The Pitfalls of Java's Verbosity: Is it Worth It?
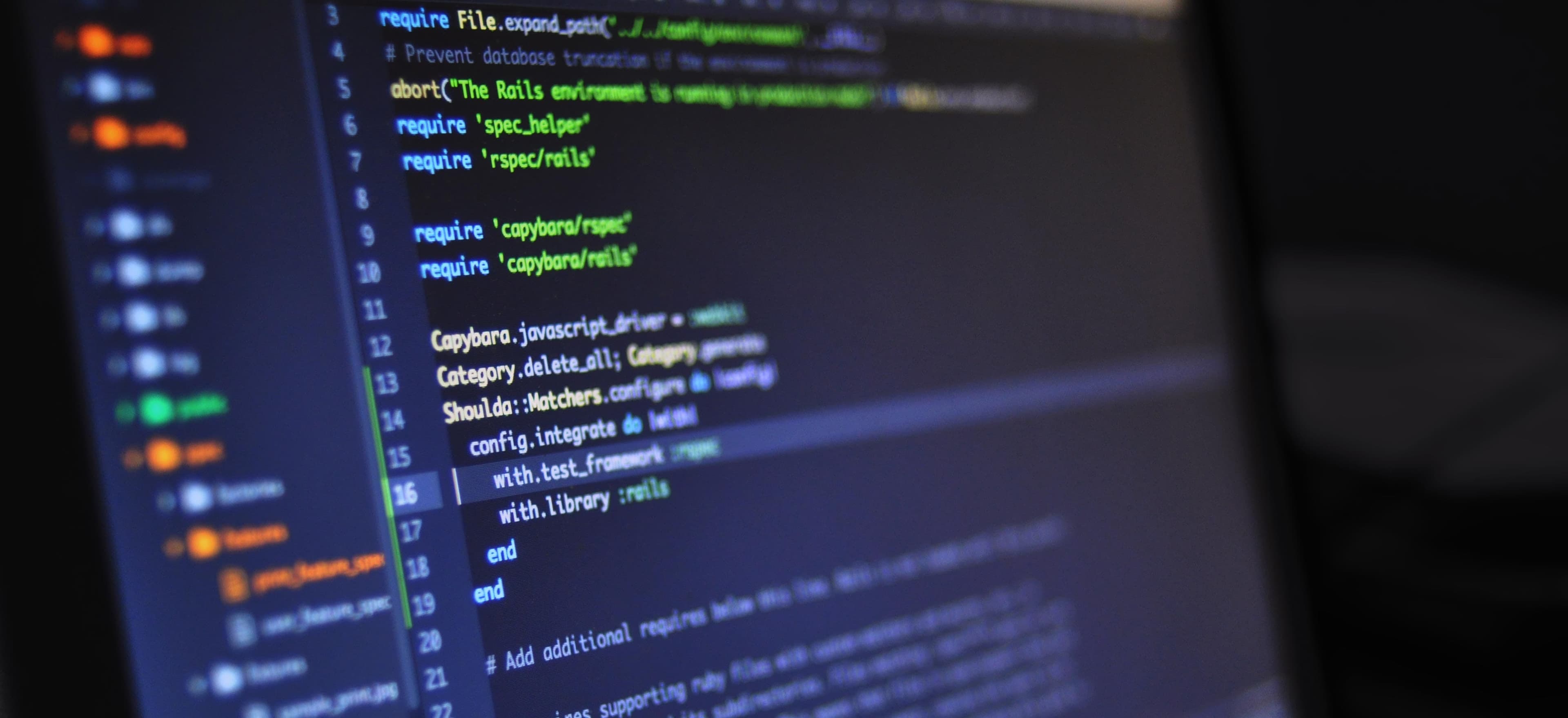
- Published on
The Pitfalls of Java's Verbosity: Is it Worth It?
Java has earned its reputation as a cornerstone of the programming world, powering vast applications and systems. However, a common critique is its verbosity. Developers can often find themselves asking: “Is the verbose nature of Java worth it?” In this blog post, we’ll explore the reasons behind Java’s verbosity, its potential pitfalls, and whether its advantages truly outweigh its drawbacks.
Understanding Verbiage in Java
Java’s syntax is often viewed as verbose when compared to more concise languages like Python or JavaScript. A simple program that prints "Hello, World!" can turn into a multi-line affair in Java:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
In contrast, here is how it looks in Python:
print("Hello, World!")
