Struggling with Log Chaos in Microservices? Here's the Fix!
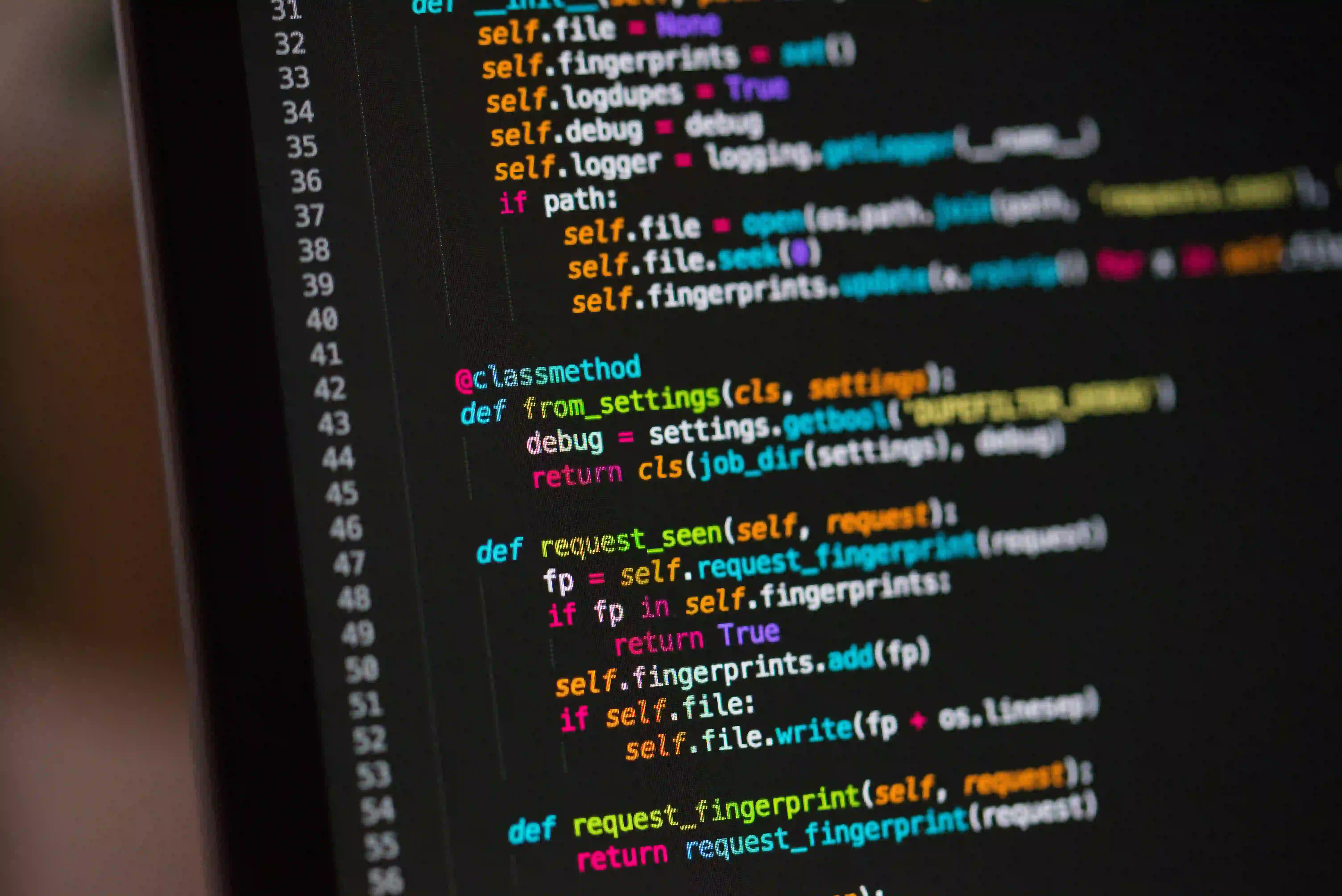
Struggling with Log Chaos in Microservices? Here's the Fix!
In the world of microservices architecture, logging plays a crucial role in maintaining system health, ensuring observability, and aiding debugging. As the number of microservices increases, the challenge of managing and interpreting logs can quickly escalate into chaos. In this blog post, we’ll explore effective strategies and tools to eliminate log chaos in microservices, ultimately leading to improved management and better insights.
