Essential Techniques for Monitoring Java EE DataSources
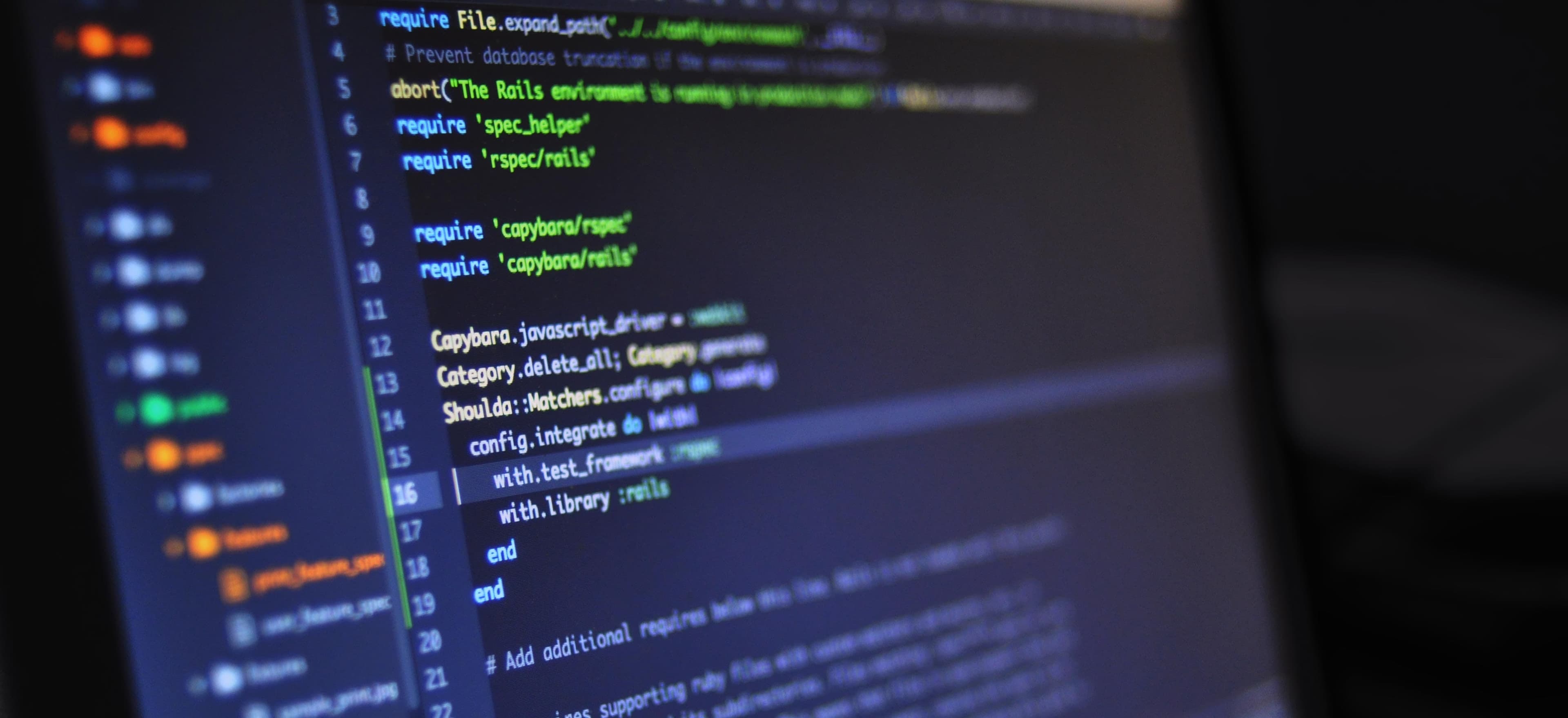
- Published on
Essential Techniques for Monitoring Java EE DataSources
In the realm of Java Enterprise Edition (Java EE), managing data connections efficiently is not just a best practice; it is vital for application performance and stability. While Java EE facilitates the creation and management of DataSources, monitoring them effectively can be a daunting task. In this blog post, we will discuss essential techniques for monitoring Java EE DataSources, providing code snippets and insights that can aid developers in optimizing database interactions.
Understanding DataSources in Java EE
A DataSource is an interface provided by JDBC (Java Database Connectivity) that enables Java applications to connect to a database. Unlike DriverManager, DataSources are more flexible and can accommodate connection pooling, which improves the application's performance by reusing existing connections instead of creating new ones every time.
The Importance of Monitoring DataSources
Monitoring DataSources helps to:
- Optimize Performance: Identify slow queries and connection bottlenecks.
- Enhance Security: Track unauthorized access attempts or suspicious activities.
- Prevent Resource Leaks: Ensure connections are closed appropriately, preventing memory leaks.
