code Commentary:
- The
showInputDialog
method presents a dialog with an input field. It has a title and an icon for clarity. - The method returns the user input as a String. If the dialog is canceled, it returns null.
- It's a simple way for the user to provide inputs like names, identifiers, or short texts.
Use Cases
Using simple input dialogs is best for small, localized inputs where user friction should be minimal—like gathering a user’s name, confirming an action, or asking for a quick comment.
Building Complex Input Forms
While simple input dialogs serve well for quick inputs, complex plugins often require more structured ways to gather user information. This is where custom dialogs come into play.
Example: Custom Dialog with Form Fields
import com.intellij.openapi.ui.DialogWrapper;
import org.jetbrains.annotations.Nullable;
import javax.swing.*;
import java.awt.*;
public class CustomInputDialog extends DialogWrapper {
private JTextField nameField;
private JTextField emailField;
protected CustomInputDialog() {
super(true); // use current window as parent
setTitle("Custom Input Dialog");
init();
}
@Nullable
@Override
protected JComponent createCenterPanel() {
JPanel panel = new JPanel(new GridLayout(2, 2));
panel.add(new JLabel("Name:"));
nameField = new JTextField();
panel.add(nameField);
panel.add(new JLabel("Email:"));
emailField = new JTextField();
panel.add(emailField);
return panel;
}
public String getName() {
return nameField.getText();
}
public String getEmail() {
return emailField.getText();
}
public static void showDialog() {
CustomInputDialog dialog = new CustomInputDialog();
dialog.show();
if (dialog.isOK()) {
System.out.println("User Name: " + dialog.getName());
System.out.println("User Email: " + dialog.getEmail());
}
}
}
Code Commentary:
- The
CustomInputDialog
extendsDialogWrapper
, which gives you full control over the components and behavior. - The
createCenterPanel
method constructs the layout for our inputs. - The
getName
andgetEmail
methods retrieve user inputs after the dialog is confirmed. - When invoking
showDialog
, the plugin captures the user inputs elegantly.
Use Cases
Custom dialogs are appropriate when several inputs are necessary or when structuring data is critical, such as gathering user profiles or settings that are pivotal to the plugin's functionality.
Listening to User Selections
Another method to handle user input in IntelliJ plugins involves listening to selections from UI components like combos or lists.
Example: ComboBox Selection Listener
import javax.swing.*;
import java.awt.event.ActionEvent;
public class ComboBoxExample {
private JComboBox<String> comboBox;
public ComboBoxExample() {
comboBox = new JComboBox<>(new String[]{"Option 1", "Option 2", "Option 3"});
comboBox.addActionListener(this::onComboBoxSelection);
}
private void onComboBoxSelection(ActionEvent e) {
String selectedOption = (String) comboBox.getSelectedItem();
System.out.println("Selected: " + selectedOption);
}
public JComboBox<String> getComboBox() {
return comboBox;
}
}
Code Commentary:
- This code creates a
JComboBox
where users can select options. - The
ActionListener
captures the selection event and allows you to react accordingly. - You can easily extend this by combining complex data structures to populate the combo box.
Use Cases
Comboboxes and list selection are excellent for scenarios where user choices matter and provide a limited set of predefined options, making it easier for users to select from them.
File Chooser for File Inputs
Sometimes, users need to select files, such as when configuring a plugin that integrates with other tools. The FileChooser
class provides an easy way to implement file selection dialogs in your plugins.
Example: Simple File Chooser
import com.intellij.openapi.fileChooser.FileChooser;
import com.intellij.openapi.fileChooser.FileChooserDescriptor;
import com.intellij.openapi.vfs.VirtualFile;
import com.intellij.openapi.project.Project;
public void openFileChooser(Project project) {
FileChooserDescriptor descriptor = new FileChooserDescriptor(true, false, false, false, false, false);
VirtualFile selectedFile = FileChooser.chooseFile(descriptor, project, null);
if (selectedFile != null) {
System.out.println("Selected file: " + selectedFile.getPath());
} else {
System.out.println("File selection was canceled.");
}
}
Code Commentary:
FileChooserDescriptor
configures what files can be chosen (e.g., folders, files etc.).- The method
chooseFile
opens a dialog and lets the user select a file. - Handling the selected file is straightforward, allowing for easy integration into the plugin’s workflow.
Use Cases
Use file choosers when your plugin interacts with external files, such as configuration files, logs, or data sources.
To Wrap Things Up
In this blog post, we've delved into several ways to master user input in IntelliJ plugin development. From simple input dialogs to custom forms and file choosers, each method plays a critical role in enhancing user interaction.
By implementing these techniques effectively, you can ensure that your plugin is both user-friendly and functional. There’s much more to learn and explore, so I encourage you to check out more information at the IntelliJ Platform SDK documentation and keep experimenting with different user input methods.
Remember, the better the input handling in your plugin, the better the overall user experience. Happy coding!
Checkout our other articles