Why Ignoring JPA Persistence Exceptions Can Lead to Data Loss
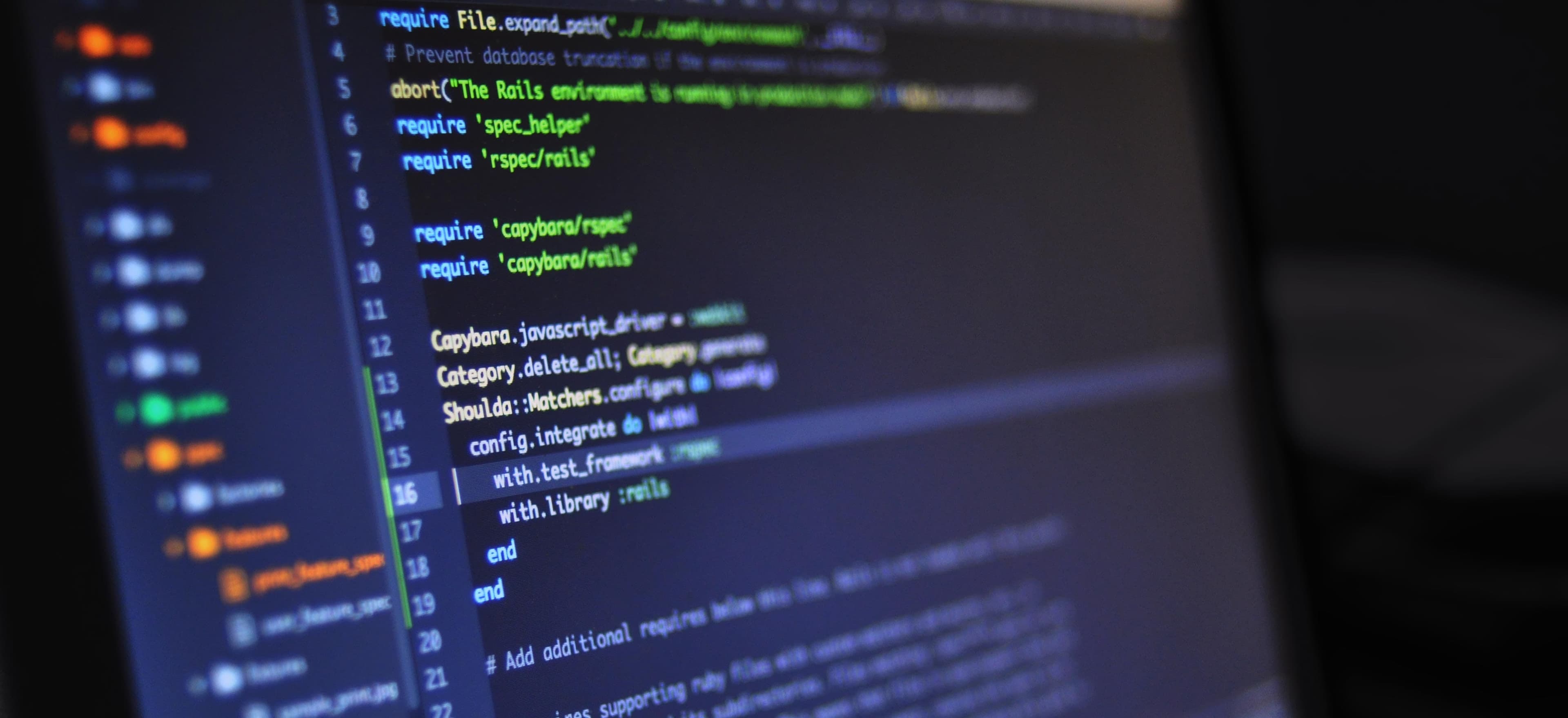
- Published on
Why Ignoring JPA Persistence Exceptions Can Lead to Data Loss
Java Persistence API (JPA) is a powerful framework for managing relational data in Java applications. While it's convenient and often straightforward to use, it's crucial to be vigilant about how you handle exceptions associated with persistence operations. Ignoring these exceptions can lead to unexpected behavior and, most importantly, data loss. In this blog post, we will delve into JPA persistence exceptions, why ignoring them can be detrimental, and how to manage these exceptions effectively.