Key Metrics to Monitor
Before jumping into the implementation of monitoring techniques, it is essential to identify key metrics to keep an eye on:
- Active Connections: The number of active connections currently in use.
- Max Connections: The maximum number of connections allowed, which helps avoid throttling.
- Connection Wait Time: How long requests wait for a connection.
- Connection Leaks: Unused connections that are not returned to the pool.
Techniques for Monitoring Java EE DataSources
1. Using JMX (Java Management Extensions)
JMX provides a powerful way to monitor Java applications. It allows you to manage and monitor resources ranging from applications to devices.
Setting Up JMX for Monitoring DataSources
First, ensure that your application server supports JMX. Most leading Java EE application servers, such as WildFly, GlassFish, and TomEE, support JMX.
Here’s a sample code snippet to expose a monitoring MBean:
import javax.management.MBeanServer;
import javax.management.ObjectName;
import java.lang.management.ManagementFactory;
public class DataSourceMonitor {
private static int activeConnections;
private static int maxConnections;
// This method simulates monitoring connection pool metrics
public void monitor() {
// Assuming there's logic to update activeConnections and maxConnections.
}
public int getActiveConnections() {
return activeConnections;
}
public int getMaxConnections() {
return maxConnections;
}
// Register the MBean
public static void registerMBean() {
try {
MBeanServer mbs = ManagementFactory.getPlatformMBeanServer();
ObjectName name = new ObjectName("com.example:type=DataSourceMonitor");
DataSourceMonitor monitor = new DataSourceMonitor();
mbs.registerMBean(monitor, name);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Advantages of Using JMX
- Real-time monitoring.
- Built-in support in many Java EE servers.
- Easy integration with monitoring tools like JConsole or VisualVM.
2. Using Application Performance Monitoring (APM) Tools
APM tools like New Relic, Dynatrace, and AppDynamics can provide deep insights into your Java EE application, including DataSource metrics. These tools often require minimal setup and offer a wide range of features necessary for production environments.
Example of Integration with New Relic
To integrate New Relic with your Java EE application, follow these steps:
- Download the New Relic Java agent.
- Add it to your Java application’s classpath.
- Configure it using the
newrelic.yml
file.
common: &default_settings
license_key: 'YOUR_NEW_RELIC_LICENSE_KEY'
app_name: 'Your Java EE Application'
# Other configuration settings...
production:
<<: *default_settings
Benefits of APM Tools
- Comprehensive monitoring dashboard.
- Alerts and notifications based on predefined thresholds.
- Analyzes transactions across multiple layers, including the database.
3. Implementing Custom Connection Pool Metrics
If you are managing your DataSource connections manually or through a custom implementation, you can add metrics logging.
Here is an example of how to implement custom connection pool metrics:
import java.sql.Connection;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
public class CustomConnectionPool {
private List<Connection> pool;
private int maxPoolSize;
public CustomConnectionPool(int size) {
this.pool = new ArrayList<>(size);
this.maxPoolSize = size;
// Logic to initialize connections...
}
public synchronized Connection getConnection() throws SQLException {
if (pool.isEmpty()) {
if (pool.size() < maxPoolSize) {
// Logic to create new connection
} else {
// Connection wait strategy (this is where you monitor wait time)
}
}
return pool.remove(pool.size() - 1);
}
public synchronized void returnConnection(Connection connection) {
pool.add(connection);
}
public int getActiveConnections() {
return maxPoolSize - pool.size();
}
}
Why Custom Metrics?
Custom metrics allow you to tailor monitoring to your specific needs. You can adjust thresholds and performance indicators based on your application’s requirements.
4. Logging and Performance Metrics
Integrate logging frameworks like SLF4J or Log4j to capture connection-related metrics at runtime. This is critical for diagnosing issues post-mortem.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class ConnectionLogger {
private static final Logger logger = LoggerFactory.getLogger(ConnectionLogger.class);
public void logConnectionDetails(Connection connection) {
try {
// Log connection details like creation time, etc.
logger.info("Connection created at: {}", connection.getMetaData().getCreationTime());
} catch (SQLException e) {
logger.error("Error logging connection details", e);
}
}
}
Advantages of Logging
- Detailed historical data for troubleshooting.
- Allows you to pinpoint times of high load.
- Easy integration with monitoring systems for alerting.
Closing the Chapter
Monitoring Java EE DataSources is integral to ensuring responsive and reliable applications. Through the use of JMX, APM tools, custom metrics, and logging, developers can gain deep insights into their application's database interactions, leading to enhanced performance and reduced downtime.
As you integrate these monitoring techniques into your Java EE applications, remember to focus on your specific use cases and metrics that resonate with your application’s performance indicators.
For further reading on JDBC and DataSource configurations, you might find these resources helpful:
- Java EE Documentation
- JMX Tutorial
Happy coding and monitoring!