Easily Accessing First and Last Array Elements in Java
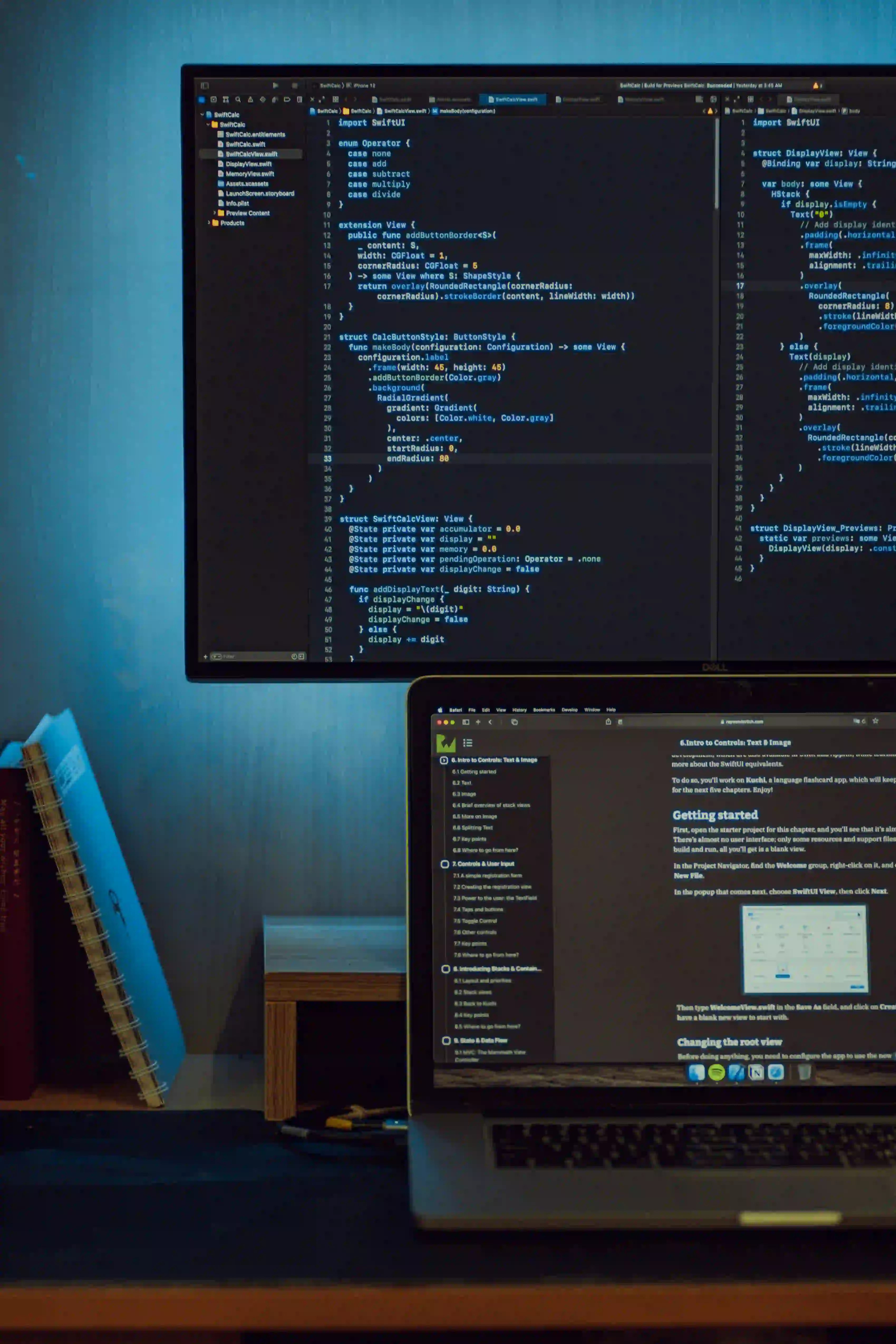
Easily Accessing First and Last Array Elements in Java
Java arrays are a fundamental data structure that allows you to store multiple values of the same type in a single variable. Accessing elements in an array is straightforward, but understanding the best practices can enhance your coding efficiency. In this blog post, we'll cover how to easily access the first and last elements of an array in Java, with clear examples and explanations.