Understanding JPA Persistence Exceptions
JPA provides a framework for interacting with databases through a consistent programming model. However, when working with databases, numerous factors can potentially trigger exceptions during persistence operations. Common examples include:
- Entity validation failures
- Connection issues
- Transaction failures
The most common JPA exceptions include:
- EntityExistsException: Thrown when an attempt is made to persist an entity that already exists in the database.
- PersistenceException: A generic error for various persistence-related issues.
- OptimisticLockException: Encountered when an attempt is made to update an entity that has been modified since it was last retrieved.
Code Example: Handling JPA Persistence Exceptions
To understand the ramifications of ignoring these exceptions, let’s look at a simple example of persisting an entity:
EntityManager em = entityManagerFactory.createEntityManager();
em.getTransaction().begin();
try {
MyEntity entity = new MyEntity();
entity.setName("Sample Data");
em.persist(entity);
em.getTransaction().commit();
} catch (EntityExistsException e) {
System.err.println("Entity already exists: " + e.getMessage());
em.getTransaction().rollback();
} catch (PersistenceException e) {
System.err.println("Persistence error: " + e.getMessage());
em.getTransaction().rollback();
} finally {
em.close();
}
Explanation of the Code
In this example:
- We create an
EntityManager
and begin a transaction. - We attempt to persist a new entity named
MyEntity
. - The
try-catch
block captures various persistence exceptions. - If an exception occurs, we log it and roll back the transaction to maintain data integrity.
- The
finally
block ensures that theEntityManager
closes regardless of success or failure.
This pattern is paramount because it prevents partial updates, which could lead to data anomalies.
The Dangers of Ignoring Persistence Exceptions
Ignoring JPA persistence exceptions could lead to several critical issues:
1. Data Corruption
If you overlook exceptions, it’s easy to end up with a corrupted database state. For instance, if an EntityExistsException
is thrown and not handled, your application might assume data was successfully persisted, while in reality, the entity was never stored in the database. By not checking for exceptions, you're risking the integrity of your data.
2. Silent Failures
When exceptions are ignored, your application can manifest silent failures. This means that while the application runs without crashing, it behaves incorrectly. Data could be missing or incorrectly displayed, leading to confusion and loss of trust in the application.
3. Resource Leaks
Failing to handle exceptions appropriately can lead to resource leaks, such as unclosed connections or stale transactions. This not only consumes unnecessary resources but can also degrade the performance of your application.
4. Performance Implications
Ignoring exceptions can also mask underlying performance issues. For example, if a transaction fails due to a database lock, continued attempts to execute other operations can cause cascading failures, which results in performance degradation.
Best Practices for Handling Exceptions in JPA
To avoid the crucible of data loss resulting from unhandled persistence exceptions, here are some best practices you should consider:
1. Always Catch Specific Exceptions
Instead of catching a generic Exception
, focus on catching specific JPA exceptions. This allows you to handle different failure scenarios appropriately.
2. Use Transaction Management
Utilize @Transactional
annotations wherever possible. This helps ensure that each transaction is either fully completed or fully rolled back, maintaining data consistency.
3. Log Exception Details
Logging is essential for understanding what went wrong. Ensure you document various persistence exceptions with relevant details to facilitate troubleshooting.
catch (EntityExistsException e) {
logger.error("Attempted to persist existing entity: " + entity, e);
em.getTransaction().rollback();
} catch (OptimisticLockException e) {
logger.warn("Optimistic locking failure occurred.", e);
em.getTransaction().rollback();
}
4. Implementing Central Error Handling
Consider implementing a central error-handling mechanism for JPA operations. This could be a service class that handles persistence across your application and centralizes the logging and exception management.
5. Testing for Edge Cases
Conduct extensive testing, especially for edge cases that could lead to exceptions. Use unit and integration tests to simulate faulty scenarios and ensure that your application handles them gracefully.
The Bottom Line: The Importance of Proper Exception Management
Ignoring JPA persistence exceptions is not trivial; it can lead to serious data loss, corruption, and various performance issues. Understanding how to handle these exceptions proactively is not just a good practice, it is essential for the robustness and reliability of your applications.
By following the best practices for handling JPA persistence exceptions outlined in this blog post, you can significantly reduce the risk of data loss while ensuring that your application maintains its integrity and performance. Always remember, in the realm of data persistence, vigilance is your best ally.
For more insights into managing JPA effectively, consider checking out Baeldung's guide to JPA and Oracle's JPA documentation.
By prioritizing exception handling, you ensure that your application remains robust and trustworthy while managing data efficiently. Happy coding!