Common JAXB Unmarshal Issues with Namespaces Explained
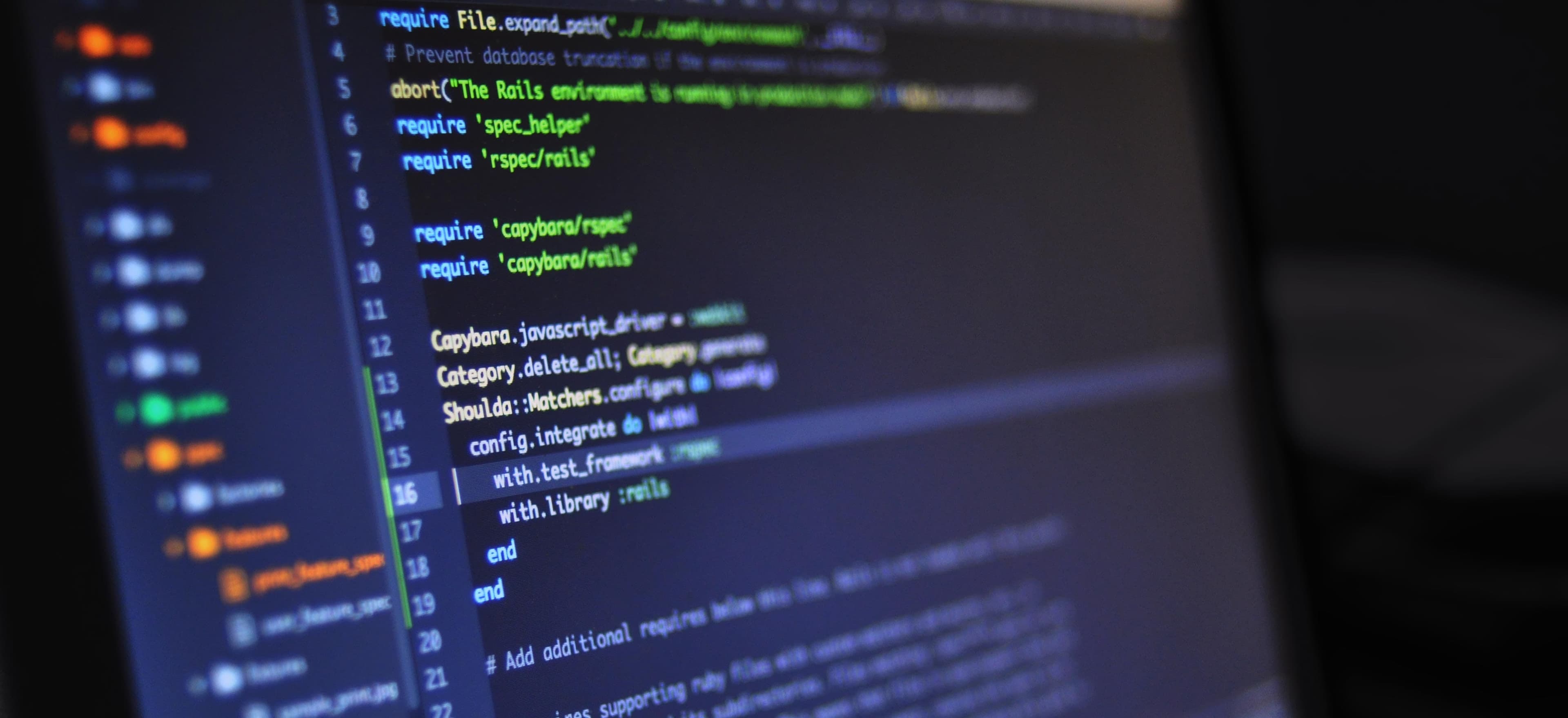
- Published on
Common JAXB Unmarshal Issues with Namespaces Explained
Java Architecture for XML Binding (JAXB) provides a convenient way to map Java classes to XML representations and back again. It simplifies the process of reading and writing XML data, but like any technology, it comes with its own set of challenges. One of the common pitfalls developers face when using JAXB is handling namespaces during the unmarshalling process. In this post, we will explore these issues and provide insight, tips, and code examples to help you navigate them effectively.
Understanding JAXB and Namespaces
Before diving into the issues, it's essential to understand what JAXB and XML namespaces are. JAXB allows you to convert XML into Java objects (unmarshalling) and vice versa (marshalling). XML namespaces are used to avoid naming conflicts by qualifying names of elements and attributes. They are often declared in XML documents like so:
<note xmlns:ex="http://example.com/">
<ex:to>Tove</ex:to>
<ex:from>Jani</ex:from>
<ex:heading>Reminder</ex:heading>
<ex:body>Don't forget me this weekend!</ex:body>
</note>
The xmlns:ex
declaration establishes a namespace, allowing elements prefixed by ex:
to be uniquely identified.