Understanding Log Chaos
Log chaos refers to the overwhelming amount of logging generated by numerous microservices, often resulting in scattered data that is difficult to aggregate, analyze, and make sense of. Without proper logging practices, developers can find it challenging to understand system behavior, which can lead to increased downtime, slower response times, and poor user experience.
Why Proper Logging is Crucial
- Debugging: When an error occurs, logs serve as the first line of defense in identifying the issue.
- Performance Monitoring: Logs help track system performance metrics over time.
- Security Auditing: Logs can be utilized to trace security incidents, identifying what happened and when.
With that dynamism comes complexity. Therefore, implementing structured logging is essential to combat log chaos.
Structured Logging: A Game-Changer
What is Structured Logging?
Structured logging means creating logs in a consistent, structured format, often in JSON or XML, which can then be parsed easily. This allows you to include key-value pairs in log entries, making it easier to filter, search, and analyze.
Example of Structured Logging
Consider a simple microservice that logs user registration details:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class UserController {
private static final Logger logger = LoggerFactory.getLogger(UserController.class);
@PostMapping("/register")
public void registerUser(@RequestBody User user) {
logger.info("User registration event",
Map.of("username", user.getUsername(),
"email", user.getEmail(),
"timestamp", System.currentTimeMillis()));
// Registration logic goes here
}
}
Why Structured Logging?
- Enhanced Searchability: Instead of sifting through unformatted text, you can filter logs based on keys.
- Improved Analysis: Tools can easily parse structured logs, allowing for insightful dashboards and alerts.
- Consistency: Makes it easy to standardize logging across multiple microservices.
Centralized Logging: The Next Step
Centralized logging involves aggregating logs from all microservices into a single location for easier management and analysis. Without centralization, developers often struggle to get a complete view of the system’s health.
Tools for Centralized Logging
-
ELK Stack (Elasticsearch, Logstash, and Kibana): The ELK Stack is a popular choice for centralized logging.
- Elasticsearch provides a powerful search engine.
- Logstash helps in parsing and transforming logs.
- Kibana offers a graphical interface to visualize logs.
-
Fluentd: This is another open-source data collector for unified logging.
-
Prometheus and Grafana: While these tools are often associated with metrics, they can also be useful in aggregating logs.
Implementing the ELK Stack
To set up the ELK Stack for your microservices, follow these steps:
-
Install Elasticsearch and Kibana.
🔧snippet.sh# For Ubuntu sudo apt-get install elasticsearch sudo apt-get install kibana
-
Set up Logstash to collect logs.
Example
logstash.conf
:📄snippet.txtinput { file { path => "/var/log/myapp/*.log" start_position => "beginning" } } output { elasticsearch { hosts => ["localhost:9200"] } }
-
Run Logstash to start collecting logs.
🔧snippet.shbin/logstash -f logstash.conf
-
Access Kibana at
http://localhost:5601
to visualize your logs.
Why Use the ELK Stack?
- Scalability: Easily handle large volumes of logs.
- Ease of Use: Powerful search capabilities enable quick identification of issues.
- Rich Visualization: Kibana's dashboards help in understanding system behavior at a glance.
Log Retention Policies
Having logs indefinitely can lead to increased storage costs and complexity. Defining log retention policies is integral for a healthy logging strategy.
Suggested Retention Policy
- Error Logs: Retain for 90 days.
- Warning Logs: Retain for 30 days.
- Info Logs: Retain for 7 days.
This approach ensures that you have enough historical data for troubleshooting while managing storage effectively.
Monitoring and Alerting
Logs can also be instrumental in monitoring the health of your microservices. Create alerts based on log entries for faster response times.
Using ELK for Alerts
Using Kibana, you can set alerts based on log patterns. For instance, you can alert on specific error codes or unexpected behavior.
Why Monitor Logs?
- Proactive Issue Resolution: Don’t wait for your users to report a problem.
- System Health Insights: Understanding trends over time can help you make informed decisions.
Closing Remarks
Log chaos in microservices is a challenge that can be overcome with the right strategies. By implementing structured logging, utilizing centralized logging solutions like the ELK Stack, and developing a robust log retention policy, you can significantly improve the clarity and utility of your logs.
Remember, logs are not just for debugging; they are an invaluable source of information for understanding and managing your systems.
Further Reading
By incorporating these practices and tools, you can take a significant stride towards eliminating log chaos, ensuring that your microservices are more manageable and reliable.
Happy Logging!