Challenges of Implementing MVC in Jakarta Framework
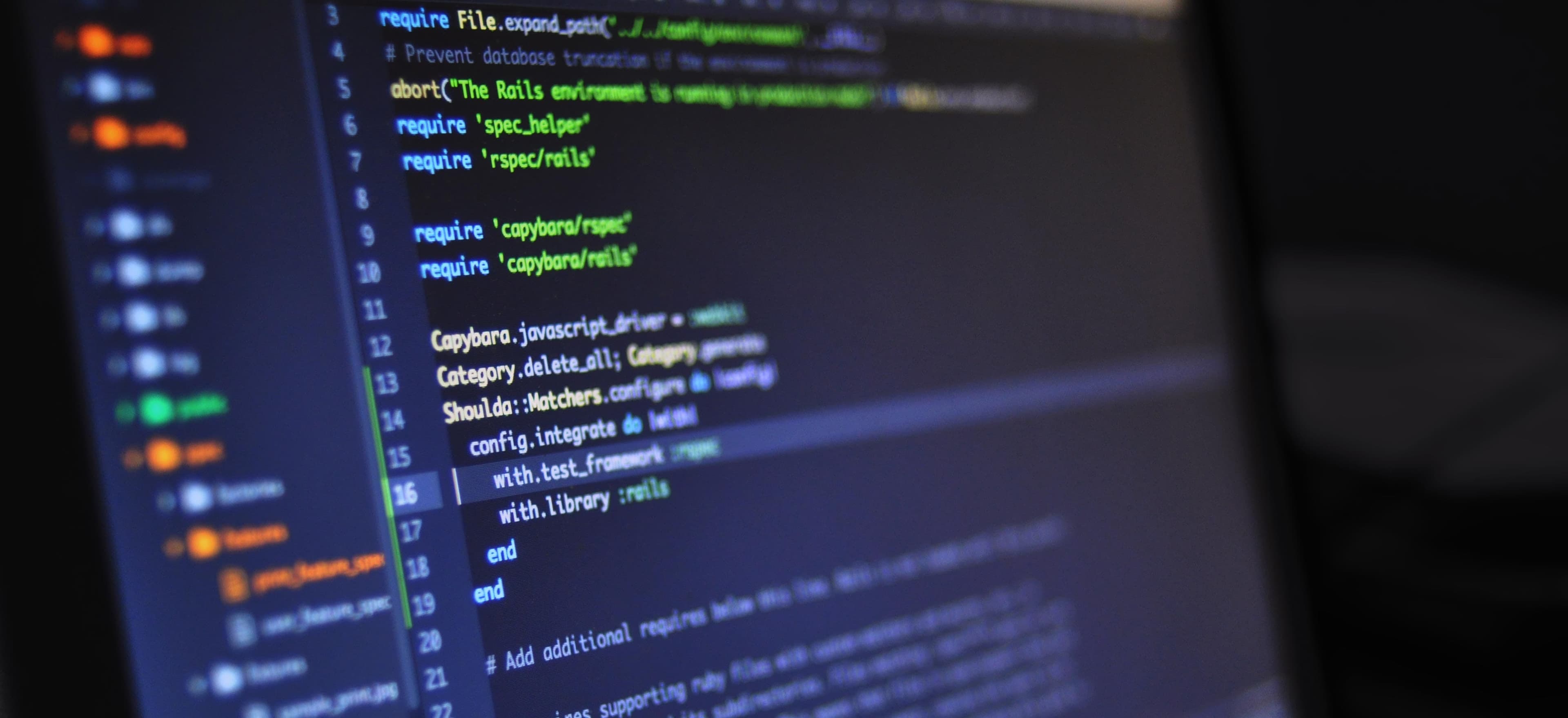
- Published on
Challenges of Implementing MVC in Jakarta Framework
The Model-View-Controller (MVC) architectural pattern is one of the most commonly used paradigms in web application development. The Jakarta Framework, a successor to Java EE, provides robust capabilities for building enterprise-level applications. Despite its strengths, implementing MVC in this framework is not without its challenges. In this blog post, we'll dive deep into these challenges while offering solutions and workarounds to ensure a smooth development process.
Understanding MVC in Jakarta Framework
Before addressing the challenges, let's briefly discuss what MVC entails.
- Model: The part of the application responsible for the data and business logic.