Examples of Unmarshal Issues with Namespaces
-
Namespace Mismatch
One common issue occurs when the namespace defined in the XML does not match the namespace expected in the JAXB-annotated Java classes.
Problem Example: Suppose you have a JAXB-annotated class expecting elements to belong to the namespace
http://example.com/note
, but the XML declareshttp://example.com/
.This mismatch will lead to JAXB throwing an
UnmarshalException
since it cannot find the expected namespace.Solution: Ensure that your JAXB-annotated classes use the correct namespace.
@XmlRootElement(namespace = "http://example.com/note") public class Note { private String to; private String from; // Getters and Setters }
-
Inconsistent Namespaces
Another issue arises when the XML document contains elements with inconsistent namespaces. For instance:
<note xmlns:ex="http://example.com/"> <ex:to>Tove</ex:to> <from>Jani</from> <!-- No namespace --> </note>
Here, the
from
element uses no namespace whileto
is correctly namespaced. This inconsistency can lead to data loss or unexpected behavior during unmarshalling.Highlight: JAXB will NOT serialize or deserialize elements that do not match the expected namespace, leading to potentially incomplete objects.
Solution: Ensure all elements are declared with the intended namespace. If your Java class is already set up to handle a certain structure, modify the XML to align with it.
-
Default Namespace Handling
JAXB also expects namespaces to be explicitly defined in some cases. If your XML uses a default namespace but you've only defined namespaces for individual elements, you may run into issues.
Problem Example:
<note xmlns="http://example.com/note"> <to>Tove</to> <from>Jani</from> </note>
In this example, both
to
andfrom
are under the default namespace, but if your JAXB class is not set up for this, you will encounter unmarshalling problems.Solution: Ensure that your JAXB classes define their namespaces correctly. You can adjust your classes like this:
@XmlRootElement(namespace = "http://example.com/note") @XmlAccessorType(XmlAccessType.FIELD) public class Note { @XmlElement(namespace = "http://example.com/note") private String to; @XmlElement(namespace = "http://example.com/note") private String from; // Getters and Setters }
-
Elements in Different Namespaces
When an XML document contains nested elements in different namespaces, unmarshalling can quickly become complex.
Problem Example: Consider an XML document with nested elements where one level is namespaced:
<note xmlns:ex="http://example.com/"> <ex:to>Tove</ex:to> <ex:from>Jani</ex:from> <message xmlns:msg="http://example.com/message">Hello!</message> <!-- Different Namespace --> </note>
Here, if your JAXB classes are not handling the
msg
namespace correctly, it will lead to failure in retrieving data from the XML.Solution: Define the appropriate namespace in your Java class with additional annotations:
@XmlRootElement(namespace = "http://example.com/note") public class Note { @XmlElement(namespace = "http://example.com/") private String to; @XmlElement(namespace = "http://example.com/") private String from; @XmlElement(namespace = "http://example.com/message") private String message; // Getters and Setters }
-
Avoiding Context Switching Errors
Context-switching errors occur when JAXB is given different contexts for unmarshalling. This can arise if you have multiple classes that correspond to different XML structures but share the same namespace.
Problem Example: If you are trying to unmarshal two different types of notes, but both are in
http://example.com/note
, it can lead to confusion for JAXB.Solution: Be explicit about your namespaces, using distinct ones for different classes. You can also use JAXB's
@XmlSeeAlso
to help JAXB understand the possible classes.@XmlSeeAlso({NoteType1.class, NoteType2.class}) @XmlRootElement(namespace = "http://example.com/note") public abstract class BaseNote { // Common properties }
Closing the Chapter
JAXB is a powerful tool for working with XML in Java, but as we've seen, it can come with nuanced challenges, especially when dealing with namespaces. Understanding how JAXB handles namespaces can help you avoid issues related to unmarshalling and ensure that your application correctly processes XML data.
By carefully managing namespaces in both XML documents and JAXB-annotated classes, you can significantly reduce the complexity and improve the reliability of your XML processing.
For more detailed resources on JAXB and XML namespaces, consider visiting Oracle's official JAXB documentation.
Additional Reading
- XML Namespaces - W3C
- Java XML Binding (JAXB) Tutorials
With this knowledge in hand, you're now equipped to tackle JAXB namespace issues with confidence! Happy coding!