While this may seem excessive, the reasons for Java's verbosity can be linked to its strong typing, object-oriented nature, and the desire for readability and maintainability.
The Case for Verbosity
-
Type Safety: Java's static type system is a blessing for large codebases. It catches errors at compile-time rather than run-time, which ultimately leads to more reliable applications. Explicitly defined types reduce ambiguity.
String message = "Hello, World!"; int number = 42;
By specifying types, developers have a clearer understanding of the data structures they work with, preventing unintended type-related errors.
-
Clear Intent: Verbosity enhances code readability. It makes the code's purpose clear to both the original developer and future maintainers. Consider the following snippet:
List<String> names = new ArrayList<>(); names.add("Alice"); names.add("Bob");
Although it takes more lines than necessary, it’s immediately clear that we are working with a list of strings. This explicitness fosters understanding and collaboration among teams.
-
Robust Documentation: Java has a well-structured documentation system. Using Javadoc, developers can generate comprehensive documentation from inline comments. Each method's purpose can be articulated clearly, resulting in high-quality documentation.
/** * Adds two integers and returns the sum. * @param a First integer * @param b Second integer * @return Sum of a and b */ public int add(int a, int b) { return a + b; }
-
Design Patterns: Java's verbosity encourages the use of established design patterns. These patterns rely on explicitness and often require boilerplate code. Patterns like Singleton and Factory design are prevalent in Java development due to their utility in solving common problems.
The Pitfalls of Verbosity
Yet, verbosity comes with its own set of challenges:
-
Boilerplate Code: The repetitive structure can lead to a large amount of boilerplate code. This refers to sections of code that have little to no variation from one instance to another. Consider how tedious it becomes to define classes that require getters and setters.
public class User { private String name; public String getName() { return name; } public void setName(String name) { this.name = name; } }
This could easily be simplified, yet Java lacks first-class support for features like properties found in languages such as Kotlin or C#.
-
Verbose API Calls: When using third-party libraries, developers may experience an excessive amount of boilerplate due to verbose Java APIs. For instance, using JDBC often requires handling numerous classes for simple database operations.
Connection connection = DriverManager.getConnection(url, user, password); Statement statement = connection.createStatement(); ResultSet resultSet = statement.executeQuery("SELECT * FROM users");
Here, key actions (like querying) involve many layers of setup — a stark contrast to the simplicity you might find in languages with more concise database interaction methods.
-
Learning Curve: New developers may find Java’s verbosity overwhelming. Understanding the multiple components at play requires a more significant time investment compared to learning simpler languages. The sheer volume of syntactical rules can be daunting.
Is It Worth It?
So, is the verbosity of Java worth the trade-off? The answer is not black and white. It depends on the context and requirements of the project:
-
Project Size and Complexity: In large applications, verbosity can become an asset. The added explicitness aids in maintaining large codebases, where understanding the complete data flow is vital.
-
Collaborative Development: When many individuals contribute to a project, readability becomes paramount. Java’s explicitness promotes easier collaboration and integration of different modules of code from various developers.
-
Community and Ecosystem: Java boasts a well-established ecosystem. Libraries, frameworks, and tools are plenty and built with a philosophy that leans towards stability and robustness—with verbosity often being a key aspect of this structure.
-
Transitioning to Modern Practices: Although Java remains verbose, newer language features introduced in recent versions (like lambdas, streams, and the var keyword) have alleviated some of that burden. For instance, the following code uses lambdas for a cleaner approach to processing collections:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie"); names.forEach(name -> System.out.println(name));
This gives a glimpse of how Java is adapting to modern programming needs while still carrying its legacy of being explicit.
Final Considerations
The verbosity of Java can indeed be seen as a pitfall, but it is also a foundational component of what makes Java unique. The trade-offs between readability, maintainability, and conciseness depend on the application and teamwork involved. As developers, being mindful of these characteristics helps us weigh the benefits against the inconveniences.
If you want to delve deeper into the strengths of Java or how to make your code cleaner and more efficient, check out Oracle’s Java Tutorials or browse community discussions on platforms like Stack Overflow for varying perspectives.
Ultimately, understanding Java's verbosity allows developers to choose strategies to harness its capabilities while mitigating its downsides effectively. The journey of mastering it is as enlightening as it is complex.