- View: The user interface that displays the data.
- Controller: The mediator that handles user input and interacts with the Model and View.
In Jakarta EE, MVC can be implemented using various technologies and libraries such as Jakarta Servlet, Jakarta REST, and Jakarta Faces. However, despite its extensive capabilities, developers may encounter the following challenges.
1. Complexity in Library Integration
One common challenge is integrating third-party libraries. While Jakarta provides built-in features, many developers rely on external libraries for functionalities such as user authentication or data access.
Solution:
Use dependency management tools like Maven or Gradle. They allow you to define all the dependencies your application requires. For example, you could add the following dependency in your pom.xml
for using Spring Security for authentication:
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-core</artifactId>
<version>5.6.0</version>
</dependency>
This ensures that you have a consistent version across different environments and simplifies the integration process.
2. Maintaining Separation of Concerns
One of the foundational principles of MVC is to maintain a clean separation of concerns. However, developers often struggle with this, leading to tightly coupled components that are hard to maintain.
Solution:
Utilize Jakarta Annotations effectively to define your components. For example, use @Inject
to manage dependencies rather than manually instantiating classes:
public class UserController {
@Inject
private UserService userService;
public void registerUser(User user) {
userService.save(user);
}
}
This promotes loose coupling and enhances testability, allowing you to focus on one aspect of your application at a time.
3. Navigating URL Mapping
Mapping URLs to their corresponding controllers can be tricky, particularly when the application expands and the number of routes increases. Confusion over which handler corresponds to a given URL may arise, leading to routing issues.
Solution:
Leverage Jakarta's annotations like @Path
for RESTful services. This makes your routing explicit. For example:
@Path("/users")
public class UserController {
@GET
@Path("/{id}")
public User getUser(@PathParam("id") Long id) {
return userService.findById(id);
}
}
By clearly defining routes, you reduce the chances of misrouting and create a more intuitive API structure.
4. Testing Challenges
Testing MVC applications presents another hurdle. Ensuring that all components like the Model, View, and Controller function correctly—both in isolation and as a whole—is vital yet daunting.
Solution:
Implement unit tests and integration tests using JUnit and Mockito. For example:
public class UserControllerTest {
@Mock
private UserService userService;
@InjectMocks
private UserController userController;
@Test
public void testRegisterUser() {
User user = new User("john.doe@example.com");
userController.registerUser(user);
verify(userService).save(user);
}
}
Using mocks allows you to isolate the controller logic and ensure that it interacts correctly with the service layer.
5. Performance Overheads
As applications scale, performance can become a concern. The abstract nature of MVC can introduce layers of indirection, which might lead to latency.
Solution:
Use caching strategies at various layers. For instance, in Jakarta EE, you can opt for the built-in caching mechanisms provided through CDI (Contexts and Dependency Injection). This would look something like this:
@Singleton
@Startup
public class DataCache {
private Map<Long, User> userCache = new HashMap<>();
@Inject
private UserService userService;
@PostConstruct
public void cacheInitialData() {
List<User> users = userService.findAll();
for (User user : users) {
userCache.put(user.getId(), user);
}
}
public User getUser(Long id) {
return userCache.get(id);
}
}
This caching approach can drastically improve response times and reduce the load on your database.
6. State Management Issues
Managing state across requests can be tricky, especially in applications that need to retain user sessions. Inconsistent state management can lead to data loss or a poor user experience.
Solution:
Utilize Jakarta Sessions effectively, which provide the ability to track user data across requests seamlessly.
@SessionScoped
public class UserSession {
private User currentUser;
public void setCurrentUser(User user) {
this.currentUser = user;
}
public User getCurrentUser() {
return currentUser;
}
}
By using session-scoped beans, the application can manage state more effectively without compromising on separation of concerns.
My Closing Thoughts on the Matter
While implementing MVC in the Jakarta Framework can present its fair share of challenges, understanding these pitfalls allows developers to implement best practices that lead to clean, maintainable code. By focusing on library integration, separation of concerns, URL mapping, testing, performance, and state management, developers can produce high-quality applications.
To deepen your understanding of MVC in Java and Jakarta EE, consider exploring the following resources:
By leveraging the solutions and strategies discussed in this post, you can navigate the complexities of MVC in the Jakarta Framework with confidence and agility. Happy coding!