Table of Contents
- Introduction to Java Arrays
- Accessing the First Element
- Accessing the Last Element
- Example Code Snippets
- Best Practices
- Conclusion
The Roadmap to Java Arrays
An array in Java is a collection of elements identified by index or key. The array is a reference type, meaning it holds the memory address of the actual data. The syntax for creating an array is as follows:
dataType[] arrayName = new dataType[arraySize];
For example:
int[] numbers = new int[5]; // An integer array of size 5
In this post, we’ll focus on accessing the first (index 0
) and last (index length-1
) elements of an array.
Accessing the First Element
To access the first element in a Java array, you simply refer to the index 0
of the array.
Example:
public class FirstElementExample {
public static void main(String[] args) {
int[] numbers = {10, 20, 30, 40, 50};
// Accessing the first element
int firstElement = numbers[0];
System.out.println("The first element is: " + firstElement);
}
}
Why it Works:
-
Zero-based Indexing: Java arrays are zero-indexed. The first element is always at index
0
, making it quick and easy to retrieve. -
Performance: Accessing an element by its index in an array has a time complexity of O(1), meaning it takes constant time regardless of the size of the array.
Accessing the Last Element
To access the last element in an array, you use the length of the array minus one.
Example:
public class LastElementExample {
public static void main(String[] args) {
int[] numbers = {10, 20, 30, 40, 50};
// Accessing the last element
int lastElement = numbers[numbers.length - 1];
System.out.println("The last element is: " + lastElement);
}
}
Why it Works:
-
Dynamic Length: The
length
property of the array gives you the total number of elements in the array. Subtracting1
provides you with the index of the last element. -
Uniform Access Time: As with the first element, accessing the last element is also O(1).
Example Code Snippets
To further illustrate these concepts, let’s look at a complete Java program that combines both first and last element access.
Complete Example:
public class ArrayAccessExample {
public static void main(String[] args) {
String[] fruits = {"Apple", "Banana", "Cherry", "Date", "Elderberry"};
// Accessing the first and last elements
String firstFruit = fruits[0];
String lastFruit = fruits[fruits.length - 1];
// Outputting the results
System.out.println("The first fruit is: " + firstFruit);
System.out.println("The last fruit is: " + lastFruit);
}
}
Output:
The first fruit is: Apple
The last fruit is: Elderberry
Best Practices
While accessing the first and last elements of arrays is simple, there are best practices to keep in mind:
-
Check for Empty Arrays: Always ensure the array is not empty before trying to access elements. Attempting to access elements in an empty array will cause an
ArrayIndexOutOfBoundsException
.☕snippet.javaif (fruits.length > 0) { String firstFruit = fruits[0]; String lastFruit = fruits[fruits.length - 1]; }
-
Use Enhanced For Loop for Iteration: While accessing the first and last elements is efficient, when iterating through an array, consider using an enhanced for loop for better readability.
☕snippet.javafor (String fruit : fruits) { System.out.println(fruit); }
-
Consider ArrayList for Dynamic Arrays: If your application requires frequent resizing, consider using
ArrayList
instead of a traditional array. It offers built-in methods to manage dynamic lists. -
Memory Management: Remember that arrays are fixed in size. If you find yourself needing variable-length data structures, consider using collections from the Java Collections Framework.
Wrapping Up
Accessing the first and last elements of an array in Java is a simple yet crucial task for any Java developer. Understanding the indexing mechanism allows for quick data retrieval, while best practices ensure your code remains robust and maintainable.
If you want to dive deeper into Java arrays, check out resources on Java SE Documentation or Effective Java by Joshua Bloch for best practices in Java programming.
By mastering these techniques, you will enhance the efficiency and effectiveness of your Java coding practices. Happy coding!